
experimental fork
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "hvcontrol.cpp" 00003 #include "detection.cpp" 00004 //#include <queue> 00005 #include "NOKIA_5110.h" 00006 00007 //set the frequency of the oscillators below 00008 int f1 = 3600; //3500; 00009 int f2 = 3497; 00010 00011 //set the coincidence times in us below 00012 int coincidence_wait = 50; //150; 00013 bool buzzer = 0; 00014 00015 /* NO USER CONFIGURABLE OPTIONS BELOW 00016 */ 00017 00018 //typically around 2 min data 00019 //assuming normal background 00020 #define DETECTION_MEMORY_LIMIT 32 //128 00021 00022 //doesn't log the GM hits with a timestamp 00023 //only counts them when set to 1 00024 #define DETECT_MUONS_ONLY 1 00025 00026 bool mdet = 0; 00027 /*volatile*/ int muon_total; 00028 /*volatile*/ int gm1_total; 00029 /*volatile*/ int gm2_total; 00030 int minutes = 0; //global minutes counter 00031 00032 //storing a detections in a FIFO queue 00033 //queue<Detection> detections; 00034 00035 //High voltage controls 00036 HVControl hv1(PA_3, PA_0); 00037 HVControl hv2(PB_1, PA_1); 00038 00039 //Serial rpi(PA_9,PA_10); 00040 00041 Timer coincidence_timer; 00042 Timer main_t; 00043 00044 Serial pc(USBTX, USBRX); 00045 // Ticker debug; 00046 Ticker hv1monitor; 00047 Ticker hv2monitor; 00048 //Ticker memory_checker; 00049 00050 DigitalOut led(LED1); 00051 DigitalOut GM1_led(PA_12); 00052 DigitalOut GM2_led(PA_8); 00053 DigitalOut C_led(PA_11); 00054 DigitalOut Buzzer(PF_0); 00055 00056 InterruptIn GM1(PB_0); 00057 InterruptIn GM2(PF_1); 00058 00059 //global variables for voltages 00060 volatile float v1 = 0; 00061 volatile float v2 = 0; 00062 00063 //ui functions 00064 void reset_counters (void) 00065 { 00066 muon_total = 0; 00067 gm1_total = 0; 00068 gm2_total = 0; 00069 } 00070 00071 void muon_buzz(void) 00072 { 00073 C_led = 1; 00074 00075 if(buzzer) { 00076 for(int i = 0; i < 32; i++) { 00077 Buzzer = !Buzzer; 00078 wait(0.002); 00079 } 00080 } else { 00081 wait(0.05); 00082 } 00083 C_led = 0; 00084 } 00085 00086 void GM1_buzz(void) 00087 { 00088 GM1_led = 1; 00089 if(buzzer) { 00090 for(int i = 0; i < 16; i++) { 00091 Buzzer = !Buzzer; 00092 wait(0.001); 00093 } 00094 } else { 00095 wait(0.05); 00096 } 00097 GM1_led = 0; 00098 } 00099 00100 void GM2_buzz(void) 00101 { 00102 GM2_led = 1; 00103 if(buzzer) { 00104 for(int i = 0; i < 16; i++) { 00105 Buzzer = !Buzzer; 00106 wait(0.001); 00107 } 00108 } else { 00109 wait(0.05); 00110 } 00111 GM2_led = 0; 00112 } 00113 00114 00115 //adds a detection onto the queue 00116 void add_detection(int channel, float time) 00117 { 00118 //Detection* det = new Detection(channel, time); 00119 //detections.push(*det); 00120 //delete det; 00121 } 00122 00123 //callback for GM1 detection 00124 void GM1_hit(void) 00125 { 00126 coincidence_timer.start(); 00127 while(coincidence_timer.read_us() < coincidence_wait) { 00128 if(GM2 == 0 && !mdet) { 00129 add_detection(3, main_t.read_us()); 00130 muon_buzz(); 00131 muon_total++; 00132 gm2_total++; 00133 mdet = 1; 00134 } 00135 wait(1e-7); 00136 } 00137 coincidence_timer.stop(); 00138 coincidence_timer.reset(); 00139 if(mdet == 0) GM1_buzz(); 00140 if ( !DETECT_MUONS_ONLY ) add_detection(1, main_t.read()); 00141 gm1_total++; 00142 mdet = 0; 00143 } 00144 00145 //callback for GM2 detection 00146 void GM2_hit(void) 00147 { 00148 coincidence_timer.start(); 00149 while(coincidence_timer.read_us() < coincidence_wait) { 00150 if(GM1 == 0 && !mdet) { 00151 add_detection(3, main_t.read()); 00152 muon_buzz(); 00153 muon_total++; 00154 gm1_total++; 00155 mdet = 1; 00156 } 00157 wait(1e-7); 00158 } 00159 coincidence_timer.stop(); 00160 coincidence_timer.reset(); 00161 if(mdet == 0) GM2_buzz(); 00162 if ( !DETECT_MUONS_ONLY ) add_detection(2, main_t.read()); 00163 gm2_total++; 00164 mdet = 0; 00165 } 00166 00167 // high voltage software monitors 00168 void hv1monitor_(void) 00169 { 00170 if(hv1.measure()) { 00171 if(v1 - hv1.get_voltage() > 100) { 00172 