PokittoLib is the library needed for programming the Pokitto DIY game console (www.pokitto.com)
Dependents: YATTT sd_map_test cPong SnowDemo ... more
core_cm7.h
00001 /**************************************************************************//** 00002 * @file core_cm7.h 00003 * @brief CMSIS Cortex-M7 Core Peripheral Access Layer Header File 00004 * @version V4.10 00005 * @date 18. March 2015 00006 * 00007 * @note 00008 * 00009 ******************************************************************************/ 00010 /* Copyright (c) 2009 - 2015 ARM LIMITED 00011 00012 All rights reserved. 00013 Redistribution and use in source and binary forms, with or without 00014 modification, are permitted provided that the following conditions are met: 00015 - Redistributions of source code must retain the above copyright 00016 notice, this list of conditions and the following disclaimer. 00017 - Redistributions in binary form must reproduce the above copyright 00018 notice, this list of conditions and the following disclaimer in the 00019 documentation and/or other materials provided with the distribution. 00020 - Neither the name of ARM nor the names of its contributors may be used 00021 to endorse or promote products derived from this software without 00022 specific prior written permission. 00023 * 00024 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE 00027 ARE DISCLAIMED. IN NO EVENT SHALL COPYRIGHT HOLDERS AND CONTRIBUTORS BE 00028 LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR 00029 CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF 00030 SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00031 INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00032 CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 00033 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00034 POSSIBILITY OF SUCH DAMAGE. 00035 ---------------------------------------------------------------------------*/ 00036 00037 00038 #if defined ( __ICCARM__ ) 00039 #pragma system_include /* treat file as system include file for MISRA check */ 00040 #endif 00041 00042 #ifndef __CORE_CM7_H_GENERIC 00043 #define __CORE_CM7_H_GENERIC 00044 00045 #ifdef __cplusplus 00046 extern "C" { 00047 #endif 00048 00049 /** \page CMSIS_MISRA_Exceptions MISRA-C:2004 Compliance Exceptions 00050 CMSIS violates the following MISRA-C:2004 rules: 00051 00052 \li Required Rule 8.5, object/function definition in header file.<br> 00053 Function definitions in header files are used to allow 'inlining'. 00054 00055 \li Required Rule 18.4, declaration of union type or object of union type: '{...}'.<br> 00056 Unions are used for effective representation of core registers. 00057 00058 \li Advisory Rule 19.7, Function-like macro defined.<br> 00059 Function-like macros are used to allow more efficient code. 00060 */ 00061 00062 00063 /******************************************************************************* 00064 * CMSIS definitions 00065 ******************************************************************************/ 00066 /** \ingroup Cortex_M7 00067 @{ 00068 */ 00069 00070 /* CMSIS CM7 definitions */ 00071 #define __CM7_CMSIS_VERSION_MAIN (0x04) /*!< [31:16] CMSIS HAL main version */ 00072 #define __CM7_CMSIS_VERSION_SUB (0x00) /*!< [15:0] CMSIS HAL sub version */ 00073 #define __CM7_CMSIS_VERSION ((__CM7_CMSIS_VERSION_MAIN << 16) | \ 00074 __CM7_CMSIS_VERSION_SUB ) /*!< CMSIS HAL version number */ 00075 00076 #define __CORTEX_M (0x07) /*!< Cortex-M Core */ 00077 00078 00079 #if defined ( __CC_ARM ) 00080 #define __ASM __asm /*!< asm keyword for ARM Compiler */ 00081 #define __INLINE __inline /*!< inline keyword for ARM Compiler */ 00082 #define __STATIC_INLINE static __inline 00083 00084 #elif defined ( __GNUC__ ) 00085 #define __ASM __asm /*!< asm keyword for GNU Compiler */ 00086 #define __INLINE inline /*!< inline keyword for GNU Compiler */ 00087 #define __STATIC_INLINE static inline 00088 00089 #elif defined ( __ICCARM__ ) 00090 #define __ASM __asm /*!< asm keyword for IAR Compiler */ 00091 #define __INLINE inline /*!< inline keyword for IAR Compiler. Only available in High optimization mode! */ 00092 #define __STATIC_INLINE static inline 00093 00094 #elif defined ( __TMS470__ ) 00095 #define __ASM __asm /*!< asm keyword for TI CCS Compiler */ 00096 #define __STATIC_INLINE static inline 00097 00098 #elif defined ( __TASKING__ ) 00099 #define __ASM __asm /*!< asm keyword for TASKING Compiler */ 00100 #define __INLINE inline /*!< inline keyword for TASKING Compiler */ 00101 #define __STATIC_INLINE static inline 00102 00103 #elif defined ( __CSMC__ ) 00104 #define __packed 00105 #define __ASM _asm /*!< asm keyword for COSMIC Compiler */ 00106 #define __INLINE inline /*use -pc99 on compile line !< inline keyword for COSMIC Compiler */ 00107 #define __STATIC_INLINE static inline 00108 00109 #endif 00110 00111 /** __FPU_USED indicates whether an FPU is used or not. 00112 For this, __FPU_PRESENT has to be checked prior to making use of FPU specific registers and functions. 00113 */ 00114 #if defined ( __CC_ARM ) 00115 #if defined __TARGET_FPU_VFP 00116 #if (__FPU_PRESENT == 1) 00117 #define __FPU_USED 1 00118 #else 00119 #warning "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00120 #define __FPU_USED 0 00121 #endif 00122 #else 00123 #define __FPU_USED 0 00124 #endif 00125 00126 #elif defined ( __GNUC__ ) 00127 #if defined (__VFP_FP__) && !defined(__SOFTFP__) 00128 #if (__FPU_PRESENT == 1) 00129 #define __FPU_USED 1 00130 #else 00131 #warning "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00132 #define __FPU_USED 0 00133 #endif 00134 #else 00135 #define __FPU_USED 0 00136 #endif 00137 00138 #elif defined ( __ICCARM__ ) 00139 #if defined __ARMVFP__ 00140 #if (__FPU_PRESENT == 1) 00141 #define __FPU_USED 1 00142 #else 00143 #warning "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00144 #define __FPU_USED 0 00145 #endif 00146 #else 00147 #define __FPU_USED 0 00148 #endif 00149 00150 #elif defined ( __TMS470__ ) 00151 #if defined __TI_VFP_SUPPORT__ 00152 #if (__FPU_PRESENT == 1) 00153 #define __FPU_USED 1 00154 #else 00155 #warning "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00156 #define __FPU_USED 0 00157 #endif 00158 #else 00159 #define __FPU_USED 0 00160 #endif 00161 00162 #elif defined ( __TASKING__ ) 00163 #if defined __FPU_VFP__ 00164 #if (__FPU_PRESENT == 1) 00165 #define __FPU_USED 1 00166 #else 00167 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00168 #define __FPU_USED 0 00169 #endif 00170 #else 00171 #define __FPU_USED 0 00172 #endif 00173 00174 #elif defined ( __CSMC__ ) /* Cosmic */ 00175 #if ( __CSMC__ & 0x400) // FPU present for parser 00176 #if (__FPU_PRESENT == 1) 00177 #define __FPU_USED 1 00178 #else 00179 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00180 #define __FPU_USED 0 00181 #endif 00182 #else 00183 #define __FPU_USED 0 00184 #endif 00185 #endif 00186 00187 #include <stdint.h> /* standard types definitions */ 00188 #include <core_cmInstr.h> /* Core Instruction Access */ 00189 #include <core_cmFunc.h> /* Core Function Access */ 00190 #include <core_cmSimd.h> /* Compiler specific SIMD Intrinsics */ 00191 00192 #ifdef __cplusplus 00193 } 00194 #endif 00195 00196 #endif /* __CORE_CM7_H_GENERIC */ 00197 00198 #ifndef __CMSIS_GENERIC 00199 00200 #ifndef __CORE_CM7_H_DEPENDANT 00201 #define __CORE_CM7_H_DEPENDANT 00202 00203 #ifdef __cplusplus 00204 extern "C" { 00205 #endif 00206 00207 /* check device defines and use defaults */ 00208 #if defined __CHECK_DEVICE_DEFINES 00209 #ifndef __CM7_REV 00210 #define __CM7_REV 0x0000 00211 #warning "__CM7_REV not defined in device header file; using default!" 00212 #endif 00213 00214 #ifndef __FPU_PRESENT 00215 #define __FPU_PRESENT 0 00216 #warning "__FPU_PRESENT not defined in device header file; using default!" 00217 #endif 00218 00219 #ifndef __MPU_PRESENT 00220 #define __MPU_PRESENT 0 00221 #warning "__MPU_PRESENT not defined in device header file; using default!" 00222 #endif 00223 00224 #ifndef __ICACHE_PRESENT 00225 #define __ICACHE_PRESENT 0 00226 #warning "__ICACHE_PRESENT not defined in device header file; using default!" 00227 #endif 00228 00229 #ifndef __DCACHE_PRESENT 00230 #define __DCACHE_PRESENT 0 00231 #warning "__DCACHE_PRESENT not defined in device header file; using default!" 00232 #endif 00233 00234 #ifndef __DTCM_PRESENT 00235 #define __DTCM_PRESENT 0 00236 #warning "__DTCM_PRESENT not defined in device header file; using default!" 00237 #endif 00238 00239 #ifndef __NVIC_PRIO_BITS 00240 #define __NVIC_PRIO_BITS 3 00241 #warning "__NVIC_PRIO_BITS not defined in device header file; using default!" 00242 #endif 00243 00244 #ifndef __Vendor_SysTickConfig 00245 #define __Vendor_SysTickConfig 0 00246 #warning "__Vendor_SysTickConfig not defined in device header file; using default!" 00247 #endif 00248 #endif 00249 00250 /* IO definitions (access restrictions to peripheral registers) */ 00251 /** 00252 \defgroup CMSIS_glob_defs CMSIS Global Defines 00253 00254 <strong>IO Type Qualifiers</strong> are used 00255 \li to specify the access to peripheral variables. 00256 \li for automatic generation of peripheral register debug information. 00257 */ 00258 #ifdef __cplusplus 00259 #define __I volatile /*!< Defines 'read only' permissions */ 00260 #else 00261 #define __I volatile const /*!< Defines 'read only' permissions */ 00262 #endif 00263 #define __O volatile /*!< Defines 'write only' permissions */ 00264 #define __IO volatile /*!< Defines 'read / write' permissions */ 00265 00266 /*@} end of group Cortex_M7 */ 00267 00268 00269 00270 /******************************************************************************* 00271 * Register Abstraction 00272 Core Register contain: 00273 - Core Register 00274 - Core NVIC Register 00275 - Core SCB Register 00276 - Core SysTick Register 00277 - Core Debug Register 00278 - Core MPU Register 00279 - Core FPU Register 00280 ******************************************************************************/ 00281 /** \defgroup CMSIS_core_register Defines and Type Definitions 00282 \brief Type definitions and defines for Cortex-M processor based devices. 00283 */ 00284 00285 /** \ingroup CMSIS_core_register 00286 \defgroup CMSIS_CORE Status and Control Registers 00287 \brief Core Register type definitions. 00288 @{ 00289 */ 00290 00291 /** \brief Union type to access the Application Program Status Register (APSR). 00292 */ 00293 typedef union 00294 { 00295 struct 00296 { 00297 uint32_t _reserved0:16; /*!< bit: 0..15 Reserved */ 00298 uint32_t GE:4; /*!< bit: 16..19 Greater than or Equal flags */ 00299 uint32_t _reserved1:7; /*!< bit: 20..26 Reserved */ 00300 uint32_t Q:1; /*!< bit: 27 Saturation condition flag */ 00301 uint32_t V:1; /*!< bit: 28 Overflow condition code flag */ 00302 uint32_t C:1; /*!< bit: 29 Carry condition code flag */ 00303 uint32_t Z:1; /*!< bit: 30 Zero condition code flag */ 00304 uint32_t N:1; /*!< bit: 31 Negative condition code flag */ 00305 } b; /*!< Structure used for bit access */ 00306 uint32_t w; /*!< Type used for word access */ 00307 } APSR_Type; 00308 00309 /* APSR Register Definitions */ 00310 #define APSR_N_Pos 31 /*!< APSR: N Position */ 00311 #define APSR_N_Msk (1UL << APSR_N_Pos) /*!< APSR: N Mask */ 00312 00313 #define APSR_Z_Pos 30 /*!< APSR: Z Position */ 00314 #define APSR_Z_Msk (1UL << APSR_Z_Pos) /*!< APSR: Z Mask */ 00315 00316 #define APSR_C_Pos 29 /*!< APSR: C Position */ 00317 #define APSR_C_Msk (1UL << APSR_C_Pos) /*!< APSR: C Mask */ 00318 00319 #define APSR_V_Pos 28 /*!< APSR: V Position */ 00320 #define APSR_V_Msk (1UL << APSR_V_Pos) /*!< APSR: V Mask */ 00321 00322 #define APSR_Q_Pos 27 /*!< APSR: Q Position */ 00323 #define APSR_Q_Msk (1UL << APSR_Q_Pos) /*!< APSR: Q Mask */ 00324 00325 #define APSR_GE_Pos 16 /*!< APSR: GE Position */ 00326 #define APSR_GE_Msk (0xFUL << APSR_GE_Pos) /*!< APSR: GE Mask */ 00327 00328 00329 /** \brief Union type to access the Interrupt Program Status Register (IPSR). 00330 */ 00331 typedef union 00332 { 00333 struct 00334 { 00335 uint32_t ISR:9; /*!< bit: 0.. 8 Exception number */ 00336 uint32_t _reserved0:23; /*!< bit: 9..31 Reserved */ 00337 } b; /*!< Structure used for bit access */ 00338 uint32_t w; /*!< Type used for word access */ 00339 } IPSR_Type; 00340 00341 /* IPSR Register Definitions */ 00342 #define IPSR_ISR_Pos 0 /*!< IPSR: ISR Position */ 00343 #define IPSR_ISR_Msk (0x1FFUL /*<< IPSR_ISR_Pos*/) /*!< IPSR: ISR Mask */ 00344 00345 00346 /** \brief Union type to access the Special-Purpose Program Status Registers (xPSR). 00347 */ 00348 typedef union 00349 { 00350 struct 00351 { 00352 uint32_t ISR:9; /*!< bit: 0.. 8 Exception number */ 00353 uint32_t _reserved0:7; /*!< bit: 9..15 Reserved */ 00354 uint32_t GE:4; /*!< bit: 16..19 Greater than or Equal flags */ 00355 uint32_t _reserved1:4; /*!< bit: 20..23 Reserved */ 00356 uint32_t T:1; /*!< bit: 24 Thumb bit (read 0) */ 00357 uint32_t IT:2; /*!< bit: 25..26 saved IT state (read 0) */ 00358 uint32_t Q:1; /*!< bit: 27 Saturation condition flag */ 00359 uint32_t V:1; /*!< bit: 28 Overflow condition code flag */ 00360 uint32_t C:1; /*!< bit: 29 Carry condition code flag */ 00361 uint32_t Z:1; /*!< bit: 30 Zero condition code flag */ 00362 uint32_t N:1; /*!< bit: 31 Negative condition code flag */ 00363 } b; /*!< Structure used for bit access */ 00364 uint32_t w; /*!< Type used for word access */ 00365 } xPSR_Type; 00366 00367 /* xPSR Register Definitions */ 00368 #define xPSR_N_Pos 31 /*!< xPSR: N Position */ 00369 #define xPSR_N_Msk (1UL << xPSR_N_Pos) /*!< xPSR: N Mask */ 00370 00371 #define xPSR_Z_Pos 30 /*!< xPSR: Z Position */ 00372 #define xPSR_Z_Msk (1UL << xPSR_Z_Pos) /*!< xPSR: Z Mask */ 00373 00374 #define xPSR_C_Pos 29 /*!< xPSR: C Position */ 00375 #define xPSR_C_Msk (1UL << xPSR_C_Pos) /*!< xPSR: C Mask */ 00376 00377 #define xPSR_V_Pos 28 /*!< xPSR: V Position */ 00378 #define xPSR_V_Msk (1UL << xPSR_V_Pos) /*!< xPSR: V Mask */ 00379 00380 #define xPSR_Q_Pos 27 /*!< xPSR: Q Position */ 00381 #define xPSR_Q_Msk (1UL << xPSR_Q_Pos) /*!< xPSR: Q Mask */ 00382 00383 #define xPSR_IT_Pos 25 /*!< xPSR: IT Position */ 00384 #define xPSR_IT_Msk (3UL << xPSR_IT_Pos) /*!