PokittoLib is the library needed for programming the Pokitto DIY game console (www.pokitto.com)
Dependents: YATTT sd_map_test cPong SnowDemo ... more
FileBase.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "FileBase.h" 00017 00018 namespace mbed { 00019 00020 FileBase *FileBase::_head = NULL; 00021 00022 FileBase::FileBase(const char *name, PathType t) : _next(NULL), 00023 _name(name), 00024 _path_type(t) { 00025 if (name != NULL) { 00026 // put this object at head of the list 00027 _next = _head; 00028 _head = this; 00029 } else { 00030 _next = NULL; 00031 } 00032 } 00033 00034 FileBase::~FileBase() { 00035 if (_name != NULL) { 00036 // remove this object from the list 00037 if (_head == this) { // first in the list, so just drop me 00038 _head = _next; 00039 } else { // find the object before me, then drop me 00040 FileBase *p = _head; 00041 while (p->_next != this) { 00042 p = p->_next; 00043 } 00044 p->_next = _next; 00045 } 00046 } 00047 } 00048 00049 FileBase *FileBase::lookup(const char *name, unsigned int len) { 00050 FileBase *p = _head; 00051 while (p != NULL) { 00052 /* Check that p->_name matches name and is the correct length */ 00053 if (p->_name != NULL && std::strncmp(p->_name, name, len) == 0 && std::strlen(p->_name) == len) { 00054 return p; 00055 } 00056 p = p->_next; 00057 } 00058 return NULL; 00059 } 00060 00061 FileBase *FileBase::get(int n) { 00062 FileBase *p = _head; 00063 int m = 0; 00064 while (p != NULL) { 00065 if (m == n) return p; 00066 00067 m++; 00068 p = p->_next; 00069 } 00070 return NULL; 00071 } 00072 00073 const char* FileBase::getName(void) { 00074 return _name; 00075 } 00076 00077 PathType FileBase::getPathType(void) { 00078 return _path_type; 00079 } 00080 00081 } // namespace mbed 00082
Generated on Tue Jul 12 2022 11:20:32 by
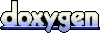