
n
Fork of LG by
Embed:
(wiki syntax)
Show/hide line numbers
core_cm3.h
Go to the documentation of this file.
00001 /**************************************************************************//** 00002 * @file core_cm3.h 00003 * @brief CMSIS Cortex-M3 Core Peripheral Access Layer Header File 00004 * @version V1.30 00005 * @date 30. October 2009 00006 * 00007 * @note 00008 * Copyright (C) 2009 ARM Limited. All rights reserved. 00009 * 00010 * @par 00011 * ARM Limited (ARM) is supplying this software for use with Cortex-M 00012 * processor based microcontrollers. This file can be freely distributed 00013 * within development tools that are supporting such ARM based processors. 00014 * 00015 * @par 00016 * THIS SOFTWARE IS PROVIDED "AS IS". NO WARRANTIES, WHETHER EXPRESS, IMPLIED 00017 * OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE APPLY TO THIS SOFTWARE. 00019 * ARM SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR SPECIAL, INCIDENTAL, OR 00020 * CONSEQUENTIAL DAMAGES, FOR ANY REASON WHATSOEVER. 00021 * 00022 ******************************************************************************/ 00023 00024 #ifndef __CM3_CORE_H__ 00025 #define __CM3_CORE_H__ 00026 00027 /** @addtogroup CMSIS_CM3_core_LintCinfiguration CMSIS CM3 Core Lint Configuration 00028 * 00029 * List of Lint messages which will be suppressed and not shown: 00030 * - Error 10: \n 00031 * register uint32_t __regBasePri __asm("basepri"); \n 00032 * Error 10: Expecting ';' 00033 * . 00034 * - Error 530: \n 00035 * return(__regBasePri); \n 00036 * Warning 530: Symbol '__regBasePri' (line 264) not initialized 00037 * . 00038 * - Error 550: \n 00039 * __regBasePri = (basePri & 0x1ff); \n 00040 * Warning 550: Symbol '__regBasePri' (line 271) not accessed 00041 * . 00042 * - Error 754: \n 00043 * uint32_t RESERVED0[24]; \n 00044 * Info 754: local structure member '<some, not used in the HAL>' (line 109, file ./cm3_core.h) not referenced 00045 * . 00046 * - Error 750: \n 00047 * #define __CM3_CORE_H__ \n 00048 * Info 750: local macro '__CM3_CORE_H__' (line 43, file./cm3_core.h) not referenced 00049 * . 00050 * - Error 528: \n 00051 * static __INLINE void NVIC_DisableIRQ(uint32_t IRQn) \n 00052 * Warning 528: Symbol 'NVIC_DisableIRQ(unsigned int)' (line 419, file ./cm3_core.h) not referenced 00053 * . 00054 * - Error 751: \n 00055 * } InterruptType_Type; \n 00056 * Info 751: local typedef 'InterruptType_Type' (line 170, file ./cm3_core.h) not referenced 00057 * . 00058 * Note: To re-enable a Message, insert a space before 'lint' * 00059 * 00060 */ 00061 00062 /*lint -save */ 00063 /*lint -e10 */ 00064 /*lint -e530 */ 00065 /*lint -e550 */ 00066 /*lint -e754 */ 00067 /*lint -e750 */ 00068 /*lint -e528 */ 00069 /*lint -e751 */ 00070 00071 00072 /** @addtogroup CMSIS_CM3_core_definitions CM3 Core Definitions 00073 This file defines all structures and symbols for CMSIS core: 00074 - CMSIS version number 00075 - Cortex-M core registers and bitfields 00076 - Cortex-M core peripheral base address 00077 @{ 00078 */ 00079 00080 #ifdef __cplusplus 00081 extern "C" { 00082 #endif 00083 00084 #define __CM3_CMSIS_VERSION_MAIN (0x01) /*!< [31:16] CMSIS HAL main version */ 00085 #define __CM3_CMSIS_VERSION_SUB (0x30) /*!< [15:0] CMSIS HAL sub version */ 00086 #define __CM3_CMSIS_VERSION ((__CM3_CMSIS_VERSION_MAIN << 16) | __CM3_CMSIS_VERSION_SUB) /*!< CMSIS HAL version number */ 00087 00088 #define __CORTEX_M (0x03) /*!< Cortex core */ 00089 00090 #include <stdint.h> /* Include standard types */ 00091 00092 #if defined (__ICCARM__) 00093 #include <intrinsics.h> /* IAR Intrinsics */ 00094 #endif 00095 00096 00097 #ifndef __NVIC_PRIO_BITS 00098 #define __NVIC_PRIO_BITS 4 /*!< standard definition for NVIC Priority Bits */ 00099 #endif 00100 00101 00102 00103 00104 /** 00105 * IO definitions 00106 * 00107 * define access restrictions to peripheral registers 00108 */ 00109 00110 #ifdef __cplusplus 00111 #define __I volatile /*!< defines 'read only' permissions */ 00112 #else 00113 #define __I volatile const /*!< defines 'read only' permissions */ 00114 #endif 00115 #define __O volatile /*!< defines 'write only' permissions */ 00116 #define __IO volatile /*!< defines 'read / write' permissions */ 00117 00118 00119 00120 /******************************************************************************* 00121 * Register Abstraction 00122 ******************************************************************************/ 00123 /** @addtogroup CMSIS_CM3_core_register CMSIS CM3 Core Register 00124 @{ 00125 */ 00126 00127 00128 /** @addtogroup CMSIS_CM3_NVIC CMSIS CM3 NVIC 00129 memory mapped structure for Nested Vectored Interrupt Controller (NVIC) 00130 @{ 00131 */ 00132 typedef struct { 00133 __IO uint32_t ISER[8]; /*!< Offset: 0x000 Interrupt Set Enable Register */ 00134 uint32_t RESERVED0[24]; 00135 __IO uint32_t ICER[8]; /*!< Offset: 0x080 Interrupt Clear Enable Register */ 00136 uint32_t RSERVED1[24]; 00137 __IO uint32_t ISPR[8]; /*!< Offset: 0x100 Interrupt Set Pending Register */ 00138 uint32_t RESERVED2[24]; 00139 __IO uint32_t ICPR[8]; /*!< Offset: 0x180 Interrupt Clear Pending Register */ 00140 uint32_t RESERVED3[24]; 00141 __IO uint32_t IABR[8]; /*!< Offset: 0x200 Interrupt Active bit Register */ 00142 uint32_t RESERVED4[56]; 00143 __IO uint8_t IP[240]; /*!< Offset: 0x300 Interrupt Priority Register (8Bit wide) */ 00144 uint32_t RESERVED5[644]; 00145 __O uint32_t STIR; /*!< Offset: 0xE00 Software Trigger Interrupt Register */ 00146 } NVIC_Type; 00147 /*@}*/ /* end of group CMSIS_CM3_NVIC */ 00148 00149 00150 /** @addtogroup CMSIS_CM3_SCB CMSIS CM3 SCB 00151 memory mapped structure for System Control Block (SCB) 00152 @{ 00153 */ 00154 typedef struct { 00155 __I uint32_t CPUID; /*!< Offset: 0x00 CPU ID Base Register */ 00156 __IO uint32_t ICSR; /*!< Offset: 0x04 Interrupt Control State Register */ 00157 __IO uint32_t VTOR; /*!< Offset: 0x08 Vector Table Offset Register */ 00158 __IO uint32_t AIRCR; /*!< Offset: 0x0C Application Interrupt / Reset Control Register */ 00159 __IO uint32_t SCR; /*!< Offset: 0x10 System Control Register */ 00160 __IO uint32_t CCR; /*!< Offset: 0x14 Configuration Control Register */ 00161 __IO uint8_t SHP[12]; /*!< Offset: 0x18 System Handlers Priority Registers (4-7, 8-11, 12-15) */ 00162 __IO uint32_t SHCSR; /*!< Offset: 0x24 System Handler Control and State Register */ 00163 __IO uint32_t CFSR; /*!< Offset: 0x28 Configurable Fault Status Register */ 00164 __IO uint32_t HFSR; /*!< Offset: 0x2C Hard Fault Status Register */ 00165 __IO uint32_t DFSR; /*!< Offset: 0x30 Debug Fault Status Register */ 00166 __IO uint32_t MMFAR; /*!< Offset: 0x34 Mem Manage Address Register */ 00167 __IO uint32_t BFAR; /*!< Offset: 0x38 Bus Fault Address Register */ 00168 __IO uint32_t AFSR; /*!< Offset: 0x3C Auxiliary Fault Status Register */ 00169 __I uint32_t PFR[2]; /*!< Offset: 0x40 Processor Feature Register */ 00170 __I uint32_t DFR; /*!< Offset: 0x48 Debug Feature Register */ 00171 __I uint32_t ADR; /*!< Offset: 0x4C Auxiliary Feature Register */ 00172 __I uint32_t MMFR[4]; /*!< Offset: 0x50 Memory Model Feature Register */ 00173 __I uint32_t ISAR[5]; /*!< Offset: 0x60 ISA Feature Register */ 00174 } SCB_Type; 00175 00176 /* SCB CPUID Register Definitions */ 00177 #define SCB_CPUID_IMPLEMENTER_Pos 24 /*!< SCB CPUID: IMPLEMENTER Position */ 00178 #define SCB_CPUID_IMPLEMENTER_Msk (0xFFul << SCB_CPUID_IMPLEMENTER_Pos) /*!< SCB CPUID: IMPLEMENTER Mask */ 00179 00180 #define SCB_CPUID_VARIANT_Pos 20 /*!< SCB CPUID: VARIANT Position */ 00181 #define SCB_CPUID_VARIANT_Msk (0xFul << SCB_CPUID_VARIANT_Pos) /*!< SCB CPUID: VARIANT Mask */ 00182 00183 #define SCB_CPUID_PARTNO_Pos 4 /*!< SCB CPUID: PARTNO Position */ 00184 #define SCB_CPUID_PARTNO_Msk (0xFFFul << SCB_CPUID_PARTNO_Pos) /*!< SCB CPUID: PARTNO Mask */ 00185 00186 #define SCB_CPUID_REVISION_Pos 0 /*!< SCB CPUID: REVISION Position */ 00187 #define SCB_CPUID_REVISION_Msk (0xFul << SCB_CPUID_REVISION_Pos) /*!< SCB CPUID: REVISION Mask */ 00188 00189 /* SCB Interrupt Control State Register Definitions */ 00190 #define SCB_ICSR_NMIPENDSET_Pos 31 /*!< SCB ICSR: NMIPENDSET Position */ 00191 #define SCB_ICSR_NMIPENDSET_Msk (1ul << SCB_ICSR_NMIPENDSET_Pos) /*!< SCB ICSR: NMIPENDSET Mask */ 00192 00193 #define SCB_ICSR_PENDSVSET_Pos 28 /*!< SCB ICSR: PENDSVSET Position */ 00194 #define SCB_ICSR_PENDSVSET_Msk (1ul << SCB_ICSR_PENDSVSET_Pos) /*!< SCB ICSR: PENDSVSET Mask */ 00195 00196 #define SCB_ICSR_PENDSVCLR_Pos 27 /*!< SCB ICSR: PENDSVCLR Position */ 00197 #define SCB_ICSR_PENDSVCLR_Msk (1ul << SCB_ICSR_PENDSVCLR_Pos) /*!< SCB ICSR: PENDSVCLR Mask */ 00198 00199 #define SCB_ICSR_PENDSTSET_Pos 26 /*!< SCB ICSR: PENDSTSET Position */ 00200 #define SCB_ICSR_PENDSTSET_Msk (1ul << SCB_ICSR_PENDSTSET_Pos) /*!< SCB ICSR: PENDSTSET Mask */ 00201 00202 #define SCB_ICSR_PENDSTCLR_Pos 25 /*!< SCB ICSR: PENDSTCLR Position */ 00203 #define SCB_ICSR_PENDSTCLR_Msk (1ul << SCB_ICSR_PENDSTCLR_Pos) /*!< SCB ICSR: PENDSTCLR Mask */ 00204 00205 #define SCB_ICSR_ISRPREEMPT_Pos 23 /*!< SCB ICSR: ISRPREEMPT Position */ 00206 #define SCB_ICSR_ISRPREEMPT_Msk (1ul << SCB_ICSR_ISRPREEMPT_Pos) /*!< SCB ICSR: ISRPREEMPT Mask */ 00207 00208 #define SCB_ICSR_ISRPENDING_Pos 22 /*!< SCB ICSR: ISRPENDING Position */ 00209 #define SCB_ICSR_ISRPENDING_Msk (1ul << SCB_ICSR_ISRPENDING_Pos) /*!< SCB ICSR: ISRPENDING Mask */ 00210 00211 #define SCB_ICSR_VECTPENDING_Pos 12 /*!< SCB ICSR: VECTPENDING Position */ 00212 #define SCB_ICSR_VECTPENDING_Msk (0x1FFul << SCB_ICSR_VECTPENDING_Pos) /*!