PokittoLib is the library needed for programming the Pokitto DIY game console (www.pokitto.com)
Dependents: YATTT sd_map_test cPong SnowDemo ... more
PokittoSound.h
00001 /**************************************************************************/ 00002 /*! 00003 @file PokittoSound.h 00004 @author Jonne Valola 00005 00006 @section LICENSE 00007 00008 Software License Agreement (BSD License) 00009 00010 Copyright (c) 2016, Jonne Valola 00011 All rights reserved. 00012 00013 Redistribution and use in source and binary forms, with or without 00014 modification, are permitted provided that the following conditions are met: 00015 1. Redistributions of source code must retain the above copyright 00016 notice, this list of conditions and the following disclaimer. 00017 2. Redistributions in binary form must reproduce the above copyright 00018 notice, this list of conditions and the following disclaimer in the 00019 documentation and/or other materials provided with the distribution. 00020 3. Neither the name of the copyright holders nor the 00021 names of its contributors may be used to endorse or promote products 00022 derived from this software without specific prior written permission. 00023 00024 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS ''AS IS'' AND ANY 00025 EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00026 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER BE LIABLE FOR ANY 00028 DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00029 (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00030 LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00031 ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00033 SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 */ 00035 /**************************************************************************/ 00036 00037 00038 #ifndef POKITTOSOUND_H 00039 #define POKITTOSOUND_H 00040 00041 #include <stdint.h> 00042 #include "Pokitto_settings.h " 00043 #include "GBcompatibility.h" 00044 #include "PokittoFakeavr.h " 00045 #include "PokittoGlobs.h " 00046 00047 extern void pokPauseStream(); 00048 extern void pokPlayStream(); 00049 extern uint8_t pokStreamPaused(); 00050 00051 //volume levels 00052 #define GLOBVOL_SHIFT 5 //shift global volume to allow for finer increments 00053 00054 00055 #ifndef MAX_VOL_TEST 00056 #define VOLUME_SPEAKER_MAX 255 //((8<<GLOBVOL_SHIFT)-1) 00057 #define VOLUME_HEADPHONE_MAX 127 00058 #define VOLUME_STARTUP VOLUME_HEADPHONE_MAX 00059 #else 00060 #define VOLUME_SPEAKER_MAX 255 00061 #define VOLUME_HEADPHONE_MAX VOLUME_SPEAKER_MAX 00062 #define VOLUME_STARTUP VOLUME_SPEAKER_MAX 00063 #endif // MAXVOLTEST 00064 00065 #ifdef POK_SIM 00066 #define VOLUME_STEP 1 00067 #else 00068 #define VOLUME_STEP 8 00069 #endif 00070 00071 00072 //commands 00073 #define CMD_VOLUME 0 00074 #define CMD_INSTRUMENT 1 00075 #define CMD_SLIDE 2 00076 #define CMD_ARPEGGIO 3 00077 #define CMD_TREMOLO 4 00078 00079 #define STR_PLAYING 0x1 00080 #define STR_VISUALIZER 0x2 00081 #define STR_LOOP 0x4 00082 #define STR_PAUSED 0x8 00083 00084 namespace Pokitto { 00085 00086 /** Sound class. 00087 * The Sound class is an API-compatible version of the Gamebuino Sound API by Aurelien Rodot. 00088 * Because it is a class consisting of only static members, there is only 1 instance running, 00089 * no matter how many Sound classes are declared (see example below). This means sound can 00090 * be used through a simple Sound class object or as a member of the Core class. 00091 * 00092 */ 00093 00094 /** discrete_vol* are needed for more accurate volume control levels on hardware **/ 00095 extern uint8_t discrete_vol; 00096 extern const uint8_t discrete_vol_levels[]; 00097 extern const uint8_t discrete_vol_hw_levels[]; 00098 extern const uint8_t discrete_vol_multipliers[]; 00099 00100 extern void audio_IRQ(); // audio interrupt 00101 00102 class Sound { 00103 private: 00104 static uint16_t volumeMax; 00105 00106 public: 00107 static const uint8_t *sfxDataPtr; 00108 static const uint8_t *sfxEndPtr; 00109 static void playSFX( const uint8_t *sfx, uint32_t length ){ 00110 sfxDataPtr = sfx; 00111 sfxEndPtr = sfx + length; 00112 }; 00113 00114 static void begin(); 00115 00116 // Headphonemode 00117 static uint8_t headPhoneLevel; // a workaround to disappearing sound at low volume 00118 static void setMaxVol(int16_t); 00119 static uint16_t getMaxVol(); 00120 static void volumeUp(); 00121 static void volumeDown(); 00122 00123 // Synth using samples support 00124 static void