PokittoLib is the library needed for programming the Pokitto DIY game console (www.pokitto.com)
Dependents: YATTT sd_map_test cPong SnowDemo ... more
PokittoItoa.cpp
00001 /**************************************************************************/ 00002 /*! 00003 @file PokittoItoa.cpp 00004 @author Jonne Valola 00005 00006 @section LICENSE 00007 00008 Software License Agreement (BSD License) 00009 00010 Copyright (c) 2016, Jonne Valola 00011 All rights reserved. 00012 00013 Redistribution and use in source and binary forms, with or without 00014 modification, are permitted provided that the following conditions are met: 00015 1. Redistributions of source code must retain the above copyright 00016 notice, this list of conditions and the following disclaimer. 00017 2. Redistributions in binary form must reproduce the above copyright 00018 notice, this list of conditions and the following disclaimer in the 00019 documentation and/or other materials provided with the distribution. 00020 3. Neither the name of the copyright holders nor the 00021 names of its contributors may be used to endorse or promote products 00022 derived from this software without specific prior written permission. 00023 00024 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS ''AS IS'' AND ANY 00025 EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00026 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER BE LIABLE FOR ANY 00028 DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00029 (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00030 LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00031 ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00033 SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 */ 00035 /**************************************************************************/ 00036 00037 #include "PokittoItoa.h " 00038 #include <string.h> 00039 #include <stdint.h> 00040 00041 /* A utility function to reverse a string */ 00042 void reverse(char str[], int length) 00043 { 00044 int start = 0; 00045 int end = length -1; 00046 while (start < end) 00047 { 00048 char t = *(str+end); 00049 *(str+end) = *(str+start); 00050 *(str+start) = t; 00051 //std::swap(*(str+start), *(str+end)); 00052 start++; 00053 end--; 00054 } 00055 } 00056 00057 void pokItoa(uint16_t value, char *str, int base) 00058 { 00059 /** pokItoa: convert value n to characters 00060 * Needed to emulate AVR LibC non-standard functions, this is a very fast version of itoa 00061 * 00062 * @param value unsigned integer to be converted 00063 * @param *str pointer to string where function stores the result as characters 00064 * @param base (not implemented in this function, base is always 10) 00065 */ 00066 00067 char *p = str; 00068 uint8_t v; 00069 if (value > 499) 00070 { 00071 if (value > 799) 00072 { 00073 if (value > 999) 00074 { 00075 // Treated as a special case as there 00076 // are only 24 values above 999 00077 *p++ = '1'; 00078 *p++ = '0'; 00079 uint8_t v = value - 1000; 00080 00081 if (v > 19) { *p++ = '2'; v -= 20; } 00082 else if (v > 9) { *p++ = '1'; v -= 10; } 00083 else { *p++ = '0'; } 00084 00085 *p++ = v + '0'; 00086 *p = 0; 00087 return; 00088 } 00089 00090 if (value > 899) { *p++ = '9'; v = value - 900; } 00091 else { *p++ = '8'; v = value - 800; } 00092 } 00093 else 00094 { 00095 if (value > 699) { *p++ = '7'; v = value - 700; } 00096 else if (value > 599) { *p++ = '6'; v = value - 600; } 00097 else { *p++ = '5'; v = value - 500; } 00098 } 00099 } 00100 else 00101 { 00102 if (value > 299) 00103 { 00104 if (value > 399) { *p++ = '4'; v = value - 400; } 00105 else { *p++ = '3'; v = value - 300; } 00106 } 00107 else 00108 { 00109 if (value > 199) { *p++ = '2'; v = value - 200; } 00110 else if (value > 99) { *p++ = '1'; v = value - 100; } 00111 else { v = value; } 00112 } 00113 } 00114 00115 if (v > 49) 00116 { 00117 if (v > 69) 00118 { 00119 if (v > 89) { *p++ = '9'; v -= 90; } 00120 else if (v > 79) { *p++ = '8'; v -= 80; } 00121 else { *p++ = '7'; v -= 70; } 00122 } 00123 else 00124 { 00125 if (v > 59) { *p++ = '6'; v -= 60; } 00126 else { *p++ = '5'; v -= 50; } 00127 } 00128 } 00129 else 00130 { 00131 if (v > 19) 00132 { 00133 if (v > 39) { *p++ = '4'; v -= 40; } 00134 else if (v > 29) { *p++ = '3'; v -= 30; } 00135 else { *p++ = '2'; v -= 20; } 00136 } 00137 else 00138 { 00139 if (v > 9) { *p++ = '1'; v -= 10; } 00140 else if (p != str) { *p++ = '0'; } 00141 } 00142 } 00143 00144 *p++ = v + '0'; 00145 *p = 0; 00146 } 00147 00148 void pokUtoa(uint16_t value, char *str, int base) { 00149 pokItoa(value,str,base); 00150 } 00151 00152 void pokLtoa(long num, char *str, int base) { 00153 int i = 0; 00154 bool isNegative = false; 00155 00156 /* Handle 0 explicitely, otherwise empty string is printed for 0 */ 00157 if (num == 0) 00158 { 00159 str[i++] = '0'; 00160 str[i] = '\0'; 00161 return;// str; 00162 } 00163 00164 // In standard itoa(), negative numbers are handled only with 00165 // base 10. Otherwise numbers are considered unsigned. 00166 if (num < 0 && base == 10) 00167 { 00168 isNegative = true; 00169 num = -num; 00170 } 00171 00172 // Process individual digits 00173 while (num != 0) 00174 { 00175 int rem = num % base; 00176 str[i++] = (rem > 9)? (rem-10) + 'a' : rem + '0'; 00177 num = num/base; 00178 } 00179 00180 // If number is negative, append '-' 00181 if (isNegative) 00182 str[i++] = '-'; 00183 00184 str[i] = '\0'; // Append string terminator 00185 00186 // Reverse the string 00187 reverse(str, i); 00188 00189 return;// str; 00190 } 00191 00192
Generated on Tue Jul 12 2022 11:20:37 by
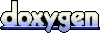