< SCB ICSR: VECTPENDING Mask */ 00213 00214 #define SCB_ICSR_RETTOBASE_Pos 11 /*!< SCB ICSR: RETTOBASE Position */ 00215 #define SCB_ICSR_RETTOBASE_Msk (1ul << SCB_ICSR_RETTOBASE_Pos) /*!< SCB ICSR: RETTOBASE Mask */ 00216 00217 #define SCB_ICSR_VECTACTIVE_Pos 0 /*!< SCB ICSR: VECTACTIVE Position */ 00218 #define SCB_ICSR_VECTACTIVE_Msk (0x1FFul << SCB_ICSR_VECTACTIVE_Pos) /*!< SCB ICSR: VECTACTIVE Mask */ 00219 00220 /* SCB Interrupt Control State Register Definitions */ 00221 #define SCB_VTOR_TBLBASE_Pos 29 /*!< SCB VTOR: TBLBASE Position */ 00222 #define SCB_VTOR_TBLBASE_Msk (0x1FFul << SCB_VTOR_TBLBASE_Pos) /*!< SCB VTOR: TBLBASE Mask */ 00223 00224 #define SCB_VTOR_TBLOFF_Pos 7 /*!< SCB VTOR: TBLOFF Position */ 00225 #define SCB_VTOR_TBLOFF_Msk (0x3FFFFFul << SCB_VTOR_TBLOFF_Pos) /*!< SCB VTOR: TBLOFF Mask */ 00226 00227 /* SCB Application Interrupt and Reset Control Register Definitions */ 00228 #define SCB_AIRCR_VECTKEY_Pos 16 /*!< SCB AIRCR: VECTKEY Position */ 00229 #define SCB_AIRCR_VECTKEY_Msk (0xFFFFul << SCB_AIRCR_VECTKEY_Pos) /*!< SCB AIRCR: VECTKEY Mask */ 00230 00231 #define SCB_AIRCR_VECTKEYSTAT_Pos 16 /*!< SCB AIRCR: VECTKEYSTAT Position */ 00232 #define SCB_AIRCR_VECTKEYSTAT_Msk (0xFFFFul << SCB_AIRCR_VECTKEYSTAT_Pos) /*!< SCB AIRCR: VECTKEYSTAT Mask */ 00233 00234 #define SCB_AIRCR_ENDIANESS_Pos 15 /*!< SCB AIRCR: ENDIANESS Position */ 00235 #define SCB_AIRCR_ENDIANESS_Msk (1ul << SCB_AIRCR_ENDIANESS_Pos) /*!< SCB AIRCR: ENDIANESS Mask */ 00236 00237 #define SCB_AIRCR_PRIGROUP_Pos 8 /*!< SCB AIRCR: PRIGROUP Position */ 00238 #define SCB_AIRCR_PRIGROUP_Msk (7ul << SCB_AIRCR_PRIGROUP_Pos) /*!< SCB AIRCR: PRIGROUP Mask */ 00239 00240 #define SCB_AIRCR_SYSRESETREQ_Pos 2 /*!< SCB AIRCR: SYSRESETREQ Position */ 00241 #define SCB_AIRCR_SYSRESETREQ_Msk (1ul << SCB_AIRCR_SYSRESETREQ_Pos) /*!< SCB AIRCR: SYSRESETREQ Mask */ 00242 00243 #define SCB_AIRCR_VECTCLRACTIVE_Pos 1 /*!< SCB AIRCR: VECTCLRACTIVE Position */ 00244 #define SCB_AIRCR_VECTCLRACTIVE_Msk (1ul << SCB_AIRCR_VECTCLRACTIVE_Pos) /*!< SCB AIRCR: VECTCLRACTIVE Mask */ 00245 00246 #define SCB_AIRCR_VECTRESET_Pos 0 /*!< SCB AIRCR: VECTRESET Position */ 00247 #define SCB_AIRCR_VECTRESET_Msk (1ul << SCB_AIRCR_VECTRESET_Pos) /*!< SCB AIRCR: VECTRESET Mask */ 00248 00249 /* SCB System Control Register Definitions */ 00250 #define SCB_SCR_SEVONPEND_Pos 4 /*!< SCB SCR: SEVONPEND Position */ 00251 #define SCB_SCR_SEVONPEND_Msk (1ul << SCB_SCR_SEVONPEND_Pos) /*!< SCB SCR: SEVONPEND Mask */ 00252 00253 #define SCB_SCR_SLEEPDEEP_Pos 2 /*!< SCB SCR: SLEEPDEEP Position */ 00254 #define SCB_SCR_SLEEPDEEP_Msk (1ul << SCB_SCR_SLEEPDEEP_Pos) /*!< SCB SCR: SLEEPDEEP Mask */ 00255 00256 #define SCB_SCR_SLEEPONEXIT_Pos 1 /*!< SCB SCR: SLEEPONEXIT Position */ 00257 #define SCB_SCR_SLEEPONEXIT_Msk (1ul << SCB_SCR_SLEEPONEXIT_Pos) /*!< SCB SCR: SLEEPONEXIT Mask */ 00258 00259 /* SCB Configuration Control Register Definitions */ 00260 #define SCB_CCR_STKALIGN_Pos 9 /*!< SCB CCR: STKALIGN Position */ 00261 #define SCB_CCR_STKALIGN_Msk (1ul << SCB_CCR_STKALIGN_Pos) /*!< SCB CCR: STKALIGN Mask */ 00262 00263 #define SCB_CCR_BFHFNMIGN_Pos 8 /*!< SCB CCR: BFHFNMIGN Position */ 00264 #define SCB_CCR_BFHFNMIGN_Msk (1ul << SCB_CCR_BFHFNMIGN_Pos) /*!< SCB CCR: BFHFNMIGN Mask */ 00265 00266 #define SCB_CCR_DIV_0_TRP_Pos 4 /*!< SCB CCR: DIV_0_TRP Position */ 00267 #define SCB_CCR_DIV_0_TRP_Msk (1ul << SCB_CCR_DIV_0_TRP_Pos) /*!< SCB CCR: DIV_0_TRP Mask */ 00268 00269 #define SCB_CCR_UNALIGN_TRP_Pos 3 /*!< SCB CCR: UNALIGN_TRP Position */ 00270 #define SCB_CCR_UNALIGN_TRP_Msk (1ul << SCB_CCR_UNALIGN_TRP_Pos) /*!< SCB CCR: UNALIGN_TRP Mask */ 00271 00272 #define SCB_CCR_USERSETMPEND_Pos 1 /*!< SCB CCR: USERSETMPEND Position */ 00273 #define SCB_CCR_USERSETMPEND_Msk (1ul << SCB_CCR_USERSETMPEND_Pos) /*!< SCB CCR: USERSETMPEND Mask */ 00274 00275 #define SCB_CCR_NONBASETHRDENA_Pos 0 /*!< SCB CCR: NONBASETHRDENA Position */ 00276 #define SCB_CCR_NONBASETHRDENA_Msk (1ul << SCB_CCR_NONBASETHRDENA_Pos) /*!< SCB CCR: NONBASETHRDENA Mask */ 00277 00278 /* SCB System Handler Control and State Register Definitions */ 00279 #define SCB_SHCSR_USGFAULTENA_Pos 18 /*!< SCB SHCSR: USGFAULTENA Position */ 00280 #define SCB_SHCSR_USGFAULTENA_Msk (1ul << SCB_SHCSR_USGFAULTENA_Pos) /*!< SCB SHCSR: USGFAULTENA Mask */ 00281 00282 #define SCB_SHCSR_BUSFAULTENA_Pos 17 /*!< SCB SHCSR: BUSFAULTENA Position */ 00283 #define SCB_SHCSR_BUSFAULTENA_Msk (1ul << SCB_SHCSR_BUSFAULTENA_Pos) /*!< SCB SHCSR: BUSFAULTENA Mask */ 00284 00285 #define SCB_SHCSR_MEMFAULTENA_Pos 16 /*!< SCB SHCSR: MEMFAULTENA Position */ 00286 #define SCB_SHCSR_MEMFAULTENA_Msk (1ul << SCB_SHCSR_MEMFAULTENA_Pos) /*!< SCB SHCSR: MEMFAULTENA Mask */ 00287 00288 #define SCB_SHCSR_SVCALLPENDED_Pos 15 /*!< SCB SHCSR: SVCALLPENDED Position */ 00289 #define SCB_SHCSR_SVCALLPENDED_Msk (1ul << SCB_SHCSR_SVCALLPENDED_Pos) /*!< SCB SHCSR: SVCALLPENDED Mask */ 00290 00291 #define SCB_SHCSR_BUSFAULTPENDED_Pos 14 /*!< SCB SHCSR: BUSFAULTPENDED Position */ 00292 #define SCB_SHCSR_BUSFAULTPENDED_Msk (1ul << SCB_SHCSR_BUSFAULTPENDED_Pos) /*!< SCB SHCSR: BUSFAULTPENDED Mask */ 00293 00294 #define SCB_SHCSR_MEMFAULTPENDED_Pos 13 /*!< SCB SHCSR: MEMFAULTPENDED Position */ 00295 #define SCB_SHCSR_MEMFAULTPENDED_Msk (1ul << SCB_SHCSR_MEMFAULTPENDED_Pos) /*!< SCB SHCSR: MEMFAULTPENDED Mask */ 00296 00297 #define SCB_SHCSR_USGFAULTPENDED_Pos 12 /*!< SCB SHCSR: USGFAULTPENDED Position */ 00298 #define SCB_SHCSR_USGFAULTPENDED_Msk (1ul << SCB_SHCSR_USGFAULTPENDED_Pos) /*!< SCB SHCSR: USGFAULTPENDED Mask */ 00299 00300 #define SCB_SHCSR_SYSTICKACT_Pos 11 /*!< SCB SHCSR: SYSTICKACT Position */ 00301 #define SCB_SHCSR_SYSTICKACT_Msk (1ul << SCB_SHCSR_SYSTICKACT_Pos) /*!< SCB SHCSR: SYSTICKACT Mask */ 00302 00303 #define SCB_SHCSR_PENDSVACT_Pos 10 /*!< SCB SHCSR: PENDSVACT Position */ 00304 #define SCB_SHCSR_PENDSVACT_Msk (1ul << SCB_SHCSR_PENDSVACT_Pos) /*!< SCB SHCSR: PENDSVACT Mask */ 00305 00306 #define SCB_SHCSR_MONITORACT_Pos 8 /*!< SCB SHCSR: MONITORACT Position */ 00307 #define SCB_SHCSR_MONITORACT_Msk (1ul << SCB_SHCSR_MONITORACT_Pos) /*!< SCB SHCSR: MONITORACT Mask */ 00308 00309 #define SCB_SHCSR_SVCALLACT_Pos 7 /*!< SCB SHCSR: SVCALLACT Position */ 00310 #define SCB_SHCSR_SVCALLACT_Msk (1ul << SCB_SHCSR_SVCALLACT_Pos) /*!< SCB SHCSR: SVCALLACT Mask */ 00311 00312 #define SCB_SHCSR_USGFAULTACT_Pos 3 /*!< SCB SHCSR: USGFAULTACT Position */ 00313 #define SCB_SHCSR_USGFAULTACT_Msk (1ul << SCB_SHCSR_USGFAULTACT_Pos) /*!< SCB SHCSR: USGFAULTACT Mask */ 00314 00315 #define SCB_SHCSR_BUSFAULTACT_Pos 1 /*!< SCB SHCSR: BUSFAULTACT Position */ 00316 #define SCB_SHCSR_BUSFAULTACT_Msk (1ul << SCB_SHCSR_BUSFAULTACT_Pos) /*!< SCB SHCSR: BUSFAULTACT Mask */ 00317 00318 #define SCB_SHCSR_MEMFAULTACT_Pos 0 /*!< SCB SHCSR: MEMFAULTACT Position */ 00319 #define SCB_SHCSR_MEMFAULTACT_Msk (1ul << SCB_SHCSR_MEMFAULTACT_Pos) /*!< SCB SHCSR: MEMFAULTACT Mask */ 00320 00321 /* SCB Configurable Fault Status Registers Definitions */ 00322 #define SCB_CFSR_USGFAULTSR_Pos 16 /*!< SCB CFSR: Usage Fault Status Register Position */ 00323 #define SCB_CFSR_USGFAULTSR_Msk (0xFFFFul << SCB_CFSR_USGFAULTSR_Pos) /*!< SCB CFSR: Usage Fault Status Register Mask */ 00324 00325 #define SCB_CFSR_BUSFAULTSR_Pos 8 /*!< SCB CFSR: Bus Fault Status Register Position */ 00326 #define SCB_CFSR_BUSFAULTSR_Msk (0xFFul << SCB_CFSR_BUSFAULTSR_Pos) /*!< SCB CFSR: Bus Fault Status Register Mask */ 00327 00328 #define SCB_CFSR_MEMFAULTSR_Pos 0 /*!< SCB CFSR: Memory Manage Fault Status Register Position */ 00329 #define SCB_CFSR_MEMFAULTSR_Msk (0xFFul << SCB_CFSR_MEMFAULTSR_Pos) /*!< SCB CFSR: Memory Manage Fault Status Register Mask */ 00330 00331 /* SCB Hard Fault Status Registers Definitions */ 00332 #define SCB_HFSR_DEBUGEVT_Pos 31 /*!< SCB HFSR: DEBUGEVT Position */ 00333 #define SCB_HFSR_DEBUGEVT_Msk (1ul << SCB_HFSR_DEBUGEVT_Pos) /*!< SCB HFSR: DEBUGEVT Mask */ 00334 00335 #define SCB_HFSR_FORCED_Pos 30 /*!< SCB HFSR: FORCED Position */ 00336 #define SCB_HFSR_FORCED_Msk (1ul << SCB_HFSR_FORCED_Pos) /*!< SCB HFSR: FORCED Mask */ 00337 00338 #define SCB_HFSR_VECTTBL_Pos 1 /*!< SCB HFSR: VECTTBL Position */ 00339 #define SCB_HFSR_VECTTBL_Msk (1ul << SCB_HFSR_VECTTBL_Pos) /*!< SCB HFSR: VECTTBL Mask */ 00340 00341 /* SCB Debug Fault Status Register Definitions */ 00342 #define SCB_DFSR_EXTERNAL_Pos 4 /*!< SCB DFSR: EXTERNAL Position */ 00343 #define SCB_DFSR_EXTERNAL_Msk (1ul << SCB_DFSR_EXTERNAL_Pos) /*!< SCB DFSR: EXTERNAL Mask */ 00344 00345 #define SCB_DFSR_VCATCH_Pos 3 /*!< SCB DFSR: VCATCH Position */ 00346 #define SCB_DFSR_VCATCH_Msk (1ul << SCB_DFSR_VCATCH_Pos) /*!< SCB DFSR: VCATCH Mask */ 00347 00348 #define SCB_DFSR_DWTTRAP_Pos 2 /*!< SCB DFSR: DWTTRAP Position */ 00349 #define SCB_DFSR_DWTTRAP_Msk (1ul << SCB_DFSR_DWTTRAP_Pos) /*!< SCB DFSR: DWTTRAP Mask */ 00350 00351 #define SCB_DFSR_BKPT_Pos 1 /*!< SCB DFSR: BKPT Position */ 00352 #define SCB_DFSR_BKPT_Msk (1ul << SCB_DFSR_BKPT_Pos) /*!< SCB DFSR: BKPT Mask */ 00353 00354 #define SCB_DFSR_HALTED_Pos 0 /*!< SCB DFSR: HALTED Position */ 00355 #define SCB_DFSR_HALTED_Msk (1ul << SCB_DFSR_HALTED_Pos) /*!< SCB DFSR: HALTED Mask */ 00356 /*@}*/ /* end of group CMSIS_CM3_SCB */ 00357 00358 00359 /** @addtogroup CMSIS_CM3_SysTick CMSIS CM3 SysTick 00360 memory mapped structure for SysTick 00361 @{ 00362 */ 00363 typedef struct { 00364 __IO uint32_t CTRL; /*!