PokittoLib is the library needed for programming the Pokitto DIY game console (www.pokitto.com)
Dependents: YATTT sd_map_test cPong SnowDemo ... more
HWSound.h
00001 /**************************************************************************/ 00002 /*! 00003 @file HWSOUND.h 00004 @author Jonne Valola 00005 00006 @section LICENSE 00007 00008 Software License Agreement (BSD License) 00009 00010 Copyright (c) 2016, Jonne Valola 00011 All rights reserved. 00012 00013 Redistribution and use in source and binary forms, with or without 00014 modification, are permitted provided that the following conditions are met: 00015 1. Redistributions of source code must retain the above copyright 00016 notice, this list of conditions and the following disclaimer. 00017 2. Redistributions in binary form must reproduce the above copyright 00018 notice, this list of conditions and the following disclaimer in the 00019 documentation and/or other materials provided with the distribution. 00020 3. Neither the name of the copyright holders nor the 00021 names of its contributors may be used to endorse or promote products 00022 derived from this software without specific prior written permission. 00023 00024 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS ''AS IS'' AND ANY 00025 EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00026 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER BE LIABLE FOR ANY 00028 DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00029 (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00030 LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00031 ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00033 SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 */ 00035 /**************************************************************************/ 00036 #ifndef HWSOUND_H 00037 #define HWSOUND_H 00038 00039 #include "mbed.h" 00040 #include "Pokitto_settings.h " 00041 00042 #define SPEAKER 3 00043 #if POK_HIGH_RAM == HIGH_RAM_MUSIC 00044 #define BUFFER_SIZE 256*4 00045 #define SBUFSIZE 256*4 00046 #else 00047 #define BUFFER_SIZE 512*4 //*8 //*8 // 512 // was 512 (works really well with crabator) was 256 00048 #define SBUFSIZE 512*4 00049 #endif 00050 00051 #if POK_BOARDREV == 1 00052 /** 2-layer board rev 1.3 **/ 00053 #define DAC0_PORT 1 00054 #define DAC0_PIN 6 00055 #define DAC1_PORT 1 00056 #define DAC1_PIN 0 00057 #define DAC2_PORT 1 00058 #define DAC2_PIN 16 00059 #define DAC3_PORT 0 00060 #define DAC3_PIN 19 00061 #define DAC4_PORT 0 00062 #define DAC4_PIN 17 00063 #define DAC5_PORT 1 00064 #define DAC5_PIN 12 00065 #define DAC6_PORT 1 00066 #define DAC6_PIN 15 00067 #define DAC7_PORT 1 00068 #define DAC7_PIN 8 00069 #else 00070 /** 4-layer board rev 2.1 **/ 00071 #define DAC0_PORT 1 00072 #define DAC0_PIN 28 00073 #define DAC1_PORT 1 00074 #define DAC1_PIN 29 00075 #define DAC2_PORT 1 00076 #define DAC2_PIN 30 00077 #define DAC3_PORT 1 00078 #define DAC3_PIN 31 00079 /** has daniel made a mistake with dac ? **/ 00080 #define DAC4_PORT 2 00081 #define DAC4_PIN 20 00082 #define DAC5_PORT 2 00083 #define DAC5_PIN 21 00084 #define DAC6_PORT 2 00085 #define DAC6_PIN 22 00086 #define DAC7_PORT 2 00087 #define DAC7_PIN 23 00088 00089 /** MASK FOR FASTER DAC **/ 00090 #define SET_MASK_DAC LPC_GPIO_PORT->MASK[1] = ~(0xF0078000); //mask