PokittoLib is the library needed for programming the Pokitto DIY game console (www.pokitto.com)
Dependents: YATTT sd_map_test cPong SnowDemo ... more
Synth_oscfuncs.cpp
00001 /**************************************************************************/ 00002 /*! 00003 @file Synth_oscfuncs.cpp 00004 @author Jonne Valola 00005 00006 @section LICENSE 00007 00008 Software License Agreement (BSD License) 00009 00010 Copyright (c) 2016, Jonne Valola 00011 All rights reserved. 00012 00013 Redistribution and use in source and binary forms, with or without 00014 modification, are permitted provided that the following conditions are met: 00015 1. Redistributions of source code must retain the above copyright 00016 notice, this list of conditions and the following disclaimer. 00017 2. Redistributions in binary form must reproduce the above copyright 00018 notice, this list of conditions and the following disclaimer in the 00019 documentation and/or other materials provided with the distribution. 00020 3. Neither the name of the copyright holders nor the 00021 names of its contributors may be used to endorse or promote products 00022 derived from this software without specific prior written permission. 00023 00024 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS ''AS IS'' AND ANY 00025 EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00026 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER BE LIABLE FOR ANY 00028 DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00029 (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00030 LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00031 ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00033 SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 */ 00035 /**************************************************************************/ 00036 00037 #include "Synth.h " 00038 00039 /** OSCILLATOR FUNCTIONS **/ 00040 00041 void setOSC(OSC* o,byte on=1, byte wave=1, byte loop=0, byte echo=0, byte adsr=0, 00042 uint8_t notenumber=25, uint16_t volume=127, 00043 uint16_t attack=0, uint16_t decay=0, uint16_t sustain=0, uint16_t release=0, 00044 int16_t maxbend=0, int16_t bendrate=0, uint8_t arpmode = 0, uint8_t overdrive=0, uint8_t kick=0){ 00045 //Serial.println("SetOsc "); osc1 00046 o->on = on; 00047 o->duration = -1; //max this out for the time being 00048 o->overdrive = overdrive; 00049 o->kick = kick; 00050 o->wave = wave; 00051 o->loop = loop; 00052 o->echo = echo; //echo shifts left 8 steps to zero 00053 o->echodiv = 0; 00054 o->adsr = adsr; 00055 if (arpmode) { 00056 if (arpmode < 4) {o->arpmode = 1; o->arpspeed = arpmode;} 00057 else if (arpmode < 7) {o->arpmode = 2; o->arpspeed = arpmode-3;} 00058 else if (arpmode < 10) {o->arpmode = 3; o->arpspeed = arpmode-6; } // vibrato trial 00059 else if (arpmode < 13) {o->arpmode = 4; o->arpspeed = arpmode-9; } // octave trial 00060 else if (arpmode < 16) {o->arpmode = 5; o->arpspeed = arpmode-12; } // funk trial*/ 00061 } else o->arpmode = 0; 00062 o->arpstep = 0; 00063 o->count = 0; 00064 