< Offset: 0x00 SysTick Control and Status Register */ 00365 __IO uint32_t LOAD; /*!< Offset: 0x04 SysTick Reload Value Register */ 00366 __IO uint32_t VAL; /*!< Offset: 0x08 SysTick Current Value Register */ 00367 __I uint32_t CALIB; /*!< Offset: 0x0C SysTick Calibration Register */ 00368 } SysTick_Type; 00369 00370 /* SysTick Control / Status Register Definitions */ 00371 #define SysTick_CTRL_COUNTFLAG_Pos 16 /*!< SysTick CTRL: COUNTFLAG Position */ 00372 #define SysTick_CTRL_COUNTFLAG_Msk (1ul << SysTick_CTRL_COUNTFLAG_Pos) /*!< SysTick CTRL: COUNTFLAG Mask */ 00373 00374 #define SysTick_CTRL_CLKSOURCE_Pos 2 /*!< SysTick CTRL: CLKSOURCE Position */ 00375 #define SysTick_CTRL_CLKSOURCE_Msk (1ul << SysTick_CTRL_CLKSOURCE_Pos) /*!< SysTick CTRL: CLKSOURCE Mask */ 00376 00377 #define SysTick_CTRL_TICKINT_Pos 1 /*!< SysTick CTRL: TICKINT Position */ 00378 #define SysTick_CTRL_TICKINT_Msk (1ul << SysTick_CTRL_TICKINT_Pos) /*!< SysTick CTRL: TICKINT Mask */ 00379 00380 #define SysTick_CTRL_ENABLE_Pos 0 /*!< SysTick CTRL: ENABLE Position */ 00381 #define SysTick_CTRL_ENABLE_Msk (1ul << SysTick_CTRL_ENABLE_Pos) /*!< SysTick CTRL: ENABLE Mask */ 00382 00383 /* SysTick Reload Register Definitions */ 00384 #define SysTick_LOAD_RELOAD_Pos 0 /*!< SysTick LOAD: RELOAD Position */ 00385 #define SysTick_LOAD_RELOAD_Msk (0xFFFFFFul << SysTick_LOAD_RELOAD_Pos) /*!< SysTick LOAD: RELOAD Mask */ 00386 00387 /* SysTick Current Register Definitions */ 00388 #define SysTick_VAL_CURRENT_Pos 0 /*!< SysTick VAL: CURRENT Position */ 00389 #define SysTick_VAL_CURRENT_Msk (0xFFFFFFul << SysTick_VAL_CURRENT_Pos) /*!< SysTick VAL: CURRENT Mask */ 00390 00391 /* SysTick Calibration Register Definitions */ 00392 #define SysTick_CALIB_NOREF_Pos 31 /*!< SysTick CALIB: NOREF Position */ 00393 #define SysTick_CALIB_NOREF_Msk (1ul << SysTick_CALIB_NOREF_Pos) /*!< SysTick CALIB: NOREF Mask */ 00394 00395 #define SysTick_CALIB_SKEW_Pos 30 /*!< SysTick CALIB: SKEW Position */ 00396 #define SysTick_CALIB_SKEW_Msk (1ul << SysTick_CALIB_SKEW_Pos) /*!< SysTick CALIB: SKEW Mask */ 00397 00398 #define SysTick_CALIB_TENMS_Pos 0 /*!< SysTick CALIB: TENMS Position */ 00399 #define SysTick_CALIB_TENMS_Msk (0xFFFFFFul << SysTick_VAL_CURRENT_Pos) /*!< SysTick CALIB: TENMS Mask */ 00400 /*@}*/ /* end of group CMSIS_CM3_SysTick */ 00401 00402 00403 /** @addtogroup CMSIS_CM3_ITM CMSIS CM3 ITM 00404 memory mapped structure for Instrumentation Trace Macrocell (ITM) 00405 @{ 00406 */ 00407 typedef struct { 00408 __O union { 00409 __O uint8_t u8; /*!< Offset: ITM Stimulus Port 8-bit */ 00410 __O uint16_t u16; /*!< Offset: ITM Stimulus Port 16-bit */ 00411 __O uint32_t u32; /*!< Offset: ITM Stimulus Port 32-bit */ 00412 } PORT [32]; /*!< Offset: 0x00 ITM Stimulus Port Registers */ 00413 uint32_t RESERVED0[864]; 00414 __IO uint32_t TER; /*!< Offset: ITM Trace Enable Register */ 00415 uint32_t RESERVED1[15]; 00416 __IO uint32_t TPR; /*!< Offset: ITM Trace Privilege Register */ 00417 uint32_t RESERVED2[15]; 00418 __IO uint32_t TCR; /*!< Offset: ITM Trace Control Register */ 00419 uint32_t RESERVED3[29]; 00420 __IO uint32_t IWR; /*!< Offset: ITM Integration Write Register */ 00421 __IO uint32_t IRR; /*!< Offset: ITM Integration Read Register */ 00422 __IO uint32_t IMCR; /*!< Offset: ITM Integration Mode Control Register */ 00423 uint32_t RESERVED4[43]; 00424 __IO uint32_t LAR; /*!< Offset: ITM Lock Access Register */ 00425 __IO uint32_t LSR; /*!< Offset: ITM Lock Status Register */ 00426 uint32_t RESERVED5[6]; 00427 __I uint32_t PID4; /*!< Offset: ITM Peripheral Identification Register #4 */ 00428 __I uint32_t PID5; /*!< Offset: ITM Peripheral Identification Register #5 */ 00429 __I uint32_t PID6; /*!< Offset: ITM Peripheral Identification Register #6 */ 00430 __I uint32_t PID7; /*!< Offset: ITM Peripheral Identification Register #7 */ 00431 __I uint32_t PID0; /*!< Offset: ITM Peripheral Identification Register #0 */ 00432 __I uint32_t PID1; /*!< Offset: ITM Peripheral Identification Register #1 */ 00433 __I uint32_t PID2; /*!< Offset: ITM Peripheral Identification Register #2 */ 00434 __I uint32_t PID3; /*!< Offset: ITM Peripheral Identification Register #3 */ 00435 __I uint32_t CID0; /*!< Offset: ITM Component Identification Register #0 */ 00436 __I uint32_t CID1; /*!< Offset: ITM Component Identification Register #1 */ 00437 __I uint32_t CID2; /*!< Offset: ITM Component Identification Register #2 */ 00438 __I uint32_t CID3; /*!< Offset: ITM Component Identification Register #3 */ 00439 } ITM_Type; 00440 00441 /* ITM Trace Privilege Register Definitions */ 00442 #define ITM_TPR_PRIVMASK_Pos 0 /*!< ITM TPR: PRIVMASK Position */ 00443 #define ITM_TPR_PRIVMASK_Msk (0xFul << ITM_TPR_PRIVMASK_Pos) /*!< ITM TPR: PRIVMASK Mask */ 00444 00445 /* ITM Trace Control Register Definitions */ 00446 #define ITM_TCR_BUSY_Pos 23 /*!< ITM TCR: BUSY Position */ 00447 #define ITM_TCR_BUSY_Msk (1ul << ITM_TCR_BUSY_Pos) /*!< ITM TCR: BUSY Mask */ 00448 00449 #define ITM_TCR_ATBID_Pos 16 /*!< ITM TCR: ATBID Position */ 00450 #define ITM_TCR_ATBID_Msk (0x7Ful << ITM_TCR_ATBID_Pos) /*!< ITM TCR: ATBID Mask */ 00451 00452 #define ITM_TCR_TSPrescale_Pos 8 /*!< ITM TCR: TSPrescale Position */ 00453 #define ITM_TCR_TSPrescale_Msk (3ul << ITM_TCR_TSPrescale_Pos) /*!< ITM TCR: TSPrescale Mask */ 00454 00455 #define ITM_TCR_SWOENA_Pos 4 /*!< ITM TCR: SWOENA Position */ 00456 #define ITM_TCR_SWOENA_Msk (1ul << ITM_TCR_SWOENA_Pos) /*!< ITM TCR: SWOENA Mask */ 00457 00458 #define ITM_TCR_DWTENA_Pos 3 /*!< ITM TCR: DWTENA Position */ 00459 #define ITM_TCR_DWTENA_Msk (1ul << ITM_TCR_DWTENA_Pos) /*!< ITM TCR: DWTENA Mask */ 00460 00461 #define ITM_TCR_SYNCENA_Pos 2 /*!< ITM TCR: SYNCENA Position */ 00462 #define ITM_TCR_SYNCENA_Msk (1ul << ITM_TCR_SYNCENA_Pos) /*!< ITM TCR: SYNCENA Mask */ 00463 00464 #define ITM_TCR_TSENA_Pos 1 /*!< ITM TCR: TSENA Position */ 00465 #define ITM_TCR_TSENA_Msk (1ul << ITM_TCR_TSENA_Pos) /*!< ITM TCR: TSENA Mask */ 00466 00467 #define ITM_TCR_ITMENA_Pos 0 /*!< ITM TCR: ITM Enable bit Position */ 00468 #define ITM_TCR_ITMENA_Msk (1ul << ITM_TCR_ITMENA_Pos) /*!< ITM TCR: ITM Enable bit Mask */ 00469 00470 /* ITM Integration Write Register Definitions */ 00471 #define ITM_IWR_ATVALIDM_Pos 0 /*!< ITM IWR: ATVALIDM Position */ 00472 #define ITM_IWR_ATVALIDM_Msk (1ul << ITM_IWR_ATVALIDM_Pos) /*!< ITM IWR: ATVALIDM Mask */ 00473 00474 /* ITM Integration Read Register Definitions */ 00475 #define ITM_IRR_ATREADYM_Pos 0 /*!< ITM IRR: ATREADYM Position */ 00476 #define ITM_IRR_ATREADYM_Msk (1ul << ITM_IRR_ATREADYM_Pos) /*!< ITM IRR: ATREADYM Mask */ 00477 00478 /* ITM Integration Mode Control Register Definitions */ 00479 #define ITM_IMCR_INTEGRATION_Pos 0 /*!< ITM IMCR: INTEGRATION Position */ 00480 #define ITM_IMCR_INTEGRATION_Msk (1ul << ITM_IMCR_INTEGRATION_Pos) /*!< ITM IMCR: INTEGRATION Mask */ 00481 00482 /* ITM Lock Status Register Definitions */ 00483 #define ITM_LSR_ByteAcc_Pos 2 /*!< ITM LSR: ByteAcc Position */ 00484 #define ITM_LSR_ByteAcc_Msk (1ul << ITM_LSR_ByteAcc_Pos) /*!< ITM LSR: ByteAcc Mask */ 00485 00486 #define ITM_LSR_Access_Pos 1 /*!< ITM LSR: Access Position */ 00487 #define ITM_LSR_Access_Msk (1ul << ITM_LSR_Access_Pos) /*!< ITM LSR: Access Mask */ 00488 00489 #define ITM_LSR_Present_Pos 0 /*!< ITM LSR: Present Position */ 00490 #define ITM_LSR_Present_Msk (1ul << ITM_LSR_Present_Pos) /*!< ITM LSR: Present Mask */ 00491 /*@}*/ /* end of group CMSIS_CM3_ITM */ 00492 00493 00494 /** @addtogroup CMSIS_CM3_InterruptType CMSIS CM3 Interrupt Type 00495 memory mapped structure for Interrupt Type 00496 @{ 00497 */ 00498 typedef struct { 00499 uint32_t RESERVED0; 00500 __I uint32_t ICTR; /*!< Offset: 0x04 Interrupt Control Type Register */ 00501 #if ((defined __CM3_REV) && (__CM3_REV >= 0x200)) 00502 __IO uint32_t ACTLR; /*!< Offset: 0x08 Auxiliary Control Register */ 00503 #else 00504 uint32_t RESERVED1; 00505 #endif 00506 } InterruptType_Type; 00507 00508 /* Interrupt Controller Type Register Definitions */ 00509 #define InterruptType_ICTR_INTLINESNUM_Pos 0 /*!< InterruptType ICTR: INTLINESNUM Position */ 00510 #define InterruptType_ICTR_INTLINESNUM_Msk (0x1Ful << InterruptType_ICTR_INTLINESNUM_Pos) /*!< InterruptType ICTR: INTLINESNUM Mask */ 00511 00512 /* Auxiliary Control Register Definitions */ 00513 #define InterruptType_ACTLR_DISFOLD_Pos 2 /*!< InterruptType ACTLR: DISFOLD Position */ 00514 #define InterruptType_ACTLR_DISFOLD_Msk (1ul << InterruptType_ACTLR_DISFOLD_Pos) /*!< InterruptType ACTLR: DISFOLD Mask */ 00515 00516 #define InterruptType_ACTLR_DISDEFWBUF_Pos 1 /*!< InterruptType ACTLR: DISDEFWBUF Position */ 00517 #define InterruptType_ACTLR_DISDEFWBUF_Msk (1ul << InterruptType_ACTLR_DISDEFWBUF_Pos) /*!< InterruptType ACTLR: DISDEFWBUF Mask */ 00518 00519 #define InterruptType_ACTLR_DISMCYCINT_Pos 0 /*!< InterruptType ACTLR: DISMCYCINT Position */ 00520 #define InterruptType_ACTLR_DISMCYCINT_Msk (1ul << InterruptType_ACTLR_DISMCYCINT_Pos) /*!< InterruptType ACTLR: DISMCYCINT Mask */ 00521 /*@}*/ /* end of group CMSIS_CM3_InterruptType */ 00522 00523 00524 #if defined (__MPU_PRESENT) && (__MPU_PRESENT == 1) 00525 /** @addtogroup CMSIS_CM3_MPU CMSIS CM3 MPU 00526 memory mapped structure for Memory Protection Unit (MPU) 00527 @{ 00528 */ 00529 typedef struct { 00530 __I uint32_t TYPE; /*!< Offset: 0x00 MPU Type Register */ 00531 __IO uint32_t CTRL; /*!< Offset: 0x04 MPU Control Register */ 00532 __IO uint32_t RNR; /*!< Offset: 0x08 MPU Region RNRber Register */ 00533 __IO uint32_t RBAR; /*!