< xPSR: IT Mask */ 00385 00386 #define xPSR_T_Pos 24 /*!< xPSR: T Position */ 00387 #define xPSR_T_Msk (1UL << xPSR_T_Pos) /*!< xPSR: T Mask */ 00388 00389 #define xPSR_GE_Pos 16 /*!< xPSR: GE Position */ 00390 #define xPSR_GE_Msk (0xFUL << xPSR_GE_Pos) /*!< xPSR: GE Mask */ 00391 00392 #define xPSR_ISR_Pos 0 /*!< xPSR: ISR Position */ 00393 #define xPSR_ISR_Msk (0x1FFUL /*<< xPSR_ISR_Pos*/) /*!< xPSR: ISR Mask */ 00394 00395 00396 /** \brief Union type to access the Control Registers (CONTROL). 00397 */ 00398 typedef union 00399 { 00400 struct 00401 { 00402 uint32_t nPRIV:1; /*!< bit: 0 Execution privilege in Thread mode */ 00403 uint32_t SPSEL:1; /*!< bit: 1 Stack to be used */ 00404 uint32_t FPCA:1; /*!< bit: 2 FP extension active flag */ 00405 uint32_t _reserved0:29; /*!< bit: 3..31 Reserved */ 00406 } b; /*!< Structure used for bit access */ 00407 uint32_t w; /*!< Type used for word access */ 00408 } CONTROL_Type; 00409 00410 /* CONTROL Register Definitions */ 00411 #define CONTROL_FPCA_Pos 2 /*!< CONTROL: FPCA Position */ 00412 #define CONTROL_FPCA_Msk (1UL << CONTROL_FPCA_Pos) /*!< CONTROL: FPCA Mask */ 00413 00414 #define CONTROL_SPSEL_Pos 1 /*!< CONTROL: SPSEL Position */ 00415 #define CONTROL_SPSEL_Msk (1UL << CONTROL_SPSEL_Pos) /*!< CONTROL: SPSEL Mask */ 00416 00417 #define CONTROL_nPRIV_Pos 0 /*!< CONTROL: nPRIV Position */ 00418 #define CONTROL_nPRIV_Msk (1UL /*<< CONTROL_nPRIV_Pos*/) /*!< CONTROL: nPRIV Mask */ 00419 00420 /*@} end of group CMSIS_CORE */ 00421 00422 00423 /** \ingroup CMSIS_core_register 00424 \defgroup CMSIS_NVIC Nested Vectored Interrupt Controller (NVIC) 00425 \brief Type definitions for the NVIC Registers 00426 @{ 00427 */ 00428 00429 /** \brief Structure type to access the Nested Vectored Interrupt Controller (NVIC). 00430 */ 00431 typedef struct 00432 { 00433 __IO uint32_t ISER[8]; /*!< Offset: 0x000 (R/W) Interrupt Set Enable Register */ 00434 uint32_t RESERVED0[24]; 00435 __IO uint32_t ICER[8]; /*!< Offset: 0x080 (R/W) Interrupt Clear Enable Register */ 00436 uint32_t RSERVED1[24]; 00437 __IO uint32_t ISPR[8]; /*!< Offset: 0x100 (R/W) Interrupt Set Pending Register */ 00438 uint32_t RESERVED2[24]; 00439 __IO uint32_t ICPR[8]; /*!< Offset: 0x180 (R/W) Interrupt Clear Pending Register */ 00440 uint32_t RESERVED3[24]; 00441 __IO uint32_t IABR[8]; /*!< Offset: 0x200 (R/W) Interrupt Active bit Register */ 00442 uint32_t RESERVED4[56]; 00443 __IO uint8_t IP[240]; /*!< Offset: 0x300 (R/W) Interrupt Priority Register (8Bit wide) */ 00444 uint32_t RESERVED5[644]; 00445 __O uint32_t STIR; /*!< Offset: 0xE00 ( /W) Software Trigger Interrupt Register */ 00446 } NVIC_Type; 00447 00448 /* Software Triggered Interrupt Register Definitions */ 00449 #define NVIC_STIR_INTID_Pos 0 /*!< STIR: INTLINESNUM Position */ 00450 #define NVIC_STIR_INTID_Msk (0x1FFUL /*<< NVIC_STIR_INTID_Pos*/) /*!< STIR: INTLINESNUM Mask */ 00451 00452 /*@} end of group CMSIS_NVIC */ 00453 00454 00455 /** \ingroup CMSIS_core_register 00456 \defgroup CMSIS_SCB System Control Block (SCB) 00457 \brief Type definitions for the System Control Block Registers 00458 @{ 00459 */ 00460 00461 /** \brief Structure type to access the System Control Block (SCB). 00462 */ 00463 typedef struct 00464 { 00465 __I uint32_t CPUID; /*!< Offset: 0x000 (R/ ) CPUID Base Register */ 00466 __IO uint32_t ICSR; /*!< Offset: 0x004 (R/W) Interrupt Control and State Register */ 00467 __IO uint32_t VTOR; /*!< Offset: 0x008 (R/W) Vector Table Offset Register */ 00468 __IO uint32_t AIRCR; /*!< Offset: 0x00C (R/W) Application Interrupt and Reset Control Register */ 00469 __IO uint32_t SCR; /*!< Offset: 0x010 (R/W) System Control Register */ 00470 __IO uint32_t CCR; /*!< Offset: 0x014 (R/W) Configuration Control Register */ 00471 __IO uint8_t SHPR[12]; /*!< Offset: 0x018 (R/W) System Handlers Priority Registers (4-7, 8-11, 12-15) */ 00472 __IO uint32_t SHCSR; /*!< Offset: 0x024 (R/W) System Handler Control and State Register */ 00473 __IO uint32_t CFSR; /*!< Offset: 0x028 (R/W) Configurable Fault Status Register */ 00474 __IO uint32_t HFSR; /*!< Offset: 0x02C (R/W) HardFault Status Register */ 00475 __IO uint32_t DFSR; /*!< Offset: 0x030 (R/W) Debug Fault Status Register */ 00476 __IO uint32_t MMFAR; /*!< Offset: 0x034 (R/W) MemManage Fault Address Register */ 00477 __IO uint32_t BFAR; /*!< Offset: 0x038 (R/W) BusFault Address Register */ 00478 __IO uint32_t AFSR; /*!< Offset: 0x03C (R/W) Auxiliary Fault Status Register */ 00479 __I uint32_t ID_PFR[2]; /*!< Offset: 0x040 (R/ ) Processor Feature Register */ 00480 __I uint32_t ID_DFR; /*!< Offset: 0x048 (R/ ) Debug Feature Register */ 00481 __I uint32_t ID_AFR; /*!< Offset: 0x04C (R/ ) Auxiliary Feature Register */ 00482 __I uint32_t ID_MFR[4]; /*!< Offset: 0x050 (R/ ) Memory Model Feature Register */ 00483 __I uint32_t ID_ISAR[5]; /*!< Offset: 0x060 (R/ ) Instruction Set Attributes Register */ 00484 uint32_t RESERVED0[1]; 00485 __I uint32_t CLIDR; /*!< Offset: 0x078 (R/ ) Cache Level ID register */ 00486 __I uint32_t CTR; /*!< Offset: 0x07C (R/ ) Cache Type register */ 00487 __I uint32_t CCSIDR; /*!< Offset: 0x080 (R/ ) Cache Size ID Register */ 00488 __IO uint32_t CSSELR; /*!< Offset: 0x084 (R/W) Cache Size Selection Register */ 00489 __IO uint32_t CPACR; /*!< Offset: 0x088 (R/W) Coprocessor Access Control Register */ 00490 uint32_t RESERVED3[93]; 00491 __O uint32_t STIR; /*!< Offset: 0x200 ( /W) Software Triggered Interrupt Register */ 00492 uint32_t RESERVED4[15]; 00493 __I uint32_t MVFR0; /*!< Offset: 0x240 (R/ ) Media and VFP Feature Register 0 */ 00494 __I uint32_t MVFR1; /*!< Offset: 0x244 (R/ ) Media and VFP Feature Register 1 */ 00495 __I uint32_t MVFR2; /*!< Offset: 0x248 (R/ ) Media and VFP Feature Register 1 */ 00496 uint32_t RESERVED5[1]; 00497 __O uint32_t ICIALLU; /*!< Offset: 0x250 ( /W) I-Cache Invalidate All to PoU */ 00498 uint32_t RESERVED6[1]; 00499 __O uint32_t ICIMVAU; /*!< Offset: 0x258 ( /W) I-Cache Invalidate by MVA to PoU */ 00500 __O uint32_t DCIMVAC; /*!< Offset: 0x25C ( /W) D-Cache Invalidate by MVA to PoC */ 00501 __O uint32_t DCISW; /*!< Offset: 0x260 ( /W) D-Cache Invalidate by Set-way */ 00502 __O uint32_t DCCMVAU; /*!< Offset: 0x264 ( /W) D-Cache Clean by MVA to PoU */ 00503 __O uint32_t DCCMVAC; /*!< Offset: 0x268 ( /W) D-Cache Clean by MVA to PoC */ 00504 __O uint32_t DCCSW; /*!< Offset: 0x26C ( /W) D-Cache Clean by Set-way */ 00505 __O uint32_t DCCIMVAC; /*!< Offset: 0x270 ( /W) D-Cache Clean and Invalidate by MVA to PoC */ 00506 __O uint32_t DCCISW; /*!< Offset: 0x274 ( /W) D-Cache Clean and Invalidate by Set-way */ 00507 uint32_t RESERVED7[6]; 00508 __IO uint32_t ITCMCR; /*!< Offset: 0x290 (R/W) Instruction Tightly-Coupled Memory Control Register */ 00509 __IO uint32_t DTCMCR; /*!< Offset: 0x294 (R/W) Data Tightly-Coupled Memory Control Registers */ 00510 __IO uint32_t AHBPCR; /*!< Offset: 0x298 (R/W) AHBP Control Register */ 00511 __IO uint32_t CACR; /*!< Offset: 0x29C (R/W) L1 Cache Control Register */ 00512 __IO uint32_t AHBSCR; /*!< Offset: 0x2A0 (R/W) AHB Slave Control Register */ 00513 uint32_t RESERVED8[1]; 00514 __IO uint32_t ABFSR; /*!< Offset: 0x2A8 (R/W) Auxiliary Bus Fault Status Register */ 00515 } SCB_Type; 00516 00517 /* SCB CPUID Register Definitions */ 00518 #define SCB_CPUID_IMPLEMENTER_Pos 24 /*!< SCB CPUID: IMPLEMENTER Position */ 00519 #define SCB_CPUID_IMPLEMENTER_Msk (0xFFUL << SCB_CPUID_IMPLEMENTER_Pos) /*!< SCB CPUID: IMPLEMENTER Mask */ 00520 00521 #define SCB_CPUID_VARIANT_Pos 20 /*!< SCB CPUID: VARIANT Position */ 00522 #define SCB_CPUID_VARIANT_Msk (0xFUL << SCB_CPUID_VARIANT_Pos) /*!< SCB CPUID: VARIANT Mask */ 00523 00524 #define SCB_CPUID_ARCHITECTURE_Pos 16 /*!< SCB CPUID: ARCHITECTURE Position */ 00525 #define SCB_CPUID_ARCHITECTURE_Msk (0xFUL << SCB_CPUID_ARCHITECTURE_Pos) /*!< SCB CPUID: ARCHITECTURE Mask */ 00526 00527 #define SCB_CPUID_PARTNO_Pos 4 /*!< SCB CPUID: PARTNO Position */ 00528 #define SCB_CPUID_PARTNO_Msk (0xFFFUL << SCB_CPUID_PARTNO_Pos) /*!< SCB CPUID: PARTNO Mask */ 00529 00530 #define SCB_CPUID_REVISION_Pos 0 /*!< SCB CPUID: REVISION Position */ 00531 #define SCB_CPUID_REVISION_Msk (0xFUL /*<< SCB_CPUID_REVISION_Pos*/) /*!< SCB CPUID: REVISION Mask */ 00532 00533 /* SCB Interrupt Control State Register Definitions */ 00534 #define SCB_ICSR_NMIPENDSET_Pos 31 /*!< SCB ICSR: NMIPENDSET Position */ 00535 #define SCB_ICSR_NMIPENDSET_Msk (1UL << SCB_ICSR_NMIPENDSET_Pos) /*!< SCB ICSR: NMIPENDSET Mask */ 00536 00537 #define SCB_ICSR_PENDSVSET_Pos 28 /*!< SCB ICSR: PENDSVSET Position */ 00538 #define SCB_ICSR_PENDSVSET_Msk (1UL << SCB_ICSR_PENDSVSET_Pos) /*!< SCB ICSR: PENDSVSET Mask */ 00539 00540 #define SCB_ICSR_PENDSVCLR_Pos 27 /*!< SCB ICSR: PENDSVCLR Position */ 00541 #define SCB_ICSR_PENDSVCLR_Msk (1UL << SCB_ICSR_PENDSVCLR_Pos) /*!< SCB ICSR: PENDSVCLR Mask */ 00542 00543 #define SCB_ICSR_PENDSTSET_Pos 26 /*!< SCB ICSR: PENDSTSET Position */ 00544 #define SCB_ICSR_PENDSTSET_Msk (1UL << SCB_ICSR_PENDSTSET_Pos) /*!< SCB ICSR: PENDSTSET Mask */ 00545 00546 #define SCB_ICSR_PENDSTCLR_Pos 25 /*!< SCB ICSR: PENDSTCLR Position */ 00547 #define SCB_ICSR_PENDSTCLR_Msk (1UL << SCB_ICSR_PENDSTCLR_Pos) /*!< SCB ICSR: PENDSTCLR Mask */ 00548 00549 #define SCB_ICSR_ISRPREEMPT_Pos 23 /*!< SCB ICSR: ISRPREEMPT Position */ 00550 #define SCB_ICSR_ISRPREEMPT_Msk (1UL << SCB_ICSR_ISRPREEMPT_Pos) /*!< SCB ICSR: ISRPREEMPT Mask */ 00551 00552 #define SCB_ICSR_ISRPENDING_Pos 22 /*!< SCB ICSR: ISRPENDING Position */ 00553 #define SCB_ICSR_ISRPENDING_Msk (1UL << SCB_ICSR_ISRPENDING_Pos) /*!< SCB ICSR: ISRPENDING Mask */ 00554 00555 #define SCB_ICSR_VECTPENDING_Pos 12 /*!< SCB ICSR: VECTPENDING Position */ 00556 #define SCB_ICSR_VECTPENDING_Msk (0x1FFUL << SCB_ICSR_VECTPENDING_Pos) /*!< SCB ICSR: VECTPENDING Mask */ 00557 00558 #define SCB_ICSR_RETTOBASE_Pos 11 /*!< SCB ICSR: RETTOBASE Position */ 00559 #define SCB_ICSR_RETTOBASE_Msk (1UL << SCB_ICSR_RETTOBASE_Pos) /*!< SCB ICSR: RETTOBASE Mask */ 00560 00561 #define SCB_ICSR_VECTACTIVE_Pos 0 /*!< SCB ICSR: VECTACTIVE Position */ 00562 #define SCB_ICSR_VECTACTIVE_Msk (0x1FFUL /*<< SCB_ICSR_VECTACTIVE_Pos*/) /*!< SCB ICSR: VECTACTIVE Mask */ 00563 00564 /* SCB Vector Table Offset Register Definitions */ 00565 #define SCB_VTOR_TBLOFF_Pos 7 /*!< SCB VTOR: TBLOFF Position */ 00566 #define SCB_VTOR_TBLOFF_Msk (0x1FFFFFFUL << SCB_VTOR_TBLOFF_Pos) /*!< SCB VTOR: TBLOFF Mask */ 00567 00568 /* SCB Application Interrupt and Reset Control Register Definitions */ 00569 #define SCB_AIRCR_VECTKEY_Pos 16 /*!< SCB AIRCR: VECTKEY Position */ 00570 #define SCB_AIRCR_VECTKEY_Msk (0xFFFFUL << SCB_AIRCR_VECTKEY_Pos) /*!< SCB AIRCR: VECTKEY Mask */ 00571 00572 #define SCB_AIRCR_VECTKEYSTAT_Pos 16 /*!< SCB AIRCR: VECTKEYSTAT Position */ 00573 #define SCB_AIRCR_VECTKEYSTAT_Msk (0xFFFFUL << SCB_AIRCR_VECTKEYSTAT_Pos) /*!< SCB AIRCR: VECTKEYSTAT Mask */ 00574 00575 #define SCB_AIRCR_ENDIANESS_Pos 15 /*!< SCB AIRCR: ENDIANESS Position */ 00576 #define SCB_AIRCR_ENDIANESS_Msk (1UL << SCB_AIRCR_ENDIANESS_Pos) /*!< SCB AIRCR: ENDIANESS Mask */ 00577 00578 #define SCB_AIRCR_PRIGROUP_Pos 8 /*!< SCB AIRCR: PRIGROUP Position */ 00579 #define SCB_AIRCR_PRIGROUP_Msk (7UL << SCB_AIRCR_PRIGROUP_Pos) /*!< SCB AIRCR: PRIGROUP Mask */ 00580 00581 #define SCB_AIRCR_SYSRESETREQ_Pos 2 /*!< SCB AIRCR: SYSRESETREQ Position */ 00582 #define SCB_AIRCR_SYSRESETREQ_Msk (1UL << SCB_AIRCR_SYSRESETREQ_Pos) /*!< SCB AIRCR: SYSRESETREQ Mask */ 00583 00584 #define SCB_AIRCR_VECTCLRACTIVE_Pos 1 /*!< SCB AIRCR: VECTCLRACTIVE Position */ 00585 #define SCB_AIRCR_VECTCLRACTIVE_Msk (1UL << SCB_AIRCR_VECTCLRACTIVE_Pos) /*!< SCB AIRCR: VECTCLRACTIVE Mask */ 00586 00587 #define SCB_AIRCR_VECTRESET_Pos 0 /*!< SCB AIRCR: VECTRESET Position */ 00588 #define SCB_AIRCR_VECTRESET_Msk (1UL /*<< SCB_AIRCR_VECTRESET_Pos*/) /*!< SCB AIRCR: VECTRESET Mask */ 00589 00590 /* SCB System Control Register Definitions */ 00591 #define SCB_SCR_SEVONPEND_Pos 4 /*!< SCB SCR: SEVONPEND Position */ 00592 #define SCB_SCR_SEVONPEND_Msk (1UL << SCB_SCR_SEVONPEND_Pos) /*!< SCB SCR: SEVONPEND Mask */ 00593 00594 #define SCB_SCR_SLEEPDEEP_Pos 2 /*!< SCB SCR: SLEEPDEEP Position */ 00595 #define SCB_SCR_SLEEPDEEP_Msk (1UL << SCB_SCR_SLEEPDEEP_Pos) /*!< SCB SCR: SLEEPDEEP Mask */ 00596 00597 #define SCB_SCR_SLEEPONEXIT_Pos 1 /*!< SCB SCR: SLEEPONEXIT Position */ 00598 #define SCB_SCR_SLEEPONEXIT_Msk (1UL << SCB_SCR_SLEEPONEXIT_Pos) /*!< SCB SCR: SLEEPONEXIT Mask */ 00599 00600 /* SCB Configuration Control Register Definitions */ 00601 #define SCB_CCR_BP_Pos 18 /*!< SCB CCR: Branch prediction enable bit Position */ 00602 #define SCB_CCR_BP_Msk (1UL << SCB_CCR_BP_Pos) /*!< SCB CCR: Branch prediction enable bit Mask */ 00603 00604 #define SCB_CCR_IC_Pos 17 /*!< SCB CCR: Instruction cache enable bit Position */ 00605 #define SCB_CCR_IC_Msk (1UL << SCB_CCR_IC_Pos) /*!< SCB CCR: Instruction cache enable bit Mask */ 00606 00607 #define SCB_CCR_DC_Pos 16 /*!< SCB CCR: Cache enable bit Position */ 00608 #define SCB_CCR_DC_Msk (1UL << SCB_CCR_DC_Pos) /*!< SCB CCR: Cache enable bit Mask */ 00609 00610 #define SCB_CCR_STKALIGN_Pos 9 /*!< SCB CCR: STKALIGN Position */ 00611 #define SCB_CCR_STKALIGN_Msk (1UL << SCB_CCR_STKALIGN_Pos) /*!< SCB CCR: STKALIGN Mask */ 00612 00613 #define SCB_CCR_BFHFNMIGN_Pos 8 /*!< SCB CCR: BFHFNMIGN Position */ 00614 #define SCB_CCR_BFHFNMIGN_Msk (1UL << SCB_CCR_BFHFNMIGN_Pos) /*!< SCB CCR: BFHFNMIGN Mask */ 00615 00616 #define SCB_CCR_DIV_0_TRP_Pos 4 /*!< SCB CCR: DIV_0_TRP Position */ 00617 #define SCB_CCR_DIV_0_TRP_Msk (1UL << SCB_CCR_DIV_0_TRP_Pos) /*!< SCB CCR: DIV_0_TRP Mask */ 00618 00619 #define SCB_CCR_UNALIGN_TRP_Pos 3 /*!< SCB CCR: UNALIGN_TRP Position */ 00620 #define SCB_CCR_UNALIGN_TRP_Msk (1UL << SCB_CCR_UNALIGN_TRP_Pos) /*!< SCB CCR: UNALIGN_TRP Mask */ 00621 00622 #define SCB_CCR_USERSETMPEND_Pos 1 /*!< SCB CCR: USERSETMPEND Position */ 00623 #define SCB_CCR_USERSETMPEND_Msk (1UL << SCB_CCR_USERSETMPEND_Pos) /*!< SCB CCR: USERSETMPEND Mask */ 00624 00625 #define SCB_CCR_NONBASETHRDENA_Pos 0 /*!< SCB CCR: NONBASETHRDENA Position */ 00626 #define SCB_CCR_NONBASETHRDENA_Msk (1UL /*<< SCB_CCR_NONBASETHRDENA_Pos*/) /*!