Simple interface for Mbed Cloud Client
FtcdCommSocket Class Reference
FtcdCommSocket implements the logic of listening for TCP connections and process incoming messages from the Factory Tool. More...
#include <ftcd_comm_socket.h>
Inherits FtcdCommBase.
Public Member Functions | |
FtcdCommSocket (const void *interfaceHandler, ftcd_socket_domain_e domain, const uint16_t port_num, ftcd_comm_network_endianness_e network_endianness=FTCD_COMM_NET_ENDIANNESS_BIG, int32_t timeout=INFINITE_SOCKET_TIMEOUT) | |
The Socket Constructor Initializes private variables and sets network interface handler, IP and port number. | |
FtcdCommSocket (const void *interfaceHandler, ftcd_socket_domain_e domain, const uint16_t port_num, ftcd_comm_network_endianness_e network_endianness, const uint8_t *header_token, bool use_signature, int32_t timeout=INFINITE_SOCKET_TIMEOUT) | |
The Socket Constructor Initializes private variables and sets network interface handler, IP and port number. | |
virtual | ~FtcdCommSocket () |
The Socket Destructor Closes opened resources and frees allocated memory. | |
virtual bool | init (void) |
Initializes Network interface and prints its address. | |
virtual void | finish (void) |
Closes opened sockets. | |
virtual ftcd_comm_status_e | wait_for_message (uint8_t **message_out, uint32_t *message_size_out) |
Wait and read complete message from the communication line. | |
virtual ftcd_comm_status_e | is_token_detected (void) |
Detects the message token from the communication line medium. | |
virtual uint32_t | read_message_size (void) |
Reads the message size in bytes from the communication line medium. | |
virtual bool | read_message (uint8_t *message_out, size_t message_size) |
Reads the message size in bytes from the communication line medium. | |
virtual bool | read_message_signature (uint8_t *sig, size_t sig_size) |
Reads the message size in bytes from the communication line medium. | |
virtual bool | send (const uint8_t *data, uint32_t data_size) |
Writes the given data to the communication line medium. | |
ftcd_comm_status_e | send_response (const uint8_t *response_message, uint32_t response_message_size) |
Writes a response message to the communication line. | |
ftcd_comm_status_e | send_response (const uint8_t *response_message, uint32_t response_message_size, ftcd_comm_status_e status_code) |
Writes a response message with status to the communication line. |
Detailed Description
FtcdCommSocket implements the logic of listening for TCP connections and process incoming messages from the Factory Tool.
Definition at line 50 of file ftcd_comm_socket.h.
Constructor & Destructor Documentation
FtcdCommSocket | ( | const void * | interfaceHandler, |
ftcd_socket_domain_e | domain, | ||
const uint16_t | port_num, | ||
ftcd_comm_network_endianness_e | network_endianness = FTCD_COMM_NET_ENDIANNESS_BIG , |
||
int32_t | timeout = INFINITE_SOCKET_TIMEOUT |
||
) |
The Socket Constructor Initializes private variables and sets network interface handler, IP and port number.
If port_num is 0, then random port will be generated.
Definition at line 35 of file ftcd_comm_socket.cpp.
FtcdCommSocket | ( | const void * | interfaceHandler, |
ftcd_socket_domain_e | domain, | ||
const uint16_t | port_num, | ||
ftcd_comm_network_endianness_e | network_endianness, | ||
const uint8_t * | header_token, | ||
bool | use_signature, | ||
int32_t | timeout = INFINITE_SOCKET_TIMEOUT |
||
) |
The Socket Constructor Initializes private variables and sets network interface handler, IP and port number.
If port_num is 0, then random port will be generated.
Definition at line 49 of file ftcd_comm_socket.cpp.
~FtcdCommSocket | ( | ) | [virtual] |
The Socket Destructor Closes opened resources and frees allocated memory.
Definition at line 64 of file ftcd_comm_socket.cpp.
Member Function Documentation
void finish | ( | void | ) | [virtual] |
Closes opened sockets.
Reimplemented from FtcdCommBase.