< Offset: 0x0C MPU Region Base Address Register */ 00534 __IO uint32_t RASR; /*!< Offset: 0x10 MPU Region Attribute and Size Register */ 00535 __IO uint32_t RBAR_A1; /*!< Offset: 0x14 MPU Alias 1 Region Base Address Register */ 00536 __IO uint32_t RASR_A1; /*!< Offset: 0x18 MPU Alias 1 Region Attribute and Size Register */ 00537 __IO uint32_t RBAR_A2; /*!< Offset: 0x1C MPU Alias 2 Region Base Address Register */ 00538 __IO uint32_t RASR_A2; /*!< Offset: 0x20 MPU Alias 2 Region Attribute and Size Register */ 00539 __IO uint32_t RBAR_A3; /*!< Offset: 0x24 MPU Alias 3 Region Base Address Register */ 00540 __IO uint32_t RASR_A3; /*!< Offset: 0x28 MPU Alias 3 Region Attribute and Size Register */ 00541 } MPU_Type; 00542 00543 /* MPU Type Register */ 00544 #define MPU_TYPE_IREGION_Pos 16 /*!< MPU TYPE: IREGION Position */ 00545 #define MPU_TYPE_IREGION_Msk (0xFFul << MPU_TYPE_IREGION_Pos) /*!< MPU TYPE: IREGION Mask */ 00546 00547 #define MPU_TYPE_DREGION_Pos 8 /*!< MPU TYPE: DREGION Position */ 00548 #define MPU_TYPE_DREGION_Msk (0xFFul << MPU_TYPE_DREGION_Pos) /*!< MPU TYPE: DREGION Mask */ 00549 00550 #define MPU_TYPE_SEPARATE_Pos 0 /*!< MPU TYPE: SEPARATE Position */ 00551 #define MPU_TYPE_SEPARATE_Msk (1ul << MPU_TYPE_SEPARATE_Pos) /*!< MPU TYPE: SEPARATE Mask */ 00552 00553 /* MPU Control Register */ 00554 #define MPU_CTRL_PRIVDEFENA_Pos 2 /*!< MPU CTRL: PRIVDEFENA Position */ 00555 #define MPU_CTRL_PRIVDEFENA_Msk (1ul << MPU_CTRL_PRIVDEFENA_Pos) /*!< MPU CTRL: PRIVDEFENA Mask */ 00556 00557 #define MPU_CTRL_HFNMIENA_Pos 1 /*!< MPU CTRL: HFNMIENA Position */ 00558 #define MPU_CTRL_HFNMIENA_Msk (1ul << MPU_CTRL_HFNMIENA_Pos) /*!< MPU CTRL: HFNMIENA Mask */ 00559 00560 #define MPU_CTRL_ENABLE_Pos 0 /*!< MPU CTRL: ENABLE Position */ 00561 #define MPU_CTRL_ENABLE_Msk (1ul << MPU_CTRL_ENABLE_Pos) /*!< MPU CTRL: ENABLE Mask */ 00562 00563 /* MPU Region Number Register */ 00564 #define MPU_RNR_REGION_Pos 0 /*!< MPU RNR: REGION Position */ 00565 #define MPU_RNR_REGION_Msk (0xFFul << MPU_RNR_REGION_Pos) /*!< MPU RNR: REGION Mask */ 00566 00567 /* MPU Region Base Address Register */ 00568 #define MPU_RBAR_ADDR_Pos 5 /*!< MPU RBAR: ADDR Position */ 00569 #define MPU_RBAR_ADDR_Msk (0x7FFFFFFul << MPU_RBAR_ADDR_Pos) /*!< MPU RBAR: ADDR Mask */ 00570 00571 #define MPU_RBAR_VALID_Pos 4 /*!< MPU RBAR: VALID Position */ 00572 #define MPU_RBAR_VALID_Msk (1ul << MPU_RBAR_VALID_Pos) /*!< MPU RBAR: VALID Mask */ 00573 00574 #define MPU_RBAR_REGION_Pos 0 /*!< MPU RBAR: REGION Position */ 00575 #define MPU_RBAR_REGION_Msk (0xFul << MPU_RBAR_REGION_Pos) /*!< MPU RBAR: REGION Mask */ 00576 00577 /* MPU Region Attribute and Size Register */ 00578 #define MPU_RASR_XN_Pos 28 /*!< MPU RASR: XN Position */ 00579 #define MPU_RASR_XN_Msk (1ul << MPU_RASR_XN_Pos) /*!< MPU RASR: XN Mask */ 00580 00581 #define MPU_RASR_AP_Pos 24 /*!< MPU RASR: AP Position */ 00582 #define MPU_RASR_AP_Msk (7ul << MPU_RASR_AP_Pos) /*!< MPU RASR: AP Mask */ 00583 00584 #define MPU_RASR_TEX_Pos 19 /*!< MPU RASR: TEX Position */ 00585 #define MPU_RASR_TEX_Msk (7ul << MPU_RASR_TEX_Pos) /*!< MPU RASR: TEX Mask */ 00586 00587 #define MPU_RASR_S_Pos 18 /*!< MPU RASR: Shareable bit Position */ 00588 #define MPU_RASR_S_Msk (1ul << MPU_RASR_S_Pos) /*!< MPU RASR: Shareable bit Mask */ 00589 00590 #define MPU_RASR_C_Pos 17 /*!< MPU RASR: Cacheable bit Position */ 00591 #define MPU_RASR_C_Msk (1ul << MPU_RASR_C_Pos) /*!< MPU RASR: Cacheable bit Mask */ 00592 00593 #define MPU_RASR_B_Pos 16 /*!< MPU RASR: Bufferable bit Position */ 00594 #define MPU_RASR_B_Msk (1ul << MPU_RASR_B_Pos) /*!< MPU RASR: Bufferable bit Mask */ 00595 00596 #define MPU_RASR_SRD_Pos 8 /*!< MPU RASR: Sub-Region Disable Position */ 00597 #define MPU_RASR_SRD_Msk (0xFFul << MPU_RASR_SRD_Pos) /*!< MPU RASR: Sub-Region Disable Mask */ 00598 00599 #define MPU_RASR_SIZE_Pos 1 /*!< MPU RASR: Region Size Field Position */ 00600 #define MPU_RASR_SIZE_Msk (0x1Ful << MPU_RASR_SIZE_Pos) /*!< MPU RASR: Region Size Field Mask */ 00601 00602 #define MPU_RASR_ENA_Pos 0 /*!< MPU RASR: Region enable bit Position */ 00603 #define MPU_RASR_ENA_Msk (0x1Ful << MPU_RASR_ENA_Pos) /*!< MPU RASR: Region enable bit Disable Mask */ 00604 00605 /*@}*/ /* end of group CMSIS_CM3_MPU */ 00606 #endif 00607 00608 00609 /** @addtogroup CMSIS_CM3_CoreDebug CMSIS CM3 Core Debug 00610 memory mapped structure for Core Debug Register 00611 @{ 00612 */ 00613 typedef struct { 00614 __IO uint32_t DHCSR; /*!< Offset: 0x00 Debug Halting Control and Status Register */ 00615 __O uint32_t DCRSR; /*!< Offset: 0x04 Debug Core Register Selector Register */ 00616 __IO uint32_t DCRDR; /*!< Offset: 0x08 Debug Core Register Data Register */ 00617 __IO uint32_t DEMCR; /*!< Offset: 0x0C Debug Exception and Monitor Control Register */ 00618 } CoreDebug_Type; 00619 00620 /* Debug Halting Control and Status Register */ 00621 #define CoreDebug_DHCSR_DBGKEY_Pos 16 /*!< CoreDebug DHCSR: DBGKEY Position */ 00622 #define CoreDebug_DHCSR_DBGKEY_Msk (0xFFFFul << CoreDebug_DHCSR_DBGKEY_Pos) /*!< CoreDebug DHCSR: DBGKEY Mask */ 00623 00624 #define CoreDebug_DHCSR_S_RESET_ST_Pos 25 /*!< CoreDebug DHCSR: S_RESET_ST Position */ 00625 #define CoreDebug_DHCSR_S_RESET_ST_Msk (1ul << CoreDebug_DHCSR_S_RESET_ST_Pos) /*!< CoreDebug DHCSR: S_RESET_ST Mask */ 00626 00627 #define CoreDebug_DHCSR_S_RETIRE_ST_Pos 24 /*!< CoreDebug DHCSR: S_RETIRE_ST Position */ 00628 #define CoreDebug_DHCSR_S_RETIRE_ST_Msk (1ul << CoreDebug_DHCSR_S_RETIRE_ST_Pos) /*!< CoreDebug DHCSR: S_RETIRE_ST Mask */ 00629 00630 #define CoreDebug_DHCSR_S_LOCKUP_Pos 19 /*!< CoreDebug DHCSR: S_LOCKUP Position */ 00631 #define CoreDebug_DHCSR_S_LOCKUP_Msk (1ul << CoreDebug_DHCSR_S_LOCKUP_Pos) /*!< CoreDebug DHCSR: S_LOCKUP Mask */ 00632 00633 #define CoreDebug_DHCSR_S_SLEEP_Pos 18 /*!< CoreDebug DHCSR: S_SLEEP Position */ 00634 #define CoreDebug_DHCSR_S_SLEEP_Msk (1ul << CoreDebug_DHCSR_S_SLEEP_Pos) /*!< CoreDebug DHCSR: S_SLEEP Mask */ 00635 00636 #define CoreDebug_DHCSR_S_HALT_Pos 17 /*!< CoreDebug DHCSR: S_HALT Position */ 00637 #define CoreDebug_DHCSR_S_HALT_Msk (1ul << CoreDebug_DHCSR_S_HALT_Pos) /*!< CoreDebug DHCSR: S_HALT Mask */ 00638 00639 #define CoreDebug_DHCSR_S_REGRDY_Pos 16 /*!< CoreDebug DHCSR: S_REGRDY Position */ 00640 #define CoreDebug_DHCSR_S_REGRDY_Msk (1ul << CoreDebug_DHCSR_S_REGRDY_Pos) /*!< CoreDebug DHCSR: S_REGRDY Mask */ 00641 00642 #define CoreDebug_DHCSR_C_SNAPSTALL_Pos 5 /*!< CoreDebug DHCSR: C_SNAPSTALL Position */ 00643 #define CoreDebug_DHCSR_C_SNAPSTALL_Msk (1ul << CoreDebug_DHCSR_C_SNAPSTALL_Pos) /*!< CoreDebug DHCSR: C_SNAPSTALL Mask */ 00644 00645 #define CoreDebug_DHCSR_C_MASKINTS_Pos 3 /*!< CoreDebug DHCSR: C_MASKINTS Position */ 00646 #define CoreDebug_DHCSR_C_MASKINTS_Msk (1ul << CoreDebug_DHCSR_C_MASKINTS_Pos) /*!< CoreDebug DHCSR: C_MASKINTS Mask */ 00647 00648 #define CoreDebug_DHCSR_C_STEP_Pos 2 /*!< CoreDebug DHCSR: C_STEP Position */ 00649 #define CoreDebug_DHCSR_C_STEP_Msk (1ul << CoreDebug_DHCSR_C_STEP_Pos) /*!< CoreDebug DHCSR: C_STEP Mask */ 00650 00651 #define CoreDebug_DHCSR_C_HALT_Pos 1 /*!< CoreDebug DHCSR: C_HALT Position */ 00652 #define CoreDebug_DHCSR_C_HALT_Msk (1ul << CoreDebug_DHCSR_C_HALT_Pos) /*!< CoreDebug DHCSR: C_HALT Mask */ 00653 00654 #define CoreDebug_DHCSR_C_DEBUGEN_Pos 0 /*!< CoreDebug DHCSR: C_DEBUGEN Position */ 00655 #define CoreDebug_DHCSR_C_DEBUGEN_Msk (1ul << CoreDebug_DHCSR_C_DEBUGEN_Pos) /*!< CoreDebug DHCSR: C_DEBUGEN Mask */ 00656 00657 /* Debug Core Register Selector Register */ 00658 #define CoreDebug_DCRSR_REGWnR_Pos 16 /*!< CoreDebug DCRSR: REGWnR Position */ 00659 #define CoreDebug_DCRSR_REGWnR_Msk (1ul << CoreDebug_DCRSR_REGWnR_Pos) /*!< CoreDebug DCRSR: REGWnR Mask */ 00660 00661 #define CoreDebug_DCRSR_REGSEL_Pos 0 /*!< CoreDebug DCRSR: REGSEL Position */ 00662 #define CoreDebug_DCRSR_REGSEL_Msk (0x1Ful << CoreDebug_DCRSR_REGSEL_Pos) /*!< CoreDebug DCRSR: REGSEL Mask */ 00663 00664 /* Debug Exception and Monitor Control Register */ 00665 #define CoreDebug_DEMCR_TRCENA_Pos 24 /*!< CoreDebug DEMCR: TRCENA Position */ 00666 #define CoreDebug_DEMCR_TRCENA_Msk (1ul << CoreDebug_DEMCR_TRCENA_Pos) /*!< CoreDebug DEMCR: TRCENA Mask */ 00667 00668 #define CoreDebug_DEMCR_MON_REQ_Pos 19 /*!< CoreDebug DEMCR: MON_REQ Position */ 00669 #define CoreDebug_DEMCR_MON_REQ_Msk (1ul << CoreDebug_DEMCR_MON_REQ_Pos) /*!< CoreDebug DEMCR: MON_REQ Mask */ 00670 00671 #define CoreDebug_DEMCR_MON_STEP_Pos 18 /*!< CoreDebug DEMCR: MON_STEP Position */ 00672 #define CoreDebug_DEMCR_MON_STEP_Msk (1ul << CoreDebug_DEMCR_MON_STEP_Pos) /*!< CoreDebug DEMCR: MON_STEP Mask */ 00673 00674 #define CoreDebug_DEMCR_MON_PEND_Pos 17 /*!< CoreDebug DEMCR: MON_PEND Position */ 00675 #define CoreDebug_DEMCR_MON_PEND_Msk (1ul << CoreDebug_DEMCR_MON_PEND_Pos) /*!< CoreDebug DEMCR: MON_PEND Mask */ 00676 00677 #define CoreDebug_DEMCR_MON_EN_Pos 16 /*!< CoreDebug DEMCR: MON_EN Position */ 00678 #define CoreDebug_DEMCR_MON_EN_Msk (1ul << CoreDebug_DEMCR_MON_EN_Pos) /*!< CoreDebug DEMCR: MON_EN Mask */ 00679 00680 #define CoreDebug_DEMCR_VC_HARDERR_Pos 10 /*!< CoreDebug DEMCR: VC_HARDERR Position */ 00681 #define CoreDebug_DEMCR_VC_HARDERR_Msk (1ul << CoreDebug_DEMCR_VC_HARDERR_Pos) /*!< CoreDebug DEMCR: VC_HARDERR Mask */ 00682 00683 #define CoreDebug_DEMCR_VC_INTERR_Pos 9 /*!< CoreDebug DEMCR: VC_INTERR Position */ 00684 #define CoreDebug_DEMCR_VC_INTERR_Msk (1ul << CoreDebug_DEMCR_VC_INTERR_Pos) /*!< CoreDebug DEMCR: VC_INTERR Mask */ 00685 00686 #define CoreDebug_DEMCR_VC_BUSERR_Pos 8 /*!