< SCB CCR: NONBASETHRDENA Mask */ 00627 00628 /* SCB System Handler Control and State Register Definitions */ 00629 #define SCB_SHCSR_USGFAULTENA_Pos 18 /*!< SCB SHCSR: USGFAULTENA Position */ 00630 #define SCB_SHCSR_USGFAULTENA_Msk (1UL << SCB_SHCSR_USGFAULTENA_Pos) /*!< SCB SHCSR: USGFAULTENA Mask */ 00631 00632 #define SCB_SHCSR_BUSFAULTENA_Pos 17 /*!< SCB SHCSR: BUSFAULTENA Position */ 00633 #define SCB_SHCSR_BUSFAULTENA_Msk (1UL << SCB_SHCSR_BUSFAULTENA_Pos) /*!< SCB SHCSR: BUSFAULTENA Mask */ 00634 00635 #define SCB_SHCSR_MEMFAULTENA_Pos 16 /*!< SCB SHCSR: MEMFAULTENA Position */ 00636 #define SCB_SHCSR_MEMFAULTENA_Msk (1UL << SCB_SHCSR_MEMFAULTENA_Pos) /*!< SCB SHCSR: MEMFAULTENA Mask */ 00637 00638 #define SCB_SHCSR_SVCALLPENDED_Pos 15 /*!< SCB SHCSR: SVCALLPENDED Position */ 00639 #define SCB_SHCSR_SVCALLPENDED_Msk (1UL << SCB_SHCSR_SVCALLPENDED_Pos) /*!< SCB SHCSR: SVCALLPENDED Mask */ 00640 00641 #define SCB_SHCSR_BUSFAULTPENDED_Pos 14 /*!< SCB SHCSR: BUSFAULTPENDED Position */ 00642 #define SCB_SHCSR_BUSFAULTPENDED_Msk (1UL << SCB_SHCSR_BUSFAULTPENDED_Pos) /*!< SCB SHCSR: BUSFAULTPENDED Mask */ 00643 00644 #define SCB_SHCSR_MEMFAULTPENDED_Pos 13 /*!< SCB SHCSR: MEMFAULTPENDED Position */ 00645 #define SCB_SHCSR_MEMFAULTPENDED_Msk (1UL << SCB_SHCSR_MEMFAULTPENDED_Pos) /*!< SCB SHCSR: MEMFAULTPENDED Mask */ 00646 00647 #define SCB_SHCSR_USGFAULTPENDED_Pos 12 /*!< SCB SHCSR: USGFAULTPENDED Position */ 00648 #define SCB_SHCSR_USGFAULTPENDED_Msk (1UL << SCB_SHCSR_USGFAULTPENDED_Pos) /*!< SCB SHCSR: USGFAULTPENDED Mask */ 00649 00650 #define SCB_SHCSR_SYSTICKACT_Pos 11 /*!< SCB SHCSR: SYSTICKACT Position */ 00651 #define SCB_SHCSR_SYSTICKACT_Msk (1UL << SCB_SHCSR_SYSTICKACT_Pos) /*!< SCB SHCSR: SYSTICKACT Mask */ 00652 00653 #define SCB_SHCSR_PENDSVACT_Pos 10 /*!< SCB SHCSR: PENDSVACT Position */ 00654 #define SCB_SHCSR_PENDSVACT_Msk (1UL << SCB_SHCSR_PENDSVACT_Pos) /*!< SCB SHCSR: PENDSVACT Mask */ 00655 00656 #define SCB_SHCSR_MONITORACT_Pos 8 /*!< SCB SHCSR: MONITORACT Position */ 00657 #define SCB_SHCSR_MONITORACT_Msk (1UL << SCB_SHCSR_MONITORACT_Pos) /*!< SCB SHCSR: MONITORACT Mask */ 00658 00659 #define SCB_SHCSR_SVCALLACT_Pos 7 /*!< SCB SHCSR: SVCALLACT Position */ 00660 #define SCB_SHCSR_SVCALLACT_Msk (1UL << SCB_SHCSR_SVCALLACT_Pos) /*!< SCB SHCSR: SVCALLACT Mask */ 00661 00662 #define SCB_SHCSR_USGFAULTACT_Pos 3 /*!< SCB SHCSR: USGFAULTACT Position */ 00663 #define SCB_SHCSR_USGFAULTACT_Msk (1UL << SCB_SHCSR_USGFAULTACT_Pos) /*!< SCB SHCSR: USGFAULTACT Mask */ 00664 00665 #define SCB_SHCSR_BUSFAULTACT_Pos 1 /*!< SCB SHCSR: BUSFAULTACT Position */ 00666 #define SCB_SHCSR_BUSFAULTACT_Msk (1UL << SCB_SHCSR_BUSFAULTACT_Pos) /*!< SCB SHCSR: BUSFAULTACT Mask */ 00667 00668 #define SCB_SHCSR_MEMFAULTACT_Pos 0 /*!< SCB SHCSR: MEMFAULTACT Position */ 00669 #define SCB_SHCSR_MEMFAULTACT_Msk (1UL /*<< SCB_SHCSR_MEMFAULTACT_Pos*/) /*!< SCB SHCSR: MEMFAULTACT Mask */ 00670 00671 /* SCB Configurable Fault Status Registers Definitions */ 00672 #define SCB_CFSR_USGFAULTSR_Pos 16 /*!< SCB CFSR: Usage Fault Status Register Position */ 00673 #define SCB_CFSR_USGFAULTSR_Msk (0xFFFFUL << SCB_CFSR_USGFAULTSR_Pos) /*!< SCB CFSR: Usage Fault Status Register Mask */ 00674 00675 #define SCB_CFSR_BUSFAULTSR_Pos 8 /*!< SCB CFSR: Bus Fault Status Register Position */ 00676 #define SCB_CFSR_BUSFAULTSR_Msk (0xFFUL << SCB_CFSR_BUSFAULTSR_Pos) /*!< SCB CFSR: Bus Fault Status Register Mask */ 00677 00678 #define SCB_CFSR_MEMFAULTSR_Pos 0 /*!< SCB CFSR: Memory Manage Fault Status Register Position */ 00679 #define SCB_CFSR_MEMFAULTSR_Msk (0xFFUL /*<< SCB_CFSR_MEMFAULTSR_Pos*/) /*!< SCB CFSR: Memory Manage Fault Status Register Mask */ 00680 00681 /* SCB Hard Fault Status Registers Definitions */ 00682 #define SCB_HFSR_DEBUGEVT_Pos 31 /*!< SCB HFSR: DEBUGEVT Position */ 00683 #define SCB_HFSR_DEBUGEVT_Msk (1UL << SCB_HFSR_DEBUGEVT_Pos) /*!< SCB HFSR: DEBUGEVT Mask */ 00684 00685 #define SCB_HFSR_FORCED_Pos 30 /*!< SCB HFSR: FORCED Position */ 00686 #define SCB_HFSR_FORCED_Msk (1UL << SCB_HFSR_FORCED_Pos) /*!< SCB HFSR: FORCED Mask */ 00687 00688 #define SCB_HFSR_VECTTBL_Pos 1 /*!< SCB HFSR: VECTTBL Position */ 00689 #define SCB_HFSR_VECTTBL_Msk (1UL << SCB_HFSR_VECTTBL_Pos) /*!< SCB HFSR: VECTTBL Mask */ 00690 00691 /* SCB Debug Fault Status Register Definitions */ 00692 #define SCB_DFSR_EXTERNAL_Pos 4 /*!< SCB DFSR: EXTERNAL Position */ 00693 #define SCB_DFSR_EXTERNAL_Msk (1UL << SCB_DFSR_EXTERNAL_Pos) /*!< SCB DFSR: EXTERNAL Mask */ 00694 00695 #define SCB_DFSR_VCATCH_Pos 3 /*!< SCB DFSR: VCATCH Position */ 00696 #define SCB_DFSR_VCATCH_Msk (1UL << SCB_DFSR_VCATCH_Pos) /*!< SCB DFSR: VCATCH Mask */ 00697 00698 #define SCB_DFSR_DWTTRAP_Pos 2 /*!< SCB DFSR: DWTTRAP Position */ 00699 #define SCB_DFSR_DWTTRAP_Msk (1UL << SCB_DFSR_DWTTRAP_Pos) /*!< SCB DFSR: DWTTRAP Mask */ 00700 00701 #define SCB_DFSR_BKPT_Pos 1 /*!< SCB DFSR: BKPT Position */ 00702 #define SCB_DFSR_BKPT_Msk (1UL << SCB_DFSR_BKPT_Pos) /*!< SCB DFSR: BKPT Mask */ 00703 00704 #define SCB_DFSR_HALTED_Pos 0 /*!< SCB DFSR: HALTED Position */ 00705 #define SCB_DFSR_HALTED_Msk (1UL /*<< SCB_DFSR_HALTED_Pos*/) /*!< SCB DFSR: HALTED Mask */ 00706 00707 /* Cache Level ID register */ 00708 #define SCB_CLIDR_LOUU_Pos 27 /*!< SCB CLIDR: LoUU Position */ 00709 #define SCB_CLIDR_LOUU_Msk (7UL << SCB_CLIDR_LOUU_Pos) /*!< SCB CLIDR: LoUU Mask */ 00710 00711 #define SCB_CLIDR_LOC_Pos 24 /*!< SCB CLIDR: LoC Position */ 00712 #define SCB_CLIDR_LOC_Msk (7UL << SCB_CLIDR_FORMAT_Pos) /*!< SCB CLIDR: LoC Mask */ 00713 00714 /* Cache Type register */ 00715 #define SCB_CTR_FORMAT_Pos 29 /*!< SCB CTR: Format Position */ 00716 #define SCB_CTR_FORMAT_Msk (7UL << SCB_CTR_FORMAT_Pos) /*!< SCB CTR: Format Mask */ 00717 00718 #define SCB_CTR_CWG_Pos 24 /*!< SCB CTR: CWG Position */ 00719 #define SCB_CTR_CWG_Msk (0xFUL << SCB_CTR_CWG_Pos) /*!< SCB CTR: CWG Mask */ 00720 00721 #define SCB_CTR_ERG_Pos 20 /*!< SCB CTR: ERG Position */ 00722 #define SCB_CTR_ERG_Msk (0xFUL << SCB_CTR_ERG_Pos) /*!< SCB CTR: ERG Mask */ 00723 00724 #define SCB_CTR_DMINLINE_Pos 16 /*!< SCB CTR: DminLine Position */ 00725 #define SCB_CTR_DMINLINE_Msk (0xFUL << SCB_CTR_DMINLINE_Pos) /*!< SCB CTR: DminLine Mask */ 00726 00727 #define SCB_CTR_IMINLINE_Pos 0 /*!< SCB CTR: ImInLine Position */ 00728 #define SCB_CTR_IMINLINE_Msk (0xFUL /*<< SCB_CTR_IMINLINE_Pos*/) /*!< SCB CTR: ImInLine Mask */ 00729 00730 /* Cache Size ID Register */ 00731 #define SCB_CCSIDR_WT_Pos 31 /*!< SCB CCSIDR: WT Position */ 00732 #define SCB_CCSIDR_WT_Msk (7UL << SCB_CCSIDR_WT_Pos) /*!< SCB CCSIDR: WT Mask */ 00733 00734 #define SCB_CCSIDR_WB_Pos 30 /*!< SCB CCSIDR: WB Position */ 00735 #define SCB_CCSIDR_WB_Msk (7UL << SCB_CCSIDR_WB_Pos) /*!< SCB CCSIDR: WB Mask */ 00736 00737 #define SCB_CCSIDR_RA_Pos 29 /*!< SCB CCSIDR: RA Position */ 00738 #define SCB_CCSIDR_RA_Msk (7UL << SCB_CCSIDR_RA_Pos) /*!< SCB CCSIDR: RA Mask */ 00739 00740 #define SCB_CCSIDR_WA_Pos 28 /*!< SCB CCSIDR: WA Position */ 00741 #define SCB_CCSIDR_WA_Msk (7UL << SCB_CCSIDR_WA_Pos) /*!< SCB CCSIDR: WA Mask */ 00742 00743 #define SCB_CCSIDR_NUMSETS_Pos 13 /*!< SCB CCSIDR: NumSets Position */ 00744 #define SCB_CCSIDR_NUMSETS_Msk (0x7FFFUL << SCB_CCSIDR_NUMSETS_Pos) /*!< SCB CCSIDR: NumSets Mask */ 00745 00746 #define SCB_CCSIDR_ASSOCIATIVITY_Pos 3 /*!< SCB CCSIDR: Associativity Position */ 00747 #define SCB_CCSIDR_ASSOCIATIVITY_Msk (0x3FFUL << SCB_CCSIDR_ASSOCIATIVITY_Pos) /*!< SCB CCSIDR: Associativity Mask */ 00748 00749 #define SCB_CCSIDR_LINESIZE_Pos 0 /*!< SCB CCSIDR: LineSize Position */ 00750 #define SCB_CCSIDR_LINESIZE_Msk (7UL /*<< SCB_CCSIDR_LINESIZE_Pos*/) /*!< SCB CCSIDR: LineSize Mask */ 00751 00752 /* Cache Size Selection Register */ 00753 #define SCB_CSSELR_LEVEL_Pos 1 /*!< SCB CSSELR: Level Position */ 00754 #define SCB_CSSELR_LEVEL_Msk (7UL << SCB_CSSELR_LEVEL_Pos) /*!< SCB CSSELR: Level Mask */ 00755 00756 #define SCB_CSSELR_IND_Pos 0 /*!< SCB CSSELR: InD Position */ 00757 #define SCB_CSSELR_IND_Msk (1UL /*<< SCB_CSSELR_IND_Pos*/) /*!< SCB CSSELR: InD Mask */ 00758 00759 /* SCB Software Triggered Interrupt Register */ 00760 #define SCB_STIR_INTID_Pos 0 /*!< SCB STIR: INTID Position */ 00761 #define SCB_STIR_INTID_Msk (0x1FFUL /*<< SCB_STIR_INTID_Pos*/) /*!< SCB STIR: INTID Mask */ 00762 00763 /* Instruction Tightly-Coupled Memory Control Register*/ 00764 #define SCB_ITCMCR_SZ_Pos 3 /*!< SCB ITCMCR: SZ Position */ 00765 #define SCB_ITCMCR_SZ_Msk (0xFUL << SCB_ITCMCR_SZ_Pos) /*!< SCB ITCMCR: SZ Mask */ 00766 00767 #define SCB_ITCMCR_RETEN_Pos 2 /*!< SCB ITCMCR: RETEN Position */ 00768 #define SCB_ITCMCR_RETEN_Msk (1UL << SCB_ITCMCR_RETEN_Pos) /*!< SCB ITCMCR: RETEN Mask */ 00769 00770 #define SCB_ITCMCR_RMW_Pos 1 /*!< SCB ITCMCR: RMW Position */ 00771 #define SCB_ITCMCR_RMW_Msk (1UL << SCB_ITCMCR_RMW_Pos) /*!< SCB ITCMCR: RMW Mask */ 00772 00773 #define SCB_ITCMCR_EN_Pos 0 /*!< SCB ITCMCR: EN Position */ 00774 #define SCB_ITCMCR_EN_Msk (1UL /*<< SCB_ITCMCR_EN_Pos*/) /*!< SCB ITCMCR: EN Mask */ 00775 00776 /* Data Tightly-Coupled Memory Control Registers */ 00777 #define SCB_DTCMCR_SZ_Pos 3 /*!< SCB DTCMCR: SZ Position */ 00778 #define SCB_DTCMCR_SZ_Msk (0xFUL << SCB_DTCMCR_SZ_Pos) /*!< SCB DTCMCR: SZ Mask */ 00779 00780 #define SCB_DTCMCR_RETEN_Pos 2 /*!< SCB DTCMCR: RETEN Position */ 00781 #define SCB_DTCMCR_RETEN_Msk (1UL << SCB_DTCMCR_RETEN_Pos) /*!< SCB DTCMCR: RETEN Mask */ 00782 00783 #define SCB_DTCMCR_RMW_Pos 1 /*!< SCB DTCMCR: RMW Position */ 00784 #define SCB_DTCMCR_RMW_Msk (1UL << SCB_DTCMCR_RMW_Pos) /*!< SCB DTCMCR: RMW Mask */ 00785 00786 #define SCB_DTCMCR_EN_Pos 0 /*!< SCB DTCMCR: EN Position */ 00787 #define SCB_DTCMCR_EN_Msk (1UL /*<< SCB_DTCMCR_EN_Pos*/) /*!< SCB DTCMCR: EN Mask */ 00788 00789 /* AHBP Control Register */ 00790 #define SCB_AHBPCR_SZ_Pos 1 /*!< SCB AHBPCR: SZ Position */ 00791 #define SCB_AHBPCR_SZ_Msk (7UL << SCB_AHBPCR_SZ_Pos) /*!< SCB AHBPCR: SZ Mask */ 00792 00793 #define SCB_AHBPCR_EN_Pos 0 /*!< SCB AHBPCR: EN Position */ 00794 #define SCB_AHBPCR_EN_Msk (1UL /*<< SCB_AHBPCR_EN_Pos*/) /*!< SCB AHBPCR: EN Mask */ 00795 00796 /* L1 Cache Control Register */ 00797 #define SCB_CACR_FORCEWT_Pos 2 /*!< SCB CACR: FORCEWT Position */ 00798 #define SCB_CACR_FORCEWT_Msk (1UL << SCB_CACR_FORCEWT_Pos) /*!< SCB CACR: FORCEWT Mask */ 00799 00800 #define SCB_CACR_ECCEN_Pos 1 /*!< SCB CACR: ECCEN Position */ 00801 #define SCB_CACR_ECCEN_Msk (1UL << SCB_CACR_ECCEN_Pos) /*!< SCB CACR: ECCEN Mask */ 00802 00803 #define SCB_CACR_SIWT_Pos 0 /*!< SCB CACR: SIWT Position */ 00804 #define SCB_CACR_SIWT_Msk (1UL /*<< SCB_CACR_SIWT_Pos*/) /*!< SCB CACR: SIWT Mask */ 00805 00806 /* AHBS control register */ 00807 #define SCB_AHBSCR_INITCOUNT_Pos 11 /*!< SCB AHBSCR: INITCOUNT Position */ 00808 #define SCB_AHBSCR_INITCOUNT_Msk (0x1FUL << SCB_AHBPCR_INITCOUNT_Pos) /*!< SCB AHBSCR: INITCOUNT Mask */ 00809 00810 #define SCB_AHBSCR_TPRI_Pos 2 /*!< SCB AHBSCR: TPRI Position */ 00811 #define SCB_AHBSCR_TPRI_Msk (0x1FFUL << SCB_AHBPCR_TPRI_Pos) /*!< SCB AHBSCR: TPRI Mask */ 00812 00813 #define SCB_AHBSCR_CTL_Pos 0 /*!< SCB AHBSCR: CTL Position*/ 00814 #define SCB_AHBSCR_CTL_Msk (3UL /*<< SCB_AHBPCR_CTL_Pos*/) /*!< SCB AHBSCR: CTL Mask */ 00815 00816 /* Auxiliary Bus Fault Status Register */ 00817 #define SCB_ABFSR_AXIMTYPE_Pos 8 /*!< SCB ABFSR: AXIMTYPE Position*/ 00818 #define SCB_ABFSR_AXIMTYPE_Msk (3UL << SCB_ABFSR_AXIMTYPE_Pos) /*!< SCB ABFSR: AXIMTYPE Mask */ 00819 00820 #define SCB_ABFSR_EPPB_Pos 4 /*!< SCB ABFSR: EPPB Position*/ 00821 #define SCB_ABFSR_EPPB_Msk (1UL << SCB_ABFSR_EPPB_Pos) /*!< SCB ABFSR: EPPB Mask */ 00822 00823 #define SCB_ABFSR_AXIM_Pos 3 /*!< SCB ABFSR: AXIM Position*/ 00824 #define SCB_ABFSR_AXIM_Msk (1UL << SCB_ABFSR_AXIM_Pos) /*!< SCB ABFSR: AXIM Mask */ 00825 00826 #define SCB_ABFSR_AHBP_Pos 2 /*!< SCB ABFSR: AHBP Position*/ 00827 #define SCB_ABFSR_AHBP_Msk (1UL << SCB_ABFSR_AHBP_Pos) /*!< SCB ABFSR: AHBP Mask */ 00828 00829 #define SCB_ABFSR_DTCM_Pos 1 /*!< SCB ABFSR: DTCM Position*/ 00830 #define SCB_ABFSR_DTCM_Msk (1UL << SCB_ABFSR_DTCM_Pos) /*!< SCB ABFSR: DTCM Mask */ 00831 00832 #define SCB_ABFSR_ITCM_Pos 0 /*!< SCB ABFSR: ITCM Position*/ 00833 #define SCB_ABFSR_ITCM_Msk (1UL /*<< SCB_ABFSR_ITCM_Pos*/) /*!< SCB ABFSR: ITCM Mask */ 00834 00835 /*@} end of group CMSIS_SCB */ 00836 00837 00838 /** \ingroup CMSIS_core_register 00839 \defgroup CMSIS_SCnSCB System Controls not in SCB (SCnSCB) 00840 \brief Type definitions for the System Control and ID Register not in the SCB 00841 @{ 00842 */ 00843 00844 /** \brief Structure type to access the System Control and ID Register not in the SCB. 00845 */ 00846 typedef struct 00847 { 00848 uint32_t RESERVED0[1]; 00849 __I uint32_t ICTR; /*!< Offset: 0x004 (R/ ) Interrupt Controller Type Register */ 00850 __IO uint32_t ACTLR; /*!< Offset: 0x008 (R/W) Auxiliary Control Register */ 00851 } SCnSCB_Type; 00852 00853 /* Interrupt Controller Type Register Definitions */ 00854 #define SCnSCB_ICTR_INTLINESNUM_Pos 0 /*!< ICTR: INTLINESNUM Position */ 00855 #define SCnSCB_ICTR_INTLINESNUM_Msk (0xFUL /*<< SCnSCB_ICTR_INTLINESNUM_Pos*/) /*!< ICTR: INTLINESNUM Mask */ 00856 00857 /* Auxiliary Control Register Definitions */ 00858 #define SCnSCB_ACTLR_DISITMATBFLUSH_Pos 12 /*!< ACTLR: DISITMATBFLUSH Position */ 00859 #define SCnSCB_ACTLR_DISITMATBFLUSH_Msk (1UL << SCnSCB_ACTLR_DISITMATBFLUSH_Pos) /*!< ACTLR: DISITMATBFLUSH Mask */ 00860 00861 #define SCnSCB_ACTLR_DISRAMODE_Pos 11 /*!< ACTLR: DISRAMODE Position */ 00862 #define SCnSCB_ACTLR_DISRAMODE_Msk (1UL << SCnSCB_ACTLR_DISRAMODE_Pos) /*!< ACTLR: DISRAMODE Mask */ 00863 00864 #define SCnSCB_ACTLR_FPEXCODIS_Pos 10 /*!< ACTLR: FPEXCODIS Position */ 00865 #define SCnSCB_ACTLR_FPEXCODIS_Msk (1UL << SCnSCB_ACTLR_FPEXCODIS_Pos) /*!< ACTLR: FPEXCODIS Mask */ 00866 00867 #define SCnSCB_ACTLR_DISFOLD_Pos 2 /*!< ACTLR: DISFOLD Position */ 00868 #define SCnSCB_ACTLR_DISFOLD_Msk (1UL << SCnSCB_ACTLR_DISFOLD_Pos) /*!< ACTLR: DISFOLD Mask */ 00869 00870 #define SCnSCB_ACTLR_DISMCYCINT_Pos 0 /*!< ACTLR: DISMCYCINT Position */ 00871 #define SCnSCB_ACTLR_DISMCYCINT_Msk (1UL /*<< SCnSCB_ACTLR_DISMCYCINT_Pos*/) /*!