Code to interface with the MCP7940 Real time clock. Supports getting and setting the time in 24 hour format
MCP7940 Class Reference
#include <MCP7940.h>
Public Member Functions | |
MCP7940 (PinName sda, PinName scl) | |
Constructor Start year will default to 2000. | |
MCP7940 (PinName sda, PinName scl, int StartYear) | |
Constructor Start year will default to 2000. | |
int | Day () |
Day Note getTime() must have been called to update the value of day. | |
int | Month () |
Month Note getTime() must have been called to update the value of day. | |
int | Year () |
Year Note getTime() must have been called to update the value of day. | |
int | Hour () |
Hour Note getTime() must have been called to update the value of day. | |
int | Minutes () |
Minutes Note getTime() must have been called to update the value of day. | |
int | Seconds () |
Seconds Note getTime() must have been called to update the value of day. | |
int | MilliSeconds () |
MilliSeconds Note getTime() must have been called to update the value of day. | |
int | DayOfWeek () |
DayOfWeek Note getTime() must have been called to update the value of day. | |
int | setTime () |
SetTime Transfers the values of the time members into the MCP7940. | |
int | setTime (int Year, int Month, int Day, int Hour, int Mins) |
SetTime Transfers the values of the time members into the MCP7940. | |
int | setTime (int Year, int Month, int Day, int Hour, int Mins, int Secs) |
SetTime Transfers the values of the time members into the MCP7940. | |
int | setTime (int Year, int Month, int Day, int Hour, int Mins, int Secs, int MiliSecs) |
SetTime Transfers the values of the time members into the MCP7940. | |
int | setTime (int Year, int Month, int Day, int Hour, int Mins, int Secs, int MiliSecs, int DayOfWeek) |
SetTime Transfers the values of the time members into the MCP7940. | |
int | getTime () |
getTime Loads the time from the MCP7940 so its upto date and can be accessed by the Day(), Month()... | |
void | StartClock () |
StartClock Clears and sets bit 7 of register 0x00 to start the clock. | |
char * | TimeStamp () |
TimeStamp getTime() is called within this function so does not need to be called from code. |
Detailed Description
MCP7940 class.
Interface to the MCP7940 allowing you to get and set the time Example:
#include "mbed.h" #include "MCP7940.h" MCP7940 clock(PinName sda, PinName scl); int main() { clock.setTime(2015, 9, 26, 22, 50); clock.StartClock(); printf("%s \r\n" clock.TimeStamp()); //or clock.getTime(); printf("Day: %i, Month: %i, Year %i \r\n" clock.Day(), clock.Month(), clock.Year()); }
Definition at line 26 of file MCP7940.h.
Constructor & Destructor Documentation
MCP7940 | ( | PinName | sda, |
PinName | scl | ||
) |
Constructor Start year will default to 2000.
- Parameters:
-
sda I2C SDA pin scl I2C SCL pin
Definition at line 3 of file MCP7940.cpp.
MCP7940 | ( | PinName | sda, |
PinName | scl, | ||
int | StartYear | ||
) |
Constructor Start year will default to 2000.
- Parameters:
-
sda I2C SDA pin scl I2C SCL pin StarYear The start year for you calender. The MCP7940 defines years as XX, so that this library can give XXXX years you need to set your year 0, ie 2000
Definition at line 8 of file MCP7940.cpp.
Member Function Documentation
int Day | ( | ) |
Day Note getTime() must have been called to update the value of day.
- Returns:
- The day of the month
Definition at line 140 of file MCP7940.cpp.
int DayOfWeek | ( | ) |
DayOfWeek Note getTime() must have been called to update the value of day.
- Returns:
- The day of the week, a number 1-7. This is user defined
Definition at line 162 of file MCP7940.cpp.
int getTime | ( | ) |
getTime Loads the time from the MCP7940 so its upto date and can be accessed by the Day(), Month()...
functions
Definition at line 93 of file MCP7940.cpp.
int Hour | ( | ) |
Hour Note getTime() must have been called to update the value of day.
- Returns:
- the hour
Definition at line 149 of file MCP7940.cpp.
int MilliSeconds | ( | ) |
MilliSeconds Note getTime() must have been called to update the value of day.
Included for completeness - but MCP7940 doesn't have a mS counter
- Returns:
- Milliseconds = 0 for MCP7940
Definition at line 159 of file MCP7940.cpp.
int Minutes | ( | ) |
Minutes Note getTime() must have been called to update the value of day.
- Returns:
- Minutes
Definition at line 152 of file MCP7940.cpp.
int Month | ( | ) |
Month Note getTime() must have been called to update the value of day.
- Returns:
- The month.
Definition at line 143 of file MCP7940.cpp.
int Seconds | ( | ) |
Seconds Note getTime() must have been called to update the value of day.
- Returns:
- seconds
Definition at line 156 of file MCP7940.cpp.
int setTime | ( | int | Year, |
int | Month, | ||
int | Day, | ||
int | Hour, | ||
int | Mins, | ||
int | Secs, | ||
int | MiliSecs, | ||
int | DayOfWeek | ||
) |
SetTime Transfers the values of the time members into the MCP7940.
Use one of the other setTime functions
- Parameters:
-
Year the year in YYYY Month Day the Day of the month Hour Minutes Secs MiliSecs - note only for completeness and future extension as MCP7940 doesn't include mS DayOfWeek - the day of the week as a number 1-7, User defined
Definition at line 35 of file MCP7940.cpp.
int setTime | ( | int | Year, |
int | Month, | ||
int | Day, | ||
int | Hour, | ||
int | Mins, | ||
int | Secs | ||
) |
SetTime Transfers the values of the time members into the MCP7940.
Use one of the other setTime functions
- Parameters:
-
Year the year in YYYY Month Day the Day of the month Hour Minutes Secs
Definition at line 29 of file MCP7940.cpp.
int setTime | ( | ) |
SetTime Transfers the values of the time members into the MCP7940.
Use one of the other setTime functions
Definition at line 75 of file MCP7940.cpp.
int setTime | ( | int | Year, |
int | Month, | ||
int | Day, | ||
int | Hour, | ||
int | Mins | ||
) |
SetTime Transfers the values of the time members into the MCP7940.
Use one of the other setTime functions
- Parameters:
-
Year the year in YYYY Month Day the Day of the month Hour Minutes
Definition at line 26 of file MCP7940.cpp.
int setTime | ( | int | Year, |
int | Month, | ||
int | Day, | ||
int | Hour, | ||
int | Mins, | ||
int | Secs, | ||
int | MiliSecs | ||
) |
SetTime Transfers the values of the time members into the MCP7940.
Use one of the other setTime functions
- Parameters:
-
Year the year in YYYY Month Day the Day of the month Hour Minutes Secs MiliSecs - note only for completeness and future extension as MCP7940 doesn't include mS
Definition at line 32 of file MCP7940.cpp.
void StartClock | ( | ) |
StartClock Clears and sets bit 7 of register 0x00 to start the clock.
Keeps the current values of 0x00 bits 0-6
Definition at line 46 of file MCP7940.cpp.
char * TimeStamp | ( | ) |
TimeStamp getTime() is called within this function so does not need to be called from code.
- Returns:
- A pointer to a time stamp as YYYY-MM-DDTHH:MM:SS
Definition at line 119 of file MCP7940.cpp.
int Year | ( | ) |
Year Note getTime() must have been called to update the value of day.
- Returns:
- The Year as XXXX = StartYear + XX as in MCP7940
Definition at line 146 of file MCP7940.cpp.
Generated on Thu Jul 21 2022 08:18:33 by
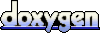