
Mbed Cloud example program for workshop in W27 2018.
cn-cbor.h File Reference
CBOR parsing. More...
Go to the source code of this file.
Data Structures | |
struct | cn_cbor |
A CBOR value. More... | |
struct | cn_cbor_errback |
Errors. More... | |
struct | cn_cbor_context |
The allocation context. More... | |
Typedefs | |
typedef enum cn_cbor_type | cn_cbor_type |
All of the different kinds of CBOR values. | |
typedef enum cn_cbor_flags | cn_cbor_flags |
Flags used during parsing. | |
typedef struct cn_cbor | cn_cbor |
A CBOR value. | |
typedef enum cn_cbor_error | cn_cbor_error |
All of the different kinds of errors. | |
typedef struct cn_cbor_errback | cn_cbor_errback |
Errors. | |
typedef void *(* | cn_calloc_func )(size_t count, size_t size, void *context) |
Allocate and zero out memory. | |
typedef void(* | cn_free_func )(void *ptr, void *context) |
Free memory previously allocated with a context. | |
typedef struct cn_cbor_context | cn_cbor_context |
The allocation context. | |
Enumerations | |
enum | cn_cbor_type { CN_CBOR_FALSE, CN_CBOR_TRUE, CN_CBOR_NULL, CN_CBOR_UNDEF, CN_CBOR_UINT, CN_CBOR_INT, CN_CBOR_BYTES, CN_CBOR_TEXT, CN_CBOR_BYTES_CHUNKED, CN_CBOR_TEXT_CHUNKED, CN_CBOR_ARRAY, CN_CBOR_MAP, CN_CBOR_TAG, CN_CBOR_SIMPLE, CN_CBOR_DOUBLE, CN_CBOR_INVALID } |
All of the different kinds of CBOR values. More... | |
enum | cn_cbor_flags { CN_CBOR_FL_COUNT = 1, CN_CBOR_FL_INDEF = 2, CN_CBOR_FL_OWNER = 0x80 } |
Flags used during parsing. More... | |
enum | cn_cbor_error { CN_CBOR_NO_ERROR, CN_CBOR_ERR_OUT_OF_DATA, CN_CBOR_ERR_NOT_ALL_DATA_CONSUMED, CN_CBOR_ERR_ODD_SIZE_INDEF_MAP, CN_CBOR_ERR_BREAK_OUTSIDE_INDEF, CN_CBOR_ERR_MT_UNDEF_FOR_INDEF, CN_CBOR_ERR_RESERVED_AI, CN_CBOR_ERR_WRONG_NESTING_IN_INDEF_STRING, CN_CBOR_ERR_INVALID_PARAMETER, CN_CBOR_ERR_OUT_OF_MEMORY, CN_CBOR_ERR_FLOAT_NOT_SUPPORTED, CN_CBOR_ERR_ENCODER } |
All of the different kinds of errors. More... | |
Functions | |
cn_cbor * | cn_cbor_decode (const uint8_t *buf, size_t len CBOR_CONTEXT, cn_cbor_errback *errp) |
Decode an array of CBOR bytes into structures. | |
cn_cbor * | cn_cbor_mapget_string (const cn_cbor *cb, const char *key) |
Get a value from a CBOR map that has the given string as a key. | |
cn_cbor * | cn_cbor_mapget_int (const cn_cbor *cb, int key) |
Get a value from a CBOR map that has the given integer as a key. | |
cn_cbor * | cn_cbor_index (const cn_cbor *cb, unsigned int idx) |
Get the item with the given index from a CBOR array. | |
void | cn_cbor_free (cn_cbor *cb CBOR_CONTEXT) |
Free the given CBOR structure. | |
int | cn_cbor_get_encoded_size (const cn_cbor *cb, cn_cbor_errback *err) |
Get the size of the buffer that must be provided to cn_cbor_encoder_write(). | |
int | cn_cbor_encoder_write (const cn_cbor *cb, uint8_t *bufOut, int bufSize, cn_cbor_errback *err) |
Write a CBOR value and all of the child values. | |
cn_cbor * | cn_cbor_map_create (CBOR_CONTEXT_COMMA cn_cbor_errback *errp) |
Create a CBOR map. | |
cn_cbor * | cn_cbor_data_create (const uint8_t *data, int len CBOR_CONTEXT, cn_cbor_errback *errp) |
Create a CBOR byte string. | |
