A class that converts byte streams into MIDI messages, and stores them in a FIFO. This is useful if you wish to read MIDI messages via polling instead of interrupts. The class supports every type of MIDI message, and System Realtime messages can be interleaved with regular ones.
MIDIparser.h
00001 /** 00002 * @file MIDIparser.h 00003 * @brief MIDI parser - converts bytes into queued MIDI messages 00004 * @author Patrick Thomas 00005 * @version 1.0 00006 * @see 00007 * 00008 * Copyright (c) 2016 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); 00011 * you may not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, 00018 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 */ 00022 00023 #ifndef MIDIPARSER_MIDIPARSER_H_ 00024 #define MIDIPARSER_MIDIPARSER_H_ 00025 00026 #include "MIDIMessage.h" 00027 #include "MyBuffer.h" 00028 #include <queue> 00029 00030 /** A parser that accepts bytes and outputs MIDI messages 00031 * 00032 * Example: 00033 * @code 00034 * #include "mbed.h" 00035 * #include "MIDIparser.h" 00036 * 00037 * MIDIparser myParser; 00038 * Serial pc(USBTX, USBRX); 00039 * 00040 * int main() 00041 * { 00042 * while(1) { 00043 * 00044 * while(pc.readable()) { 00045 * 00046 * myParser.parse(pc.getc()); 00047 * } 00048 * 00049 * while (myParser.available()) { 00050 * 00051 * MIDIMessage myMessage = myParser.grab(); 00052 * pc.printf("%d %d %d %d\n", myMessage.type(), myMessage.channel(), myMessage.key(), myMessage.velocity()); 00053 * } 00054 * 00055 * } 00056 * } 00057 * @endcode 00058 */ 00059 00060 class MIDIparser { 00061 00062 private: 00063 00064 MyBuffer<uint8_t> input_vector; 00065 queue<MIDIMessage> output_queue; 00066 00067 bool sysex; 00068 bool dual; 00069 00070 public: 00071 00072 /** Create a parser and allocate input/output buffers 00073 */ 00074 MIDIparser(); 00075 00076 /** Input a byte to the parser 00077 * @param data The byte to parse 00078 */ 00079 void parse(uint8_t data); 00080 00081 /** Determine if a MIDI message is in the queue 00082 * @return 1 if something is ready, 0 otherwise 00083 */ 00084 uint32_t available(); 00085 00086 /** Grab the next MIDI message 00087 * @return The next MIDI message 00088 */ 00089 MIDIMessage grab(); 00090 }; 00091 00092 #endif /* MIDIPARSER_MIDIPARSER_H_ */
Generated on Tue Jul 12 2022 21:41:49 by
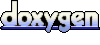