
Program to interface with mbed using RPC over Serial
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "mbed_rpc.h" 00003 00004 /** 00005 * This example program has been updated to use the RPC implementation in the new mbed libraries. 00006 * This example demonstrates using RPC over serial 00007 */ 00008 00009 //Use the RPC enabled wrapped class - see RpcClasses.h for more info 00010 RpcDigitalOut myled(LED4,"myled"); 00011 00012 Serial pc(USBTX, USBRX); 00013 int main() { 00014 //The mbed RPC classes are now wrapped to create an RPC enabled version - see RpcClasses.h so don't add to base class 00015 00016 // receive commands, and send back the responses 00017 char buf[256], outbuf[256]; 00018 while(1) { 00019 pc.gets(buf, 256); 00020 //Call the static call method on the RPC class 00021 RPC::call(buf, outbuf); 00022 pc.printf("%s\n", outbuf); 00023 } 00024 }
Generated on Tue Jul 12 2022 13:34:51 by
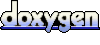