
Test program for the Nordic Semi nRF24L01+ Transceiver Module (http://www.sparkfun.com/products/691), talking to another module connected to SparkFun\'s Nordic Serial Interface Board (http://www.sparkfun.com/products/9019).
main.cpp
00001 #include "mbed.h" 00002 #include "nRF24L01P.h" 00003 00004 Serial pc(USBTX, USBRX); // tx, rx 00005 00006 nRF24L01P my_nrf24l01p(p5, p6, p7, p8, p9, p10); // mosi, miso, sck, csn, ce, irq 00007 00008 DigitalOut myled1(LED1); 00009 DigitalOut myled2(LED2); 00010 00011 int main() { 00012 00013 // The nRF24L01+ supports transfers from 1 to 32 bytes, but Sparkfun's 00014 // "Nordic Serial Interface Board" (http://www.sparkfun.com/products/9019) 00015 // only handles 4 byte transfers in the ATMega code. 00016 #define TRANSFER_SIZE 4 00017 00018 char txData[TRANSFER_SIZE], rxData[TRANSFER_SIZE]; 00019 int txDataCnt = 0; 00020 int rxDataCnt = 0; 00021 00022 my_nrf24l01p.powerUp(); 00023 00024 // Display the (default) setup of the nRF24L01+ chip 00025 pc.printf( "nRF24L01+ Frequency : %d MHz\r\n", my_nrf24l01p.getRfFrequency() ); 00026 pc.printf( "nRF24L01+ Output power : %d dBm\r\n", my_nrf24l01p.getRfOutputPower() ); 00027 pc.printf( "nRF24L01+ Data Rate : %d kbps\r\n", my_nrf24l01p.getAirDataRate() ); 00028 pc.printf( "nRF24L01+ TX Address : 0x%010llX\r\n", my_nrf24l01p.getTxAddress() ); 00029 pc.printf( "nRF24L01+ RX Address : 0x%010llX\r\n", my_nrf24l01p.getRxAddress() ); 00030 00031 pc.printf( "Type keys to test transfers:\r\n (transfers are grouped into %d characters)\r\n", TRANSFER_SIZE ); 00032 00033 my_nrf24l01p.setTransferSize( TRANSFER_SIZE ); 00034 00035 my_nrf24l01p.setReceiveMode(); 00036 my_nrf24l01p.enable(); 00037 00038 while (1) { 00039 00040 // If we've received anything over the host serial link... 00041 if ( pc.readable() ) { 00042 00043 // ...add it to the transmit buffer 00044 txData[txDataCnt++] = pc.getc(); 00045 00046 // If the transmit buffer is full 00047 if ( txDataCnt >= sizeof( txData ) ) { 00048 00049 // Send the transmitbuffer via the nRF24L01+ 00050 my_nrf24l01p.write( NRF24L01P_PIPE_P0, txData, txDataCnt ); 00051 00052 txDataCnt = 0; 00053 } 00054 00055 // Toggle LED1 (to help debug Host -> nRF24L01+ communication) 00056 myled1 = !myled1; 00057 } 00058 00059 // If we've received anything in the nRF24L01+... 00060 if ( my_nrf24l01p.readable() ) { 00061 00062 // ...read the data into the receive buffer 00063 rxDataCnt = my_nrf24l01p.read( NRF24L01P_PIPE_P0, rxData, sizeof( rxData ) ); 00064 00065 // Display the receive buffer contents via the host serial link 00066 for ( int i = 0; rxDataCnt > 0; rxDataCnt--, i++ ) { 00067 00068 pc.putc( rxData[i] ); 00069 } 00070 00071 // Toggle LED2 (to help debug nRF24L01+ -> Host communication) 00072 myled2 = !myled2; 00073 } 00074 } 00075 }
Generated on Tue Jul 12 2022 17:37:07 by
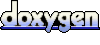