
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
cn-create.c
00001 #ifndef CN_CREATE_C 00002 #define CN_CREATE_C 00003 00004 #ifdef __cplusplus 00005 extern "C" { 00006 #endif 00007 00008 #include <string.h> 00009 #include <stdlib.h> 00010 00011 #include "cn-cbor.h" 00012 #include "cbor.h" 00013 00014 #define INIT_CB(v) \ 00015 if (errp) {errp->err = CN_CBOR_NO_ERROR;} \ 00016 (v) = CN_CALLOC_CONTEXT(); \ 00017 if (!(v)) { if (errp) {errp->err = CN_CBOR_ERR_OUT_OF_MEMORY;} return NULL; } 00018 00019 cn_cbor* cn_cbor_map_create(CBOR_CONTEXT_COMMA cn_cbor_errback *errp) 00020 { 00021 cn_cbor* ret; 00022 INIT_CB(ret); 00023 00024 ret->type = CN_CBOR_MAP; 00025 ret->flags |= CN_CBOR_FL_COUNT; 00026 00027 return ret; 00028 } 00029 00030 cn_cbor* cn_cbor_data_create(const uint8_t* data, int len 00031 CBOR_CONTEXT, 00032 cn_cbor_errback *errp) 00033 { 00034 cn_cbor* ret; 00035 INIT_CB(ret); 00036 00037 ret->type = CN_CBOR_BYTES; 00038 ret->length = len; 00039 ret->v.str = (const char*) data; // TODO: add v.ustr to the union? 00040 00041 return ret; 00042 } 00043 00044 cn_cbor* cn_cbor_string_create(const char* data 00045 CBOR_CONTEXT, 00046 cn_cbor_errback *errp) 00047 { 00048 cn_cbor* ret; 00049 INIT_CB(ret); 00050 00051 ret->type = CN_CBOR_TEXT; 00052 ret->length = strlen(data); 00053 ret->v.str = data; 00054 00055 return ret; 00056 } 00057 00058 cn_cbor* cn_cbor_int_create(int64_t value 00059 CBOR_CONTEXT, 00060 cn_cbor_errback *errp) 00061 { 00062 cn_cbor* ret; 00063 INIT_CB(ret); 00064 00065 if (value<0) { 00066 ret->type = CN_CBOR_INT; 00067 ret->v.sint = value; 00068 } else { 00069 ret->type = CN_CBOR_UINT; 00070 ret->v.uint = value; 00071 } 00072 00073 return ret; 00074 } 00075 00076 static bool _append_kv(cn_cbor *cb_map, cn_cbor *key, cn_cbor *val) 00077 { 00078 //Connect key and value and insert them into the map. 00079 key->parent = cb_map; 00080 key->next = val; 00081 val->parent = cb_map; 00082 val->next = NULL; 00083 00084 if(cb_map->last_child) { 00085 cb_map->last_child->next = key; 00086 } else { 00087 cb_map->first_child = key; 00088 } 00089 cb_map->last_child = val; 00090 cb_map->length += 2; 00091 return true; 00092 } 00093 00094 bool cn_cbor_map_put(cn_cbor* cb_map, 00095 cn_cbor *cb_key, cn_cbor *cb_value, 00096 cn_cbor_errback *errp) 00097 { 00098 //Make sure input is a map. Otherwise 00099 if(!cb_map || !cb_key || !cb_value || cb_map->type != CN_CBOR_MAP) 00100 { 00101 if (errp) {errp->err = CN_CBOR_ERR_INVALID_PARAMETER;} 00102 return false; 00103 } 00104 00105 return _append_kv(cb_map, cb_key, cb_value); 00106 } 00107 00108 bool cn_cbor_mapput_int(cn_cbor* cb_map, 00109 int64_t key, cn_cbor* cb_value 00110 CBOR_CONTEXT, 00111 cn_cbor_errback *errp) 00112 { 00113 cn_cbor* cb_key; 00114 00115 //Make sure input is a map. Otherwise 00116 if(!cb_map || !cb_value || cb_map->type != CN_CBOR_MAP) 00117 { 00118 if (errp) {errp->err = CN_CBOR_ERR_INVALID_PARAMETER;} 00119 return false; 00120 } 00121 00122 cb_key = cn_cbor_int_create(key CBOR_CONTEXT_PARAM, errp); 00123 if (!cb_key) { return false; } 00124 return _append_kv(cb_map, cb_key, cb_value); 00125 } 00126 00127 bool cn_cbor_mapput_string(cn_cbor* cb_map, 00128 const char* key, cn_cbor* cb_value 00129 CBOR_CONTEXT, 00130 cn_cbor_errback *errp) 00131 { 00132 cn_cbor* cb_key; 00133 00134 //Make sure input is a map. Otherwise 00135 if(!cb_map || !cb_value || cb_map->type != CN_CBOR_MAP) 00136 { 00137 if (errp) {errp->err = CN_CBOR_ERR_INVALID_PARAMETER;} 00138 return false; 00139 } 00140 00141 cb_key = cn_cbor_string_create(key CBOR_CONTEXT_PARAM, errp); 00142 if (!cb_key) { return false; } 00143 return _append_kv(cb_map, cb_key, cb_value); 00144 } 00145 00146 cn_cbor* cn_cbor_array_create(CBOR_CONTEXT_COMMA cn_cbor_errback *errp) 00147 { 00148 cn_cbor* ret; 00149 INIT_CB(ret); 00150 00151 ret->type = CN_CBOR_ARRAY; 00152 ret->flags |= CN_CBOR_FL_COUNT; 00153 00154 return ret; 00155 } 00156 00157 bool cn_cbor_array_append(cn_cbor* cb_array, 00158 cn_cbor* cb_value, 00159 cn_cbor_errback *errp) 00160 { 00161 //Make sure input is an array. 00162 if(!cb_array || !cb_value || cb_array->type != CN_CBOR_ARRAY) 00163 { 00164 if (errp) {errp->err = CN_CBOR_ERR_INVALID_PARAMETER;} 00165 return false; 00166 } 00167 00168 cb_value->parent = cb_array; 00169 cb_value->next = NULL; 00170 if(cb_array->last_child) { 00171 cb_array->last_child->next = cb_value; 00172 } else { 00173 cb_array->first_child = cb_value; 00174 } 00175 cb_array->last_child = cb_value; 00176 cb_array->length++; 00177 return true; 00178 } 00179 00180 #ifdef __cplusplus 00181 } 00182 #endif 00183 00184 #endif /* CN_CBOR_C */
Generated on Tue Jul 12 2022 16:22:04 by
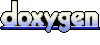