PokittoLib is the library needed for programming the Pokitto DIY game console (www.pokitto.com)
Dependents: YATTT sd_map_test cPong SnowDemo ... more
Synth_mixfuncs.cpp
00001 /**************************************************************************/ 00002 /*! 00003 @file Synth_mixfuncs.cpp 00004 @author Jonne Valola 00005 00006 @section LICENSE 00007 00008 Software License Agreement (BSD License) 00009 00010 Copyright (c) 2016, Jonne Valola 00011 All rights reserved. 00012 00013 Redistribution and use in source and binary forms, with or without 00014 modification, are permitted provided that the following conditions are met: 00015 1. Redistributions of source code must retain the above copyright 00016 notice, this list of conditions and the following disclaimer. 00017 2. Redistributions in binary form must reproduce the above copyright 00018 notice, this list of conditions and the following disclaimer in the 00019 documentation and/or other materials provided with the distribution. 00020 3. Neither the name of the copyright holders nor the 00021 names of its contributors may be used to endorse or promote products 00022 derived from this software without specific prior written permission. 00023 00024 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS ''AS IS'' AND ANY 00025 EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00026 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER BE LIABLE FOR ANY 00028 DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00029 (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00030 LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00031 ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00033 SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 */ 00035 /**************************************************************************/ 00036 00037 #include "PokittoGlobs.h " 00038 #include "Synth.h " 00039 00040 /** MIXING FUNCTIONS **/ 00041 00042 char voltick=0; // i need to make volume changes even slower 00043 uint16_t arptick=0; // i need to make volume changes even slower 00044 int8_t bendtick = 0; // ditto for bend. 00045 00046 00047 void mix1 (){ 00048 // Track 1 00049 if (osc1.on) { 00050 Farr[osc1.wave](&osc1); 00051 //#if PROJ_ARDUBOY > 0 00052 if (osc1.duration) { 00053 /**this is special for osc1 and is only used to emulate arduino Tone(); */ 00054 osc1.duration--; 00055 } else osc1.on = 0; 00056 //#endif 00057 00058 #ifdef POK_SIM 00059 soundbyte = (((osc1.output>>8) * (osc1.adsrvol >>8 )) >> 8) >> osc1.echodiv; // To output, shift back to 8-bit 00060 if (osc1.overdrive) soundbyte *= OVERDRIVE; 00061 if (osc1.kick ) soundbyte >>= 2; 00062 osc1.output = soundbyte; 00063 #else 00064 //OCR2B = osc1.output>>8; 00065 #if POK_ENABLE_SOUND > 0 00066 soundbyte = (((osc1.output>>8) * (osc1.adsrvol >>8 )) >> 8) >> osc1.echodiv; // To output, shift back to 8-bit 00067 if (osc1.overdrive) soundbyte *= OVERDRIVE; 00068 if (osc1.kick ) soundbyte >>= 2; 00069 osc1.output = soundbyte; 00070 #endif 00071 #endif 00072 } 00073 } 00074 00075 void mix2(){ 00076 // Track 2 00077 if (osc2.on) { 00078 Farr[osc2.wave](&osc2); 00079 if (osc2.duration) { 00080 osc2.duration--; 00081 } else osc2.on = 0; 00082 00083 #ifdef POK_SIM 00084 soundbyte = (((osc2.output>>8) * (osc2.adsrvol >>8 )) >> 8) >> osc2.echodiv; 00085 if (osc2.overdrive) soundbyte *= OVERDRIVE; 00086 if (osc2.kick ) soundbyte >>= 2; 00087 osc2.output = soundbyte; 00088 #else 00089 //OCR2B = osc2.output>>8; 00090 #if POK_ENABLE_SOUND > 0 00091 soundbyte = (((osc2.output>>8) * (osc2.adsrvol >>8 )) >> 8) >> osc2.echodiv; 00092 if (osc2.overdrive) soundbyte *= OVERDRIVE; 00093 if (osc2.kick ) soundbyte >>= 2; 00094 osc2.output = soundbyte; 00095 #endif 00096 #endif 00097 } 00098 } 00099 00100 void mix3(){ 00101 // Track 3 00102 if (osc3.on) { 00103 Farr[osc3.wave](&osc3); 00104 if (osc3.duration) { 00105 osc3.duration--; 00106 } else osc3.on = 0; 00107 #ifdef POK_SIM 00108 soundbyte = (((osc3.output>>8) * (osc3.adsrvol >>8 )) >> 8) >> osc3.echodiv; 00109 if (osc3.overdrive) soundbyte *= OVERDRIVE; 00110 if (osc3.kick ) soundbyte >>= 2; 00111 osc3.output = soundbyte; 00112 #else 00113 //OCR2B = osc3.output>>8; 00114 #if POK_ENABLE_SOUND > 0 00115 soundbyte = (((osc3.output>>8) * (osc3.adsrvol >>8 )) >> 8) >> osc3.echodiv; 00116 if (osc3.overdrive) soundbyte *= OVERDRIVE; 00117 if (osc3.kick ) soundbyte >>= 2; 00118 osc3.output = soundbyte; 00119 #endif 00120 #endif 00121 } 00122 } 00123 00124 void updateEnvelopes(){ 00125 //calculate volume envelopes, I do this to save cpu power 00126 #if POK_ALT_MIXING > 0 00127 if (arptick) --arptick; 00128 #else 00129 if (arptick) --arptick; 00130 #endif 00131 else { 00132 if (osc1.arpmode && osc1.on) { 00133 osc1.cinc = cincs[osc1.tonic+arptable[osc1.arpmode][osc1.arpstep]]; 00134 osc1.arpstep++; 00135 if (osc1.arpstep==ARPSTEPMAX) osc1.arpstep = 0; 00136 arptick = ARPTICK << (3-osc1.arpspeed); 00137 } 00138 if (osc2.arpmode && osc2.on) { 00139 osc2.cinc = cincs[osc2.tonic+arptable[osc2.arpmode][osc2.arpstep]]; 00140 osc2.arpstep++; 00141 if (osc2.arpstep==ARPSTEPMAX) osc2.arpstep = 0; 00142 arptick = ARPTICK << (3-osc2.arpspeed); 00143 } 00144 if (osc3.arpmode && osc3.on) { 00145 osc3.cinc = cincs[osc3.tonic+arptable[osc3.arpmode][osc3.arpstep]]; 00146 osc3.arpstep++; 00147 if (osc3.arpstep==ARPSTEPMAX) osc3.arpstep = 0; 00148 arptick = ARPTICK << (3-osc3.arpspeed); 00149 } 00150 00151 } 00152 00153 #if POK_ALT_MIXING > 0 00154 if (voltick) --voltick; 00155 #else 00156 if (voltick) --voltick; 00157 #endif 00158 else { 00159 bendtick = !bendtick; 00160 if (osc1.on) Earr[osc1.adsrphase](&osc1); 00161 if (bendtick) { 00162 osc1.pitchbend += osc1.bendrate; //slow bend to every second beat 00163 /*if (osc1.wave == 6 && osc1.bendrate) { 00164 if (osc1.samplebendtick > osc1.samplebendcount) { 00165 if (osc1.bendrate>0) osc1.samplestep++; 00166 else if (osc1.bendrate<0) osc1.samplestep--; 00167 osc1.samplebendtick=0; 00168 } else osc1.samplebendtick++; 00169 00170 }*/ 00171 } 00172 if (osc1.bendrate > 0 && osc1.pitchbend > osc1.maxbend) { 00173 osc1.pitchbend = osc1.maxbend; 00174 osc1.bendrate = 0; // STOP BENDING ! 00175 } 00176 else if (osc1.bendrate < 0 && osc1.pitchbend < osc1.maxbend) { 00177 osc1.pitchbend = osc1.maxbend; 00178 osc1.bendrate = 0; // STOP BENDING ! 00179 } 00180 00181 if (osc2.on) Earr[osc2.adsrphase](&osc2); 00182 if (bendtick) osc2.pitchbend += osc2.bendrate; 00183 if (osc2.bendrate > 0 && osc2.pitchbend > osc2.maxbend) osc2.pitchbend = osc2.maxbend; 00184 else if (osc2.bendrate < 0 && osc2.pitchbend < osc2.maxbend) osc2.pitchbend = osc2.maxbend; 00185 00186 if (osc3.on) Earr[osc3.adsrphase](&osc3); 00187 if (bendtick) osc3.pitchbend += osc3.bendrate; 00188 if (osc3.bendrate > 0 && osc3.pitchbend > osc3.maxbend) osc3.pitchbend = osc3.maxbend; 00189 else if (osc3.bendrate < 0 && osc3.pitchbend < osc3.maxbend) osc3.pitchbend = osc3.maxbend; 00190 00191 voltick = VOLTICK; 00192 } 00193 tick = 4; 00194 } 00195 00196
Generated on Tue Jul 12 2022 11:20:40 by
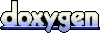