transplanted from the original URL : https://github.com/adafruit/Adafruit-RGB-LCD-Shield-Library
Embed:
(wiki syntax)
Show/hide line numbers
Adafruit_MCP23017.h
00001 /*************************************************** 00002 This is a library for the MCP23017 i2c port expander 00003 00004 These displays use I2C to communicate, 2 pins are required to 00005 interface 00006 Adafruit invests time and resources providing this open source code, 00007 please support Adafruit and open-source hardware by purchasing 00008 products from Adafruit! 00009 00010 Written by Limor Fried/Ladyada for Adafruit Industries. 00011 BSD license, all text above must be included in any redistribution 00012 ****************************************************/ 00013 00014 #ifndef _Adafruit_MCP23017_H_ 00015 #define _Adafruit_MCP23017_H_ 00016 00017 #include "mbed.h" 00018 00019 class Adafruit_MCP23017 00020 { 00021 public: 00022 Adafruit_MCP23017(I2C *master); 00023 ~Adafruit_MCP23017(){}; 00024 00025 void pinMode(uint8_t p, bool input_mode); 00026 void digitalWrite(uint8_t p, bool high); 00027 void pullUp(uint8_t p, bool pullup_anable); 00028 uint8_t digitalRead(uint8_t p); 00029 00030 void writeGPIOAB(uint16_t); 00031 uint16_t readGPIOAB(); 00032 00033 private: 00034 I2C *_master; 00035 char *_buffer; 00036 }; 00037 00038 //#define MCP23017_ADDRESS 0x20 00039 #define MCP23017_ADDRESS 0x40 00040 00041 #define INPUT 1 00042 #define OUTPUT 0 00043 00044 // registers 00045 #define MCP23017_IODIRA 0x00 00046 #define MCP23017_IPOLA 0x02 00047 #define MCP23017_GPINTENA 0x04 00048 #define MCP23017_DEFVALA 0x06 00049 #define MCP23017_INTCONA 0x08 00050 #define MCP23017_IOCONA 0x0A 00051 #define MCP23017_GPPUA 0x0C 00052 #define MCP23017_INTFA 0x0E 00053 #define MCP23017_INTCAPA 0x10 00054 #define MCP23017_GPIOA 0x12 00055 #define MCP23017_OLATA 0x14 00056 00057 00058 #define MCP23017_IODIRB 0x01 00059 #define MCP23017_IPOLB 0x03 00060 #define MCP23017_GPINTENB 0x05 00061 #define MCP23017_DEFVALB 0x07 00062 #define MCP23017_INTCONB 0x09 00063 #define MCP23017_IOCONB 0x0B 00064 #define MCP23017_GPPUB 0x0D 00065 #define MCP23017_INTFB 0x0F 00066 #define MCP23017_INTCAPB 0x11 00067 #define MCP23017_GPIOB 0x13 00068 #define MCP23017_OLATB 0x15 00069 00070 #endif 00071
Generated on Mon Jul 18 2022 20:57:22 by
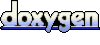