< CoreDebug DEMCR: VC_BUSERR Position */ 00687 #define CoreDebug_DEMCR_VC_BUSERR_Msk (1ul << CoreDebug_DEMCR_VC_BUSERR_Pos) /*!< CoreDebug DEMCR: VC_BUSERR Mask */ 00688 00689 #define CoreDebug_DEMCR_VC_STATERR_Pos 7 /*!< CoreDebug DEMCR: VC_STATERR Position */ 00690 #define CoreDebug_DEMCR_VC_STATERR_Msk (1ul << CoreDebug_DEMCR_VC_STATERR_Pos) /*!< CoreDebug DEMCR: VC_STATERR Mask */ 00691 00692 #define CoreDebug_DEMCR_VC_CHKERR_Pos 6 /*!< CoreDebug DEMCR: VC_CHKERR Position */ 00693 #define CoreDebug_DEMCR_VC_CHKERR_Msk (1ul << CoreDebug_DEMCR_VC_CHKERR_Pos) /*!< CoreDebug DEMCR: VC_CHKERR Mask */ 00694 00695 #define CoreDebug_DEMCR_VC_NOCPERR_Pos 5 /*!< CoreDebug DEMCR: VC_NOCPERR Position */ 00696 #define CoreDebug_DEMCR_VC_NOCPERR_Msk (1ul << CoreDebug_DEMCR_VC_NOCPERR_Pos) /*!< CoreDebug DEMCR: VC_NOCPERR Mask */ 00697 00698 #define CoreDebug_DEMCR_VC_MMERR_Pos 4 /*!< CoreDebug DEMCR: VC_MMERR Position */ 00699 #define CoreDebug_DEMCR_VC_MMERR_Msk (1ul << CoreDebug_DEMCR_VC_MMERR_Pos) /*!< CoreDebug DEMCR: VC_MMERR Mask */ 00700 00701 #define CoreDebug_DEMCR_VC_CORERESET_Pos 0 /*!< CoreDebug DEMCR: VC_CORERESET Position */ 00702 #define CoreDebug_DEMCR_VC_CORERESET_Msk (1ul << CoreDebug_DEMCR_VC_CORERESET_Pos) /*!< CoreDebug DEMCR: VC_CORERESET Mask */ 00703 /*@}*/ /* end of group CMSIS_CM3_CoreDebug */ 00704 00705 00706 /* Memory mapping of Cortex-M3 Hardware */ 00707 #define SCS_BASE (0xE000E000) /*!< System Control Space Base Address */ 00708 #define ITM_BASE (0xE0000000) /*!< ITM Base Address */ 00709 #define CoreDebug_BASE (0xE000EDF0) /*!< Core Debug Base Address */ 00710 #define SysTick_BASE (SCS_BASE + 0x0010) /*!< SysTick Base Address */ 00711 #define NVIC_BASE (SCS_BASE + 0x0100) /*!< NVIC Base Address */ 00712 #define SCB_BASE (SCS_BASE + 0x0D00) /*!< System Control Block Base Address */ 00713 00714 #define InterruptType ((InterruptType_Type *) SCS_BASE) /*!< Interrupt Type Register */ 00715 #define SCB ((SCB_Type *) SCB_BASE) /*!< SCB configuration struct */ 00716 #define SysTick ((SysTick_Type *) SysTick_BASE) /*!< SysTick configuration struct */ 00717 #define NVIC ((NVIC_Type *) NVIC_BASE) /*!< NVIC configuration struct */ 00718 #define ITM ((ITM_Type *) ITM_BASE) /*!< ITM configuration struct */ 00719 #define CoreDebug ((CoreDebug_Type *) CoreDebug_BASE) /*!< Core Debug configuration struct */ 00720 00721 #if defined (__MPU_PRESENT) && (__MPU_PRESENT == 1) 00722 #define MPU_BASE (SCS_BASE + 0x0D90) /*!< Memory Protection Unit */ 00723 #define MPU ((MPU_Type*) MPU_BASE) /*!< Memory Protection Unit */ 00724 #endif 00725 00726 /*@}*/ /* end of group CMSIS_CM3_core_register */ 00727 00728 00729 /******************************************************************************* 00730 * Hardware Abstraction Layer 00731 ******************************************************************************/ 00732 00733 #if defined ( __CC_ARM ) 00734 #define __ASM __asm /*!< asm keyword for ARM Compiler */ 00735 #define __INLINE __inline /*!< inline keyword for ARM Compiler */ 00736 00737 #elif defined ( __ICCARM__ ) 00738 #define __ASM __asm /*!< asm keyword for IAR Compiler */ 00739 #define __INLINE inline /*!< inline keyword for IAR Compiler. Only avaiable in High optimization mode! */ 00740 00741 #elif defined ( __GNUC__ ) 00742 #define __ASM __asm /*!< asm keyword for GNU Compiler */ 00743 #define __INLINE inline /*!< inline keyword for GNU Compiler */ 00744 00745 #elif defined ( __TASKING__ ) 00746 #define __ASM __asm /*!< asm keyword for TASKING Compiler */ 00747 #define __INLINE inline /*!< inline keyword for TASKING Compiler */ 00748 00749 #endif 00750 00751 00752 /* ################### Compiler specific Intrinsics ########################### */ 00753 00754 #if defined ( __CC_ARM ) /*------------------RealView Compiler -----------------*/ 00755 /* ARM armcc specific functions */ 00756 00757 #define __enable_fault_irq __enable_fiq 00758 #define __disable_fault_irq __disable_fiq 00759 00760 #define __NOP __nop 00761 #define __WFI __wfi 00762 #define __WFE __wfe 00763 #define __SEV __sev 00764 #define __ISB() __isb(0) 00765 #define __DSB() __dsb(0) 00766 #define __DMB() __dmb(0) 00767 #define __REV __rev 00768 #define __RBIT __rbit 00769 #define __LDREXB(ptr) ((unsigned char ) __ldrex(ptr)) 00770 #define __LDREXH(ptr) ((unsigned short) __ldrex(ptr)) 00771 #define __LDREXW(ptr) ((unsigned int ) __ldrex(ptr)) 00772 #define __STREXB(value, ptr) __strex(value, ptr) 00773 #define __STREXH(value, ptr) __strex(value, ptr) 00774 #define __STREXW(value, ptr) __strex(value, ptr) 00775 00776 00777 /* intrinsic unsigned long long __ldrexd(volatile void *ptr) */ 00778 /* intrinsic int __strexd(unsigned long long val, volatile void *ptr) */ 00779 /* intrinsic void __enable_irq(); */ 00780 /* intrinsic void __disable_irq(); */ 00781 00782 00783 /** 00784 * @brief Return the Process Stack Pointer 00785 * 00786 * @return ProcessStackPointer 00787 * 00788 * Return the actual process stack pointer 00789 */ 00790 extern uint32_t __get_PSP(void); 00791 00792 /** 00793 * @brief Set the Process Stack Pointer 00794 * 00795 * @param topOfProcStack Process Stack Pointer 00796 * 00797 * Assign the value ProcessStackPointer to the MSP 00798 * (process stack pointer) Cortex processor register 00799 */ 00800 extern void __set_PSP(uint32_t topOfProcStack); 00801 00802 /** 00803 * @brief Return the Main Stack Pointer 00804 * 00805 * @return Main Stack Pointer 00806 * 00807 * Return the current value of the MSP (main stack pointer) 00808 * Cortex processor register 00809 */ 00810 extern uint32_t __get_MSP(void); 00811 00812 /** 00813 * @brief Set the Main Stack Pointer 00814 * 00815 * @param topOfMainStack Main Stack Pointer 00816 * 00817 * Assign the value mainStackPointer to the MSP 00818 * (main stack pointer) Cortex processor register 00819 */ 00820 extern void __set_MSP(uint32_t topOfMainStack); 00821 00822 /** 00823 * @brief Reverse byte order in unsigned short value 00824 * 00825 * @param value value to reverse 00826 * @return reversed value 00827 * 00828 * Reverse byte order in unsigned short value 00829 */ 00830 extern uint32_t __REV16(uint16_t value); 00831 00832 /** 00833 * @brief Reverse byte order in signed short value with sign extension to integer 00834 * 00835 * @param value value to reverse 00836 * @return reversed value 00837 * 00838 * Reverse byte order in signed short value with sign extension to integer 00839 */ 00840 extern int32_t __REVSH(int16_t value); 00841 00842 00843 #if (__ARMCC_VERSION < 400000) 00844 00845 /** 00846 * @brief Remove the exclusive lock created by ldrex 00847 * 00848 * Removes the exclusive lock which is created by ldrex. 00849 */ 00850 extern void __CLREX(void); 00851 00852 /** 00853 * @brief Return the Base Priority value 00854 * 00855 * @return BasePriority 00856 * 00857 * Return the content of the base priority register 00858 */ 00859 extern uint32_t __get_BASEPRI(void); 00860 00861 /** 00862 * @brief Set the Base Priority value 00863 * 00864 * @param basePri BasePriority 00865 * 00866 * Set the base priority register 00867 */ 00868 extern void __set_BASEPRI(uint32_t basePri); 00869 00870 /** 00871 * @brief Return the Priority Mask value 00872 * 00873 * @return PriMask 00874 * 00875 * Return state of the priority mask bit from the priority mask register 00876 */ 00877 extern uint32_t __get_PRIMASK(void); 00878 00879 /** 00880 * @brief Set the Priority Mask value 00881 * 00882 * @param priMask PriMask 00883 * 00884 * Set the priority mask bit in the priority mask register 00885 */ 00886 extern void __set_PRIMASK(uint32_t priMask); 00887 00888 /** 00889 * @brief Return the Fault Mask value 00890 * 00891 * @return FaultMask 00892 * 00893 * Return the content of the fault mask register 00894 */ 00895 extern uint32_t __get_FAULTMASK(void); 00896 00897 /** 00898 * @brief Set the Fault Mask value 00899 * 00900 * @param faultMask faultMask value 00901 * 00902 * Set the fault mask register 00903 */ 00904 extern void __set_FAULTMASK(uint32_t faultMask); 00905 00906 /** 00907 * @brief Return the Control Register value 00908 * 00909 * @return Control value 00910 * 00911 * Return the content of the control register 00912 */ 00913 extern uint32_t __get_CONTROL(void); 00914 00915 /** 00916 * @brief Set the Control Register value 00917 * 00918 * @param control Control value 00919 * 00920 * Set the control register 00921 */ 00922 extern void __set_CONTROL(uint32_t control); 00923 00924 #else /* (__ARMCC_VERSION >= 400000) */ 00925 00926 /** 00927 * @brief Remove the exclusive lock created by ldrex 00928 * 00929 * Removes the exclusive lock which is created by ldrex. 