PokittoLib is the library needed for programming the Pokitto DIY game console (www.pokitto.com)
Dependents: YATTT sd_map_test cPong SnowDemo ... more
CallChain.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_CALLCHAIN_H 00017 #define MBED_CALLCHAIN_H 00018 00019 #include "FunctionPointer.h" 00020 #include <string.h> 00021 00022 namespace mbed { 00023 00024 /** Group one or more functions in an instance of a CallChain, then call them in 00025 * sequence using CallChain::call(). Used mostly by the interrupt chaining code, 00026 * but can be used for other purposes. 00027 * 00028 * Example: 00029 * @code 00030 * #include "mbed.h" 00031 * 00032 * CallChain chain; 00033 * 00034 * void first(void) { 00035 * printf("'first' function.\n"); 00036 * } 00037 * 00038 * void second(void) { 00039 * printf("'second' function.\n"); 00040 * } 00041 * 00042 * class Test { 00043 * public: 00044 * void f(void) { 00045 * printf("A::f (class member).\n"); 00046 * } 00047 * }; 00048 * 00049 * int main() { 00050 * Test test; 00051 * 00052 * chain.add(second); 00053 * chain.add_front(first); 00054 * chain.add(&test, &Test::f); 00055 * chain.call(); 00056 * } 00057 * @endcode 00058 */ 00059 00060 typedef FunctionPointer* pFunctionPointer_t; 00061 00062 class CallChain { 00063 public: 00064 /** Create an empty chain 00065 * 00066 * @param size (optional) Initial size of the chain 00067 */ 00068 CallChain(int size = 4); 00069 virtual ~CallChain(); 00070 00071 /** Add a function at the end of the chain 00072 * 00073 * @param function A pointer to a void function 00074 * 00075 * @returns 00076 * The function object created for 'function' 00077 */ 00078 pFunctionPointer_t add(void (*function)(void)); 00079 00080 /** Add a function at the end of the chain 00081 * 00082 * @param tptr pointer to the object to call the member function on 00083 * @param mptr pointer to the member function to be called 00084 * 00085 * @returns 00086 * The function object created for 'tptr' and 'mptr' 00087 */ 00088 template<typename T> 00089 pFunctionPointer_t add(T *tptr, void (T::*mptr)(void)) { 00090 return common_add(new FunctionPointer(tptr, mptr)); 00091 } 00092 00093 /** Add a function at the beginning of the chain 00094 * 00095 * @param function A pointer to a void function 00096 * 00097 * @returns 00098 * The function object created for 'function' 00099 */ 00100 pFunctionPointer_t add_front(void (*function)(void)); 00101 00102 /** Add a function at the beginning of the chain 00103 * 00104 * @param tptr pointer to the object to call the member function on 00105 * @param mptr pointer to the member function to be called 00106 * 00107 * @returns 00108 * The function object created for 'tptr' and 'mptr' 00109 */ 00110 template<typename T> 00111 pFunctionPointer_t add_front(T *tptr, void (T::*mptr)(void)) { 00112 return common_add_front(new FunctionPointer(tptr, mptr)); 00113 } 00114 00115 /** Get the number of functions in the chain 00116 */ 00117 int size() const; 00118 00119 /** Get a function object from the chain 00120 * 00121 * @param i function object index 00122 * 00123 * @returns 00124 * The function object at position 'i' in the chain 00125 */ 00126 pFunctionPointer_t get(int i) const; 00127 00128 /** Look for a function object in the call chain 00129 * 00130 * @param f the function object to search 00131 * 00132 * @returns 00133 * The index of the function object if found, -1 otherwise. 00134 */ 00135 int find(pFunctionPointer_t f) const; 00136 00137 /** Clear the call chain (remove all functions in the chain). 00138 */ 00139 void clear(); 00140 00141 /** Remove a function object from the chain 00142 * 00143 * @arg f the function object to remove 00144 * 00145 * @returns 00146 * true if the function object was found and removed, false otherwise. 00147 */ 00148 bool remove(pFunctionPointer_t f); 00149 00150 /** Call all the functions in the chain in sequence 00151 */ 00152 void call(); 00153 00154 #ifdef MBED_OPERATORS 00155 void operator ()(void) { 00156 call(); 00157 } 00158 pFunctionPointer_t operator [](int i) const { 00159 return get(i); 00160 } 00161 #endif 00162 00163 private: 00164 void _check_size(); 00165 pFunctionPointer_t common_add(pFunctionPointer_t pf); 00166 pFunctionPointer_t common_add_front(pFunctionPointer_t pf); 00167 00168 pFunctionPointer_t* _chain; 00169 int _size; 00170 int _elements; 00171 00172 /* disallow copy constructor and assignment operators */ 00173 private: 00174 CallChain(const CallChain&); 00175 CallChain & operator = (const CallChain&); 00176 }; 00177 00178 } // namespace mbed 00179 00180 #endif 00181
Generated on Tue Jul 12 2022 11:20:30 by
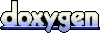