
Русифицированная версия программы для измерения температуры и отн. влажности и вывода информации на сенсорный TFT
Dependencies: FT800_2 HYT mbed
display.StringsTransform.cpp
00001 #include "display.h" 00002 00003 /************************************************************************************************************************** 00004 ************************** Transform humiditity / temperature float value to string *************************************** 00005 **************************************************************************************************************************/ 00006 // If isTemp = 0, resulting string includes 00007 // 1. current humididty 00008 // 3. " %" 00009 00010 // If isTemp = 1, resulting string includes 00011 // 1. "-" (optional), 00012 // 2. current temperature with decimal mark 00013 // 3. " С" 00014 00015 // Returns offset using for placing degree sign 00016 char Display::CreateStringTempHum(char *str, float number, bool isTemp) 00017 { 00018 char offsetForDegreeSign; 00019 short int multipedNumber = (short int)(number * 100); 00020 char strCnt = 0; 00021 if (isTemp) { 00022 if (multipedNumber < 0) { 00023 multipedNumber = -multipedNumber; 00024 str[strCnt] = '-'; 00025 strCnt++; 00026 } 00027 } 00028 if (multipedNumber >= 10000) { 00029 str[strCnt] = '0' + (multipedNumber % 100000) / 10000; 00030 strCnt++; 00031 } 00032 if (multipedNumber >= 1000) { 00033 str[strCnt] = '0' + (multipedNumber % 10000) / 1000; 00034 strCnt++; 00035 } 00036 if (multipedNumber >= 100) { 00037 str[strCnt] = '0' + (multipedNumber % 1000) / 100; 00038 strCnt++; 00039 } 00040 if (isTemp) { 00041 str[strCnt] = '.'; 00042 strCnt++; 00043 str[strCnt] = '0' + (multipedNumber % 100) / 10; 00044 strCnt++; 00045 str[strCnt] = ' '; 00046 strCnt++; 00047 offsetForDegreeSign = strCnt; 00048 str[strCnt] = 'C'; 00049 strCnt++; 00050 } else { 00051 str[strCnt] = '%'; 00052 strCnt++; 00053 } 00054 str[strCnt] = 0; 00055 00056 return offsetForDegreeSign; 00057 } 00058 00059 00060 /************************************************************************************************************************** 00061 ************************** Transform multiplied temperature value to string *********************************************** 00062 **************************************************************************************************************************/ 00063 // Resulting string includes 00064 // 1. "-" (optional), 00065 // 2. current temperature with decimal mark 00066 void Display::CreateStringMultipliedTemp(char *str, short int number) 00067 { 00068 char strCnt = 0; 00069 if (number < 0) { 00070 number = -number; 00071 str[strCnt] = '-'; 00072 strCnt++; 00073 } 00074 if (number >= 1000) { 00075 str[strCnt] = '0' + (number % 10000) / 1000; 00076 strCnt++; 00077 } 00078 if (number >= 100) { 00079 str[strCnt] = '0' + (number % 1000) / 100; 00080 strCnt++; 00081 } 00082 if (number >= 10) { 00083 str[strCnt] = '0' + (number % 100) / 10; 00084 strCnt++; 00085 } 00086 str[strCnt] = '.'; 00087 strCnt++; 00088 str[strCnt] = '0' + (number % 100) / 10; 00089 strCnt++; 00090 str[strCnt] = 0; 00091 } 00092 00093 00094 /************************************************************************************************************************** 00095 ************************** Transform number of seconds to HH:MM:SS string ************************************************* 00096 **************************************************************************************************************************/ 00097 void Display::CreateStringTime(char *str, uint32_t numberOfSeconds) 00098 { 00099 char hrs = (numberOfSeconds / 3600); 00100 if (hrs < 10) { 00101 str[0] = '0'; 00102 } else { 00103 str[0] = '0' + hrs / 10; 00104 } 00105 str[1] = '0' + hrs % 10; 00106 str[2] = ':'; 00107 char minutes = (numberOfSeconds / 60) % 60; 00108 if (minutes < 10) { 00109 str[3] = '0'; 00110 } else { 00111 str[3] = '0' + minutes / 10; 00112 } 00113 str[4] = '0' + minutes % 10; 00114 str[5] = ':'; 00115 char sec = numberOfSeconds % 60; 00116 if (sec < 10) { 00117 str[6] = '0'; 00118 } else { 00119 str[6] = '0' + sec / 10; 00120 } 00121 str[7] = '0' + sec % 10; 00122 str[8] = 0; 00123 } 00124 00125 void Display::CreateStringRussian(const string rustext) 00126 { 00127 // CHANGED ASCII: 00128 // 0123456789АБВГДЕЖЗИЙКЛМНОПРСТУФХЦЧШЩЪЫЬЭЮЯабвгдежзийклмнопрстуфхцчшщъыьэюя.,:-°±%<>rHYTICS 00129 int len = rustext.length(); 00130 int j = 0; 00131 for (int i = 0; i < len; i ++) { 00132 uint16_t res = uint8_t(rustext[i]); 00133 if (res > 0x7F) { 00134 res = res << 8 | uint8_t(rustext[i + 1]); 00135 // АБВ ... ноп 00136 if ((res >= 0xD090) && (res <= 0xD0BF)) { 00137 char offset = (char)(res - 0xD090); 00138 russianStr[j] = 32 + 11 + offset; 00139 // рст ... эюя 00140 } else if ((res >= 0xD180) && (res <= 0xD18F)) { 00141 char offset = (char)(res - 0xD180); 00142 russianStr[j] = 32 + 59 + offset; 00143 } 00144 // Degree sign 00145 else if (res == 0xC2B0) { 00146 russianStr[j] = 32 + 79; 00147 } 00148 // Plus-minus sign 00149 else if (res == 0xC2B1) { 00150 russianStr[j] = 32 + 80; 00151 } 00152 i++; 00153 } else { 00154 // Space 00155 if (res == 0x20) { 00156 russianStr[j] = 32; 00157 } 00158 // Numbers 00159 else if (res >= 0x30 && res <= 0x39) { 00160 russianStr[j] = 32 + 1 + (res - 0x30); 00161 } 00162 // . 00163 else if (res == 0x2E) { 00164 russianStr[j] = 32 + 75; 00165 } 00166 // , 00167 else if (res == 0x2C) { 00168 russianStr[j] = 32 + 76; 00169 } 00170 // : 00171 else if (res == 0x3A) { 00172 russianStr[j] = 32 + 77; 00173 } 00174 // - 00175 else if (res == 0x2D) { 00176 russianStr[j] = 32 + 78; 00177 } 00178 // % 00179 else if (res == 0x25) { 00180 russianStr[j] = 32 + 81; 00181 } 00182 // < 00183 else if (res == 0x3C) { 00184 russianStr[j] = 32 + 82; 00185 } 00186 // > 00187 else if (res == 0x3C) { 00188 russianStr[j] = 32 + 83; 00189 } 00190 // "r" 00191 else if (res == 0x72) { 00192 russianStr[j] = 32 + 84; 00193 } 00194 // "H" 00195 else if (res == 0x48) { 00196 russianStr[j] = 32 + 85; 00197 } 00198 // "Y" 00199 else if (res == 0x59) { 00200 russianStr[j] = 32 + 86; 00201 } 00202 // "T" 00203 else if (res == 0x54) { 00204 russianStr[j] = 32 + 87; 00205 } 00206 // "I" 00207 else if (res == 0x49) { 00208 russianStr[j] = 32 + 88; 00209 } 00210 // "C" 00211 else if (res == 0x43) { 00212 russianStr[j] = 32 + 89; 00213 } 00214 // "S" 00215 else if (res == 0x53) { 00216 russianStr[j] = 32 + 90; 00217 } 00218 } 00219 j++; 00220 } 00221 russianStr[j] = 0; 00222 }
Generated on Wed Jul 13 2022 04:41:34 by
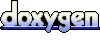