cn_cbor * | cn_cbor_string_create (const char *data CBOR_CONTEXT, cn_cbor_errback *errp) |
Create a CBOR UTF-8 string. | |
cn_cbor * | cn_cbor_int_create (int64_t value CBOR_CONTEXT, cn_cbor_errback *errp) |
Create a CBOR integer (either positive or negative). | |
bool | cn_cbor_map_put (cn_cbor *cb_map, cn_cbor *cb_key, cn_cbor *cb_value, cn_cbor_errback *errp) |
Put a CBOR object into a map with a CBOR object key. | |
bool | cn_cbor_mapput_int (cn_cbor *cb_map, int64_t key, cn_cbor *cb_value CBOR_CONTEXT, cn_cbor_errback *errp) |
Put a CBOR object into a map with an integer key. | |
bool | cn_cbor_mapput_string (cn_cbor *cb_map, const char *key, cn_cbor *cb_value CBOR_CONTEXT, cn_cbor_errback *errp) |
Put a CBOR object into a map with a string key. | |
cn_cbor * | cn_cbor_array_create (CBOR_CONTEXT_COMMA cn_cbor_errback *errp) |
Create a CBOR array. | |
bool | cn_cbor_array_append (cn_cbor *cb_array, cn_cbor *cb_value, cn_cbor_errback *errp) |
Append an item to the end of a CBOR array. | |
Variables | |
const char * | cn_cbor_error_str [] |
Strings matching the `cn_cbor_error` conditions. |
Detailed Description
CBOR parsing.
Definition in file cn-cbor.h.
Typedef Documentation
typedef void*(* cn_calloc_func)(size_t count, size_t size, void *context) |
Allocate and zero out memory.
`count` elements of `size` are required, as for `calloc(3)`. The `context` is the `cn_cbor_context` passed in earlier to the CBOR routine.
- Parameters:
-
[in] count The number of items to allocate [in] size The size of each item [in] context The allocation context
typedef struct cn_cbor_context cn_cbor_context |
The allocation context.
typedef struct cn_cbor_errback cn_cbor_errback |
Errors.
typedef enum cn_cbor_error cn_cbor_error |
All of the different kinds of errors.
typedef enum cn_cbor_flags cn_cbor_flags |
Flags used during parsing.
Not useful for consumers of the `cn_cbor` structure.
typedef enum cn_cbor_type cn_cbor_type |
All of the different kinds of CBOR values.
typedef void(* cn_free_func)(void *ptr, void *context) |
Free memory previously allocated with a context.
If using a pool allocator, this function will often be a no-op, but it must be supplied in order to prevent the CBOR library from calling `free(3)`.
- Note:
- : it may be that this is never needed; if so, it will be removed for clarity and speed.
- Parameters:
-
context [description]
- Returns:
- [description]
Enumeration Type Documentation
enum cn_cbor_error |
All of the different kinds of errors.
- Enumerator:
CN_CBOR_NO_ERROR No error has occurred.
CN_CBOR_ERR_OUT_OF_DATA More data was expected while parsing.
CN_CBOR_ERR_NOT_ALL_DATA_CONSUMED Some extra data was left over at the end of parsing.
CN_CBOR_ERR_ODD_SIZE_INDEF_MAP A map should be alternating keys and values.
A break was found when a value was expected
CN_CBOR_ERR_BREAK_OUTSIDE_INDEF A break was found where it wasn't expected.
CN_CBOR_ERR_MT_UNDEF_FOR_INDEF Indefinite encoding works for bstrs, strings, arrays, and maps.
A different major type tried to use it.
CN_CBOR_ERR_RESERVED_AI Additional Information values 28-30 are reserved.