< ACTLR: DISMCYCINT Mask */ 00872 00873 /*@} end of group CMSIS_SCnotSCB */ 00874 00875 00876 /** \ingroup CMSIS_core_register 00877 \defgroup CMSIS_SysTick System Tick Timer (SysTick) 00878 \brief Type definitions for the System Timer Registers. 00879 @{ 00880 */ 00881 00882 /** \brief Structure type to access the System Timer (SysTick). 00883 */ 00884 typedef struct 00885 { 00886 __IO uint32_t CTRL; /*!< Offset: 0x000 (R/W) SysTick Control and Status Register */ 00887 __IO uint32_t LOAD; /*!< Offset: 0x004 (R/W) SysTick Reload Value Register */ 00888 __IO uint32_t VAL; /*!< Offset: 0x008 (R/W) SysTick Current Value Register */ 00889 __I uint32_t CALIB; /*!< Offset: 0x00C (R/ ) SysTick Calibration Register */ 00890 } SysTick_Type; 00891 00892 /* SysTick Control / Status Register Definitions */ 00893 #define SysTick_CTRL_COUNTFLAG_Pos 16 /*!< SysTick CTRL: COUNTFLAG Position */ 00894 #define SysTick_CTRL_COUNTFLAG_Msk (1UL << SysTick_CTRL_COUNTFLAG_Pos) /*!< SysTick CTRL: COUNTFLAG Mask */ 00895 00896 #define SysTick_CTRL_CLKSOURCE_Pos 2 /*!< SysTick CTRL: CLKSOURCE Position */ 00897 #define SysTick_CTRL_CLKSOURCE_Msk (1UL << SysTick_CTRL_CLKSOURCE_Pos) /*!< SysTick CTRL: CLKSOURCE Mask */ 00898 00899 #define SysTick_CTRL_TICKINT_Pos 1 /*!< SysTick CTRL: TICKINT Position */ 00900 #define SysTick_CTRL_TICKINT_Msk (1UL << SysTick_CTRL_TICKINT_Pos) /*!< SysTick CTRL: TICKINT Mask */ 00901 00902 #define SysTick_CTRL_ENABLE_Pos 0 /*!< SysTick CTRL: ENABLE Position */ 00903 #define SysTick_CTRL_ENABLE_Msk (1UL /*<< SysTick_CTRL_ENABLE_Pos*/) /*!< SysTick CTRL: ENABLE Mask */ 00904 00905 /* SysTick Reload Register Definitions */ 00906 #define SysTick_LOAD_RELOAD_Pos 0 /*!< SysTick LOAD: RELOAD Position */ 00907 #define SysTick_LOAD_RELOAD_Msk (0xFFFFFFUL /*<< SysTick_LOAD_RELOAD_Pos*/) /*!< SysTick LOAD: RELOAD Mask */ 00908 00909 /* SysTick Current Register Definitions */ 00910 #define SysTick_VAL_CURRENT_Pos 0 /*!< SysTick VAL: CURRENT Position */ 00911 #define SysTick_VAL_CURRENT_Msk (0xFFFFFFUL /*<< SysTick_VAL_CURRENT_Pos*/) /*!< SysTick VAL: CURRENT Mask */ 00912 00913 /* SysTick Calibration Register Definitions */ 00914 #define SysTick_CALIB_NOREF_Pos 31 /*!< SysTick CALIB: NOREF Position */ 00915 #define SysTick_CALIB_NOREF_Msk (1UL << SysTick_CALIB_NOREF_Pos) /*!< SysTick CALIB: NOREF Mask */ 00916 00917 #define SysTick_CALIB_SKEW_Pos 30 /*!< SysTick CALIB: SKEW Position */ 00918 #define SysTick_CALIB_SKEW_Msk (1UL << SysTick_CALIB_SKEW_Pos) /*!< SysTick CALIB: SKEW Mask */ 00919 00920 #define SysTick_CALIB_TENMS_Pos 0 /*!< SysTick CALIB: TENMS Position */ 00921 #define SysTick_CALIB_TENMS_Msk (0xFFFFFFUL /*<< SysTick_CALIB_TENMS_Pos*/) /*!< SysTick CALIB: TENMS Mask */ 00922 00923 /*@} end of group CMSIS_SysTick */ 00924 00925 00926 /** \ingroup CMSIS_core_register 00927 \defgroup CMSIS_ITM Instrumentation Trace Macrocell (ITM) 00928 \brief Type definitions for the Instrumentation Trace Macrocell (ITM) 00929 @{ 00930 */ 00931 00932 /** \brief Structure type to access the Instrumentation Trace Macrocell Register (ITM). 00933 */ 00934 typedef struct 00935 { 00936 __O union 00937 { 00938 __O uint8_t u8; /*!< Offset: 0x000 ( /W) ITM Stimulus Port 8-bit */ 00939 __O uint16_t u16; /*!< Offset: 0x000 ( /W) ITM Stimulus Port 16-bit */ 00940 __O uint32_t u32; /*!< Offset: 0x000 ( /W) ITM Stimulus Port 32-bit */ 00941 } PORT [32]; /*!< Offset: 0x000 ( /W) ITM Stimulus Port Registers */ 00942 uint32_t RESERVED0[864]; 00943 __IO uint32_t TER; /*!< Offset: 0xE00 (R/W) ITM Trace Enable Register */ 00944 uint32_t RESERVED1[15]; 00945 __IO uint32_t TPR; /*!< Offset: 0xE40 (R/W) ITM Trace Privilege Register */ 00946 uint32_t RESERVED2[15]; 00947 __IO uint32_t TCR; /*!< Offset: 0xE80 (R/W) ITM Trace Control Register */ 00948 uint32_t RESERVED3[29]; 00949 __O uint32_t IWR; /*!< Offset: 0xEF8 ( /W) ITM Integration Write Register */ 00950 __I uint32_t IRR; /*!< Offset: 0xEFC (R/ ) ITM Integration Read Register */ 00951 __IO uint32_t IMCR; /*!< Offset: 0xF00 (R/W) ITM Integration Mode Control Register */ 00952 uint32_t RESERVED4[43]; 00953 __O uint32_t LAR; /*!< Offset: 0xFB0 ( /W) ITM Lock Access Register */ 00954 __I uint32_t LSR; /*!< Offset: 0xFB4 (R/ ) ITM Lock Status Register */ 00955 uint32_t RESERVED5[6]; 00956 __I uint32_t PID4; /*!< Offset: 0xFD0 (R/ ) ITM Peripheral Identification Register #4 */ 00957 __I uint32_t PID5; /*!< Offset: 0xFD4 (R/ ) ITM Peripheral Identification Register #5 */ 00958 __I uint32_t PID6; /*!< Offset: 0xFD8 (R/ ) ITM Peripheral Identification Register #6 */ 00959 __I uint32_t PID7; /*!< Offset: 0xFDC (R/ ) ITM Peripheral Identification Register #7 */ 00960 __I uint32_t PID0; /*!< Offset: 0xFE0 (R/ ) ITM Peripheral Identification Register #0 */ 00961 __I uint32_t PID1; /*!< Offset: 0xFE4 (R/ ) ITM Peripheral Identification Register #1 */ 00962 __I uint32_t PID2; /*!< Offset: 0xFE8 (R/ ) ITM Peripheral Identification Register #2 */ 00963 __I uint32_t PID3; /*!< Offset: 0xFEC (R/ ) ITM Peripheral Identification Register #3 */ 00964 __I uint32_t CID0; /*!< Offset: 0xFF0 (R/ ) ITM Component Identification Register #0 */ 00965 __I uint32_t CID1; /*!< Offset: 0xFF4 (R/ ) ITM Component Identification Register #1 */ 00966 __I uint32_t CID2; /*!< Offset: 0xFF8 (R/ ) ITM Component Identification Register #2 */ 00967 __I uint32_t CID3; /*!< Offset: 0xFFC (R/ ) ITM Component Identification Register #3 */ 00968 } ITM_Type; 00969 00970 /* ITM Trace Privilege Register Definitions */ 00971 #define ITM_TPR_PRIVMASK_Pos 0 /*!< ITM TPR: PRIVMASK Position */ 00972 #define ITM_TPR_PRIVMASK_Msk (0xFUL /*<< ITM_TPR_PRIVMASK_Pos*/) /*!< ITM TPR: PRIVMASK Mask */ 00973 00974 /* ITM Trace Control Register Definitions */ 00975 #define ITM_TCR_BUSY_Pos 23 /*!< ITM TCR: BUSY Position */ 00976 #define ITM_TCR_BUSY_Msk (1UL << ITM_TCR_BUSY_Pos) /*!< ITM TCR: BUSY Mask */ 00977 00978 #define ITM_TCR_TraceBusID_Pos 16 /*!< ITM TCR: ATBID Position */ 00979 #define ITM_TCR_TraceBusID_Msk (0x7FUL << ITM_TCR_TraceBusID_Pos) /*!< ITM TCR: ATBID Mask */ 00980 00981 #define ITM_TCR_GTSFREQ_Pos 10 /*!< ITM TCR: Global timestamp frequency Position */ 00982 #define ITM_TCR_GTSFREQ_Msk (3UL << ITM_TCR_GTSFREQ_Pos) /*!< ITM TCR: Global timestamp frequency Mask */ 00983 00984 #define ITM_TCR_TSPrescale_Pos 8 /*!< ITM TCR: TSPrescale Position */ 00985 #define ITM_TCR_TSPrescale_Msk (3UL << ITM_TCR_TSPrescale_Pos) /*!< ITM TCR: TSPrescale Mask */ 00986 00987 #define ITM_TCR_SWOENA_Pos 4 /*!< ITM TCR: SWOENA Position */ 00988 #define ITM_TCR_SWOENA_Msk (1UL << ITM_TCR_SWOENA_Pos) /*!< ITM TCR: SWOENA Mask */ 00989 00990 #define ITM_TCR_DWTENA_Pos 3 /*!< ITM TCR: DWTENA Position */ 00991 #define ITM_TCR_DWTENA_Msk (1UL << ITM_TCR_DWTENA_Pos) /*!< ITM TCR: DWTENA Mask */ 00992 00993 #define ITM_TCR_SYNCENA_Pos 2 /*!< ITM TCR: SYNCENA Position */ 00994 #define ITM_TCR_SYNCENA_Msk (1UL << ITM_TCR_SYNCENA_Pos) /*!< ITM TCR: SYNCENA Mask */ 00995 00996 #define ITM_TCR_TSENA_Pos 1 /*!< ITM TCR: TSENA Position */ 00997 #define ITM_TCR_TSENA_Msk (1UL << ITM_TCR_TSENA_Pos) /*!< ITM TCR: TSENA Mask */ 00998 00999 #define ITM_TCR_ITMENA_Pos 0 /*!< ITM TCR: ITM Enable bit Position */ 01000 #define ITM_TCR_ITMENA_Msk (1UL /*<< ITM_TCR_ITMENA_Pos*/) /*!< ITM TCR: ITM Enable bit Mask */ 01001 01002 /* ITM Integration Write Register Definitions */ 01003 #define ITM_IWR_ATVALIDM_Pos 0 /*!< ITM IWR: ATVALIDM Position */ 01004 #define ITM_IWR_ATVALIDM_Msk (1UL /*<< ITM_IWR_ATVALIDM_Pos*/) /*!< ITM IWR: ATVALIDM Mask */ 01005 01006 /* ITM Integration Read Register Definitions */ 01007 #define ITM_IRR_ATREADYM_Pos 0 /*!< ITM IRR: ATREADYM Position */ 01008 #define ITM_IRR_ATREADYM_Msk (1UL /*<< ITM_IRR_ATREADYM_Pos*/) /*!< ITM IRR: ATREADYM Mask */ 01009 01010 /* ITM Integration Mode Control Register Definitions */ 01011 #define ITM_IMCR_INTEGRATION_Pos 0 /*!< ITM IMCR: INTEGRATION Position */ 01012 #define ITM_IMCR_INTEGRATION_Msk (1UL /*<< ITM_IMCR_INTEGRATION_Pos*/) /*!< ITM IMCR: INTEGRATION Mask */ 01013 01014 /* ITM Lock Status Register Definitions */ 01015 #define ITM_LSR_ByteAcc_Pos 2 /*!< ITM LSR: ByteAcc Position */ 01016 #define ITM_LSR_ByteAcc_Msk (1UL << ITM_LSR_ByteAcc_Pos) /*!< ITM LSR: ByteAcc Mask */ 01017 01018 #define ITM_LSR_Access_Pos 1 /*!< ITM LSR: Access Position */ 01019 #define ITM_LSR_Access_Msk (1UL << ITM_LSR_Access_Pos) /*!< ITM LSR: Access Mask */ 01020 01021 #define ITM_LSR_Present_Pos 0 /*!< ITM LSR: Present Position */ 01022 #define ITM_LSR_Present_Msk (1UL /*<< ITM_LSR_Present_Pos*/) /*!< ITM LSR: Present Mask */ 01023 01024 /*@}*/ /* end of group CMSIS_ITM */ 01025 01026 01027 /** \ingroup CMSIS_core_register 01028 \defgroup CMSIS_DWT Data Watchpoint and Trace (DWT) 01029 \brief Type definitions for the Data Watchpoint and Trace (DWT) 01030 @{ 01031 */ 01032 01033 /** \brief Structure type to access the Data Watchpoint and Trace Register (DWT). 01034 */ 01035 typedef struct 01036 { 01037 __IO uint32_t CTRL; /*!< Offset: 0x000 (R/W) Control Register */ 01038 __IO uint32_t CYCCNT; /*!< Offset: 0x004 (R/W) Cycle Count Register */ 01039 __IO uint32_t CPICNT; /*!< Offset: 0x008 (R/W) CPI Count Register */ 01040 __IO uint32_t EXCCNT; /*!< Offset: 0x00C (R/W) Exception Overhead Count Register */ 01041 __IO uint32_t SLEEPCNT; /*!< Offset: 0x010 (R/W) Sleep Count Register */ 01042 __IO uint32_t LSUCNT; /*!< Offset: 0x014 (R/W) LSU Count Register */ 01043 __IO uint32_t FOLDCNT; /*!< Offset: 0x018 (R/W) Folded-instruction Count Register */ 01044 __I uint32_t PCSR; /*!< Offset: 0x01C (R/ ) Program Counter Sample Register */ 01045 __IO uint32_t COMP0; /*!< Offset: 0x020 (R/W) Comparator Register 0 */ 01046 __IO uint32_t MASK0; /*!< Offset: 0x024 (R/W) Mask Register 0 */ 01047 __IO uint32_t FUNCTION0; /*!< Offset: 0x028 (R/W) Function Register 0 */ 01048 uint32_t RESERVED0[1]; 01049 __IO uint32_t COMP1; /*!< Offset: 0x030 (R/W) Comparator Register 1 */ 01050 __IO uint32_t MASK1; /*!< Offset: 0x034 (R/W) Mask Register 1 */ 01051 __IO uint32_t FUNCTION1; /*!< Offset: 0x038 (R/W) Function Register 1 */ 01052 uint32_t RESERVED1[1]; 01053 __IO uint32_t COMP2; /*!< Offset: 0x040 (R/W) Comparator Register 2 */ 01054 __IO uint32_t MASK2; /*!< Offset: 0x044 (R/W) Mask Register 2 */ 01055 __IO uint32_t FUNCTION2; /*!< Offset: 0x048 (R/W) Function Register 2 */ 01056 uint32_t RESERVED2[1]; 01057 __IO uint32_t COMP3; /*!< Offset: 0x050 (R/W) Comparator Register 3 */ 01058 __IO uint32_t MASK3; /*!< Offset: 0x054 (R/W) Mask Register 3 */ 01059 __IO uint32_t FUNCTION3; /*!< Offset: 0x058 (R/W) Function Register 3 */ 01060 uint32_t RESERVED3[981]; 01061 __O uint32_t LAR; /*!< Offset: 0xFB0 ( W) Lock Access Register */ 01062 __I uint32_t LSR; /*!< Offset: 0xFB4 (R ) Lock Status Register */ 01063 } DWT_Type; 01064 01065 /* DWT Control Register Definitions */ 01066 #define DWT_CTRL_NUMCOMP_Pos 28 /*!< DWT CTRL: NUMCOMP Position */ 01067 #define DWT_CTRL_NUMCOMP_Msk (0xFUL << DWT_CTRL_NUMCOMP_Pos) /*!< DWT CTRL: NUMCOMP Mask */ 01068 01069 #define DWT_CTRL_NOTRCPKT_Pos 27 /*!< DWT CTRL: NOTRCPKT Position */ 01070 #define DWT_CTRL_NOTRCPKT_Msk (0x1UL << DWT_CTRL_NOTRCPKT_Pos) /*!< DWT CTRL: NOTRCPKT Mask */ 01071 01072 #define DWT_CTRL_NOEXTTRIG_Pos 26 /*!< DWT CTRL: NOEXTTRIG Position */ 01073 #define DWT_CTRL_NOEXTTRIG_Msk (0x1UL << DWT_CTRL_NOEXTTRIG_Pos) /*!< DWT CTRL: NOEXTTRIG Mask */ 01074 01075 #define DWT_CTRL_NOCYCCNT_Pos 25 /*!< DWT CTRL: NOCYCCNT Position */ 01076 #define DWT_CTRL_NOCYCCNT_Msk (0x1UL << DWT_CTRL_NOCYCCNT_Pos) /*!< DWT CTRL: NOCYCCNT Mask */ 01077 01078 #define DWT_CTRL_NOPRFCNT_Pos 24 /*!< DWT CTRL: NOPRFCNT Position */ 01079 #define DWT_CTRL_NOPRFCNT_Msk (0x1UL << DWT_CTRL_NOPRFCNT_Pos) /*!< DWT CTRL: NOPRFCNT Mask */ 01080 01081 #define DWT_CTRL_CYCEVTENA_Pos 22 /*!< DWT CTRL: CYCEVTENA Position */ 01082 #define DWT_CTRL_CYCEVTENA_Msk (0x1UL << DWT_CTRL_CYCEVTENA_Pos) /*!< DWT CTRL: CYCEVTENA Mask */ 01083 01084 #define DWT_CTRL_FOLDEVTENA_Pos 21 /*!< DWT CTRL: FOLDEVTENA Position */ 01085 #define DWT_CTRL_FOLDEVTENA_Msk (0x1UL << DWT_CTRL_FOLDEVTENA_Pos) /*!< DWT CTRL: FOLDEVTENA Mask */ 01086 01087 #define DWT_CTRL_LSUEVTENA_Pos 20 /*!< DWT CTRL: LSUEVTENA Position */ 01088 #define DWT_CTRL_LSUEVTENA_Msk (0x1UL << DWT_CTRL_LSUEVTENA_Pos) /*!< DWT CTRL: LSUEVTENA Mask */ 01089 01090 #define DWT_CTRL_SLEEPEVTENA_Pos 19 /*!< DWT CTRL: SLEEPEVTENA Position */ 01091 #define DWT_CTRL_SLEEPEVTENA_Msk (0x1UL << DWT_CTRL_SLEEPEVTENA_Pos) /*!< DWT CTRL: SLEEPEVTENA Mask */ 01092 01093 #define DWT_CTRL_EXCEVTENA_Pos 18 /*!< DWT CTRL: EXCEVTENA Position */ 01094 #define DWT_CTRL_EXCEVTENA_Msk (0x1UL << DWT_CTRL_EXCEVTENA_Pos) /*!< DWT CTRL: EXCEVTENA Mask */ 01095 01096 #define DWT_CTRL_CPIEVTENA_Pos 17 /*!< DWT CTRL: CPIEVTENA Position */ 01097 #define DWT_CTRL_CPIEVTENA_Msk (0x1UL << DWT_CTRL_CPIEVTENA_Pos) /*!< DWT CTRL: CPIEVTENA Mask */ 01098 01099 #define DWT_CTRL_EXCTRCENA_Pos 16 /*!< DWT CTRL: EXCTRCENA Position */ 01100 #define DWT_CTRL_EXCTRCENA_Msk (0x1UL << DWT_CTRL_EXCTRCENA_Pos) /*!< DWT CTRL: EXCTRCENA Mask */ 01101 01102 #define DWT_CTRL_PCSAMPLENA_Pos 12 /*!< DWT CTRL: PCSAMPLENA Position */ 01103 #define DWT_CTRL_PCSAMPLENA_Msk (0x1UL << DWT_CTRL_PCSAMPLENA_Pos) /*!< DWT CTRL: PCSAMPLENA Mask */ 01104 01105 #define DWT_CTRL_SYNCTAP_Pos 10 /*!< DWT CTRL: SYNCTAP Position */ 01106 #define DWT_CTRL_SYNCTAP_Msk (0x3UL << DWT_CTRL_SYNCTAP_Pos) /*!< DWT CTRL: SYNCTAP Mask */ 01107 01108 #define DWT_CTRL_CYCTAP_Pos 9 /*!< DWT CTRL: CYCTAP Position */ 01109 #define DWT_CTRL_CYCTAP_Msk (0x1UL << DWT_CTRL_CYCTAP_Pos) /*!< DWT CTRL: CYCTAP Mask */ 01110 01111 #define DWT_CTRL_POSTINIT_Pos 5 /*!< DWT CTRL: POSTINIT Position */ 01112 #define DWT_CTRL_POSTINIT_Msk (0xFUL << DWT_CTRL_POSTINIT_Pos) /*!< DWT CTRL: POSTINIT Mask */ 01113 01114 #define DWT_CTRL_POSTPRESET_Pos 1 /*!< DWT CTRL: POSTPRESET Position */ 01115 #define DWT_CTRL_POSTPRESET_Msk (0xFUL << DWT_CTRL_POSTPRESET_Pos) /*!< DWT CTRL: POSTPRESET Mask */ 01116 01117 #define DWT_CTRL_CYCCNTENA_Pos 0 /*!< DWT CTRL: CYCCNTENA Position */ 01118 #define DWT_CTRL_CYCCNTENA_Msk (0x1UL /*<< DWT_CTRL_CYCCNTENA_Pos*/) /*!