P1_15...P1_18 and P1_28...P1_31 00091 #define CLR_MASK_DAC LPC_GPIO_PORT->MASK[1] = 0; // all on 00092 00093 /* fixing wrong pins from daniel*/ 00094 #define SET_MASK_DAC_LO LPC_GPIO_PORT->MASK[1] = ~(0xF0000000); //mask P1_28...P1_31 00095 #define CLR_MASK_DAC_LO LPC_GPIO_PORT->MASK[1] = 0; // all on 00096 #define SET_MASK_DAC_HI LPC_GPIO_PORT->MASK[2] = ~(0xF00000); //mask P2_20...P2_23 00097 #define CLR_MASK_DAC_HI LPC_GPIO_PORT->MASK[2] = 0; // all on 00098 00099 #endif 00100 00101 #define CLR_DAC0 LPC_GPIO_PORT->CLR[DAC0_PORT] = 1UL << DAC0_PIN; 00102 #define SET_DAC0 LPC_GPIO_PORT->SET[DAC0_PORT] = 1UL << DAC0_PIN; 00103 #define CLR_DAC1 LPC_GPIO_PORT->CLR[DAC1_PORT] = 1UL << DAC1_PIN; 00104 #define SET_DAC1 LPC_GPIO_PORT->SET[DAC1_PORT] = 1UL << DAC1_PIN; 00105 #define CLR_DAC2 LPC_GPIO_PORT->CLR[DAC2_PORT] = 1UL << DAC2_PIN; 00106 #define SET_DAC2 LPC_GPIO_PORT->SET[DAC2_PORT] = 1UL << DAC2_PIN; 00107 #define CLR_DAC3 LPC_GPIO_PORT->CLR[DAC3_PORT] = 1UL << DAC3_PIN; 00108 #define SET_DAC3 LPC_GPIO_PORT->SET[DAC3_PORT] = 1UL << DAC3_PIN; 00109 #define CLR_DAC4 LPC_GPIO_PORT->CLR[DAC4_PORT] = 1UL << DAC4_PIN; 00110 #define SET_DAC4 LPC_GPIO_PORT->SET[DAC4_PORT] = 1UL << DAC4_PIN; 00111 #define CLR_DAC5 LPC_GPIO_PORT->CLR[DAC5_PORT] = 1UL << DAC5_PIN; 00112 #define SET_DAC5 LPC_GPIO_PORT->SET[DAC5_PORT] = 1UL << DAC5_PIN; 00113 #define CLR_DAC6 LPC_GPIO_PORT->CLR[DAC6_PORT] = 1UL << DAC6_PIN; 00114 #define SET_DAC6 LPC_GPIO_PORT->SET[DAC6_PORT] = 1UL << DAC6_PIN; 00115 #define CLR_DAC7 LPC_GPIO_PORT->CLR[DAC7_PORT] = 1UL << DAC7_PIN; 00116 #define SET_DAC7 LPC_GPIO_PORT->SET[DAC7_PORT] = 1UL << DAC7_PIN; 00117 00118 /** the output holder **/ 00119 extern uint16_t soundbyte; 00120 00121 00122 namespace Pokitto { 00123 00124 /** stream output and status */ 00125 extern uint8_t streambyte, streamon, HWvolume; 00126 00127 extern float pwm2; //virtual pwm output 00128 extern void soundInit(); 00129 extern void soundInit(uint8_t); 00130 extern void dac_write(uint8_t value); 00131 extern uint8_t ampIsOn(); 00132 extern void ampEnable(uint8_t v); 00133 extern void audio_IRQ(); 00134 extern void updateSDAudioStream(); 00135 extern uint8_t streamPaused(); 00136 extern void pauseStream(); 00137 extern void playStream(); 00138 extern int setHWvolume(uint8_t); 00139 extern uint8_t getHWvolume(); 00140 extern void changeHWvolume(int8_t); 00141 00142 extern pwmout_t audiopwm; 00143 extern uint8_t pokAmpIsOn(); 00144 extern void pokAmpEnable(uint8_t); 00145 00146 extern Ticker audio; 00147 00148 extern void update_SDAudioStream(); 00149 } 00150 00151 00152 extern void pokSoundIRQ (); 00153 extern void pokSoundBufferedIRQ(); 00154 00155 #if POK_STREAMING_MUSIC > 0 00156 #if POK_HIGH_RAM == HIGH_RAM_MUSIC 00157 extern unsigned char *buffers[]; 00158 #else 00159 extern unsigned char buffers[][BUFFER_SIZE]; 00160 #endif 00161 extern volatile int currentBuffer, oldBuffer; 00162 extern volatile int bufindex, vol; 00163 extern volatile unsigned char * currentPtr; 00164 extern volatile unsigned char * endPtr; 00165 extern int8_t streamvol; 00166 extern uint32_t streamcounter; 00167 extern uint8_t streamstep; 00168 #endif 00169 00170 #endif //HWSOUND_H 00171 00172 00173
Generated on Tue Jul 12 2022 11:20:32 by
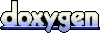