Definition at line 168 of file ftcd_comm_socket.cpp.
bool init | ( | void | ) | [virtual] |
Initializes Network interface and prints its address.
Reimplemented from FtcdCommBase.
Definition at line 79 of file ftcd_comm_socket.cpp.
ftcd_comm_status_e is_token_detected | ( | void | ) | [virtual] |
Detects the message token from the communication line medium.
- Returns:
- true, if token detected and false otherwise
Implements FtcdCommBase.
Definition at line 199 of file ftcd_comm_socket.cpp.
bool read_message | ( | uint8_t * | message_out, |
size_t | message_size | ||
) | [virtual] |
Reads the message size in bytes from the communication line medium.
This is the amount of bytes needed to allocate for the upcoming message bytes.
- Parameters:
-
message_out The buffer to read into and return to the caller. message_size The message size in bytes.
- Returns:
- true upon success, false otherwise
Implements FtcdCommBase.
Definition at line 238 of file ftcd_comm_socket.cpp.
bool read_message_signature | ( | uint8_t * | sig, |
size_t | sig_size | ||
) | [virtual] |
Reads the message size in bytes from the communication line medium.
This is the amount of bytes needed to allocate for the upcoming message bytes.
- Parameters:
-
sig The buffer to read into and return to the caller. sig_size The sig buffer size in bytes.
- Returns:
- The message size in bytes in case of success, zero bytes otherwise.
Implements FtcdCommBase.
Definition at line 258 of file ftcd_comm_socket.cpp.
uint32_t read_message_size | ( | void | ) | [virtual] |
Reads the message size in bytes from the communication line medium.
This is the amount of bytes needed to allocate for the upcoming message bytes.
- Returns:
- The message size in bytes in case of success, zero bytes otherwise.
Implements FtcdCommBase.
Definition at line 224 of file ftcd_comm_socket.cpp.
bool send | ( | const uint8_t * | data, |
uint32_t | data_size | ||
) | [virtual] |
Writes the given data to the communication line medium.
- Parameters:
-
data The bytes to send through the communication line medium data_size The data size in bytes
- Returns:
- true upon success, false otherwise
Implements FtcdCommBase.
Definition at line 278 of file ftcd_comm_socket.cpp.
ftcd_comm_status_e send_response | ( | const uint8_t * | response_message, |
uint32_t | response_message_size | ||
) | [inherited] |
Writes a response message to the communication line.
The method build response message with header and signature (if requested) and writes it to the line
- Parameters:
-
response_message The message to send through the communication line medium response_message_size The message size in bytes
- Returns:
- FTCD_COMM_STATUS_SUCCESS on success, otherwise appropriate error from ftcd_comm_status_e
Definition at line 153 of file ftcd_comm_base.cpp.
ftcd_comm_status_e send_response | ( | const uint8_t * | response_message, |
uint32_t | response_message_size, | ||
ftcd_comm_status_e | status_code | ||
) | [inherited] |
Writes a response message with status to the communication line.
The method build response message with status, header and signature (if requested) and writes it to the line
- Parameters:
-
response_message The message to send through the communication line medium response_message_size The message size in bytes
- Returns:
- FTCD_COMM_STATUS_SUCCESS on success, otherwise appropriate error from ftcd_comm_status_e
Definition at line 158 of file ftcd_comm_base.cpp.
ftcd_comm_status_e wait_for_message | ( | uint8_t ** | message_out, |
uint32_t * | message_size_out | ||
) | [virtual] |
Wait and read complete message from the communication line.
The method waits in blocking mode for new message, allocate and read the message, and sets message_out and message_size_out
- Parameters:
-
message_out The message allocated and read from the communication line message_size_out The message size in bytes
- Returns:
- FTCD_COMM_STATUS_SUCCESS on success, otherwise appropriate error from ftcd_comm_status_e
Reimplemented from FtcdCommBase.
Definition at line 181 of file ftcd_comm_socket.cpp.
Generated on Tue Jul 12 2022 19:01:39 by
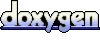