noiseval = xorshift16(); //random(0,0xFFFF); 00065 00066 o->cinc = cincs[notenumber]; // direct cinc from table, no calculation 00067 o->tonic = notenumber; // save tonic for arpeggio use 00068 if (wave == 2) o->cinc >>= 1; // correct pitch for saw wave 00069 if (wave == 4) o->cinc <<= 1; // enable higher pitch for pure noise 00070 if (wave == 6) o->samplestep = (o->cinc/(cincs[37]>>8)); 00071 o->vol = volume << 8;//volume; 00072 00073 if (adsr) { 00074 o->attack = attack; 00075 o->decay = decay; 00076 o->sustain = sustain<<8; //sustain needs to be multiplied by 256 also 00077 o->release = release; 00078 o->adsrphase = 1; 00079 if (!o->attack) o->adsrvol = o->vol; // start directly, no attack ramp 00080 else o->adsrvol = 0; 00081 } else { 00082 o->attack = 0; 00083 o->decay = 0; 00084 o->sustain = 0; 00085 o->release = 0; 00086 o->adsrphase = 0; 00087 o->adsrvol = o->vol; // will stay same all the time 00088 } 00089 00090 o->pitchbend = 0; //this has to be zeroed always! 00091 if (bendrate != 0) { 00092 o->bendrate = (int32_t)bendrate*(o->cinc/10000); // test value 00093 o->maxbend = (int32_t)maxbend*(o->cinc/100); 00094 //o->samplebendcount = o->maxbend>>24; 00095 //o->samplebendtick = 0; 00096 } else { 00097 o->bendrate = 0; 00098 o->maxbend = 0; 00099 } 00100 } 00101 00102 void setOSC(OSC* o,byte on, byte wave, uint16_t frq, uint8_t volume, uint32_t duration){ 00103 o->on = on; 00104 o->overdrive = 0; 00105 o->kick = 0; 00106 o->wave = wave; 00107 o->loop = 0;//1; 00108 o->echo = 0;//1; //echo shifts left 8 steps to zero 00109 o->echodiv = 0; 00110 o->adsr = 0;//1; 00111 o->attack = 0;//200; 00112 o->decay = 0;//200; 00113 o->sustain = 0;//20; 00114 o->release = 0;//10; 00115 o->adsrphase = 0;//1; 00116 o->arpmode = 0; 00117 o->count = 0; 00118 noiseval = xorshift16(); //random(0,0xFFFF); 00119 o->cinc = ((float)0xFFFFFFFF/(float)POK_AUD_FREQ)*frq; 00120 if (wave == 2) o->cinc >>= 1; // correct pitch for saw wave 00121 if (wave == 4) o->cinc <<= 1; // enable higher pitch for pure noise 00122 o->vol = volume << 8;//volume; 00123 o->adsrvol = o->vol; 00124 o->duration = 0;//duration*100; 00125 o->maxbend = 0;//-4000; 00126 o->bendrate = 0;//1000; 00127 } 00128 00129 00130 00131 void emptyOscillators(){ 00132 osc1.on = false; osc1.wave = 0; osc1.echo = 0; osc1.count = 0; osc1.cinc =0; 00133 osc1.attack = 0; osc1.loop = 0; osc1.adsrphase = 1; osc1.adsr = 1; osc1.decay = 100; 00134 osc1.pitchbend = 0; osc1.bendrate = 0; osc1.maxbend = 0; osc1.sustain = 0; osc1.release = 0; 00135 00136 osc2.on = false; osc2.wave = 0; osc2.echo = 0; osc2.count = 0; osc2.cinc =0; 00137 osc2.attack = 0; osc2.loop = 0; osc2.adsrphase = 1; osc2.adsr = 1; osc2.decay = 100; 00138 osc2.pitchbend = 0; osc2.bendrate = 0; osc2.maxbend = 0; osc2.sustain = 0; osc2.release = 0; 00139 00140 osc3.on = false; osc3.wave = 0; osc3.echo = 0; osc3.count = 0; osc3.cinc =0; 00141 osc3.attack = 0; osc3.loop = 0; osc3.adsrphase = 1; osc3.adsr = 1; osc3.decay = 100; 00142 osc3.pitchbend = 0; osc3.bendrate = 0; osc3.maxbend = 