< DWT CTRL: CYCCNTENA Mask */ 01119 01120 /* DWT CPI Count Register Definitions */ 01121 #define DWT_CPICNT_CPICNT_Pos 0 /*!< DWT CPICNT: CPICNT Position */ 01122 #define DWT_CPICNT_CPICNT_Msk (0xFFUL /*<< DWT_CPICNT_CPICNT_Pos*/) /*!< DWT CPICNT: CPICNT Mask */ 01123 01124 /* DWT Exception Overhead Count Register Definitions */ 01125 #define DWT_EXCCNT_EXCCNT_Pos 0 /*!< DWT EXCCNT: EXCCNT Position */ 01126 #define DWT_EXCCNT_EXCCNT_Msk (0xFFUL /*<< DWT_EXCCNT_EXCCNT_Pos*/) /*!< DWT EXCCNT: EXCCNT Mask */ 01127 01128 /* DWT Sleep Count Register Definitions */ 01129 #define DWT_SLEEPCNT_SLEEPCNT_Pos 0 /*!< DWT SLEEPCNT: SLEEPCNT Position */ 01130 #define DWT_SLEEPCNT_SLEEPCNT_Msk (0xFFUL /*<< DWT_SLEEPCNT_SLEEPCNT_Pos*/) /*!< DWT SLEEPCNT: SLEEPCNT Mask */ 01131 01132 /* DWT LSU Count Register Definitions */ 01133 #define DWT_LSUCNT_LSUCNT_Pos 0 /*!< DWT LSUCNT: LSUCNT Position */ 01134 #define DWT_LSUCNT_LSUCNT_Msk (0xFFUL /*<< DWT_LSUCNT_LSUCNT_Pos*/) /*!< DWT LSUCNT: LSUCNT Mask */ 01135 01136 /* DWT Folded-instruction Count Register Definitions */ 01137 #define DWT_FOLDCNT_FOLDCNT_Pos 0 /*!< DWT FOLDCNT: FOLDCNT Position */ 01138 #define DWT_FOLDCNT_FOLDCNT_Msk (0xFFUL /*<< DWT_FOLDCNT_FOLDCNT_Pos*/) /*!< DWT FOLDCNT: FOLDCNT Mask */ 01139 01140 /* DWT Comparator Mask Register Definitions */ 01141 #define DWT_MASK_MASK_Pos 0 /*!< DWT MASK: MASK Position */ 01142 #define DWT_MASK_MASK_Msk (0x1FUL /*<< DWT_MASK_MASK_Pos*/) /*!< DWT MASK: MASK Mask */ 01143 01144 /* DWT Comparator Function Register Definitions */ 01145 #define DWT_FUNCTION_MATCHED_Pos 24 /*!< DWT FUNCTION: MATCHED Position */ 01146 #define DWT_FUNCTION_MATCHED_Msk (0x1UL << DWT_FUNCTION_MATCHED_Pos) /*!< DWT FUNCTION: MATCHED Mask */ 01147 01148 #define DWT_FUNCTION_DATAVADDR1_Pos 16 /*!< DWT FUNCTION: DATAVADDR1 Position */ 01149 #define DWT_FUNCTION_DATAVADDR1_Msk (0xFUL << DWT_FUNCTION_DATAVADDR1_Pos) /*!< DWT FUNCTION: DATAVADDR1 Mask */ 01150 01151 #define DWT_FUNCTION_DATAVADDR0_Pos 12 /*!< DWT FUNCTION: DATAVADDR0 Position */ 01152 #define DWT_FUNCTION_DATAVADDR0_Msk (0xFUL << DWT_FUNCTION_DATAVADDR0_Pos) /*!< DWT FUNCTION: DATAVADDR0 Mask */ 01153 01154 #define DWT_FUNCTION_DATAVSIZE_Pos 10 /*!< DWT FUNCTION: DATAVSIZE Position */ 01155 #define DWT_FUNCTION_DATAVSIZE_Msk (0x3UL << DWT_FUNCTION_DATAVSIZE_Pos) /*!< DWT FUNCTION: DATAVSIZE Mask */ 01156 01157 #define DWT_FUNCTION_LNK1ENA_Pos 9 /*!< DWT FUNCTION: LNK1ENA Position */ 01158 #define DWT_FUNCTION_LNK1ENA_Msk (0x1UL << DWT_FUNCTION_LNK1ENA_Pos) /*!< DWT FUNCTION: LNK1ENA Mask */ 01159 01160 #define DWT_FUNCTION_DATAVMATCH_Pos 8 /*!< DWT FUNCTION: DATAVMATCH Position */ 01161 #define DWT_FUNCTION_DATAVMATCH_Msk (0x1UL << DWT_FUNCTION_DATAVMATCH_Pos) /*!< DWT FUNCTION: DATAVMATCH Mask */ 01162 01163 #define DWT_FUNCTION_CYCMATCH_Pos 7 /*!< DWT FUNCTION: CYCMATCH Position */ 01164 #define DWT_FUNCTION_CYCMATCH_Msk (0x1UL << DWT_FUNCTION_CYCMATCH_Pos) /*!< DWT FUNCTION: CYCMATCH Mask */ 01165 01166 #define DWT_FUNCTION_EMITRANGE_Pos 5 /*!< DWT FUNCTION: EMITRANGE Position */ 01167 #define DWT_FUNCTION_EMITRANGE_Msk (0x1UL << DWT_FUNCTION_EMITRANGE_Pos) /*!< DWT FUNCTION: EMITRANGE Mask */ 01168 01169 #define DWT_FUNCTION_FUNCTION_Pos 0 /*!< DWT FUNCTION: FUNCTION Position */ 01170 #define DWT_FUNCTION_FUNCTION_Msk (0xFUL /*<< DWT_FUNCTION_FUNCTION_Pos*/) /*!< DWT FUNCTION: FUNCTION Mask */ 01171 01172 /*@}*/ /* end of group CMSIS_DWT */ 01173 01174 01175 /** \ingroup CMSIS_core_register 01176 \defgroup CMSIS_TPI Trace Port Interface (TPI) 01177 \brief Type definitions for the Trace Port Interface (TPI) 01178 @{ 01179 */ 01180 01181 /** \brief Structure type to access the Trace Port Interface Register (TPI). 01182 */ 01183 typedef struct 01184 { 01185 __IO uint32_t SSPSR; /*!< Offset: 0x000 (R/ ) Supported Parallel Port Size Register */ 01186 __IO uint32_t CSPSR; /*!< Offset: 0x004 (R/W) Current Parallel Port Size Register */ 01187 uint32_t RESERVED0[2]; 01188 __IO uint32_t ACPR; /*!< Offset: 0x010 (R/W) Asynchronous Clock Prescaler Register */ 01189 uint32_t RESERVED1[55]; 01190 __IO uint32_t SPPR; /*!< Offset: 0x0F0 (R/W) Selected Pin Protocol Register */ 01191 uint32_t RESERVED2[131]; 01192 __I uint32_t FFSR; /*!< Offset: 0x300 (R/ ) Formatter and Flush Status Register */ 01193 __IO uint32_t FFCR; /*!< Offset: 0x304 (R/W) Formatter and Flush Control Register */ 01194 __I uint32_t FSCR; /*!< Offset: 0x308 (R/ ) Formatter Synchronization Counter Register */ 01195 uint32_t RESERVED3[759]; 01196 __I uint32_t TRIGGER; /*!< Offset: 0xEE8 (R/ ) TRIGGER */ 01197 __I uint32_t FIFO0; /*!< Offset: 0xEEC (R/ ) Integration ETM Data */ 01198 __I uint32_t ITATBCTR2; /*!< Offset: 0xEF0 (R/ ) ITATBCTR2 */ 01199 uint32_t RESERVED4[1]; 01200 __I uint32_t ITATBCTR0; /*!< Offset: 0xEF8 (R/ ) ITATBCTR0 */ 01201 __I uint32_t FIFO1; /*!< Offset: 0xEFC (R/ ) Integration ITM Data */ 01202 __IO uint32_t ITCTRL; /*!< Offset: 0xF00 (R/W) Integration Mode Control */ 01203 uint32_t RESERVED5[39]; 01204 __IO uint32_t CLAIMSET; /*!< Offset: 0xFA0 (R/W) Claim tag set */ 01205 __IO uint32_t CLAIMCLR; /*!< Offset: 0xFA4 (R/W) Claim tag clear */ 01206 uint32_t RESERVED7[8]; 01207 __I uint32_t DEVID; /*!< Offset: 0xFC8 (R/ ) TPIU_DEVID */ 01208 __I uint32_t DEVTYPE; /*!< Offset: 0xFCC (R/ ) TPIU_DEVTYPE */ 01209 } TPI_Type; 01210 01211 /* TPI Asynchronous Clock Prescaler Register Definitions */ 01212 #define TPI_ACPR_PRESCALER_Pos 0 /*!< TPI ACPR: PRESCALER Position */ 01213 #define TPI_ACPR_PRESCALER_Msk (0x1FFFUL /*<< TPI_ACPR_PRESCALER_Pos*/) /*!< TPI ACPR: PRESCALER Mask */ 01214 01215 /* TPI Selected Pin Protocol Register Definitions */ 01216 #define TPI_SPPR_TXMODE_Pos 0 /*!< TPI SPPR: TXMODE Position */ 01217 #define TPI_SPPR_TXMODE_Msk (0x3UL /*<< TPI_SPPR_TXMODE_Pos*/) /*!< TPI SPPR: TXMODE Mask */ 01218 01219 /* TPI Formatter and Flush Status Register Definitions */ 01220 #define TPI_FFSR_FtNonStop_Pos 3 /*!< TPI FFSR: FtNonStop Position */ 01221 #define TPI_FFSR_FtNonStop_Msk (0x1UL << TPI_FFSR_FtNonStop_Pos) /*!< TPI FFSR: FtNonStop Mask */ 01222 01223 #define TPI_FFSR_TCPresent_Pos 2 /*!< TPI FFSR: TCPresent Position */ 01224 #define TPI_FFSR_TCPresent_Msk (0x1UL << TPI_FFSR_TCPresent_Pos) /*!< TPI FFSR: TCPresent Mask */ 01225 01226 #define TPI_FFSR_FtStopped_Pos 1 /*!< TPI FFSR: FtStopped Position */ 01227 #define TPI_FFSR_FtStopped_Msk (0x1UL << TPI_FFSR_FtStopped_Pos) /*!< TPI FFSR: FtStopped Mask */ 01228 01229 #define TPI_FFSR_FlInProg_Pos 0 /*!< TPI FFSR: FlInProg Position */ 01230 #define TPI_FFSR_FlInProg_Msk (0x1UL /*<< TPI_FFSR_FlInProg_Pos*/) /*!< TPI FFSR: FlInProg Mask */ 01231 01232 /* TPI Formatter and Flush Control Register Definitions */ 01233 #define TPI_FFCR_TrigIn_Pos 8 /*!< TPI FFCR: TrigIn Position */ 01234 #define TPI_FFCR_TrigIn_Msk (0x1UL << TPI_FFCR_TrigIn_Pos) /*!< TPI FFCR: TrigIn Mask */ 01235 01236 #define TPI_FFCR_EnFCont_Pos 1 /*!< TPI FFCR: EnFCont Position */ 01237 #define TPI_FFCR_EnFCont_Msk (0x1UL << TPI_FFCR_EnFCont_Pos) /*!< TPI FFCR: EnFCont Mask */ 01238 01239 /* TPI TRIGGER Register Definitions */ 01240 #define TPI_TRIGGER_TRIGGER_Pos 0 /*!< TPI TRIGGER: TRIGGER Position */ 01241 #define TPI_TRIGGER_TRIGGER_Msk (0x1UL /*<< TPI_TRIGGER_TRIGGER_Pos*/) /*!< TPI TRIGGER: TRIGGER Mask */ 01242 01243 /* TPI Integration ETM Data Register Definitions (FIFO0) */ 01244 #define TPI_FIFO0_ITM_ATVALID_Pos 29 /*!< TPI FIFO0: ITM_ATVALID Position */ 01245 #define TPI_FIFO0_ITM_ATVALID_Msk (0x3UL << TPI_FIFO0_ITM_ATVALID_Pos) /*!< TPI FIFO0: ITM_ATVALID Mask */ 01246 01247 #define TPI_FIFO0_ITM_bytecount_Pos 27 /*!< TPI FIFO0: ITM_bytecount Position */ 01248 #define TPI_FIFO0_ITM_bytecount_Msk (0x3UL << TPI_FIFO0_ITM_bytecount_Pos) /*!< TPI FIFO0: ITM_bytecount Mask */ 01249 01250 #define TPI_FIFO0_ETM_ATVALID_Pos 26 /*!< TPI FIFO0: ETM_ATVALID Position */ 01251 #define TPI_FIFO0_ETM_ATVALID_Msk (0x3UL << TPI_FIFO0_ETM_ATVALID_Pos) /*!< TPI FIFO0: ETM_ATVALID Mask */ 01252 01253 #define TPI_FIFO0_ETM_bytecount_Pos 24 /*!< TPI FIFO0: ETM_bytecount Position */ 01254 #define TPI_FIFO0_ETM_bytecount_Msk (0x3UL << TPI_FIFO0_ETM_bytecount_Pos) /*!< TPI FIFO0: ETM_bytecount Mask */ 01255 01256 #define TPI_FIFO0_ETM2_Pos 16 /*!< TPI FIFO0: ETM2 Position */ 01257 #define TPI_FIFO0_ETM2_Msk (0xFFUL << TPI_FIFO0_ETM2_Pos) /*!< TPI FIFO0: ETM2 Mask */ 01258 01259 #define TPI_FIFO0_ETM1_Pos 8 /*!< TPI FIFO0: ETM1 Position */ 01260 #define TPI_FIFO0_ETM1_Msk (0xFFUL << TPI_FIFO0_ETM1_Pos) /*!< TPI FIFO0: ETM1 Mask */ 01261 01262 #define TPI_FIFO0_ETM0_Pos 0 /*!< TPI FIFO0: ETM0 Position */ 01263 #define TPI_FIFO0_ETM0_Msk (0xFFUL /*<< TPI_FIFO0_ETM0_Pos*/) /*!< TPI FIFO0: ETM0 Mask */ 01264 01265 /* TPI ITATBCTR2 Register Definitions */ 01266 #define TPI_ITATBCTR2_ATREADY_Pos 0 /*!< TPI ITATBCTR2: ATREADY Position */ 01267 #define TPI_ITATBCTR2_ATREADY_Msk (0x1UL /*<< TPI_ITATBCTR2_ATREADY_Pos*/) /*!< TPI ITATBCTR2: ATREADY Mask */ 01268 01269 /* TPI Integration ITM Data Register Definitions (FIFO1) */ 01270 #define TPI_FIFO1_ITM_ATVALID_Pos 29 /*!< TPI FIFO1: ITM_ATVALID Position */ 01271 #define TPI_FIFO1_ITM_ATVALID_Msk (0x3UL << TPI_FIFO1_ITM_ATVALID_Pos) /*!< TPI FIFO1: ITM_ATVALID Mask */ 01272 01273 #define TPI_FIFO1_ITM_bytecount_Pos 27 /*!< TPI FIFO1: ITM_bytecount Position */ 01274 #define TPI_FIFO1_ITM_bytecount_Msk (0x3UL << TPI_FIFO1_ITM_bytecount_Pos) /*!< TPI FIFO1: ITM_bytecount Mask */ 01275 01276 #define TPI_FIFO1_ETM_ATVALID_Pos 26 /*!< TPI FIFO1: ETM_ATVALID Position */ 01277 #define TPI_FIFO1_ETM_ATVALID_Msk (0x3UL << TPI_FIFO1_ETM_ATVALID_Pos) /*!< TPI FIFO1: ETM_ATVALID Mask */ 01278 01279 #define TPI_FIFO1_ETM_bytecount_Pos 24 /*!< TPI FIFO1: ETM_bytecount Position */ 01280 #define TPI_FIFO1_ETM_bytecount_Msk (0x3UL << TPI_FIFO1_ETM_bytecount_Pos) /*!< TPI FIFO1: ETM_bytecount Mask */ 01281 01282 #define TPI_FIFO1_ITM2_Pos 16 /*!< TPI FIFO1: ITM2 Position */ 01283 #define TPI_FIFO1_ITM2_Msk (0xFFUL << TPI_FIFO1_ITM2_Pos) /*!< TPI FIFO1: ITM2 Mask */ 01284 01285 #define TPI_FIFO1_ITM1_Pos 8 /*!< TPI FIFO1: ITM1 Position */ 01286 #define TPI_FIFO1_ITM1_Msk (0xFFUL << TPI_FIFO1_ITM1_Pos) /*!< TPI FIFO1: ITM1 Mask */ 01287 01288 #define TPI_FIFO1_ITM0_Pos 0 /*!< TPI FIFO1: ITM0 Position */ 01289 #define TPI_FIFO1_ITM0_Msk (0xFFUL /*<< TPI_FIFO1_ITM0_Pos*/) /*!< TPI FIFO1: ITM0 Mask */ 01290 01291 /* TPI ITATBCTR0 Register Definitions */ 01292 #define TPI_ITATBCTR0_ATREADY_Pos 0 /*!< TPI ITATBCTR0: ATREADY Position */ 01293 #define TPI_ITATBCTR0_ATREADY_Msk (0x1UL /*<< TPI_ITATBCTR0_ATREADY_Pos*/) /*!< TPI ITATBCTR0: ATREADY Mask */ 01294 01295 /* TPI Integration Mode Control Register Definitions */ 01296 #define TPI_ITCTRL_Mode_Pos 0 /*!< TPI ITCTRL: Mode Position */ 01297 #define TPI_ITCTRL_Mode_Msk (0x1UL /*<< TPI_ITCTRL_Mode_Pos*/) /*!< TPI ITCTRL: Mode Mask */ 01298 01299 /* TPI DEVID Register Definitions */ 01300 #define TPI_DEVID_NRZVALID_Pos 11 /*!< TPI DEVID: NRZVALID Position */ 01301 #define TPI_DEVID_NRZVALID_Msk (0x1UL << TPI_DEVID_NRZVALID_Pos) /*!< TPI DEVID: NRZVALID Mask */ 01302 01303 #define TPI_DEVID_MANCVALID_Pos 10 /*!< TPI DEVID: MANCVALID Position */ 01304 #define TPI_DEVID_MANCVALID_Msk (0x1UL << TPI_DEVID_MANCVALID_Pos) /*!< TPI DEVID: MANCVALID Mask */ 01305 01306 #define TPI_DEVID_PTINVALID_Pos 9 /*!< TPI DEVID: PTINVALID Position */ 01307 #define TPI_DEVID_PTINVALID_Msk (0x1UL << TPI_DEVID_PTINVALID_Pos) /*!< TPI DEVID: PTINVALID Mask */ 01308 01309 #define TPI_DEVID_MinBufSz_Pos 6 /*!< TPI DEVID: MinBufSz Position */ 01310 #define TPI_DEVID_MinBufSz_Msk (0x7UL << TPI_DEVID_MinBufSz_Pos) /*!< TPI DEVID: MinBufSz Mask */ 01311 01312 #define TPI_DEVID_AsynClkIn_Pos 5 /*!< TPI DEVID: AsynClkIn Position */ 01313 #define TPI_DEVID_AsynClkIn_Msk (0x1UL << TPI_DEVID_AsynClkIn_Pos) /*!< TPI DEVID: AsynClkIn Mask */ 01314 01315 #define TPI_DEVID_NrTraceInput_Pos 0 /*!< TPI DEVID: NrTraceInput Position */ 01316 #define TPI_DEVID_NrTraceInput_Msk (0x1FUL /*<< TPI_DEVID_NrTraceInput_Pos*/) /*!< TPI DEVID: NrTraceInput Mask */ 01317 01318 /* TPI DEVTYPE Register Definitions */ 01319 #define TPI_DEVTYPE_MajorType_Pos 4 /*!< TPI DEVTYPE: MajorType Position */ 01320 #define TPI_DEVTYPE_MajorType_Msk (0xFUL << TPI_DEVTYPE_MajorType_Pos) /*!< TPI DEVTYPE: MajorType Mask */ 01321 01322 #define TPI_DEVTYPE_SubType_Pos 0 /*!< TPI DEVTYPE: SubType Position */ 01323 #define TPI_DEVTYPE_SubType_Msk (0xFUL /*<< TPI_DEVTYPE_SubType_Pos*/) /*!< TPI DEVTYPE: SubType Mask */ 01324 01325 /*@}*/ /* end of group CMSIS_TPI */ 01326 01327 01328 #if (__MPU_PRESENT == 1) 01329 /** \ingroup CMSIS_core_register 01330 \defgroup CMSIS_MPU Memory Protection Unit (MPU) 01331 \brief Type definitions for the Memory Protection Unit (MPU) 01332 @{ 01333 */ 01334 01335 /** \brief Structure type to access the Memory Protection Unit (MPU). 01336 */ 01337 typedef struct 01338 { 01339 __I uint32_t TYPE; /*!< Offset: 0x000 (R/ ) MPU Type Register */ 01340 __IO uint32_t CTRL; /*!< Offset: 0x004 (R/W) MPU Control Register */ 01341 __IO uint32_t RNR; /*!< Offset: 0x008 (R/W) MPU Region RNRber Register */ 01342 __IO uint32_t RBAR; /*!< Offset: 0x00C (R/W) MPU Region Base Address Register */ 01343 __IO uint32_t RASR; /*!< Offset: 0x010 (R/W) MPU Region Attribute and Size Register */ 01344 __IO uint32_t RBAR_A1; /*!< Offset: 0x014 (R/W) MPU Alias 1 Region Base Address Register */ 01345 __IO uint32_t RASR_A1; /*!< Offset: 0x018 (R/W) MPU Alias 1 Region Attribute and Size Register */ 01346 __IO uint32_t RBAR_A2; /*!< Offset: 0x01C (R/W) MPU Alias 2 Region Base Address Register */ 01347 __IO uint32_t RASR_A2; /*!< Offset: 0x020 (R/W) MPU Alias 2 Region Attribute and Size Register */ 01348 __IO uint32_t RBAR_A3; /*!< Offset: 0x024 (R/W) MPU Alias 3 Region Base Address Register */ 01349 __IO uint32_t RASR_A3; /*!< Offset: 0x028 (R/W) MPU Alias 3 Region Attribute and Size Register */ 01350 } MPU_Type; 01351 01352 /* MPU Type Register */ 01353 #define MPU_TYPE_IREGION_Pos 16 /*!< MPU TYPE: IREGION Position */ 01354 #define MPU_TYPE_IREGION_Msk (0xFFUL << MPU_TYPE_IREGION_Pos) /*!< MPU TYPE: IREGION Mask */ 01355 01356 #define MPU_TYPE_DREGION_Pos 8 /*!< MPU TYPE: DREGION Position */ 01357 #define MPU_TYPE_DREGION_Msk (0xFFUL << MPU_TYPE_DREGION_Pos) /*!