PokittoLib is the library needed for programming the Pokitto DIY game console (www.pokitto.com)
Dependents: YATTT sd_map_test cPong SnowDemo ... more
Timer.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "Timer.h" 00017 #include "ticker_api.h" 00018 #include "us_ticker_api.h" 00019 00020 namespace mbed { 00021 00022 Timer::Timer() : _running(), _start(), _time(), _ticker_data(get_us_ticker_data()) { 00023 reset(); 00024 } 00025 00026 Timer::Timer(const ticker_data_t *data) : _running(), _start(), _time(), _ticker_data(data) { 00027 reset(); 00028 } 00029 00030 void Timer::start() { 00031 if (!_running) { 00032 _start = ticker_read(_ticker_data); 00033 _running = 1; 00034 } 00035 } 00036 00037 void Timer::stop() { 00038 _time += slicetime(); 00039 _running = 0; 00040 } 00041 00042 int Timer::read_us() { 00043 return _time + slicetime(); 00044 } 00045 00046 float Timer::read() { 00047 return (float)read_us() / 1000000.0f; 00048 } 00049 00050 int Timer::read_ms() { 00051 return read_us() / 1000; 00052 } 00053 00054 int Timer::slicetime() { 00055 if (_running) { 00056 return ticker_read(_ticker_data) - _start; 00057 } else { 00058 return 0; 00059 } 00060 } 00061 00062 void Timer::reset() { 00063 _start = ticker_read(_ticker_data); 00064 _time = 0; 00065 } 00066 00067 #ifdef MBED_OPERATORS 00068 Timer::operator float() { 00069 return read(); 00070 } 00071 #endif 00072 00073 } // namespace mbed
Generated on Tue Jul 12 2022 11:20:41 by
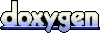