0; osc3.sustain = 0; osc3.release = 0; 00143 } 00144 00145 00146 void testOsc(){ 00147 setOSC(&osc1,1,WTRI,1,0,1,25,127,10,10,20,2,0,0,0,0,0); // C3 = 25 00148 setOSC(&osc2,1,WTRI,1,0,1,29-12,63,2,1,20,2,0,0,14,0,0); // E3 = 29 00149 setOSC(&osc3,1,WSAW,1,0,1,25,15,30,30,20,2,-1,-1000,12,0,0); // G3 = 32 00150 } 00151 00152 void playNote(uint8_t oscnum, uint8_t notenum, uint8_t i) { 00153 OSC* o; 00154 if (oscnum == 1) o = &osc1; else if (oscnum == 2) o = &osc2; else o = &osc3; 00155 setOSC(o,1,patch[i].wave,patch[i].loop,patch[i].echo,patch[i].adsr,notenum,patch[i].vol, 00156 patch[i].attack,patch[i].decay,patch[i].sustain,patch[i].release, 00157 patch[i].maxbend,patch[i].bendrate,patch[i].arpmode,patch[i].overdrive,patch[i].kick); 00158 } 00159 00160 void makeSampleInstruments() { 00161 /* sample instruments for testing */ 00162 patch[0].wave = WSQUARE; 00163 patch[0].on = 1; 00164 patch[0].vol = 127; 00165 patch[0].loop = 0; 00166 patch[0].echo = 0; 00167 00168 patch[0].adsr = 0; 00169 patch[0].attack = 0; 00170 patch[0].decay = 0; 00171 patch[0].sustain = 0; 00172 patch[0].release = 0; 00173 00174 patch[0].maxbend = -1000; 00175 patch[0].bendrate = 100; 00176 patch[0].arpmode = 3; 00177 patch[0].overdrive = 0; 00178 patch[0].kick = 0; 00179 00180 patch[1].wave = WSAW; 00181 patch[1].on = 1; 00182 patch[1].vol = 200; 00183 patch[1].loop = 0; 00184 patch[1].echo = 0; 00185 00186 patch[1].adsr = 0; 00187 patch[1].attack = 0; 00188 patch[1].decay = 0; 00189 patch[1].sustain = 0; 00190 patch[1].release = 0; 00191 00192 patch[1].maxbend = 0; 00193 patch[1].bendrate = 0; 00194 patch[1].arpmode = 1; 00195 patch[1].overdrive = 0; 00196 patch[1].kick = 0; 00197 00198 patch[2].wave = WTRI; 00199 patch[2].on = 1; 00200 patch[2].vol = 127; 00201 patch[2].loop = 0; 00202 patch[2].echo = 0; 00203 00204 patch[2].adsr = 1; 00205 patch[2].attack = 10; 00206 patch[2].decay = 0; 00207 patch[2].sustain = 0; 00208 patch[2].release = 0; 00209 00210 patch[2].maxbend = 0; 00211 patch[2].bendrate = 0; 00212 patch[2].arpmode = 1; 00213 patch[2].overdrive = 0; 00214 patch[2].kick = 0; 00215 00216 patch[3].wave = WNOISE; 00217 patch[3].on = 1; 00218 patch[3].vol = 127; 00219 patch[3].loop = 1; 00220 patch[3].echo = 1; 00221 00222 patch[3].adsr = 1; 00223 patch[3].attack = 0; 00224 patch[3].decay = 30; 00225 patch[3].sustain = 30; 00226 patch[3].release = 5; 00227 00228 patch[3].maxbend = 0; 00229 patch[3].bendrate = 0; 00230 patch[3].arpmode = 0; 00231 patch[3].overdrive = 0; 00232 patch[3].kick = 0; 00233 00234 patch[4].wave = WPNOISE; 00235 patch[4].on = 1; 00236 patch[4].vol = 127; 00237 patch[4].loop = 0; 00238 patch[4].echo = 0; 00239 00240 patch[4].adsr = 1; 00241 patch[4].attack = 0; 00242 patch[4].decay = 30; 00243 patch[4].sustain = 30; 00244 patch[4].release = 5; 00245 00246 patch[4].maxbend = 0; 00247 patch[4].bendrate = 0; 00248 patch[4].arpmode = 1; 00249 patch[4].overdrive = 0; 00250 patch[4].kick = 0; 00251 } 00252 00253
Generated on Tue Jul 12 2022 11:20:40 by
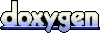