< MPU TYPE: DREGION Mask */ 01358 01359 #define MPU_TYPE_SEPARATE_Pos 0 /*!< MPU TYPE: SEPARATE Position */ 01360 #define MPU_TYPE_SEPARATE_Msk (1UL /*<< MPU_TYPE_SEPARATE_Pos*/) /*!< MPU TYPE: SEPARATE Mask */ 01361 01362 /* MPU Control Register */ 01363 #define MPU_CTRL_PRIVDEFENA_Pos 2 /*!< MPU CTRL: PRIVDEFENA Position */ 01364 #define MPU_CTRL_PRIVDEFENA_Msk (1UL << MPU_CTRL_PRIVDEFENA_Pos) /*!< MPU CTRL: PRIVDEFENA Mask */ 01365 01366 #define MPU_CTRL_HFNMIENA_Pos 1 /*!< MPU CTRL: HFNMIENA Position */ 01367 #define MPU_CTRL_HFNMIENA_Msk (1UL << MPU_CTRL_HFNMIENA_Pos) /*!< MPU CTRL: HFNMIENA Mask */ 01368 01369 #define MPU_CTRL_ENABLE_Pos 0 /*!< MPU CTRL: ENABLE Position */ 01370 #define MPU_CTRL_ENABLE_Msk (1UL /*<< MPU_CTRL_ENABLE_Pos*/) /*!< MPU CTRL: ENABLE Mask */ 01371 01372 /* MPU Region Number Register */ 01373 #define MPU_RNR_REGION_Pos 0 /*!< MPU RNR: REGION Position */ 01374 #define MPU_RNR_REGION_Msk (0xFFUL /*<< MPU_RNR_REGION_Pos*/) /*!< MPU RNR: REGION Mask */ 01375 01376 /* MPU Region Base Address Register */ 01377 #define MPU_RBAR_ADDR_Pos 5 /*!< MPU RBAR: ADDR Position */ 01378 #define MPU_RBAR_ADDR_Msk (0x7FFFFFFUL << MPU_RBAR_ADDR_Pos) /*!< MPU RBAR: ADDR Mask */ 01379 01380 #define MPU_RBAR_VALID_Pos 4 /*!< MPU RBAR: VALID Position */ 01381 #define MPU_RBAR_VALID_Msk (1UL << MPU_RBAR_VALID_Pos) /*!< MPU RBAR: VALID Mask */ 01382 01383 #define MPU_RBAR_REGION_Pos 0 /*!< MPU RBAR: REGION Position */ 01384 #define MPU_RBAR_REGION_Msk (0xFUL /*<< MPU_RBAR_REGION_Pos*/) /*!< MPU RBAR: REGION Mask */ 01385 01386 /* MPU Region Attribute and Size Register */ 01387 #define MPU_RASR_ATTRS_Pos 16 /*!< MPU RASR: MPU Region Attribute field Position */ 01388 #define MPU_RASR_ATTRS_Msk (0xFFFFUL << MPU_RASR_ATTRS_Pos) /*!< MPU RASR: MPU Region Attribute field Mask */ 01389 01390 #define MPU_RASR_XN_Pos 28 /*!< MPU RASR: ATTRS.XN Position */ 01391 #define MPU_RASR_XN_Msk (1UL << MPU_RASR_XN_Pos) /*!< MPU RASR: ATTRS.XN Mask */ 01392 01393 #define MPU_RASR_AP_Pos 24 /*!< MPU RASR: ATTRS.AP Position */ 01394 #define MPU_RASR_AP_Msk (0x7UL << MPU_RASR_AP_Pos) /*!< MPU RASR: ATTRS.AP Mask */ 01395 01396 #define MPU_RASR_TEX_Pos 19 /*!< MPU RASR: ATTRS.TEX Position */ 01397 #define MPU_RASR_TEX_Msk (0x7UL << MPU_RASR_TEX_Pos) /*!< MPU RASR: ATTRS.TEX Mask */ 01398 01399 #define MPU_RASR_S_Pos 18 /*!< MPU RASR: ATTRS.S Position */ 01400 #define MPU_RASR_S_Msk (1UL << MPU_RASR_S_Pos) /*!< MPU RASR: ATTRS.S Mask */ 01401 01402 #define MPU_RASR_C_Pos 17 /*!< MPU RASR: ATTRS.C Position */ 01403 #define MPU_RASR_C_Msk (1UL << MPU_RASR_C_Pos) /*!< MPU RASR: ATTRS.C Mask */ 01404 01405 #define MPU_RASR_B_Pos 16 /*!< MPU RASR: ATTRS.B Position */ 01406 #define MPU_RASR_B_Msk (1UL << MPU_RASR_B_Pos) /*!< MPU RASR: ATTRS.B Mask */ 01407 01408 #define MPU_RASR_SRD_Pos 8 /*!< MPU RASR: Sub-Region Disable Position */ 01409 #define MPU_RASR_SRD_Msk (0xFFUL << MPU_RASR_SRD_Pos) /*!< MPU RASR: Sub-Region Disable Mask */ 01410 01411 #define MPU_RASR_SIZE_Pos 1 /*!< MPU RASR: Region Size Field Position */ 01412 #define MPU_RASR_SIZE_Msk (0x1FUL << MPU_RASR_SIZE_Pos) /*!< MPU RASR: Region Size Field Mask */ 01413 01414 #define MPU_RASR_ENABLE_Pos 0 /*!< MPU RASR: Region enable bit Position */ 01415 #define MPU_RASR_ENABLE_Msk (1UL /*<< MPU_RASR_ENABLE_Pos*/) /*!< MPU RASR: Region enable bit Disable Mask */ 01416 01417 /*@} end of group CMSIS_MPU */ 01418 #endif 01419 01420 01421 #if (__FPU_PRESENT == 1) 01422 /** \ingroup CMSIS_core_register 01423 \defgroup CMSIS_FPU Floating Point Unit (FPU) 01424 \brief Type definitions for the Floating Point Unit (FPU) 01425 @{ 01426 */ 01427 01428 /** \brief Structure type to access the Floating Point Unit (FPU). 01429 */ 01430 typedef struct 01431 { 01432 uint32_t RESERVED0[1]; 01433 __IO uint32_t FPCCR; /*!< Offset: 0x004 (R/W) Floating-Point Context Control Register */ 01434 __IO uint32_t FPCAR; /*!< Offset: 0x008 (R/W) Floating-Point Context Address Register */ 01435 __IO uint32_t FPDSCR; /*!< Offset: 0x00C (R/W) Floating-Point Default Status Control Register */ 01436 __I uint32_t MVFR0; /*!< Offset: 0x010 (R/ ) Media and FP Feature Register 0 */ 01437 __I uint32_t MVFR1; /*!< Offset: 0x014 (R/ ) Media and FP Feature Register 1 */ 01438 __I uint32_t MVFR2; /*!< Offset: 0x018 (R/ ) Media and FP Feature Register 2 */ 01439 } FPU_Type; 01440 01441 /* Floating-Point Context Control Register */ 01442 #define FPU_FPCCR_ASPEN_Pos 31 /*!< FPCCR: ASPEN bit Position */ 01443 #define FPU_FPCCR_ASPEN_Msk (1UL << FPU_FPCCR_ASPEN_Pos) /*!< FPCCR: ASPEN bit Mask */ 01444 01445 #define FPU_FPCCR_LSPEN_Pos 30 /*!< FPCCR: LSPEN Position */ 01446 #define FPU_FPCCR_LSPEN_Msk (1UL << FPU_FPCCR_LSPEN_Pos) /*!< FPCCR: LSPEN bit Mask */ 01447 01448 #define FPU_FPCCR_MONRDY_Pos 8 /*!< FPCCR: MONRDY Position */ 01449 #define FPU_FPCCR_MONRDY_Msk (1UL << FPU_FPCCR_MONRDY_Pos) /*!< FPCCR: MONRDY bit Mask */ 01450 01451 #define FPU_FPCCR_BFRDY_Pos 6 /*!< FPCCR: BFRDY Position */ 01452 #define FPU_FPCCR_BFRDY_Msk (1UL << FPU_FPCCR_BFRDY_Pos) /*!< FPCCR: BFRDY bit Mask */ 01453 01454 #define FPU_FPCCR_MMRDY_Pos 5 /*!< FPCCR: MMRDY Position */ 01455 #define FPU_FPCCR_MMRDY_Msk (1UL << FPU_FPCCR_MMRDY_Pos) /*!< FPCCR: MMRDY bit Mask */ 01456 01457 #define FPU_FPCCR_HFRDY_Pos 4 /*!< FPCCR: HFRDY Position */ 01458 #define FPU_FPCCR_HFRDY_Msk (1UL << FPU_FPCCR_HFRDY_Pos) /*!< FPCCR: HFRDY bit Mask */ 01459 01460 #define FPU_FPCCR_THREAD_Pos 3 /*!< FPCCR: processor mode bit Position */ 01461 #define FPU_FPCCR_THREAD_Msk (1UL << FPU_FPCCR_THREAD_Pos) /*!< FPCCR: processor mode active bit Mask */ 01462 01463 #define FPU_FPCCR_USER_Pos 1 /*!< FPCCR: privilege level bit Position */ 01464 #define FPU_FPCCR_USER_Msk (1UL << FPU_FPCCR_USER_Pos) /*!< FPCCR: privilege level bit Mask */ 01465 01466 #define FPU_FPCCR_LSPACT_Pos 0 /*!< FPCCR: Lazy state preservation active bit Position */ 01467 #define FPU_FPCCR_LSPACT_Msk (1UL /*<< FPU_FPCCR_LSPACT_Pos*/) /*!< FPCCR: Lazy state preservation active bit Mask */ 01468 01469 /* Floating-Point Context Address Register */ 01470 #define FPU_FPCAR_ADDRESS_Pos 3 /*!< FPCAR: ADDRESS bit Position */ 01471 #define FPU_FPCAR_ADDRESS_Msk (0x1FFFFFFFUL << FPU_FPCAR_ADDRESS_Pos) /*!< FPCAR: ADDRESS bit Mask */ 01472 01473 /* Floating-Point Default Status Control Register */ 01474 #define FPU_FPDSCR_AHP_Pos 26 /*!< FPDSCR: AHP bit Position */ 01475 #define FPU_FPDSCR_AHP_Msk (1UL << FPU_FPDSCR_AHP_Pos) /*!< FPDSCR: AHP bit Mask */ 01476 01477 #define FPU_FPDSCR_DN_Pos 25 /*!< FPDSCR: DN bit Position */ 01478 #define FPU_FPDSCR_DN_Msk (1UL << FPU_FPDSCR_DN_Pos) /*!< FPDSCR: DN bit Mask */ 01479 01480 #define FPU_FPDSCR_FZ_Pos 24 /*!< FPDSCR: FZ bit Position */ 01481 #define FPU_FPDSCR_FZ_Msk (1UL << FPU_FPDSCR_FZ_Pos) /*!< FPDSCR: FZ bit Mask */ 01482 01483 #define FPU_FPDSCR_RMode_Pos 22 /*!< FPDSCR: RMode bit Position */ 01484 #define FPU_FPDSCR_RMode_Msk (3UL << FPU_FPDSCR_RMode_Pos) /*!< FPDSCR: RMode bit Mask */ 01485 01486 /* Media and FP Feature Register 0 */ 01487 #define FPU_MVFR0_FP_rounding_modes_Pos 28 /*!< MVFR0: FP rounding modes bits Position */ 01488 #define FPU_MVFR0_FP_rounding_modes_Msk (0xFUL << FPU_MVFR0_FP_rounding_modes_Pos) /*!< MVFR0: FP rounding modes bits Mask */ 01489 01490 #define FPU_MVFR0_Short_vectors_Pos 24 /*!< MVFR0: Short vectors bits Position */ 01491 #define FPU_MVFR0_Short_vectors_Msk (0xFUL << FPU_MVFR0_Short_vectors_Pos) /*!< MVFR0: Short vectors bits Mask */ 01492 01493 #define FPU_MVFR0_Square_root_Pos 20 /*!< MVFR0: Square root bits Position */ 01494 #define FPU_MVFR0_Square_root_Msk (0xFUL << FPU_MVFR0_Square_root_Pos) /*!< MVFR0: Square root bits Mask */ 01495 01496 #define FPU_MVFR0_Divide_Pos 16 /*!< MVFR0: Divide bits Position */ 01497 #define FPU_MVFR0_Divide_Msk (0xFUL << FPU_MVFR0_Divide_Pos) /*!< MVFR0: Divide bits Mask */ 01498 01499 #define FPU_MVFR0_FP_excep_trapping_Pos 12 /*!< MVFR0: FP exception trapping bits Position */ 01500 #define FPU_MVFR0_FP_excep_trapping_Msk (0xFUL << FPU_MVFR0_FP_excep_trapping_Pos) /*!< MVFR0: FP exception trapping bits Mask */ 01501 01502 #define FPU_MVFR0_Double_precision_Pos 8 /*!< MVFR0: Double-precision bits Position */ 01503 #define FPU_MVFR0_Double_precision_Msk (0xFUL << FPU_MVFR0_Double_precision_Pos) /*!< MVFR0: Double-precision bits Mask */ 01504 01505 #define FPU_MVFR0_Single_precision_Pos 4 /*!< MVFR0: Single-precision bits Position */ 01506 #define FPU_MVFR0_Single_precision_Msk (0xFUL << FPU_MVFR0_Single_precision_Pos) /*!< MVFR0: Single-precision bits Mask */ 01507 01508 #define FPU_MVFR0_A_SIMD_registers_Pos 0 /*!< MVFR0: A_SIMD registers bits Position */ 01509 #define FPU_MVFR0_A_SIMD_registers_Msk (0xFUL /*<< FPU_MVFR0_A_SIMD_registers_Pos*/) /*!< MVFR0: A_SIMD registers bits Mask */ 01510 01511 /* Media and FP Feature Register 1 */ 01512 #define FPU_MVFR1_FP_fused_MAC_Pos 28 /*!< MVFR1: FP fused MAC bits Position */ 01513 #define FPU_MVFR1_FP_fused_MAC_Msk (0xFUL << FPU_MVFR1_FP_fused_MAC_Pos) /*!< MVFR1: FP fused MAC bits Mask */ 01514 01515 #define FPU_MVFR1_FP_HPFP_Pos 24 /*!< MVFR1: FP HPFP bits Position */ 01516 #define FPU_MVFR1_FP_HPFP_Msk (0xFUL << FPU_MVFR1_FP_HPFP_Pos) /*!< MVFR1: FP HPFP bits Mask */ 01517 01518 #define FPU_MVFR1_D_NaN_mode_Pos 4 /*!< MVFR1: D_NaN mode bits Position */ 01519 #define FPU_MVFR1_D_NaN_mode_Msk (0xFUL << FPU_MVFR1_D_NaN_mode_Pos) /*!< MVFR1: D_NaN mode bits Mask */ 01520 01521 #define FPU_MVFR1_FtZ_mode_Pos 0 /*!< MVFR1: FtZ mode bits Position */ 01522 #define FPU_MVFR1_FtZ_mode_Msk (0xFUL /*<< FPU_MVFR1_FtZ_mode_Pos*/) /*!< MVFR1: FtZ mode bits Mask */ 01523 01524 /* Media and FP Feature Register 2 */ 01525 01526 /*@} end of group CMSIS_FPU */ 01527 #endif 01528 01529 01530 /** \ingroup CMSIS_core_register 01531 \defgroup CMSIS_CoreDebug Core Debug Registers (CoreDebug) 01532 \brief Type definitions for the Core Debug Registers 01533 @{ 01534 */ 01535 01536 /** \brief Structure type to access the Core Debug Register (CoreDebug). 01537 */ 01538 typedef struct 01539 { 01540 __IO uint32_t DHCSR; /*!< Offset: 0x000 (R/W) Debug Halting Control and Status Register */ 01541 __O uint32_t DCRSR; /*!< Offset: 0x004 ( /W) Debug Core Register Selector Register */ 01542 __IO uint32_t DCRDR; /*!< Offset: 0x008 (R/W) Debug Core Register Data Register */ 01543 __IO uint32_t DEMCR; /*!< Offset: 0x00C (R/W) Debug Exception and Monitor Control Register */ 01544 } CoreDebug_Type; 01545 01546 /* Debug Halting Control and Status Register */ 01547 #define CoreDebug_DHCSR_DBGKEY_Pos 16 /*!< CoreDebug DHCSR: DBGKEY Position */ 01548 #define CoreDebug_DHCSR_DBGKEY_Msk (0xFFFFUL << CoreDebug_DHCSR_DBGKEY_Pos) /*!< CoreDebug DHCSR: DBGKEY Mask */ 01549 01550 #define CoreDebug_DHCSR_S_RESET_ST_Pos 25 /*!< CoreDebug DHCSR: S_RESET_ST Position */ 01551 #define CoreDebug_DHCSR_S_RESET_ST_Msk (1UL << CoreDebug_DHCSR_S_RESET_ST_Pos) /*!< CoreDebug DHCSR: S_RESET_ST Mask */ 01552 01553 #define CoreDebug_DHCSR_S_RETIRE_ST_Pos 24 /*!< CoreDebug DHCSR: S_RETIRE_ST Position */ 01554 #define CoreDebug_DHCSR_S_RETIRE_ST_Msk (1UL << CoreDebug_DHCSR_S_RETIRE_ST_Pos) /*!< CoreDebug DHCSR: S_RETIRE_ST Mask */ 01555 01556 #define CoreDebug_DHCSR_S_LOCKUP_Pos 19 /*!< CoreDebug DHCSR: S_LOCKUP Position */ 01557 #define CoreDebug_DHCSR_S_LOCKUP_Msk (1UL << CoreDebug_DHCSR_S_LOCKUP_Pos) /*!< CoreDebug DHCSR: S_LOCKUP Mask */ 01558 01559 #define CoreDebug_DHCSR_S_SLEEP_Pos 18 /*!< CoreDebug DHCSR: S_SLEEP Position */ 01560 #define CoreDebug_DHCSR_S_SLEEP_Msk (1UL << CoreDebug_DHCSR_S_SLEEP_Pos) /*!< CoreDebug DHCSR: S_SLEEP Mask */ 01561 01562 #define CoreDebug_DHCSR_S_HALT_Pos 17 /*!< CoreDebug DHCSR: S_HALT Position */ 01563 #define CoreDebug_DHCSR_S_HALT_Msk (1UL << CoreDebug_DHCSR_S_HALT_Pos) /*!< CoreDebug DHCSR: S_HALT Mask */ 01564 01565 #define CoreDebug_DHCSR_S_REGRDY_Pos 16 /*!< CoreDebug DHCSR: S_REGRDY Position */ 01566 #define CoreDebug_DHCSR_S_REGRDY_Msk (1UL << CoreDebug_DHCSR_S_REGRDY_Pos) /*!< CoreDebug DHCSR: S_REGRDY Mask */ 01567 01568 #define CoreDebug_DHCSR_C_SNAPSTALL_Pos 5 /*!< CoreDebug DHCSR: C_SNAPSTALL Position */ 01569 #define CoreDebug_DHCSR_C_SNAPSTALL_Msk (1UL << CoreDebug_DHCSR_C_SNAPSTALL_Pos) /*!< CoreDebug DHCSR: C_SNAPSTALL Mask */ 01570 01571 #define CoreDebug_DHCSR_C_MASKINTS_Pos 3 /*!< CoreDebug DHCSR: C_MASKINTS Position */ 01572 #define CoreDebug_DHCSR_C_MASKINTS_Msk (1UL << CoreDebug_DHCSR_C_MASKINTS_Pos) /*!< CoreDebug DHCSR: C_MASKINTS Mask */ 01573 01574 #define CoreDebug_DHCSR_C_STEP_Pos 2 /*!< CoreDebug DHCSR: C_STEP Position */ 01575 #define CoreDebug_DHCSR_C_STEP_Msk (1UL << CoreDebug_DHCSR_C_STEP_Pos) /*!< CoreDebug DHCSR: C_STEP Mask */ 01576 01577 #define CoreDebug_DHCSR_C_HALT_Pos 1 /*!< CoreDebug DHCSR: C_HALT Position */ 01578 #define CoreDebug_DHCSR_C_HALT_Msk (1UL << CoreDebug_DHCSR_C_HALT_Pos) /*!< CoreDebug DHCSR: C_HALT Mask */ 01579 01580 #define CoreDebug_DHCSR_C_DEBUGEN_Pos 0 /*!< CoreDebug DHCSR: C_DEBUGEN Position */ 01581 #define CoreDebug_DHCSR_C_DEBUGEN_Msk (1UL /*<< CoreDebug_DHCSR_C_DEBUGEN_Pos*/) /*!< CoreDebug DHCSR: C_DEBUGEN Mask */ 01582 01583 /* Debug Core Register Selector Register */ 01584 #define CoreDebug_DCRSR_REGWnR_Pos 16 /*!< CoreDebug DCRSR: REGWnR Position */ 01585 #define CoreDebug_DCRSR_REGWnR_Msk (1UL << CoreDebug_DCRSR_REGWnR_Pos) /*!< CoreDebug DCRSR: REGWnR Mask */ 01586 01587 #define CoreDebug_DCRSR_REGSEL_Pos 0 /*!< CoreDebug DCRSR: REGSEL Position */ 01588 #define CoreDebug_DCRSR_REGSEL_Msk (0x1FUL /*<< CoreDebug_DCRSR_REGSEL_Pos*/) /*!< CoreDebug DCRSR: REGSEL Mask */ 01589 01590 /* Debug Exception and Monitor Control Register */ 01591 #define CoreDebug_DEMCR_TRCENA_Pos 24 /*!< CoreDebug DEMCR: TRCENA Position */ 01592 #define CoreDebug_DEMCR_TRCENA_Msk (1UL << CoreDebug_DEMCR_TRCENA_Pos) /*!< CoreDebug DEMCR: TRCENA Mask */ 01593 01594 #define CoreDebug_DEMCR_MON_REQ_Pos 19 /*!< CoreDebug DEMCR: MON_REQ Position */ 01595 #define CoreDebug_DEMCR_MON_REQ_Msk (1UL << CoreDebug_DEMCR_MON_REQ_Pos) /*!< CoreDebug DEMCR: MON_REQ Mask */ 01596 01597 #define CoreDebug_DEMCR_MON_STEP_Pos 18 /*!