00930 */ 00931 #define __CLREX __clrex 00932 00933 /** 00934 * @brief Return the Base Priority value 00935 * 00936 * @return BasePriority 00937 * 00938 * Return the content of the base priority register 00939 */ 00940 static __INLINE uint32_t __get_BASEPRI(void) 00941 { 00942 register uint32_t __regBasePri __ASM("basepri"); 00943 return(__regBasePri); 00944 } 00945 00946 /** 00947 * @brief Set the Base Priority value 00948 * 00949 * @param basePri BasePriority 00950 * 00951 * Set the base priority register 00952 */ 00953 static __INLINE void __set_BASEPRI(uint32_t basePri) 00954 { 00955 register uint32_t __regBasePri __ASM("basepri"); 00956 __regBasePri = (basePri & 0xff); 00957 } 00958 00959 /** 00960 * @brief Return the Priority Mask value 00961 * 00962 * @return PriMask 00963 * 00964 * Return state of the priority mask bit from the priority mask register 00965 */ 00966 static __INLINE uint32_t __get_PRIMASK(void) 00967 { 00968 register uint32_t __regPriMask __ASM("primask"); 00969 return(__regPriMask); 00970 } 00971 00972 /** 00973 * @brief Set the Priority Mask value 00974 * 00975 * @param priMask PriMask 00976 * 00977 * Set the priority mask bit in the priority mask register 00978 */ 00979 static __INLINE void __set_PRIMASK(uint32_t priMask) 00980 { 00981 register uint32_t __regPriMask __ASM("primask"); 00982 __regPriMask = (priMask); 00983 } 00984 00985 /** 00986 * @brief Return the Fault Mask value 00987 * 00988 * @return FaultMask 00989 * 00990 * Return the content of the fault mask register 00991 */ 00992 static __INLINE uint32_t __get_FAULTMASK(void) 00993 { 00994 register uint32_t __regFaultMask __ASM("faultmask"); 00995 return(__regFaultMask); 00996 } 00997 00998 /** 00999 * @brief Set the Fault Mask value 01000 * 01001 * @param faultMask faultMask value 01002 * 01003 * Set the fault mask register 01004 */ 01005 static __INLINE void __set_FAULTMASK(uint32_t faultMask) 01006 { 01007 register uint32_t __regFaultMask __ASM("faultmask"); 01008 __regFaultMask = (faultMask & 1); 01009 } 01010 01011 /** 01012 * @brief Return the Control Register value 01013 * 01014 * @return Control value 01015 * 01016 * Return the content of the control register 01017 */ 01018 static __INLINE uint32_t __get_CONTROL(void) 01019 { 01020 register uint32_t __regControl __ASM("control"); 01021 return(__regControl); 01022 } 01023 01024 /** 01025 * @brief Set the Control Register value 01026 * 01027 * @param control Control value 01028 * 01029 * Set the control register 01030 */ 01031 static __INLINE void __set_CONTROL(uint32_t control) 01032 { 01033 register uint32_t __regControl __ASM("control"); 01034 __regControl = control; 01035 } 01036 01037 #endif /* __ARMCC_VERSION */ 01038 01039 01040 01041 #elif (defined (__ICCARM__)) /*------------------ ICC Compiler -------------------*/ 01042 /* IAR iccarm specific functions */ 01043 01044 #define __enable_irq __enable_interrupt /*!< global Interrupt enable */ 01045 #define __disable_irq __disable_interrupt /*!< global Interrupt disable */ 01046 01047 static __INLINE void __enable_fault_irq() 01048 { 01049 __ASM ("cpsie f"); 01050 } 01051 static __INLINE void __disable_fault_irq() 01052 { 01053 __ASM ("cpsid f"); 01054 } 01055 01056 #define __NOP __no_operation /*!< no operation intrinsic in IAR Compiler */ 01057 static __INLINE void __WFI() 01058 { 01059 __ASM ("wfi"); 01060 } 01061 static __INLINE void __WFE() 01062 { 01063 __ASM ("wfe"); 01064 } 01065 static __INLINE void __SEV() 01066 { 01067 __ASM ("sev"); 01068 } 01069 static __INLINE void __CLREX() 01070 { 01071 __ASM ("clrex"); 01072 } 01073 01074 /* intrinsic void __ISB(void) */ 01075 /* intrinsic void __DSB(void) */ 01076 /* intrinsic void __DMB(void) */ 01077 /* intrinsic void __set_PRIMASK(); */ 01078 /* intrinsic void __get_PRIMASK(); */ 01079 /* intrinsic void __set_FAULTMASK(); */ 01080 /* intrinsic void __get_FAULTMASK(); */ 01081 /* intrinsic uint32_t __REV(uint32_t value); */ 01082 /* intrinsic uint32_t __REVSH(uint32_t value); */ 01083 /* intrinsic unsigned long __STREX(unsigned long, unsigned long); */ 01084 /* intrinsic unsigned long __LDREX(unsigned long *); */ 01085 01086 01087 /** 01088 * @brief Return the Process Stack Pointer 01089 * 01090 * @return ProcessStackPointer 01091 * 01092 * Return the actual process stack pointer 01093 */ 01094 extern uint32_t __get_PSP(void); 01095 01096 /** 01097 * @brief Set the Process Stack Pointer 01098 * 01099 * @param topOfProcStack Process Stack Pointer 01100 * 01101 * Assign the value ProcessStackPointer to the MSP 01102 * (process stack pointer) Cortex processor register 01103 */ 01104 extern void __set_PSP(uint32_t topOfProcStack); 01105 01106 /** 01107 * @brief Return the Main Stack Pointer 01108 * 01109 * @return Main Stack Pointer 01110 * 01111 * Return the current value of the MSP (main stack pointer) 01112 * Cortex processor register 01113 */ 01114 extern uint32_t __get_MSP(void); 01115 01116 /** 01117 * @brief Set the Main Stack Pointer 01118 * 01119 * @param topOfMainStack Main Stack Pointer 01120 * 01121 * Assign the value mainStackPointer to the MSP 01122 * (main stack pointer) Cortex processor register 01123 */ 01124 extern void __set_MSP(uint32_t topOfMainStack); 01125 01126 /** 01127 * @brief Reverse byte order in unsigned short value 01128 * 01129 * @param value value to reverse 01130 * @return reversed value 01131 * 01132 * Reverse byte order in unsigned short value 01133 */ 01134 extern uint32_t __REV16(uint16_t value); 01135 01136 /** 01137 * @brief Reverse bit order of value 01138 * 01139 * @param value value to reverse 01140 * @return reversed value 01141 * 01142 * Reverse bit order of value 01143 */ 01144 extern uint32_t __RBIT(uint32_t value); 01145 01146 /** 01147 * @brief LDR Exclusive (8 bit) 01148 * 01149 * @param *addr address pointer 01150 * @return value of (*address) 01151 * 01152 * Exclusive LDR command for 8 bit values) 01153 */ 01154 extern uint8_t __LDREXB(uint8_t *addr); 01155 01156 /** 01157 * @brief LDR Exclusive (16 bit) 01158 * 01159 * @param *addr address pointer 01160 * @return value of (*address) 01161 * 01162 * Exclusive LDR command for 16 bit values 01163 */ 01164 extern uint16_t __LDREXH(uint16_t *addr); 01165 01166 /** 01167 * @brief LDR Exclusive (32 bit) 01168 * 01169 * @param *addr address pointer 01170 * @return value of (*address) 01171 * 01172 * Exclusive LDR command for 32 bit values 01173 */ 01174 extern uint32_t __LDREXW(uint32_t *addr); 01175 01176 /** 01177 * @brief STR Exclusive (8 bit) 01178 * 01179 * @param value value to store 01180 * @param *addr address pointer 01181 * @return successful / failed 01182 * 01183 * Exclusive STR command for 8 bit values 01184 */ 01185 extern uint32_t __STREXB(uint8_t value, uint8_t *addr); 01186 01187 /** 01188 * @brief STR Exclusive (16 bit) 01189 * 01190 * @param value value to store 01191 * @param *addr address pointer 01192 * @return successful / failed 01193 * 01194 * Exclusive STR command for 16 bit values 01195 */ 01196 extern uint32_t __STREXH(uint16_t value, uint16_t *addr); 01197 01198 /** 01199 * @brief STR Exclusive (32 bit) 01200 * 01201 * @param value value to store 01202 * @param *addr address pointer 01203 * @return successful / failed 01204 * 01205 * Exclusive STR command for 32 bit values 01206 */ 01207 extern uint32_t __STREXW(uint32_t value, uint32_t *addr); 01208 01209 01210 01211 #elif (defined (__GNUC__)) /*------------------ GNU Compiler ---------------------*/ 01212 /* GNU gcc specific functions */ 01213 01214 static __INLINE void __enable_irq() 01215 { 01216 __ASM volatile ("cpsie i"); 01217 } 01218 static __INLINE void __disable_irq() 01219 { 01220 __ASM volatile ("cpsid i"); 01221 } 01222 01223 static __INLINE void __enable_fault_irq() 01224 { 01225 __ASM volatile ("cpsie f"); 01226 } 01227 static __INLINE void __disable_fault_irq() 01228 { 01229 __ASM volatile ("cpsid f"); 01230 } 01231 01232 static __INLINE void __NOP() 01233 { 01234 __ASM volatile ("nop"); 01235 } 01236 static __INLINE void __WFI() 01237 { 01238 __ASM volatile ("wfi"); 01239 } 01240 static __INLINE void __WFE() 01241 { 01242 __ASM volatile ("wfe"); 01243 } 01244 static __INLINE void __SEV() 01245 { 01246 __ASM volatile ("sev"); 01247 } 01248 static __INLINE void __ISB() 01249 { 01250 __ASM volatile ("isb"); 01251 } 01252 static __INLINE void __DSB() 01253 { 01254 __ASM volatile ("dsb"); 01255 } 01256 static __INLINE void __DMB() 01257 { 01258 __ASM volatile ("dmb"); 01259 } 01260 static __INLINE void __CLREX() 01261 { 01262 __ASM volatile ("clrex"); 01263 } 01264 01265 01266 /** 01267 * @brief Return the Process Stack Pointer 01268 * 01269 * @return ProcessStackPointer 01270 * 01271 * Return the actual process stack pointer 01272 */ 01273 extern uint32_t __get_PSP(void); 01274 01275 /** 01276 * @brief Set the Process Stack Pointer 01277 * 01278 * @param topOfProcStack Process Stack Pointer 01279 * 01280 * Assign the value ProcessStackPointer to the MSP 01281 * (process stack pointer) Cortex processor register 01282 */ 01283 extern void __set_PSP(uint32_t topOfProcStack); 01284 01285 /** 01286 * @brief Return the Main Stack Pointer 01287 * 01288 * @return Main Stack Pointer 01289 * 01290 * Return the current value of the MSP (main stack pointer) 01291 * Cortex processor register 01292 */ 01293 extern uint32_t __get_MSP(void); 01294 01295 /** 01296 * @brief Set the Main Stack Pointer 01297 * 01298 * @param topOfMainStack Main Stack Pointer 01299 * 01300 * Assign the value mainStackPointer to the MSP 01301 * (main stack pointer) Cortex processor register 01302 */ 01303 extern void __set_MSP(uint32_t topOfMainStack); 01304 01305 /** 01306 * @brief Return the Base Priority value 01307 * 01308 * @return BasePriority 01309 * 01310 * Return the content of the base priority register 01311 */ 01312 extern uint32_t __get_BASEPRI(void); 01313 01314 /** 01315 * @brief Set the Base Priority value 01316 * 01317 * @param basePri BasePriority 01318 * 01319 * Set the base priority register 01320 */ 01321 extern void __set_BASEPRI(uint32_t basePri); 01322 01323 /** 01324 * @brief Return the Priority Mask value 01325 * 01326 * @return PriMask 01327 * 01328 * Return state of the priority mask bit from the priority mask register 01329 */ 01330 extern uint32_t __get_PRIMASK(void); 01331 01332 /** 01333 * @brief