hv1.shutdown(); //unusual voltage drop detected 00173 } 00174 } else { 00175 hv1.shutdown(); 00176 } 00177 v1 = hv1.get_voltage(); 00178 } 00179 00180 void hv2monitor_(void) 00181 { 00182 if(hv2.measure()) { 00183 if(v2 - hv2.get_voltage() > 100) { 00184 hv2.shutdown(); //unusual voltage drop detected 00185 } 00186 } else { 00187 hv2.shutdown(); 00188 } 00189 v2 = hv2.get_voltage(); 00190 } 00191 00192 //discarding older items if buffer gets too big 00193 //void memory_checker_(void) 00194 //{ 00195 // if ( detections.size() > (DETECTION_MEMORY_LIMIT - (DETECTION_MEMORY_LIMIT/8)) ) { 00196 // while ( detections.size() > (DETECTION_MEMORY_LIMIT - (DETECTION_MEMORY_LIMIT/4)) ) { 00197 // detections.pop(); 00198 // } 00199 // } 00200 //} 00201 00202 char* itoa(int value, char* result, int base) 00203 { 00204 // check that the base if valid 00205 if ( base < 2 || base > 36 ) { 00206 *result = '\0'; 00207 return result; 00208 } 00209 00210 char* ptr = result, *ptr1 = result, tmp_char; 00211 int tmp_value; 00212 00213 do { 00214 tmp_value = value; 00215 value /= base; 00216 *ptr++ = "zyxwvutsrqponmlkjihgfedcba9876543210123456789abcdefghijklmnopqrstuvwxyz"[35 + (tmp_value - value * base)]; 00217 } while ( value ); 00218 00219 // Apply negative sign 00220 if ( tmp_value < 0 ) 00221 *ptr++ = '-'; 00222 *ptr-- = '\0'; 00223 00224 while ( ptr1 < ptr ) { 00225 tmp_char = *ptr; 00226 *ptr-- = *ptr1; 00227 *ptr1++ = tmp_char; 00228 } 00229 00230 return result; 00231 } 00232 00233 int main() 00234 { 00235 //start main timer 00236 pc.baud(230400); 00237 main_t.start(); 00238 LcdPins myPins; 00239 00240 myPins.rst = PA_6; 00241 myPins.sce = PA_4; 00242 myPins.dc = PB_4; 00243 myPins.mosi = PA_7;//SPI_MOSI; 00244 myPins.miso = NC; 00245 myPins.sclk = PA_5;//SPI_SCK; 00246 00247 // Start the LCD 00248 NokiaLcd myLcd( myPins ); 00249 myLcd.InitLcd(); // LCD is reset and DDRAM is cleared 00250 myLcd.DrawString("MUON HUNTER V2"); 00251 myLcd.SetXY(0,1); 00252 myLcd.DrawString("TIME "); 00253 myLcd.SetXY(0,3); 00254 myLcd.DrawString("MUON COUNT"); 00255 myLcd.SetXY(0,4); 00256 myLcd.DrawString("GM1 COUNT"); 00257 myLcd.SetXY(0,5); 00258 myLcd.DrawString("GM2 COUNT"); 00259 00260 hv1.set_frequency(f1); 00261 hv2.set_frequency(f2); 00262 GM1.fall(&GM1_hit); 00263 GM2.fall(&GM2_hit); 00264 00265 //tickers to monitor the high voltage 00266 hv1monitor.attach(&hv1monitor_, 0.1); 00267 hv2monitor.attach(&hv2monitor_, 0.1); 00268 00269 char number[10]; 00270 00271 //ticker to monitor memory usage 00272 //memory_checker.attach(&memory_checker_, 0.2); 00273 while(1) { 00274 00275 myLcd.SetXY(5*6,1); 00276 unsigned int seconds = (int) main_t.read(); 00277 //int minutes = (seconds/60); 00278 if (seconds >= 60) { 00279 main_t.reset(); 00280 minutes++; 00281 } 00282 if (minutes < 10) 00283 myLcd.DrawChar('0'); 00284 itoa(minutes, number, 10); 00285 myLcd.DrawString(number); 00286 myLcd.DrawChar(':'); 00287 if ((seconds%60) < 10) 00288 myLcd.DrawChar('0'); 00289 itoa(seconds%60, number,10); 00290 myLcd.DrawString(number); 00291 00292 myLcd.SetXY(11*6,3); 00293 itoa(muon_total, number, 10); 00294 myLcd.DrawString(number); 00295 00296 myLcd.SetXY(11*6,4); 00297 itoa(gm1_total, number, 10); 00298 myLcd.DrawString(number); 00299 00300 myLcd.SetXY(11*6,5); 00301 itoa(gm2_total, number, 10); 00302 myLcd.DrawString(number); 00303 00304 00305 // while(!detections.empty()){ 00306 // Detection temp = detections.front(); 00307 // 00308 // pc.printf("Ch: %d, Time: %.2fs, V1: %.1fV, V2: %.1fV \n\r", temp.channel, temp.time, v1, v2); 00309 // pc.printf("Total GM1: %d, Total GM2: %d, Total Coinc: %d \n\r \n\r", gm1_total, gm2_total, muon_total); 00310 // detections.pop(); 00311 // } 00312 00313 pc.printf("%.fs, GM1: %d, GM2: %d, Muons: %d \n\r", main_t.read(), gm1_total, gm2_total, muon_total); 00314 pc.printf("V1: %.1fV, V2: %.1fV \n\r", v1, v2); 00315 wait(1); 00316 } 00317 }
Generated on Sun Jul 17 2022 00:14:18 by
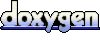