QP is an event-driven, RTOS-like, active object framework for microcontrollers, such as mbed. The QP framework provides thread-safe execution of active objects (concurrent state machines) and support both manual and automatic coding of UML statecharts in readable, production-quality C or C++. Automatic code generation of QP code is supported by the free QM modeling tool.
Dependents: qp_hangman qp_dpp qp_blinky
QFsm Class Reference
Finite State Machine base class. More...
#include <qp.h>
Public Member Functions | |
virtual | ~QFsm () |
virtual destructor | |
void | init (QEvt const *const e=static_cast< QEvt const * >(0)) |
Performs the second step of FSM initialization by triggering the top-most initial transition. | |
void | dispatch (QEvt const *const e) |
Dispatches an event to a FSM. | |
Protected Types | |
enum | ReservedFsmSignals { Q_ENTRY_SIG = 1, Q_EXIT_SIG } |
Protected Member Functions | |
QFsm (QStateHandler const initial) | |
Protected constructor of a FSM. | |
QStateHandler | state (void) const |
Return the current active state. | |
QState | tran (QStateHandler const target) |
internal helper function to record a state transition | |
Static Protected Member Functions | |
static QState | Q_IGNORED (void) |
Inline function to specify the return of a state-handler when it igonres (does not handle in any way) the event. | |
static QState | Q_HANDLED (void) |
Inline function to specify the return of a state-handler when it handles the event. | |
static QState | Q_UNHANDLED (void) |
Macro to specify the return of a state-handler function when it attempts to handle the event but a guard condition evaluates to false and there is no other explicit way of handling the event. |
Detailed Description
Finite State Machine base class.
QFsm represents a traditional non-hierarchical Finite State Machine (FSM) without state hierarchy, but with entry/exit actions.
QFsm is also a base structure for the QHsm class.
- Note:
- QFsm is not intended to be instantiated directly, but rather serves as the base class for derivation of state machines in the application code.
The following example illustrates how to derive a state machine class from QFsm.
Definition at line 352 of file qp.h.
Member Enumeration Documentation
enum ReservedFsmSignals [protected] |
Constructor & Destructor Documentation
virtual ~QFsm | ( | ) | [virtual] |
virtual destructor
QFsm | ( | QStateHandler const | initial ) | [protected] |
Protected constructor of a FSM.
Performs the first step of FSM initialization by assigning the initial pseudostate to the currently active state of the state machine.
- Note:
- The constructor is protected to prevent direct instantiating of QFsm objects. This class is intended for subclassing only.
Member Function Documentation
void dispatch | ( | QEvt const *const | e ) |
Dispatches an event to a FSM.
Processes one event at a time in Run-to-Completion (RTC) fashion. The argument e is a constant pointer the QEvt or a class derived from QEvt.
- Note:
- Must be called after QFsm::init().
- See also:
- example for QFsm::init()
Performs the second step of FSM initialization by triggering the top-most initial transition.
The argument e is constant pointer to QEvt or a class derived from QEvt.
- Note:
- Must be called only ONCE before QFsm::dispatch()
The following example illustrates how to initialize a FSM, and dispatch events to it:
static QState Q_HANDLED | ( | void | ) | [static, protected] |
static QState Q_IGNORED | ( | void | ) | [static, protected] |
static QState Q_UNHANDLED | ( | void | ) | [static, protected] |
QStateHandler state | ( | void | ) | const [protected] |
Generated on Wed Jul 13 2022 11:50:18 by
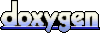