< CoreDebug DEMCR: MON_STEP Position */ 01598 #define CoreDebug_DEMCR_MON_STEP_Msk (1UL << CoreDebug_DEMCR_MON_STEP_Pos) /*!< CoreDebug DEMCR: MON_STEP Mask */ 01599 01600 #define CoreDebug_DEMCR_MON_PEND_Pos 17 /*!< CoreDebug DEMCR: MON_PEND Position */ 01601 #define CoreDebug_DEMCR_MON_PEND_Msk (1UL << CoreDebug_DEMCR_MON_PEND_Pos) /*!< CoreDebug DEMCR: MON_PEND Mask */ 01602 01603 #define CoreDebug_DEMCR_MON_EN_Pos 16 /*!< CoreDebug DEMCR: MON_EN Position */ 01604 #define CoreDebug_DEMCR_MON_EN_Msk (1UL << CoreDebug_DEMCR_MON_EN_Pos) /*!< CoreDebug DEMCR: MON_EN Mask */ 01605 01606 #define CoreDebug_DEMCR_VC_HARDERR_Pos 10 /*!< CoreDebug DEMCR: VC_HARDERR Position */ 01607 #define CoreDebug_DEMCR_VC_HARDERR_Msk (1UL << CoreDebug_DEMCR_VC_HARDERR_Pos) /*!< CoreDebug DEMCR: VC_HARDERR Mask */ 01608 01609 #define CoreDebug_DEMCR_VC_INTERR_Pos 9 /*!< CoreDebug DEMCR: VC_INTERR Position */ 01610 #define CoreDebug_DEMCR_VC_INTERR_Msk (1UL << CoreDebug_DEMCR_VC_INTERR_Pos) /*!< CoreDebug DEMCR: VC_INTERR Mask */ 01611 01612 #define CoreDebug_DEMCR_VC_BUSERR_Pos 8 /*!< CoreDebug DEMCR: VC_BUSERR Position */ 01613 #define CoreDebug_DEMCR_VC_BUSERR_Msk (1UL << CoreDebug_DEMCR_VC_BUSERR_Pos) /*!< CoreDebug DEMCR: VC_BUSERR Mask */ 01614 01615 #define CoreDebug_DEMCR_VC_STATERR_Pos 7 /*!< CoreDebug DEMCR: VC_STATERR Position */ 01616 #define CoreDebug_DEMCR_VC_STATERR_Msk (1UL << CoreDebug_DEMCR_VC_STATERR_Pos) /*!< CoreDebug DEMCR: VC_STATERR Mask */ 01617 01618 #define CoreDebug_DEMCR_VC_CHKERR_Pos 6 /*!< CoreDebug DEMCR: VC_CHKERR Position */ 01619 #define CoreDebug_DEMCR_VC_CHKERR_Msk (1UL << CoreDebug_DEMCR_VC_CHKERR_Pos) /*!< CoreDebug DEMCR: VC_CHKERR Mask */ 01620 01621 #define CoreDebug_DEMCR_VC_NOCPERR_Pos 5 /*!< CoreDebug DEMCR: VC_NOCPERR Position */ 01622 #define CoreDebug_DEMCR_VC_NOCPERR_Msk (1UL << CoreDebug_DEMCR_VC_NOCPERR_Pos) /*!< CoreDebug DEMCR: VC_NOCPERR Mask */ 01623 01624 #define CoreDebug_DEMCR_VC_MMERR_Pos 4 /*!< CoreDebug DEMCR: VC_MMERR Position */ 01625 #define CoreDebug_DEMCR_VC_MMERR_Msk (1UL << CoreDebug_DEMCR_VC_MMERR_Pos) /*!< CoreDebug DEMCR: VC_MMERR Mask */ 01626 01627 #define CoreDebug_DEMCR_VC_CORERESET_Pos 0 /*!< CoreDebug DEMCR: VC_CORERESET Position */ 01628 #define CoreDebug_DEMCR_VC_CORERESET_Msk (1UL /*<< CoreDebug_DEMCR_VC_CORERESET_Pos*/) /*!< CoreDebug DEMCR: VC_CORERESET Mask */ 01629 01630 /*@} end of group CMSIS_CoreDebug */ 01631 01632 01633 /** \ingroup CMSIS_core_register 01634 \defgroup CMSIS_core_base Core Definitions 01635 \brief Definitions for base addresses, unions, and structures. 01636 @{ 01637 */ 01638 01639 /* Memory mapping of Cortex-M4 Hardware */ 01640 #define SCS_BASE (0xE000E000UL) /*!< System Control Space Base Address */ 01641 #define ITM_BASE (0xE0000000UL) /*!< ITM Base Address */ 01642 #define DWT_BASE (0xE0001000UL) /*!< DWT Base Address */ 01643 #define TPI_BASE (0xE0040000UL) /*!< TPI Base Address */ 01644 #define CoreDebug_BASE (0xE000EDF0UL) /*!< Core Debug Base Address */ 01645 #define SysTick_BASE (SCS_BASE + 0x0010UL) /*!< SysTick Base Address */ 01646 #define NVIC_BASE (SCS_BASE + 0x0100UL) /*!< NVIC Base Address */ 01647 #define SCB_BASE (SCS_BASE + 0x0D00UL) /*!< System Control Block Base Address */ 01648 01649 #define SCnSCB ((SCnSCB_Type *) SCS_BASE ) /*!< System control Register not in SCB */ 01650 #define SCB ((SCB_Type *) SCB_BASE ) /*!< SCB configuration struct */ 01651 #define SysTick ((SysTick_Type *) SysTick_BASE ) /*!< SysTick configuration struct */ 01652 #define NVIC ((NVIC_Type *) NVIC_BASE ) /*!< NVIC configuration struct */ 01653 #define ITM ((ITM_Type *) ITM_BASE ) /*!< ITM configuration struct */ 01654 #define DWT ((DWT_Type *) DWT_BASE ) /*!< DWT configuration struct */ 01655 #define TPI ((TPI_Type *) TPI_BASE ) /*!< TPI configuration struct */ 01656 #define CoreDebug ((CoreDebug_Type *) CoreDebug_BASE) /*!< Core Debug configuration struct */ 01657 01658 #if (__MPU_PRESENT == 1) 01659 #define MPU_BASE (SCS_BASE + 0x0D90UL) /*!< Memory Protection Unit */ 01660 #define MPU ((MPU_Type *) MPU_BASE ) /*!< Memory Protection Unit */ 01661 #endif 01662 01663 #if (__FPU_PRESENT == 1) 01664 #define FPU_BASE (SCS_BASE + 0x0F30UL) /*!< Floating Point Unit */ 01665 #define FPU ((FPU_Type *) FPU_BASE ) /*!< Floating Point Unit */ 01666 #endif 01667 01668 /*@} */ 01669 01670 01671 01672 /******************************************************************************* 01673 * Hardware Abstraction Layer 01674 Core Function Interface contains: 01675 - Core NVIC Functions 01676 - Core SysTick Functions 01677 - Core Debug Functions 01678 - Core Register Access Functions 01679 ******************************************************************************/ 01680 /** \defgroup CMSIS_Core_FunctionInterface Functions and Instructions Reference 01681 */ 01682 01683 01684 01685 /* ########################## NVIC functions #################################### */ 01686 /** \ingroup CMSIS_Core_FunctionInterface 01687 \defgroup CMSIS_Core_NVICFunctions NVIC Functions 01688 \brief Functions that manage interrupts and exceptions via the NVIC. 01689 @{ 01690 */ 01691 01692 /** \brief Set Priority Grouping 01693 01694 The function sets the priority grouping field using the required unlock sequence. 01695 The parameter PriorityGroup is assigned to the field SCB->AIRCR [10:8] PRIGROUP field. 01696 Only values from 0..7 are used. 01697 In case of a conflict between priority grouping and available 01698 priority bits (__NVIC_PRIO_BITS), the smallest possible priority group is set. 01699 01700 \param [in] PriorityGroup Priority grouping field. 01701 */ 01702 __STATIC_INLINE void NVIC_SetPriorityGrouping(uint32_t PriorityGroup) 01703 { 01704 uint32_t reg_value; 01705 uint32_t PriorityGroupTmp = (PriorityGroup & (uint32_t)0x07UL); /* only values 0..7 are used */ 01706 01707 reg_value = SCB->AIRCR; /* read old register configuration */ 01708 reg_value &= ~((uint32_t)(SCB_AIRCR_VECTKEY_Msk | SCB_AIRCR_PRIGROUP_Msk)); /* clear bits to change */ 01709 reg_value = (reg_value | 01710 ((uint32_t)0x5FAUL << SCB_AIRCR_VECTKEY_Pos) | 01711 (PriorityGroupTmp << 8) ); /* Insert write key and priorty group */ 01712 SCB->AIRCR = reg_value; 01713 } 01714 01715 01716 /** \brief Get Priority Grouping 01717 01718 The function reads the priority grouping field from the NVIC Interrupt Controller. 01719 01720 \return Priority grouping field (SCB->AIRCR [10:8] PRIGROUP field). 01721 */ 01722 __STATIC_INLINE uint32_t NVIC_GetPriorityGrouping(void) 01723 { 01724 return ((uint32_t)((SCB->AIRCR & SCB_AIRCR_PRIGROUP_Msk) >> SCB_AIRCR_PRIGROUP_Pos)); 01725 } 01726 01727 01728 /** \brief Enable External Interrupt 01729 01730 The function enables a device-specific interrupt in the NVIC interrupt controller. 01731 01732 \param [in] IRQn External interrupt number. Value cannot be negative. 01733 */ 01734 __STATIC_INLINE void NVIC_EnableIRQ(IRQn_Type IRQn) 01735 { 01736 NVIC->ISER[(((uint32_t)(int32_t)IRQn) >> 5UL)] = (uint32_t)(1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL)); 01737 } 01738 01739 01740 /** \brief Disable External Interrupt 01741 01742 The function disables a device-specific interrupt in the NVIC interrupt controller. 01743 01744 \param [in] IRQn External interrupt number. Value cannot be negative. 01745 */ 01746 __STATIC_INLINE void NVIC_DisableIRQ(IRQn_Type IRQn) 01747 { 01748 NVIC->ICER[(((uint32_t)(int32_t)IRQn) >> 5UL)] = (uint32_t)(1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL)); 01749 } 01750 01751 01752 /** \brief Get Pending Interrupt 01753 01754 The function reads the pending register in the NVIC and returns the pending bit 01755 for the specified interrupt. 01756 01757 \param [in] IRQn Interrupt number. 01758 01759 \return 0 Interrupt status is not pending. 01760 \return 1 Interrupt status is pending. 01761 */ 01762 __STATIC_INLINE uint32_t NVIC_GetPendingIRQ(IRQn_Type IRQn) 01763 { 01764 return((uint32_t)(((NVIC->ISPR[(((uint32_t)(int32_t)IRQn) >> 5UL)] & (1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL))) != 0UL) ? 1UL : 0UL)); 01765 } 01766 01767 01768 /** \brief Set Pending Interrupt 01769 01770 The function sets the pending bit of an external interrupt. 01771 01772 \param [in] IRQn Interrupt number. Value cannot be negative. 01773 */ 01774 __STATIC_INLINE void NVIC_SetPendingIRQ(IRQn_Type IRQn) 01775 { 01776 NVIC->ISPR[(((uint32_t)(int32_t)IRQn) >> 5UL)] = (uint32_t)(1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL)); 01777 } 01778 01779 01780 /** \brief Clear Pending Interrupt 01781 01782 The function clears the pending bit of an external interrupt. 01783 01784 \param [in] IRQn External interrupt number. Value cannot be negative. 01785 */ 01786 __STATIC_INLINE void NVIC_ClearPendingIRQ(IRQn_Type IRQn) 01787 { 01788 NVIC->ICPR[(((uint32_t)(int32_t)IRQn) >> 5UL)] = (uint32_t)(1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL)); 01789 } 01790 01791 01792 /** \brief Get Active Interrupt 01793 01794 The function reads the active register in NVIC and returns the active bit. 01795 01796 \param [in] IRQn Interrupt number. 01797 01798 \return 0 Interrupt status is not active. 01799 \return 1 Interrupt status is active. 01800 */ 01801 __STATIC_INLINE uint32_t NVIC_GetActive(IRQn_Type IRQn) 01802 { 01803 return((uint32_t)(((NVIC->IABR[(((uint32_t)(int32_t)IRQn) >> 5UL)] & (1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL))) != 0UL) ? 1UL : 0UL)); 01804 } 01805 01806 01807 /** \brief Set Interrupt Priority 01808 01809 The function sets the priority of an interrupt. 01810 01811 \note The priority cannot be set for every core interrupt. 01812 01813 \param [in] IRQn Interrupt number. 01814 \param [in] priority Priority to set. 01815 */ 01816 __STATIC_INLINE void NVIC_SetPriority(IRQn_Type IRQn, uint32_t priority) 01817 { 01818 if((int32_t)IRQn < 0) { 01819 SCB->SHPR[(((uint32_t)(int32_t)IRQn) & 0xFUL)-4UL] = (uint8_t)((priority << (8 - __NVIC_PRIO_BITS)) & (uint32_t)0xFFUL); 01820 } 01821 else { 01822 NVIC->IP[((uint32_t)(int32_t)IRQn)] = (uint8_t)((priority << (8 - __NVIC_PRIO_BITS)) & (uint32_t)0xFFUL); 01823 } 01824 } 01825 01826 01827 /** \brief Get Interrupt Priority 01828 01829 The function reads the priority of an interrupt. The interrupt 01830 number can be positive to specify an external (device specific) 01831 interrupt, or negative to specify an internal (core) interrupt. 01832 01833 01834 \param [in] IRQn Interrupt number. 01835 \return Interrupt Priority. Value is aligned automatically to the implemented 01836 priority bits of the microcontroller. 01837 */ 01838 __STATIC_INLINE uint32_t NVIC_GetPriority(IRQn_Type IRQn) 01839 { 01840 01841 if((int32_t)IRQn < 0) { 01842 return(((uint32_t)SCB->SHPR[(((uint32_t)(int32_t)IRQn) & 0xFUL)-4UL] >> (8 - __NVIC_PRIO_BITS))); 01843 } 01844 else { 01845 return(((uint32_t)NVIC->IP[((uint32_t)(int32_t)IRQn)] >> (8 - __NVIC_PRIO_BITS))); 01846 } 01847 } 01848 01849 01850 /** \brief Encode Priority 01851 01852 The function encodes the priority for an interrupt with the given priority group, 01853 preemptive priority value, and subpriority value. 01854 In case of a conflict between priority grouping and available 01855 priority bits (__NVIC_PRIO_BITS), the smallest possible priority group is set. 01856 01857 \param [in] PriorityGroup Used priority group. 01858 \param [in] PreemptPriority Preemptive priority value (starting from 0). 01859 \param [in] SubPriority Subpriority value (starting from 0). 01860 \return Encoded priority. Value can be used in the function \ref NVIC_SetPriority(). 01861 */ 01862 __STATIC_INLINE uint32_t NVIC_EncodePriority (uint32_t PriorityGroup, uint32_t PreemptPriority, uint32_t SubPriority) 01863 { 01864 uint32_t PriorityGroupTmp = (PriorityGroup & (uint32_t)0x07UL); /* only values 0..7 are used */ 01865 uint32_t PreemptPriorityBits; 01866 uint32_t SubPriorityBits; 01867 01868 PreemptPriorityBits = ((7UL - PriorityGroupTmp) > (uint32_t)(__NVIC_PRIO_BITS)) ? (uint32_t)(__NVIC_PRIO_BITS) : (uint32_t)(7UL - PriorityGroupTmp); 01869 SubPriorityBits = ((PriorityGroupTmp + (uint32_t)(__NVIC_PRIO_BITS)) < (uint32_t)7UL) ? (uint32_t)0UL : (uint32_t)((PriorityGroupTmp - 7UL) + (uint32_t)(__NVIC_PRIO_BITS)); 01870 01871 return ( 01872 ((PreemptPriority & (uint32_t)((1UL << (PreemptPriorityBits)) - 1UL)) << SubPriorityBits) | 01873 ((SubPriority & (uint32_t)((1UL << (SubPriorityBits )) - 1UL))) 01874 ); 01875 } 01876 01877 01878 /** \brief Decode Priority 01879 01880 The function decodes an interrupt priority value with a given priority group to 01881 preemptive priority value and subpriority value. 01882 In case of a conflict between priority grouping and available 01883 priority bits (__NVIC_PRIO_BITS) the smallest possible priority group is set. 01884 01885 \param [in] Priority Priority value, which can be retrieved with the function \ref NVIC_GetPriority(). 01886 \param [in] PriorityGroup Used priority group. 01887 \param [out] pPreemptPriority Preemptive priority value (starting from 0). 01888 \param [out] pSubPriority Subpriority value (starting from 0). 