CN_CBOR_ERR_WRONG_NESTING_IN_INDEF_STRING A chunked encoding was used for a string or bstr, and one of the elements wasn't the expected (string/bstr) type.
CN_CBOR_ERR_INVALID_PARAMETER An invalid parameter was passed to a function.
CN_CBOR_ERR_OUT_OF_MEMORY Allocation failed.
CN_CBOR_ERR_FLOAT_NOT_SUPPORTED A float was encountered during parse but the library was built without support for float types.
CN_CBOR_ERR_ENCODER Encoder was unable to encode the cn_cbor object that was provided.
enum cn_cbor_flags |
Flags used during parsing.
Not useful for consumers of the `cn_cbor` structure.
enum cn_cbor_type |
All of the different kinds of CBOR values.
- Enumerator:
Function Documentation
bool cn_cbor_array_append | ( | cn_cbor * | cb_array, |
cn_cbor * | cb_value, | ||
cn_cbor_errback * | errp | ||
) |
Append an item to the end of a CBOR array.
- Parameters:
-
[in] cb_array The array into which to insert [in] cb_value The value to insert [out] errp Error
- Returns:
- True on success
Definition at line 157 of file cn-create.c.
cn_cbor* cn_cbor_array_create | ( | CBOR_CONTEXT_COMMA cn_cbor_errback * | errp ) |
Create a CBOR array.
- Parameters:
-
[in] CBOR_CONTEXT Allocation context (only if USE_CBOR_CONTEXT is defined) [out] errp Error, if NULL is returned
- Returns:
- The created object, or NULL on error
Definition at line 146 of file cn-create.c.
cn_cbor* cn_cbor_data_create | ( | const uint8_t * | data, |
int len | CBOR_CONTEXT, | ||
cn_cbor_errback * | errp | ||
) |
Create a CBOR byte string.
The data in the byte string is *not* owned by the CBOR object, so it is not freed automatically.
- Parameters:
-
[in] data The data [in] len The number of bytes of data [in] CBOR_CONTEXT Allocation context (only if USE_CBOR_CONTEXT is defined) [out] errp Error, if NULL is returned
- Returns:
- The created object, or NULL on error
Definition at line 30 of file cn-create.c.
cn_cbor* cn_cbor_decode | ( | const uint8_t * | buf, |
size_t len | CBOR_CONTEXT, | ||
cn_cbor_errback * | errp | ||
) |
Decode an array of CBOR bytes into structures.
- Parameters:
-
[in] buf The array of bytes to parse [in] len The number of bytes in the array [in] CBOR_CONTEXT Allocation context (only if USE_CBOR_CONTEXT is defined) [out] errp Error, if NULL is returned
- Returns:
- The parsed CBOR structure, or NULL on error
int cn_cbor_encoder_write | ( | const cn_cbor * | cb, |
uint8_t * | bufOut, | ||
int | bufSize, | ||
cn_cbor_errback * | err | ||
) |
Write a CBOR value and all of the child values.
Allocates a buffer of the correct size and fills it with the encoded CBOR. User must free the buffer.
- Parameters:
-
[in] cb Pointer to a cn_cbor structure, which the user wishes to encode. [out] bufOut Pointer a buffer which will be filled with the encoded data. [in] bufSize Size of the provided buffer in bytes. [out] err Error, if -1 is returned.
- Returns:
- -1 on fail, or number encoded bytes written to the provided buffer.
Definition at line 380 of file cn-encoder.c.
void cn_cbor_free | ( | cn_cbor *cb | CBOR_CONTEXT ) |
Free the given CBOR structure.
You MUST NOT try to free a cn_cbor structure with a parent (i.e., one that is not a root in the tree).
- Parameters:
-
[in] cb The CBOR value to free. May be NULL, or a root object. [in] CBOR_CONTEXT Allocation context (only if USE_CBOR_CONTEXT is defined)
int cn_cbor_get_encoded_size | ( | const cn_cbor * | cb, |
cn_cbor_errback * | err | ||
) |
Get the size of the buffer that must be provided to cn_cbor_encoder_write().
