PokittoLib is the library needed for programming the Pokitto DIY game console (www.pokitto.com)
Dependents: YATTT sd_map_test cPong SnowDemo ... more
Serial.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_SERIAL_H 00017 #define MBED_SERIAL_H 00018 00019 #include "platform.h" 00020 00021 #if DEVICE_SERIAL 00022 00023 #include "Stream.h" 00024 #include "SerialBase.h" 00025 #include "serial_api.h" 00026 00027 namespace mbed { 00028 00029 /** A serial port (UART) for communication with other serial devices 00030 * 00031 * Can be used for Full Duplex communication, or Simplex by specifying 00032 * one pin as NC (Not Connected) 00033 * 00034 * Example: 00035 * @code 00036 * // Print "Hello World" to the PC 00037 * 00038 * #include "mbed.h" 00039 * 00040 * Serial pc(USBTX, USBRX); 00041 * 00042 * int main() { 00043 * pc.printf("Hello World\n"); 00044 * } 00045 * @endcode 00046 */ 00047 class Serial : public SerialBase, public Stream { 00048 00049 public: 00050 #if DEVICE_SERIAL_ASYNCH 00051 using SerialBase::read; 00052 using SerialBase::write; 00053 #endif 00054 00055 /** Create a Serial port, connected to the specified transmit and receive pins 00056 * 00057 * @param tx Transmit pin 00058 * @param rx Receive pin 00059 * 00060 * @note 00061 * Either tx or rx may be specified as NC if unused 00062 */ 00063 Serial(PinName tx, PinName rx, const char *name=NULL); 00064 00065 protected: 00066 virtual int _getc(); 00067 virtual int _putc(int c); 00068 }; 00069 00070 } // namespace mbed 00071 00072 #endif 00073 00074 #endif
Generated on Tue Jul 12 2022 11:20:40 by
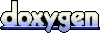