Set the Priority Mask value 01334 * 01335 * @param priMask PriMask 01336 * 01337 * Set the priority mask bit in the priority mask register 01338 */ 01339 extern void __set_PRIMASK(uint32_t priMask); 01340 01341 /** 01342 * @brief Return the Fault Mask value 01343 * 01344 * @return FaultMask 01345 * 01346 * Return the content of the fault mask register 01347 */ 01348 extern uint32_t __get_FAULTMASK(void); 01349 01350 /** 01351 * @brief Set the Fault Mask value 01352 * 01353 * @param faultMask faultMask value 01354 * 01355 * Set the fault mask register 01356 */ 01357 extern void __set_FAULTMASK(uint32_t faultMask); 01358 01359 /** 01360 * @brief Return the Control Register value 01361 * 01362 * @return Control value 01363 * 01364 * Return the content of the control register 01365 */ 01366 extern uint32_t __get_CONTROL(void); 01367 01368 /** 01369 * @brief Set the Control Register value 01370 * 01371 * @param control Control value 01372 * 01373 * Set the control register 01374 */ 01375 extern void __set_CONTROL(uint32_t control); 01376 01377 /** 01378 * @brief Reverse byte order in integer value 01379 * 01380 * @param value value to reverse 01381 * @return reversed value 01382 * 01383 * Reverse byte order in integer value 01384 */ 01385 extern uint32_t __REV(uint32_t value); 01386 01387 /** 01388 * @brief Reverse byte order in unsigned short value 01389 * 01390 * @param value value to reverse 01391 * @return reversed value 01392 * 01393 * Reverse byte order in unsigned short value 01394 */ 01395 extern uint32_t __REV16(uint16_t value); 01396 01397 /** 01398 * @brief Reverse byte order in signed short value with sign extension to integer 01399 * 01400 * @param value value to reverse 01401 * @return reversed value 01402 * 01403 * Reverse byte order in signed short value with sign extension to integer 01404 */ 01405 extern int32_t __REVSH(int16_t value); 01406 01407 /** 01408 * @brief Reverse bit order of value 01409 * 01410 * @param value value to reverse 01411 * @return reversed value 01412 * 01413 * Reverse bit order of value 01414 */ 01415 extern uint32_t __RBIT(uint32_t value); 01416 01417 /** 01418 * @brief LDR Exclusive (8 bit) 01419 * 01420 * @param *addr address pointer 01421 * @return value of (*address) 01422 * 01423 * Exclusive LDR command for 8 bit value 01424 */ 01425 extern uint8_t __LDREXB(uint8_t *addr); 01426 01427 /** 01428 * @brief LDR Exclusive (16 bit) 01429 * 01430 * @param *addr address pointer 01431 * @return value of (*address) 01432 * 01433 * Exclusive LDR command for 16 bit values 01434 */ 01435 extern uint16_t __LDREXH(uint16_t *addr); 01436 01437 /** 01438 * @brief LDR Exclusive (32 bit) 01439 * 01440 * @param *addr address pointer 01441 * @return value of (*address) 01442 * 01443 * Exclusive LDR command for 32 bit values 01444 */ 01445 extern uint32_t __LDREXW(uint32_t *addr); 01446 01447 /** 01448 * @brief STR Exclusive (8 bit) 01449 * 01450 * @param value value to store 01451 * @param *addr address pointer 01452 * @return successful / failed 01453 * 01454 * Exclusive STR command for 8 bit values 01455 */ 01456 extern uint32_t __STREXB(uint8_t value, uint8_t *addr); 01457 01458 /** 01459 * @brief STR Exclusive (16 bit) 01460 * 01461 * @param value value to store 01462 * @param *addr address pointer 01463 * @return successful / failed 01464 * 01465 * Exclusive STR command for 16 bit values 01466 */ 01467 extern uint32_t __STREXH(uint16_t value, uint16_t *addr); 01468 01469 /** 01470 * @brief STR Exclusive (32 bit) 01471 * 01472 * @param value value to store 01473 * @param *addr address pointer 01474 * @return successful / failed 01475 * 01476 * Exclusive STR command for 32 bit values 01477 */ 01478 extern uint32_t __STREXW(uint32_t value, uint32_t *addr); 01479 01480 01481 #elif (defined (__TASKING__)) /*------------------ TASKING Compiler ---------------------*/ 01482 /* TASKING carm specific functions */ 01483 01484 /* 01485 * The CMSIS functions have been implemented as intrinsics in the compiler. 01486 * Please use "carm -?i" to get an up to date list of all instrinsics, 01487 * Including the CMSIS ones. 01488 */ 01489 01490 #endif 01491 01492 01493 /** @addtogroup CMSIS_CM3_Core_FunctionInterface CMSIS CM3 Core Function Interface 01494 Core Function Interface containing: 01495 - Core NVIC Functions 01496 - Core SysTick Functions 01497 - Core Reset Functions 01498 */ 01499 /*@{*/ 01500 01501 /* ########################## NVIC functions #################################### */ 01502 01503 /** 01504 * @brief Set the Priority Grouping in NVIC Interrupt Controller 01505 * 01506 * @param PriorityGroup is priority grouping field 01507 * 01508 * Set the priority grouping field using the required unlock sequence. 01509 * The parameter priority_grouping is assigned to the field 01510 * SCB->AIRCR [10:8] PRIGROUP field. Only values from 0..7 are used. 01511 * In case of a conflict between priority grouping and available 01512 * priority bits (__NVIC_PRIO_BITS) the smallest possible priority group is set. 01513 */ 01514 static __INLINE void NVIC_SetPriorityGrouping(uint32_t PriorityGroup) 01515 { 01516 uint32_t reg_value; 01517 uint32_t PriorityGroupTmp = (PriorityGroup & 0x07); /* only values 0..7 are used */ 01518 01519 reg_value = SCB->AIRCR; /* read old register configuration */ 01520 reg_value &= ~(SCB_AIRCR_VECTKEY_Msk | SCB_AIRCR_PRIGROUP_Msk); /* clear bits to change */ 01521 reg_value = (reg_value | 01522 (0x5FA << SCB_AIRCR_VECTKEY_Pos) | 01523 (PriorityGroupTmp << 8)); /* Insert write key and priorty group */ 01524 SCB->AIRCR = reg_value; 01525 } 01526 01527 /** 01528 * @brief Get the Priority Grouping from NVIC Interrupt Controller 01529 * 01530 * @return priority grouping field 01531 * 01532 * Get the priority grouping from NVIC Interrupt Controller. 01533 * priority grouping is SCB->AIRCR [10:8] PRIGROUP field. 01534 */ 01535 static __INLINE uint32_t NVIC_GetPriorityGrouping(void) 01536 { 01537 return ((SCB->AIRCR & SCB_AIRCR_PRIGROUP_Msk) >> SCB_AIRCR_PRIGROUP_Pos); /* read priority grouping field */ 01538 } 01539 01540 /** 01541 * @brief Enable Interrupt in NVIC Interrupt Controller 01542 * 01543 * @param IRQn The positive number of the external interrupt to enable 01544 * 01545 * Enable a device specific interupt in the NVIC interrupt controller. 01546 * The interrupt number cannot be a negative value. 01547 */ 01548 static __INLINE void NVIC_EnableIRQ(IRQn_Type IRQn) 01549 { 01550 NVIC->ISER[((uint32_t)(IRQn) >> 5)] = (1 << ((uint32_t)(IRQn) & 0x1F)); /* enable interrupt */ 01551 } 01552 01553 /** 01554 * @brief Disable the interrupt line for external interrupt specified 01555 * 01556 * @param IRQn The positive number of the external interrupt to disable 01557 * 01558 * Disable a device specific interupt in the NVIC interrupt controller. 01559 * The interrupt number cannot be a negative value. 01560 */ 01561 static __INLINE void NVIC_DisableIRQ(IRQn_Type IRQn) 01562 { 01563 NVIC->ICER[((uint32_t)(IRQn) >> 5)] = (1 << ((uint32_t)(IRQn) & 0x1F)); /* disable interrupt */ 01564 } 01565 01566 /** 01567 * @brief Read the interrupt pending bit for a device specific interrupt source 01568 * 01569 * @param IRQn The number of the device specifc interrupt 01570 * @return 1 = interrupt pending, 0 = interrupt not pending 01571 * 01572 * Read the pending register in NVIC and return 1 if its status is pending, 01573 * otherwise it returns 0 01574 */ 01575 static __INLINE uint32_t NVIC_GetPendingIRQ(IRQn_Type IRQn) 01576 { 01577 return((uint32_t) ((NVIC->ISPR[(uint32_t)(IRQn) >> 5] & (1 << ((uint32_t)(IRQn) & 0x1F)))?1:0)); /* Return 1 if pending else 0 */ 01578 } 01579 01580 /** 01581 * @brief Set the pending bit for an external interrupt 01582 * 01583 * @param IRQn The number of the interrupt for set pending 01584 * 01585 * Set the pending bit for the specified interrupt. 01586 * The interrupt number cannot be a negative value. 01587 */ 01588 static __INLINE void NVIC_SetPendingIRQ(IRQn_Type IRQn) 01589 { 01590 NVIC->ISPR[((uint32_t)(IRQn) >> 5)] = (1 << ((uint32_t)(IRQn) & 0x1F)); /* set interrupt pending */ 01591 } 01592 01593 /** 01594 * @brief Clear the pending bit for an external interrupt 01595 * 01596 * @param IRQn The number of the interrupt for clear pending 01597 * 01598 * Clear the pending bit for the specified interrupt. 01599 * The interrupt number cannot be a negative value. 01600 */ 01601 static __INLINE void NVIC_ClearPendingIRQ(IRQn_Type IRQn) 01602 { 01603 NVIC->ICPR[((uint32_t)(IRQn) >> 5)] = (1 << ((uint32_t)(IRQn) & 0x1F)); /* Clear pending interrupt */ 01604 } 01605 01606 /** 01607 * @brief Read the active bit for an external interrupt 01608 * 01609 * @param IRQn The number of the interrupt for read active bit 01610 * @return 1 = interrupt active, 0 = interrupt not active 01611 * 01612 * Read the active register in NVIC and returns 1 if its status is active, 01613 * otherwise it returns 0. 01614 */ 01615 static __INLINE uint32_t NVIC_GetActive(IRQn_Type IRQn) 01616 { 01617 return((uint32_t)((NVIC->IABR[(uint32_t)(IRQn) >> 5] & (1 << ((uint32_t)(IRQn) & 0x1F)))?1:0)); /* Return 1 if active else 0 */ 01618 } 01619 01620 /** 01621 * @brief Set the priority for an interrupt 01622 * 01623 * @param IRQn The number of the interrupt for set priority 01624 * @param priority The priority to set 01625 * 01626 * Set the priority for the specified interrupt. The interrupt 01627 * number can be positive to specify an external (device specific) 01628 * interrupt, or negative to specify an internal (core) interrupt. 