ESC class used to controll standard Electronic Speed Controllers for brushless motors of RC models
Dependents: MTQuadControl Base_Hybrid_V2 base_rekam_darurat_v1 Base_Hybrid_Latihan_Ok_Hajar_servo_pwm ... more
esc.cpp
00001 #include "mbed.h" 00002 #include "esc.h" 00003 00004 ESC::ESC(const PinName pwmPinOut, const int period) 00005 : esc(pwmPinOut), period(period), throttle(1000) 00006 { 00007 esc.period_ms(period); 00008 esc.pulsewidth_us(throttle); 00009 } 00010 00011 bool ESC::setThrottle (const float t) 00012 { 00013 if (t >= 0.0 && t <= 1.0) { // qualify range, 0-1 00014 throttle = 1000.0*t + 1000; // map to range, 1-2 ms (1000-2000us) 00015 return true; 00016 } 00017 return false; 00018 } 00019 bool ESC::operator= (const float t){ 00020 return this->setThrottle(t); 00021 } 00022 00023 float ESC::getThrottle () const{ 00024 return throttle; 00025 } 00026 ESC::operator float () const{ 00027 return this->getThrottle(); 00028 } 00029 00030 void ESC::pulse () 00031 { 00032 esc.pulsewidth_us(throttle); 00033 } 00034 void ESC::operator() () 00035 { 00036 this->pulse(); 00037 }
Generated on Wed Jul 13 2022 06:39:55 by
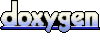