Simple interface for Mbed Cloud Client
Embed:
(wiki syntax)
Show/hide line numbers
common_utils.c
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // Licensed under the Apache License, Version 2.0 (the "License"); 00005 // you may not use this file except in compliance with the License. 00006 // You may obtain a copy of the License at 00007 // 00008 // http://www.apache.org/licenses/LICENSE-2.0 00009 // 00010 // Unless required by applicable law or agreed to in writing, software 00011 // distributed under the License is distributed on an "AS IS" BASIS, 00012 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 // See the License for the specific language governing permissions and 00014 // limitations under the License. 00015 // ---------------------------------------------------------------------------- 00016 00017 #include "factory_configurator_client.h" 00018 #include "fcc_status.h" 00019 #include "fcc_verification.h" 00020 #include "key_config_manager.h" 00021 #include "pv_error_handling.h" 00022 #include "cs_der_certs.h" 00023 #include "cs_utils.h" 00024 #include "fcc_output_info_handler.h" 00025 #include "fcc_malloc.h" 00026 #include "time.h" 00027 #include "cs_der_keys.h" 00028 #include "cs_utils.h" 00029 #include "fcc_sotp.h" 00030 00031 00032 fcc_status_e fcc_get_kcm_data(const uint8_t *parameter_name, size_t size_of_parameter_name, kcm_item_type_e kcm_type, uint8_t **kcm_data, size_t *kcm_data_size) 00033 { 00034 00035 kcm_status_e kcm_status = KCM_STATUS_SUCCESS; 00036 fcc_status_e fcc_status = FCC_STATUS_SUCCESS; 00037 00038 SA_PV_LOG_INFO_FUNC_ENTER_NO_ARGS(); 00039 SA_PV_ERR_RECOVERABLE_RETURN_IF((parameter_name == NULL), fcc_status = FCC_STATUS_INVALID_PARAMETER, "Wrong parameter_name pointer"); 00040 SA_PV_ERR_RECOVERABLE_RETURN_IF((size_of_parameter_name == 0), fcc_status = FCC_STATUS_INVALID_PARAMETER, "Wrong parameter_name size."); 00041 SA_PV_ERR_RECOVERABLE_RETURN_IF((*kcm_data != NULL), fcc_status = FCC_STATUS_INVALID_PARAMETER, "Wrong *kcm_data pointer, should be NULL"); 00042 SA_PV_ERR_RECOVERABLE_RETURN_IF((kcm_data_size == NULL), fcc_status = FCC_STATUS_INVALID_PARAMETER, "Wrong kcm_data_size pointer."); 00043 00044 //Get size of kcm data 00045 kcm_status = kcm_item_get_data_size(parameter_name, 00046 size_of_parameter_name, 00047 kcm_type, 00048 kcm_data_size); 00049 if (kcm_status == KCM_STATUS_ITEM_NOT_FOUND) { 00050 return FCC_STATUS_ITEM_NOT_EXIST; 00051 } 00052 SA_PV_ERR_RECOVERABLE_RETURN_IF((kcm_status != KCM_STATUS_SUCCESS), fcc_status = FCC_STATUS_KCM_STORAGE_ERROR, "Failed to get kcm data size"); 00053 SA_PV_ERR_RECOVERABLE_RETURN_IF((*kcm_data_size == 0), fcc_status = FCC_STATUS_EMPTY_ITEM, "KCM item is empty"); 00054 00055 //Alocate memory and get device certificate data 00056 *kcm_data = fcc_malloc(*kcm_data_size); 00057 SA_PV_ERR_RECOVERABLE_RETURN_IF((*kcm_data == NULL), fcc_status = FCC_STATUS_MEMORY_OUT, "Failed to allocate buffer for kcm data"); 00058 00059 kcm_status = kcm_item_get_data(parameter_name, 00060 size_of_parameter_name, 00061 kcm_type, 00062 *kcm_data, *kcm_data_size, kcm_data_size); 00063 SA_PV_ERR_RECOVERABLE_GOTO_IF((kcm_status == KCM_STATUS_ITEM_NOT_FOUND), fcc_status = FCC_STATUS_ITEM_NOT_EXIST, exit, "KCM is not found"); 00064 SA_PV_ERR_RECOVERABLE_GOTO_IF((kcm_status != KCM_STATUS_SUCCESS), fcc_status = FCC_STATUS_KCM_STORAGE_ERROR, exit, "Failed to get device certificate data"); 00065 00066 exit: 00067 if (fcc_status != FCC_STATUS_SUCCESS) { 00068 fcc_free(*kcm_data); 00069 *kcm_data = NULL; 00070 } 00071 SA_PV_LOG_TRACE_FUNC_EXIT_NO_ARGS(); 00072 return fcc_status; 00073 } 00074 00075 fcc_status_e fcc_get_certificate_attribute(palX509Handle_t x509_cert, cs_certificate_attribute_type_e attribute_type, uint8_t **attribute_data, size_t *attribute_act_data_size) 00076 { 00077 00078 kcm_status_e kcm_status = KCM_STATUS_SUCCESS; 00079 fcc_status_e fcc_status = FCC_STATUS_SUCCESS; 00080 00081 SA_PV_LOG_INFO_FUNC_ENTER_NO_ARGS(); 00082 00083 SA_PV_ERR_RECOVERABLE_RETURN_IF((x509_cert == NULLPTR), fcc_status = FCC_STATUS_INVALID_PARAMETER, "Wrong x509 handle."); 00084 SA_PV_ERR_RECOVERABLE_RETURN_IF((*attribute_data != NULL), fcc_status = FCC_STATUS_INVALID_PARAMETER, "Wrong attribute_data pointer."); 00085 SA_PV_ERR_RECOVERABLE_RETURN_IF((attribute_act_data_size == NULL), fcc_status = FCC_STATUS_INVALID_PARAMETER, "attribute_act_data_size pointer is NULL."); 00086 00087 //Get attribute size 00088 kcm_status = cs_attr_get_data_size_x509_cert(x509_cert, attribute_type, attribute_act_data_size); 00089 SA_PV_ERR_RECOVERABLE_RETURN_IF((kcm_status != KCM_STATUS_SUCCESS), fcc_status = FCC_STATUS_INVALID_CERT_ATTRIBUTE, "Failed to get size of attribute"); 00090 00091 *attribute_data = fcc_malloc(*attribute_act_data_size); 00092 SA_PV_ERR_RECOVERABLE_RETURN_IF((*attribute_data == NULL), fcc_status = FCC_STATUS_MEMORY_OUT, "Failed to allocate memory for attribute"); 00093 00094 //Get data of "CN" attribute 00095 kcm_status = cs_attr_get_data_x509_cert(x509_cert, 00096 attribute_type, 00097 *attribute_data, 00098 *attribute_act_data_size, 00099 attribute_act_data_size); 00100 SA_PV_ERR_RECOVERABLE_GOTO_IF((kcm_status != KCM_STATUS_SUCCESS || *attribute_act_data_size == 0), fcc_status = FCC_STATUS_INVALID_CERT_ATTRIBUTE, exit, "Failed to get attribute data"); 00101 00102 00103 exit: 00104 if (fcc_status != FCC_STATUS_SUCCESS) { 00105 fcc_free(*attribute_data); 00106 *attribute_data = NULL; 00107 } 00108 SA_PV_LOG_TRACE_FUNC_EXIT_NO_ARGS(); 00109 return fcc_status; 00110 } 00111 00112 fcc_status_e fcc_get_certificate_attribute_by_name(const uint8_t *cert_name, size_t size_of_cert_name, cs_certificate_attribute_type_e attribute_type, uint8_t *attribute_data,size_t attribute_data_size, size_t *attribute_act_data_size) 00113 { 00114 kcm_status_e kcm_status = KCM_STATUS_SUCCESS; 00115 fcc_status_e fcc_status = FCC_STATUS_SUCCESS; 00116 uint8_t *kcm_data = NULL; 00117 size_t kcm_data_size = 0; 00118 palX509Handle_t x509_cert = NULLPTR; 00119 00120 SA_PV_LOG_INFO_FUNC_ENTER_NO_ARGS(); 00121 00122 SA_PV_ERR_RECOVERABLE_GOTO_IF((cert_name == NULL), fcc_status = FCC_STATUS_INVALID_PARAMETER, exit, "Wrong cert name"); 00123 SA_PV_ERR_RECOVERABLE_GOTO_IF((size_of_cert_name == 0), fcc_status = FCC_STATUS_INVALID_PARAMETER, exit, "Wrong cert name size"); 00124 SA_PV_ERR_RECOVERABLE_GOTO_IF((attribute_data == NULL), fcc_status = FCC_STATUS_INVALID_PARAMETER, exit, "Wrong attribute data buffer pointer"); 00125 SA_PV_ERR_RECOVERABLE_GOTO_IF((attribute_data_size == 0), fcc_status = FCC_STATUS_INVALID_PARAMETER, exit, "Wrong attribute data buffer size"); 00126 SA_PV_ERR_RECOVERABLE_GOTO_IF((attribute_act_data_size == NULL), fcc_status = FCC_STATUS_INVALID_PARAMETER, exit, "Wrong attribute_act_data_size pointer"); 00127 00128 //For now we save ca id only for bootstrap server 00129 fcc_status = fcc_get_kcm_data((const uint8_t*)cert_name, size_of_cert_name, KCM_CERTIFICATE_ITEM, &kcm_data, &kcm_data_size); 00130 SA_PV_ERR_RECOVERABLE_GOTO_IF((fcc_status != FCC_STATUS_SUCCESS), fcc_status = fcc_status, exit, "Failed to read cert data"); 00131 00132 //Create device certificate handle 00133 kcm_status = cs_create_handle_from_der_x509_cert(kcm_data, kcm_data_size, &x509_cert); 00134 SA_PV_ERR_RECOVERABLE_GOTO_IF((kcm_status != KCM_STATUS_SUCCESS), fcc_status = FCC_STATUS_INVALID_CERTIFICATE, exit, "Failed to get device certificate descriptor"); 00135 00136 //Get certificate attribute data 00137 kcm_status = cs_attr_get_data_x509_cert(x509_cert, 00138 attribute_type, 00139 attribute_data, 00140 attribute_data_size, 00141 attribute_act_data_size); 00142 SA_PV_ERR_RECOVERABLE_GOTO_IF((kcm_status != KCM_STATUS_SUCCESS), fcc_status = FCC_STATUS_INVALID_CERT_ATTRIBUTE, exit, "Failed to get attribute data"); 00143 00144 00145 exit: 00146 fcc_free(kcm_data); 00147 cs_close_handle_x509_cert(&x509_cert); 00148 SA_PV_LOG_TRACE_FUNC_EXIT_NO_ARGS(); 00149 return fcc_status; 00150 }
Generated on Tue Jul 12 2022 19:01:33 by
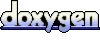