loadSampleToOsc(uint8_t os, uint8_t* sampdata, uint32_t sampsize); 00125 00126 // Original functions 00127 static void updateStream(); 00128 static void playTone(uint8_t os, int frq, uint8_t amp, uint8_t wav,uint8_t arpmode); 00129 static void playTone(uint8_t os, uint16_t freq, uint8_t volume, uint32_t duration); 00130 static uint8_t ampIsOn(); 00131 static void ampEnable(uint8_t); 00132 static int playMusicStream(char* filename, uint8_t options); 00133 static int playMusicStream(char* filename); 00134 static int playMusicStream(); 00135 static void pauseMusicStream(); 00136 static uint32_t getMusicStreamElapsedSec(); 00137 static uint32_t getMusicStreamElapsedMilliSec(); 00138 00139 // GB compatibility functions 00140 static void playTrack(const uint16_t* track, uint8_t channel); 00141 static void updateTrack(uint8_t channel); 00142 static void updateTrack(); 00143 static void stopTrack(uint8_t channel); 00144 static void stopTrack(); 00145 static void changePatternSet(const uint16_t* const* patterns, uint8_t channel); 00146 static bool trackIsPlaying[NUM_CHANNELS]; 00147 00148 static void playPattern(const uint16_t* pattern, uint8_t channel); 00149 static void changeInstrumentSet(const uint16_t* const* instruments, uint8_t channel); 00150 static void updatePattern(uint8_t i); 00151 static void updatePattern(); 00152 static void setPatternLooping(bool loop, uint8_t channel); 00153 static void stopPattern(uint8_t channel); 00154 static void stopPattern(); 00155 static bool patternIsPlaying[NUM_CHANNELS]; 00156 00157 static void command(uint8_t cmd, uint8_t X, int8_t Y, uint8_t i); 00158 static void playNote(uint8_t pitch, uint8_t duration, uint8_t channel); 00159 static void updateNote(); 00160 static void updateNote(uint8_t i); 00161 static void stopNote(uint8_t channel); 00162 static void stopNote(); 00163 00164 static uint8_t outputPitch[NUM_CHANNELS]; 00165 static int8_t outputVolume[NUM_CHANNELS]; 00166 00167 static void setMasterVolume(uint8_t); 00168 static uint8_t GetMasterVolume(); 00169 static void setVolume(int16_t volume); 00170 static uint16_t getVolume(); 00171 static void setVolume(int8_t volume, uint8_t channel); 00172 static uint8_t getVolume(uint8_t channel); 00173 00174 static void playOK(); 00175 static void playCancel(); 00176 static void playTick(); 00177 00178 static uint8_t prescaler; 00179 00180 static void setChannelHalfPeriod(uint8_t channel, uint8_t halfPeriod); 00181 00182 static void generateOutput(); //!\\ DO NOT USE 00183 static uint16_t globalVolume; 00184 00185 00186 #if (NUM_CHANNELS > 0) 00187 //tracks data 00188 static uint16_t *trackData[NUM_CHANNELS]; 00189 static uint8_t trackCursor[NUM_CHANNELS]; 00190 static uint16_t **patternSet[NUM_CHANNELS]; 00191 static int8_t patternPitch[NUM_CHANNELS]; 00192 00193 // pattern data 00194 static uint16_t *patternData[NUM_CHANNELS]; 00195 static uint16_t **instrumentSet[NUM_CHANNELS]; 00196 static bool patternLooping[NUM_CHANNELS]; 00197 static uint16_t patternCursor[NUM_CHANNELS]; 00198 00199 // note data 00200 static uint8_t notePitch[NUM_CHANNELS]; 00201 static uint8_t noteDuration[NUM_CHANNELS]; 00202 static int8_t noteVolume[NUM_CHANNELS]; 00203 static bool notePlaying[NUM_CHANNELS]; 00204 00205 // commands data 00206 static int8_t commandsCounter[NUM_CHANNELS]; 00207 static int8_t volumeSlideStepDuration[NUM_CHANNELS]; 00208 static int8_t volumeSlideStepSize[NUM_CHANNELS]; 00209 static uint8_t arpeggioStepDuration[NUM_CHANNELS]; 00210 static int8_t arpeggioStepSize[NUM_CHANNELS]; 00211 static uint8_t tremoloStepDuration[NUM_CHANNELS]; 00212 static int8_t tremoloStepSize[NUM_CHANNELS]; 00213 00214 00215 // instrument data 00216 static uint16_t *instrumentData[NUM_CHANNELS]; 00217 static uint8_t instrumentLength[NUM_CHANNELS]; //number of steps in the instrument 00218 static uint8_t instrumentLooping[NUM_CHANNELS]; //how many steps to loop on when the last step of the instrument is reached 00219 static uint16_t instrumentCursor[NUM_CHANNELS]; //which step is being played 00220 static uint8_t instrumentNextChange[NUM_CHANNELS]; //how many frames before the next step 00221 00222 //current step data 00223 static int8_t stepVolume[NUM_CHANNELS]; 00224 static uint8_t stepPitch[NUM_CHANNELS]; 00225 00226 static uint8_t chanVolumes[NUM_CHANNELS]; 00227 #endif 00228 static void updateOutput(); 00229 }; 00230 00231 } 00232 00233 #endif // POKITTOSOUND_H 00234 00235 00236 00237 00238 00239
Generated on Tue Jul 12 2022 11:20:38 by
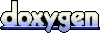