
blablabla
Dependencies: MAG3110 MMA8451Q SLCD- TSI USBDevice mbed
USBSerial Class Reference
#include <USBSerial.h>
Inherits USBCDC.
Public Member Functions | |
USBSerial (uint16_t vendor_id=0x1f00, uint16_t product_id=0x2012, uint16_t product_release=0x0001, bool connect_blocking=true) | |
Constructor. | |
virtual int | _putc (int c) |
Send a character. | |
virtual int | _getc () |
Read a character: blocking. | |
uint8_t | available () |
Check the number of bytes available. | |
int | readable () |
Determine if there is a character available to read. | |
int | writeable () |
Determine if there is space available to write a character. | |
bool | writeBlock (uint8_t *buf, uint16_t size) |
Write a block of data. | |
template<typename T > | |
void | attach (T *tptr, void(T::*mptr)(void)) |
Attach a member function to call when a packet is received. | |
void | attach (void(*fptr)(void)) |
Attach a callback called when a packet is received. | |
void | attach (void(*fptr)(int baud, int bits, int parity, int stop)) |
Attach a callback to call when serial's settings are changed. |
Detailed Description
USBSerial example.
#include "mbed.h" #include "USBSerial.h" //Virtual serial port over USB USBSerial serial; int main(void) { while(1) { serial.printf("I am a virtual serial port\n"); wait(1); } }
Definition at line 47 of file USBSerial.h.
Constructor & Destructor Documentation
USBSerial | ( | uint16_t | vendor_id = 0x1f00 , |
uint16_t | product_id = 0x2012 , |
||
uint16_t | product_release = 0x0001 , |
||
bool | connect_blocking = true |
||
) |
Constructor.
- Parameters:
-
vendor_id Your vendor_id (default: 0x1f00) product_id Your product_id (default: 0x2012) product_release Your preoduct_release (default: 0x0001) connect_blocking define if the connection must be blocked if USB not plugged in
Definition at line 59 of file USBSerial.h.
Member Function Documentation
int _getc | ( | ) | [virtual] |
int _putc | ( | int | c ) | [virtual] |
Send a character.
You can use puts, printf.
- Parameters:
-
c character to be sent
- Returns:
- true if there is no error, false otherwise
Definition at line 22 of file USBSerial.cpp.
void attach | ( | void(*)(void) | fptr ) |
Attach a callback called when a packet is received.
- Parameters:
-
fptr function pointer
Definition at line 132 of file USBSerial.h.
void attach | ( | void(*)(int baud, int bits, int parity, int stop) | fptr ) |
Attach a callback to call when serial's settings are changed.
- Parameters:
-
fptr function pointer
Definition at line 143 of file USBSerial.h.
void attach | ( | T * | tptr, |
void(T::*)(void) | mptr | ||
) |
Attach a member function to call when a packet is received.
- Parameters:
-
tptr pointer to the object to call the member function on mptr pointer to the member function to be called
Definition at line 121 of file USBSerial.h.
uint8_t available | ( | ) |
Check the number of bytes available.
- Returns:
- the number of bytes available
Definition at line 67 of file USBSerial.cpp.
int readable | ( | ) |
Determine if there is a character available to read.
- Returns:
- 1 if there is a character available to read, 0 otherwise
Definition at line 92 of file USBSerial.h.
int writeable | ( | ) |
Determine if there is space available to write a character.
- Returns:
- 1 if there is space to write a character, 0 otherwise
Definition at line 100 of file USBSerial.h.
bool writeBlock | ( | uint8_t * | buf, |
uint16_t | size | ||
) |
Write a block of data.
For more efficiency, a block of size 64 (maximum size of a bulk endpoint) has to be written.
- Parameters:
-
buf pointer on data which will be written size size of the buffer. The maximum size of a block is limited by the size of the endpoint (64 bytes)
- Returns:
- true if successfull
Definition at line 37 of file USBSerial.cpp.
Generated on Tue Jul 12 2022 16:20:44 by
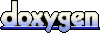