Library to provide a mechanism to make it easier to add RPC to custom code by using RPCFunction and RPCVariable objects. Also includes a class to receive and process RPC over serial.
Dependents: GSL_10-Pololu_A4983_STEPMOTORDRIVER Protodrive RPC_HTTP RPC_TestHack ... more
SerialRPCInterface.h
00001 /** 00002 * @section LICENSE 00003 *Copyright (c) 2010 ARM Ltd. 00004 * 00005 *Permission is hereby granted, free of charge, to any person obtaining a copy 00006 *of this software and associated documentation files (the "Software"), to deal 00007 *in the Software without restriction, including without limitation the rights 00008 *to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 *copies of the Software, and to permit persons to whom the Software is 00010 *furnished to do so, subject to the following conditions: 00011 * 00012 *The above copyright notice and this permission notice shall be included in 00013 *all copies or substantial portions of the Software. 00014 * 00015 *THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 *IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 *FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 *AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 *LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 *OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 *THE SOFTWARE. 00022 * 00023 * 00024 * @section DESCRIPTION 00025 * 00026 *This class sets up RPC communication over serial. 00027 */ 00028 #ifndef INTERFACE 00029 #define INTERFACE 00030 00031 /** 00032 *Includes 00033 */ 00034 #include "mbed.h" 00035 #include "platform.h" 00036 #include "rpc.h" 00037 #include "RpcClasses.h" 00038 #include "RPCFunction.h" 00039 #include "RPCVariable.h" 00040 00041 00042 namespace mbed{ 00043 /** 00044 *Provides an Interface to mbed over RPC. 00045 * 00046 *For the chosen communication type this class sets up the necessary interrupts to receive RPC messages. Receives the messages, passes them to the rpc function and then returns the result. 00047 */ 00048 class SerialRPCInterface{ 00049 public: 00050 /** 00051 *Constructor 00052 * 00053 *Sets up RPC communication using serial communication. 00054 * 00055 *@param tx The transmit pin of the serial port. 00056 *@param rx The receive pin of the serial port. 00057 *@param baud Set the baud rate, default is 9600. 00058 */ 00059 SerialRPCInterface(PinName tx, PinName rx, int baud = 9600); 00060 00061 /** 00062 *Disable the RPC. 00063 * 00064 *This will stop RPC messages being recevied and interpreted by this library. This might be used to prevent RPC commands interrupting an important piece of code on mbed. 00065 */ 00066 void Disable(void); 00067 00068 /** 00069 *Enable the RPC 00070 * 00071 *This will set this class to receiving and executing RPC commands. The class starts in this mode so this function only needs to be called if you have previosuly disabled the RPC. 00072 * 00073 */ 00074 void Enable(void); 00075 00076 //The Serial Port 00077 Serial pc; 00078 00079 00080 private: 00081 //Handle messgaes and take appropriate action 00082 void _MsgProcess(void); 00083 void _RegClasses(void); 00084 void _RPCSerial(); 00085 bool _enabled; 00086 char _command[256]; 00087 char _response[256]; 00088 bool _RPCflag; 00089 }; 00090 } 00091 #endif
Generated on Tue Jul 12 2022 13:34:52 by
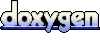