01629 * 01630 * Note: The priority cannot be set for every core interrupt. 01631 */ 01632 static __INLINE void NVIC_SetPriority(IRQn_Type IRQn, uint32_t priority) 01633 { 01634 if(IRQn < 0) { 01635 SCB->SHP[((uint32_t)(IRQn) & 0xF)-4] = ((priority << (8 - __NVIC_PRIO_BITS)) & 0xff); 01636 } /* set Priority for Cortex-M3 System Interrupts */ 01637 else { 01638 NVIC->IP[(uint32_t)(IRQn)] = ((priority << (8 - __NVIC_PRIO_BITS)) & 0xff); 01639 } /* set Priority for device specific Interrupts */ 01640 } 01641 01642 /** 01643 * @brief Read the priority for an interrupt 01644 * 01645 * @param IRQn The number of the interrupt for get priority 01646 * @return The priority for the interrupt 01647 * 01648 * Read the priority for the specified interrupt. The interrupt 01649 * number can be positive to specify an external (device specific) 01650 * interrupt, or negative to specify an internal (core) interrupt. 01651 * 01652 * The returned priority value is automatically aligned to the implemented 01653 * priority bits of the microcontroller. 01654 * 01655 * Note: The priority cannot be set for every core interrupt. 01656 */ 01657 static __INLINE uint32_t NVIC_GetPriority(IRQn_Type IRQn) 01658 { 01659 01660 if(IRQn < 0) { 01661 return((uint32_t)(SCB->SHP[((uint32_t)(IRQn) & 0xF)-4] >> (8 - __NVIC_PRIO_BITS))); 01662 } /* get priority for Cortex-M3 system interrupts */ 01663 else { 01664 return((uint32_t)(NVIC->IP[(uint32_t)(IRQn)] >> (8 - __NVIC_PRIO_BITS))); 01665 } /* get priority for device specific interrupts */ 01666 } 01667 01668 01669 /** 01670 * @brief Encode the priority for an interrupt 01671 * 01672 * @param PriorityGroup The used priority group 01673 * @param PreemptPriority The preemptive priority value (starting from 0) 01674 * @param SubPriority The sub priority value (starting from 0) 01675 * @return The encoded priority for the interrupt 01676 * 01677 * Encode the priority for an interrupt with the given priority group, 01678 * preemptive priority value and sub priority value. 01679 * In case of a conflict between priority grouping and available 01680 * priority bits (__NVIC_PRIO_BITS) the samllest possible priority group is set. 01681 * 01682 * The returned priority value can be used for NVIC_SetPriority(...) function 01683 */ 01684 static __INLINE uint32_t NVIC_EncodePriority (uint32_t PriorityGroup, uint32_t PreemptPriority, uint32_t SubPriority) 01685 { 01686 uint32_t PriorityGroupTmp = (PriorityGroup & 0x07); /* only values 0..7 are used */ 01687 uint32_t PreemptPriorityBits; 01688 uint32_t SubPriorityBits; 01689 01690 PreemptPriorityBits = ((7 - PriorityGroupTmp) > __NVIC_PRIO_BITS) ? __NVIC_PRIO_BITS : 7 - PriorityGroupTmp; 01691 SubPriorityBits = ((PriorityGroupTmp + __NVIC_PRIO_BITS) < 7) ? 0 : PriorityGroupTmp - 7 + __NVIC_PRIO_BITS; 01692 01693 return ( 01694 ((PreemptPriority & ((1 << (PreemptPriorityBits)) - 1)) << SubPriorityBits) | 01695 ((SubPriority & ((1 << (SubPriorityBits )) - 1))) 01696 ); 01697 } 01698 01699 01700 /** 01701 * @brief Decode the priority of an interrupt 01702 * 01703 * @param Priority The priority for the interrupt 01704 * @param PriorityGroup The used priority group 01705 * @param pPreemptPriority The preemptive priority value (starting from 0) 01706 * @param pSubPriority The sub priority value (starting from 0) 01707 * 01708 * Decode an interrupt priority value with the given priority group to 01709 * preemptive priority value and sub priority value. 01710 * In case of a conflict between priority grouping and available 01711 * priority bits (__NVIC_PRIO_BITS) the samllest possible priority group is set. 01712 * 01713 * The priority value can be retrieved with NVIC_GetPriority(...) function 01714 */ 01715 static __INLINE void NVIC_DecodePriority (uint32_t Priority, uint32_t PriorityGroup, uint32_t* pPreemptPriority, uint32_t* pSubPriority) 01716 { 01717 uint32_t PriorityGroupTmp = (PriorityGroup & 0x07); /* only values 0..7 are used */ 01718 uint32_t PreemptPriorityBits; 01719 uint32_t SubPriorityBits; 01720 01721 PreemptPriorityBits = ((7 - PriorityGroupTmp) > __NVIC_PRIO_BITS) ? __NVIC_PRIO_BITS : 7 - PriorityGroupTmp; 01722 SubPriorityBits = ((PriorityGroupTmp + __NVIC_PRIO_BITS) < 7) ? 0 : PriorityGroupTmp - 7 + __NVIC_PRIO_BITS; 01723 01724 *pPreemptPriority = (Priority >> SubPriorityBits) & ((1 << (PreemptPriorityBits)) - 1); 01725 *pSubPriority = (Priority ) & ((1 << (SubPriorityBits )) - 1); 01726 } 01727 01728 01729 01730 /* ################################## SysTick function ############################################ */ 01731 01732 #if (!defined (__Vendor_SysTickConfig)) || (__Vendor_SysTickConfig == 0) 01733 01734 /** 01735 * @brief Initialize and start the SysTick counter and its interrupt. 01736 * 01737 * @param ticks number of ticks between two interrupts 01738 * @return 1 = failed, 0 = successful 01739 * 01740 * Initialise the system tick timer and its interrupt and start the 01741 * system tick timer / counter in free running mode to generate 01742 * periodical interrupts. 01743 */ 01744 static __INLINE uint32_t SysTick_Config(uint32_t ticks) 01745 { 01746 if (ticks > SysTick_LOAD_RELOAD_Msk) return (1); /* Reload value impossible */ 01747 01748 SysTick->LOAD = (ticks & SysTick_LOAD_RELOAD_Msk) - 1; /* set reload register */ 01749 NVIC_SetPriority (SysTick_IRQn , (1<<__NVIC_PRIO_BITS) - 1); /* set Priority for Cortex-M0 System Interrupts */ 01750 SysTick->VAL = 0; /* Load the SysTick Counter Value */ 01751 SysTick->CTRL = SysTick_CTRL_CLKSOURCE_Msk | 01752 SysTick_CTRL_TICKINT_Msk | 01753 SysTick_CTRL_ENABLE_Msk; /* Enable SysTick IRQ and SysTick Timer */ 01754 return (0); /* Function successful */ 01755 } 01756 01757 #endif 01758 01759 01760 01761 01762 /* ################################## Reset function ############################################ */ 01763 01764 /** 01765 * @brief Initiate a system reset request. 01766 * 01767 * Initiate a system reset request to reset the MCU 01768 */ 01769 static __INLINE void NVIC_SystemReset(void) 01770 { 01771 SCB->AIRCR = ((0x5FA << SCB_AIRCR_VECTKEY_Pos) | 01772 (SCB->AIRCR & SCB_AIRCR_PRIGROUP_Msk) | 01773 SCB_AIRCR_SYSRESETREQ_Msk); /* Keep priority group unchanged */ 01774 __DSB(); /* Ensure completion of memory access */ 01775 while(1); /* wait until reset */ 01776 } 01777 01778 /*@}*/ /* end of group CMSIS_CM3_Core_FunctionInterface */ 01779 01780 01781 01782 /* ##################################### Debug In/Output function ########################################### */ 01783 01784 /** @addtogroup CMSIS_CM3_CoreDebugInterface CMSIS CM3 Core Debug Interface 01785 Core Debug Interface containing: 01786 - Core Debug Receive / Transmit Functions 01787 - Core Debug Defines 01788 - Core Debug Variables 01789 */ 01790 /*@{*/ 01791 01792 extern volatile int ITM_RxBuffer ; /*!< variable to receive characters */ 01793 #define ITM_RXBUFFER_EMPTY 0x5AA55AA5 /*!< value identifying ITM_RxBuffer is ready for next character */ 01794 01795 01796 /** 01797 * @brief Outputs a character via the ITM channel 0 01798 * 01799 * @param ch character to output 01800 * @return character to output 01801 * 01802 * The function outputs a character via the ITM channel 0. 01803 * The function returns when no debugger is connected that has booked the output. 01804 * It is blocking when a debugger is connected, but the previous character send is not transmitted. 01805 */ 01806 static __INLINE uint32_t ITM_SendChar (uint32_t ch) 01807 { 01808 if ((CoreDebug->DEMCR & CoreDebug_DEMCR_TRCENA_Msk) && /* Trace enabled */ 01809 (ITM->TCR & ITM_TCR_ITMENA_Msk) && /* ITM enabled */ 01810 (ITM->TER & (1ul << 0) ) ) { /* ITM Port #0 enabled */ 01811 while (ITM->PORT[0].u32 == 0); 01812 ITM->PORT[0].u8 = (uint8_t) ch; 01813 } 01814 return (ch); 01815 } 01816 01817 01818 /** 01819 * @brief Inputs a character via variable ITM_RxBuffer 01820 * 01821 * @return received character, -1 = no character received 01822 * 01823 * The function inputs a character via variable ITM_RxBuffer. 01824 * The function returns when no debugger is connected that has booked the output. 01825 * It is blocking when a debugger is connected, but the previous character send is not transmitted. 01826 */ 01827 static __INLINE int ITM_ReceiveChar (void) 01828 { 01829 int ch = -1; /* no character available */ 01830 01831 if (ITM_RxBuffer != ITM_RXBUFFER_EMPTY) { 01832 ch = ITM_RxBuffer ; 01833 ITM_RxBuffer = ITM_RXBUFFER_EMPTY; /* ready for next character */ 01834 } 01835 01836 return (ch); 01837 } 01838 01839 01840 /** 01841 * @brief Check if a character via variable ITM_RxBuffer is available 01842 * 01843 * @return 1 = character available, 0 = no character available 01844 * 01845 * The function checks variable ITM_RxBuffer whether a character is available or not. 01846 * The function returns '1' if a character is available and '0' if no character is available. 01847 */ 01848 static __INLINE int ITM_CheckChar (void) 01849 { 01850 01851 if (ITM_RxBuffer == ITM_RXBUFFER_EMPTY) { 01852 return (0); /* no character available */ 01853 } else { 01854 return (1); /* character available */ 01855 } 01856 } 01857 01858 /*@}*/ /* end of group CMSIS_CM3_core_DebugInterface */ 01859 01860 01861 #ifdef __cplusplus 01862 } 01863 #endif 01864 01865 /*@}*/ /* end of group CMSIS_CM3_core_definitions */ 01866 01867 #endif /* __CM3_CORE_H__ */ 01868 01869 /*lint -restore */ 01870
Generated on Tue Jul 12 2022 15:16:11 by
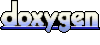