This is the size of the CBOR that will be encoded.
- Parameters:
-
[in] cb Pointer to a cn_cbor structure, which the user wishes to encode. [out] err Error, if -1 is returned.
- Returns:
- -1 on fail, or number size of the allocated buffer containing the encoded data.
Definition at line 361 of file cn-encoder.c.
cn_cbor* cn_cbor_int_create | ( | int64_t value | CBOR_CONTEXT, |
cn_cbor_errback * | errp | ||
) |
Create a CBOR integer (either positive or negative).
- Parameters:
-
[in] value the value of the integer [in] CBOR_CONTEXT Allocation context (only if USE_CBOR_CONTEXT is defined) [out] errp Error, if NULL is returned
- Returns:
- The created object, or NULL on error
Definition at line 58 of file cn-create.c.
cn_cbor* cn_cbor_map_create | ( | CBOR_CONTEXT_COMMA cn_cbor_errback * | errp ) |
Create a CBOR map.
- Parameters:
-
[in] CBOR_CONTEXT Allocation context (only if USE_CBOR_CONTEXT is defined) [out] errp Error, if NULL is returned
- Returns:
- The created map, or NULL on error
Definition at line 19 of file cn-create.c.
bool cn_cbor_map_put | ( | cn_cbor * | cb_map, |
cn_cbor * | cb_key, | ||
cn_cbor * | cb_value, | ||
cn_cbor_errback * | errp | ||
) |
Put a CBOR object into a map with a CBOR object key.
Duplicate checks are NOT currently performed.
- Parameters:
-
[in] cb_map The map to insert into [in] key The key [in] cb_value The value [out] errp Error
- Returns:
- True on success
Definition at line 94 of file cn-create.c.
bool cn_cbor_mapput_int | ( | cn_cbor * | cb_map, |
int64_t | key, | ||
cn_cbor *cb_value | CBOR_CONTEXT, | ||
cn_cbor_errback * | errp | ||
) |
Put a CBOR object into a map with an integer key.
Duplicate checks are NOT currently performed.
- Parameters:
-
[in] cb_map The map to insert into [in] key The integer key [in] cb_value The value [in] CBOR_CONTEXT Allocation context (only if USE_CBOR_CONTEXT is defined) [out] errp Error
- Returns:
- True on success
Definition at line 108 of file cn-create.c.
bool cn_cbor_mapput_string | ( | cn_cbor * | cb_map, |
const char * | key, | ||
cn_cbor *cb_value | CBOR_CONTEXT, | ||
cn_cbor_errback * | errp | ||
) |
Put a CBOR object into a map with a string key.
Duplicate checks are NOT currently performed.
- Note:
- : do not call this routine with untrusted string data. It calls strlen, and requires a properly NULL-terminated key.
- Parameters:
-
[in] cb_map The map to insert into [in] key The string key [in] cb_value The value [in] CBOR_CONTEXT Allocation context (only if USE_CBOR_CONTEXT is defined) [out] errp Error
- Returns:
- True on success
Definition at line 127 of file cn-create.c.
cn_cbor* cn_cbor_string_create | ( | const char *data | CBOR_CONTEXT, |
cn_cbor_errback * | errp | ||
) |
Create a CBOR UTF-8 string.
The data is not checked for UTF-8 correctness. The data being stored in the string is *not* owned the CBOR object, so it is not freed automatically.
- Note:
- : Do NOT use this function with untrusted data. It calls strlen, and relies on proper NULL-termination.
- Parameters:
-
[in] data NULL-terminated UTF-8 string [in] CBOR_CONTEXT Allocation context (only if USE_CBOR_CONTEXT is defined) [out] errp Error, if NULL is returned
- Returns:
- The created object, or NULL on error
Definition at line 44 of file cn-create.c.
Variable Documentation
const char* cn_cbor_error_str[] |
Strings matching the `cn_cbor_error` conditions.
Definition at line 1 of file cn-error.c.
Generated on Tue Jul 12 2022 16:22:13 by
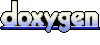