First class data visualization and communication library with embedded devices. Code is maintained at github.com/Overdrivr/Telemetry
Dependents: telemetry_car_demo telemetry_demo_FRDM-TFC telemetry_example_01 telemetry_indexed_data_demo ... more
Telemetry.cpp
00001 #include "Telemetry.hpp" 00002 #include "c_api/telemetry_core.h" 00003 #include "BufferedSerial.h" 00004 00005 static BufferedSerial pc(USBTX, USBRX); 00006 00007 int32_t read(void * buf, uint32_t sizeToRead) 00008 { 00009 *(uint8_t*)(buf) = pc.getc(); 00010 return 1; 00011 } 00012 00013 int32_t write(void * buf, uint32_t sizeToWrite) 00014 { 00015 pc.write(buf,sizeToWrite); 00016 return 0; 00017 } 00018 00019 int32_t readable() 00020 { 00021 return pc.readable(); 00022 } 00023 00024 int32_t writeable() 00025 { 00026 return pc.writeable(); 00027 } 00028 00029 Telemetry::Telemetry(uint32_t bauds) 00030 { 00031 transport.read = read; 00032 transport.write = write; 00033 transport.readable = readable; 00034 transport.writeable = writeable; 00035 00036 init_telemetry(&transport); 00037 00038 pc.baud(bauds); 00039 } 00040 00041 void Telemetry::attach_f32_to(const char * topic, float * variable) 00042 { 00043 attach_f32(topic, variable); 00044 } 00045 00046 void Telemetry::attach_u8_to(const char * topic, uint8_t * variable) 00047 { 00048 attach_u8(topic, variable); 00049 } 00050 00051 void Telemetry::attach_u16_to(const char * topic, uint16_t * variable) 00052 { 00053 attach_u16(topic, variable); 00054 } 00055 00056 void Telemetry::attach_u32_to(const char * topic, uint32_t * variable) 00057 { 00058 attach_u32(topic, variable); 00059 } 00060 00061 void Telemetry::attach_i8_to(const char * topic, int8_t * variable) 00062 { 00063 attach_i8(topic, variable); 00064 } 00065 00066 void Telemetry::attach_i16_to(const char * topic, int16_t * variable) 00067 { 00068 attach_i16(topic, variable); 00069 } 00070 00071 void Telemetry::attach_i32_to(const char * topic, int32_t * variable) 00072 { 00073 attach_i32(topic, variable); 00074 } 00075 00076 void Telemetry::begin(uint32_t bauds) 00077 { 00078 pc.baud(bauds); 00079 } 00080 00081 TM_transport * Telemetry::get_transport() 00082 { 00083 return &transport; 00084 } 00085 00086 void Telemetry::pub(const char * topic, const char * msg) 00087 { 00088 publish(topic,msg); 00089 } 00090 00091 void Telemetry::pub_u8(const char * topic, uint8_t msg) 00092 { 00093 publish_u8(topic,msg); 00094 } 00095 00096 void Telemetry::pub_u16(const char * topic, uint16_t msg) 00097 { 00098 publish_u16(topic,msg); 00099 } 00100 00101 void Telemetry::pub_u32(const char * topic, uint32_t msg) 00102 { 00103 publish_u32(topic,msg); 00104 } 00105 00106 void Telemetry::pub_i8(const char * topic, int8_t msg) 00107 { 00108 publish_i8(topic,msg); 00109 } 00110 00111 void Telemetry::pub_i16(const char * topic, int16_t msg) 00112 { 00113 publish_i16(topic,msg); 00114 } 00115 00116 void Telemetry::pub_i32(const char * topic, int32_t msg) 00117 { 00118 publish_i32(topic,msg); 00119 } 00120 00121 void Telemetry::pub_f32(const char * topic, float msg) 00122 { 00123 publish_f32(topic,msg); 00124 } 00125 00126 void Telemetry::sub(void (*callback)(TM_state * s, TM_msg * m), 00127 TM_state* userData) 00128 { 00129 subscribe(callback,userData); 00130 } 00131 00132 void Telemetry::update() 00133 { 00134 update_telemetry(0); 00135 }
Generated on Wed Jul 13 2022 21:24:00 by
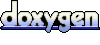