01889 */ 01890 __STATIC_INLINE void NVIC_DecodePriority (uint32_t Priority, uint32_t PriorityGroup, uint32_t* pPreemptPriority, uint32_t* pSubPriority) 01891 { 01892 uint32_t PriorityGroupTmp = (PriorityGroup & (uint32_t)0x07UL); /* only values 0..7 are used */ 01893 uint32_t PreemptPriorityBits; 01894 uint32_t SubPriorityBits; 01895 01896 PreemptPriorityBits = ((7UL - PriorityGroupTmp) > (uint32_t)(__NVIC_PRIO_BITS)) ? (uint32_t)(__NVIC_PRIO_BITS) : (uint32_t)(7UL - PriorityGroupTmp); 01897 SubPriorityBits = ((PriorityGroupTmp + (uint32_t)(__NVIC_PRIO_BITS)) < (uint32_t)7UL) ? (uint32_t)0UL : (uint32_t)((PriorityGroupTmp - 7UL) + (uint32_t)(__NVIC_PRIO_BITS)); 01898 01899 *pPreemptPriority = (Priority >> SubPriorityBits) & (uint32_t)((1UL << (PreemptPriorityBits)) - 1UL); 01900 *pSubPriority = (Priority ) & (uint32_t)((1UL << (SubPriorityBits )) - 1UL); 01901 } 01902 01903 01904 /** \brief System Reset 01905 01906 The function initiates a system reset request to reset the MCU. 01907 */ 01908 __STATIC_INLINE void NVIC_SystemReset(void) 01909 { 01910 __DSB(); /* Ensure all outstanding memory accesses included 01911 buffered write are completed before reset */ 01912 SCB->AIRCR = (uint32_t)((0x5FAUL << SCB_AIRCR_VECTKEY_Pos) | 01913 (SCB->AIRCR & SCB_AIRCR_PRIGROUP_Msk) | 01914 SCB_AIRCR_SYSRESETREQ_Msk ); /* Keep priority group unchanged */ 01915 __DSB(); /* Ensure completion of memory access */ 01916 while(1) { __NOP(); } /* wait until reset */ 01917 } 01918 01919 /*@} end of CMSIS_Core_NVICFunctions */ 01920 01921 01922 /* ########################## FPU functions #################################### */ 01923 /** \ingroup CMSIS_Core_FunctionInterface 01924 \defgroup CMSIS_Core_FpuFunctions FPU Functions 01925 \brief Function that provides FPU type. 01926 @{ 01927 */ 01928 01929 /** 01930 \fn uint32_t SCB_GetFPUType(void) 01931 \brief get FPU type 01932 \returns 01933 - \b 0: No FPU 01934 - \b 1: Single precision FPU 01935 - \b 2: Double + Single precision FPU 01936 */ 01937 __STATIC_INLINE uint32_t SCB_GetFPUType(void) 01938 { 01939 uint32_t mvfr0; 01940 01941 mvfr0 = SCB->MVFR0; 01942 if ((mvfr0 & 0x00000FF0UL) == 0x220UL) { 01943 return 2UL; // Double + Single precision FPU 01944 } else if ((mvfr0 & 0x00000FF0UL) == 0x020UL) { 01945 return 1UL; // Single precision FPU 01946 } else { 01947 return 0UL; // No FPU 01948 } 01949 } 01950 01951 01952 /*@} end of CMSIS_Core_FpuFunctions */ 01953 01954 01955 01956 /* ########################## Cache functions #################################### */ 01957 /** \ingroup CMSIS_Core_FunctionInterface 01958 \defgroup CMSIS_Core_CacheFunctions Cache Functions 01959 \brief Functions that configure Instruction and Data cache. 01960 @{ 01961 */ 01962 01963 /* Cache Size ID Register Macros */ 01964 #define CCSIDR_WAYS(x) (((x) & SCB_CCSIDR_ASSOCIATIVITY_Msk) >> SCB_CCSIDR_ASSOCIATIVITY_Pos) 01965 #define CCSIDR_SETS(x) (((x) & SCB_CCSIDR_NUMSETS_Msk ) >> SCB_CCSIDR_NUMSETS_Pos ) 01966 #define CCSIDR_LSSHIFT(x) (((x) & SCB_CCSIDR_LINESIZE_Msk ) /*>> SCB_CCSIDR_LINESIZE_Pos*/ ) 01967 01968 01969 /** \brief Enable I-Cache 01970 01971 The function turns on I-Cache 01972 */ 01973 __STATIC_INLINE void SCB_EnableICache (void) 01974 { 01975 #if (__ICACHE_PRESENT == 1) 01976 __DSB(); 01977 __ISB(); 01978 SCB->ICIALLU = 0UL; // invalidate I-Cache 01979 SCB->CCR |= (uint32_t)SCB_CCR_IC_Msk; // enable I-Cache 01980 __DSB(); 01981 __ISB(); 01982 #endif 01983 } 01984 01985 01986 /** \brief Disable I-Cache 01987 01988 The function turns off I-Cache 01989 */ 01990 __STATIC_INLINE void SCB_DisableICache (void) 01991 { 01992 #if (__ICACHE_PRESENT == 1) 01993 __DSB(); 01994 __ISB(); 01995 SCB->CCR &= ~(uint32_t)SCB_CCR_IC_Msk; // disable I-Cache 01996 SCB->ICIALLU = 0UL; // invalidate I-Cache 01997 __DSB(); 01998 __ISB(); 01999 #endif 02000 } 02001 02002 02003 /** \brief Invalidate I-Cache 02004 02005 The function invalidates I-Cache 02006 */ 02007 __STATIC_INLINE void SCB_InvalidateICache (void) 02008 { 02009 #if (__ICACHE_PRESENT == 1) 02010 __DSB(); 02011 __ISB(); 02012 SCB->ICIALLU = 0UL; 02013 __DSB(); 02014 __ISB(); 02015 #endif 02016 } 02017 02018 02019 /** \brief Enable D-Cache 02020 02021 The function turns on D-Cache 02022 */ 02023 __STATIC_INLINE void SCB_EnableDCache (void) 02024 { 02025 #if (__DCACHE_PRESENT == 1) 02026 uint32_t ccsidr, sshift, wshift, sw; 02027 uint32_t sets, ways; 02028 02029 SCB->CSSELR = (0UL << 1) | 0UL; // Level 1 data cache 02030 ccsidr = SCB->CCSIDR; 02031 sets = (uint32_t)(CCSIDR_SETS(ccsidr)); 02032 sshift = (uint32_t)(CCSIDR_LSSHIFT(ccsidr) + 4UL); 02033 ways = (uint32_t)(CCSIDR_WAYS(ccsidr)); 02034 wshift = (uint32_t)((uint32_t)__CLZ(ways) & 0x1FUL); 02035 02036 __DSB(); 02037 02038 do { // invalidate D-Cache 02039 uint32_t tmpways = ways; 02040 do { 02041 sw = ((tmpways << wshift) | (sets << sshift)); 02042 SCB->DCISW = sw; 02043 } while(tmpways--); 02044 } while(sets--); 02045 __DSB(); 02046 02047 SCB->CCR |= (uint32_t)SCB_CCR_DC_Msk; // enable D-Cache 02048 02049 __DSB(); 02050 __ISB(); 02051 #endif 02052 } 02053 02054 02055 /** \brief Disable D-Cache 02056 02057 The function turns off D-Cache 02058 */ 02059 __STATIC_INLINE void SCB_DisableDCache (void) 02060 { 02061 #if (__DCACHE_PRESENT == 1) 02062 uint32_t ccsidr, sshift, wshift, sw; 02063 uint32_t sets, ways; 02064 02065 SCB->CSSELR = (0UL << 1) | 0UL; // Level 1 data cache 02066 ccsidr = SCB->CCSIDR; 02067 sets = (uint32_t)(CCSIDR_SETS(ccsidr)); 02068 sshift = (uint32_t)(CCSIDR_LSSHIFT(ccsidr) + 4UL); 02069 ways = (uint32_t)(CCSIDR_WAYS(ccsidr)); 02070 wshift = (uint32_t)((uint32_t)__CLZ(ways) & 0x1FUL); 02071 02072 __DSB(); 02073 02074 SCB->CCR &= ~(uint32_t)SCB_CCR_DC_Msk; // disable D-Cache 02075 02076 do { // clean & invalidate D-Cache 02077 uint32_t tmpways = ways; 02078 do { 02079 sw = ((tmpways << wshift) | (sets << sshift)); 02080 SCB->DCCISW = sw; 02081 } while(tmpways--); 02082 } while(sets--); 02083 02084 02085 __DSB(); 02086 __ISB(); 02087 #endif 02088 } 02089 02090 02091 /** \brief Invalidate D-Cache 02092 02093 The function invalidates D-Cache 02094 */ 02095 __STATIC_INLINE void SCB_InvalidateDCache (void) 02096 { 02097 #if (__DCACHE_PRESENT == 1) 02098 uint32_t ccsidr, sshift, wshift, sw; 02099 uint32_t sets, ways; 02100 02101 SCB->CSSELR = (0UL << 1) | 0UL; // Level 1 data cache 02102 ccsidr = SCB->CCSIDR; 02103 sets = (uint32_t)(CCSIDR_SETS(ccsidr)); 02104 sshift = (uint32_t)(CCSIDR_LSSHIFT(ccsidr) + 4UL); 02105 ways = (uint32_t)(CCSIDR_WAYS(ccsidr)); 02106 wshift = (uint32_t)((uint32_t)__CLZ(ways) & 0x1FUL); 02107 02108 __DSB(); 02109 02110 do { // invalidate D-Cache 02111 uint32_t tmpways = ways; 02112 do { 02113 sw = ((tmpways << wshift) | (sets << sshift)); 02114 SCB->DCISW = sw; 02115 } while(tmpways--); 02116 } while(sets--); 02117 02118 __DSB(); 02119 __ISB(); 02120 #endif 02121 } 02122 02123 02124 /** \brief Clean D-Cache 02125 02126 The function cleans D-Cache 02127 */ 02128 __STATIC_INLINE void SCB_CleanDCache (void) 02129 { 02130 #if (__DCACHE_PRESENT == 1) 02131 uint32_t ccsidr, sshift, wshift, sw; 02132 uint32_t sets, ways; 02133 02134 SCB->CSSELR = (0UL << 1) | 0UL; // Level 1 data cache 02135 ccsidr = SCB->CCSIDR; 02136 sets = (uint32_t)(CCSIDR_SETS(ccsidr)); 02137 sshift = (uint32_t)(CCSIDR_LSSHIFT(ccsidr) + 4UL); 02138 ways = (uint32_t)(CCSIDR_WAYS(ccsidr)); 02139 wshift = (uint32_t)((uint32_t)__CLZ(ways) & 0x1FUL); 02140 02141 __DSB(); 02142 02143 do { // clean D-Cache 02144 uint32_t tmpways = ways; 02145 do { 02146 sw = ((tmpways << wshift) | (sets << sshift)); 02147 SCB->DCCSW = sw; 02148 } while(tmpways--); 02149 } while(sets--); 02150 02151 __DSB(); 02152 __ISB(); 02153 #endif 02154 } 02155 02156 02157 /** \brief Clean & Invalidate D-Cache 02158 02159 The function cleans and Invalidates D-Cache 02160 */ 02161 __STATIC_INLINE void SCB_CleanInvalidateDCache (void) 02162 { 02163 #if (__DCACHE_PRESENT == 1) 02164 uint32_t ccsidr, sshift, wshift, sw; 02165 uint32_t sets, ways; 02166 02167 SCB->CSSELR = (0UL << 1) | 0UL; // Level 1 data cache 02168 ccsidr = SCB->CCSIDR; 02169 sets = (uint32_t)(CCSIDR_SETS(ccsidr)); 02170 sshift = (uint32_t)(CCSIDR_LSSHIFT(ccsidr) + 4UL); 02171 ways = (uint32_t)(CCSIDR_WAYS(ccsidr)); 02172 wshift = (uint32_t)((uint32_t)__CLZ(ways) & 0x1FUL); 02173 02174 __DSB(); 02175 02176 do { // clean & invalidate D-Cache 02177 uint32_t tmpways = ways; 02178 do { 02179 sw = ((tmpways << wshift) | (sets << sshift)); 02180 SCB->DCCISW = sw; 02181 } while(tmpways--); 02182 } while(sets--); 02183 02184 __DSB(); 02185 __ISB(); 02186 #endif 02187 } 02188 02189 02190 /** 02191 \fn void SCB_InvalidateDCache_by_Addr(volatile uint32_t *addr, int32_t dsize) 02192 \brief D-Cache Invalidate by address 02193 \param[in] addr address (aligned to 32-byte boundary) 02194 \param[in] dsize size of memory block (in number of bytes) 02195 */ 02196 __STATIC_INLINE void SCB_InvalidateDCache_by_Addr (uint32_t *addr, int32_t dsize) 02197 { 02198 #if (__DCACHE_PRESENT == 1) 02199 int32_t op_size = dsize; 02200 uint32_t op_addr = (uint32_t)addr; 02201 uint32_t linesize = 32UL; // in Cortex-M7 size of cache line is fixed to 8 words (32 bytes) 02202 02203 __DSB(); 02204 02205 while (op_size > 0) { 02206 SCB->DCIMVAC = op_addr; 02207 op_addr += linesize; 02208 op_size -= (int32_t)linesize; 02209 } 02210 02211 __DSB(); 02212 __ISB(); 02213 #endif 02214 } 02215 02216 02217 /** 02218 \fn void SCB_CleanDCache_by_Addr(volatile uint32_t *addr, int32_t dsize) 02219 \brief D-Cache Clean by address 02220 \param[in] addr address (aligned to 32-byte boundary) 02221 \param[in] dsize size of memory block (in number of bytes) 02222 */ 02223 __STATIC_INLINE void SCB_CleanDCache_by_Addr (uint32_t *addr, int32_t dsize) 02224 { 02225 #if (__DCACHE_PRESENT == 1) 02226 int32_t op_size = dsize; 02227 uint32_t op_addr = (uint32_t) addr; 02228 uint32_t linesize = 32UL; // in Cortex-M7 size of cache line is fixed to 8 words (32 bytes) 02229 02230 __DSB(); 02231 02232 while (op_size > 0) { 02233 SCB->DCCMVAC = op_addr; 02234 op_addr += linesize; 02235 op_size -= (int32_t)linesize; 02236 } 02237 02238 __DSB(); 02239 __ISB(); 02240 #endif 02241 } 02242 02243 02244 /** 02245 \fn void SCB_CleanInvalidateDCache_by_Addr(volatile uint32_t *addr, int32_t dsize) 02246 \brief D-Cache Clean and Invalidate by address 02247 \param[in] addr address (aligned to 32-byte boundary) 02248 \param[in] dsize size of memory block (in number of bytes) 02249 */ 02250 __STATIC_INLINE void SCB_CleanInvalidateDCache_by_Addr (uint32_t *addr, int32_t dsize) 02251 { 02252 #if (__DCACHE_PRESENT == 1) 02253 int32_t op_size = dsize; 02254 uint32_t op_addr = (uint32_t) addr; 02255 uint32_t linesize = 32UL; // in Cortex-M7 size of cache line is fixed to 8 words (32 bytes) 02256 02257 __DSB(); 02258 02259 while (op_size > 0) { 02260 SCB->DCCIMVAC = op_addr; 02261 op_addr += linesize; 02262 op_size -= (int32_t)linesize; 02263 } 02264 02265 __DSB(); 02266 __ISB(); 02267 #endif 02268 } 02269 02270 02271 /*@} end of CMSIS_Core_CacheFunctions */ 02272 02273 02274 02275 /* ################################## SysTick function ############################################ */ 02276 /** \ingroup CMSIS_Core_FunctionInterface 02277 \defgroup CMSIS_Core_SysTickFunctions SysTick Functions 02278 \brief Functions that configure the System. 02279 @{ 02280 */ 02281 02282 #if (__Vendor_SysTickConfig == 0) 02283 02284 /** \brief System Tick Configuration 02285 02286 The function initializes the System Timer and its interrupt, and starts the System Tick Timer. 02287 Counter is in free running mode to generate periodic interrupts. 02288 02289 \param [in] ticks Number of ticks between two interrupts. 02290 02291 \return 0 Function succeeded. 02292 \return 1 Function failed. 02293 02294 \note When the variable <b>__Vendor_SysTickConfig</b> is set to 1, then the 02295 function <b>SysTick_Config</b> is not included. In this case, the file <b><i>device</i>.h</b> 02296 must contain a vendor-specific implementation of this function. 02297 02298 */ 02299 __STATIC_INLINE uint32_t SysTick_Config(uint32_t ticks) 02300 { 02301 if ((ticks - 1UL) > SysTick_LOAD_RELOAD_Msk) { return (1UL); } /* Reload value impossible */ 02302 02303 SysTick->LOAD = (uint32_t)(ticks - 1UL); /* set reload register */ 02304 NVIC_SetPriority (SysTick_IRQn, (1UL << __NVIC_PRIO_BITS) - 1UL); /* set Priority for Systick Interrupt */ 02305 SysTick->VAL = 0UL; /* Load the SysTick Counter Value */ 02306 SysTick->CTRL = SysTick_CTRL_CLKSOURCE_Msk | 02307 SysTick_CTRL_TICKINT_Msk | 02308 SysTick_CTRL_ENABLE_Msk; /* Enable SysTick IRQ and SysTick Timer */ 02309 return (0UL); /* Function successful */ 02310 } 02311 02312 #endif 02313 02314 /*@} end of CMSIS_Core_SysTickFunctions */ 02315 02316 02317 02318 /* ##################################### Debug In/Output function ########################################### */ 02319 /** \ingroup CMSIS_Core_FunctionInterface 02320 \defgroup CMSIS_core_DebugFunctions ITM Functions 02321 \brief Functions that access the ITM debug interface. 02322 @{ 02323 */ 02324 02325 extern volatile int32_t ITM_RxBuffer; /*!< External variable to receive characters. */ 02326 #define ITM_RXBUFFER_EMPTY 0x5AA55AA5 /*!< Value identifying \ref ITM_RxBuffer is ready for next character. */ 02327 02328 02329 /** \brief ITM Send Character 02330 02331 The function transmits a character via the ITM channel 0, and 02332 \li Just returns when no debugger is connected that has booked the output. 02333 \li Is blocking when a debugger is connected, but the previous character sent has not been transmitted. 02334 02335 \param [in] ch Character to transmit. 02336 02337 \returns Character to transmit. 02338 */ 02339 __STATIC_INLINE uint32_t ITM_SendChar (uint32_t ch) 02340 { 02341 if (((ITM->TCR & ITM_TCR_ITMENA_Msk) != 0UL) && /* ITM enabled */ 02342 ((ITM->TER & 1UL ) != 0UL) ) /* ITM Port #0 enabled */ 02343 { 02344 while (ITM->PORT[0].u32 == 0UL) { __NOP(); } 02345 ITM->PORT[0].u8 = (uint8_t)ch; 02346 } 02347 return (ch); 02348 } 02349 02350 02351 /** \brief ITM Receive Character 02352 02353 The function inputs a character via the external variable \ref ITM_RxBuffer. 02354 02355 \return Received character. 02356 \return -1 No character pending. 02357 */ 02358 __STATIC_INLINE int32_t ITM_ReceiveChar (void) { 02359 int32_t ch = -1; /* no character available */ 02360 02361 if (ITM_RxBuffer != ITM_RXBUFFER_EMPTY) { 02362 ch = ITM_RxBuffer; 02363 ITM_RxBuffer = ITM_RXBUFFER_EMPTY; /* ready for next character */ 02364 } 02365 02366 return (ch); 02367 } 02368 02369 02370 /** \brief ITM Check Character 02371 02372 The function checks whether a character is pending for reading in the variable \ref ITM_RxBuffer. 02373 02374 \return 0 No character available. 02375 \return 1 Character available. 02376 */ 02377 __STATIC_INLINE int32_t ITM_CheckChar (void) { 02378 02379 if (ITM_RxBuffer == ITM_RXBUFFER_EMPTY) { 02380 return (0); /* no character available */ 02381 } else { 02382 return (1); /* character available */ 02383 } 02384 } 02385 02386 /*@} end of CMSIS_core_DebugFunctions */ 02387 02388 02389 02390 02391 #ifdef __cplusplus 02392 } 02393 #endif 02394 02395 #endif /* __CORE_CM7_H_DEPENDANT */ 02396 02397 #endif /* __CMSIS_GENERIC */
Generated on Tue Jul 12 2022 11:20:31 by
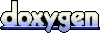