forked
mbed Namespace Reference
Parser class for parsing AT commands. More...
Data Structures | |
class | AnalogIn |
An analog input, used for reading the voltage on a pin. More... | |
class | AnalogOut |
An analog output, used for setting the voltage on a pin. More... | |
class | BusIn |
A digital input bus, used for reading the state of a collection of pins. More... | |
class | BusInOut |
A digital input output bus, used for setting the state of a collection of pins. More... | |
class | BusOut |
A digital output bus, used for setting the state of a collection of pins. More... | |
class | CANMessage |
CANMessage class. More... | |
class | CAN |
A can bus client, used for communicating with can devices. More... | |
class | DigitalIn |
A digital input, used for reading the state of a pin. More... | |
class | DigitalInOut |
A digital input/output, used for setting or reading a bi-directional pin. More... | |
class | DigitalOut |
A digital output, used for setting the state of a pin. More... | |
class | Ethernet |
An ethernet interface, to use with the ethernet pins. More... | |
class | FlashIAP |
Flash IAP driver. More... | |
class | I2C |
An I2C Master, used for communicating with I2C slave devices. More... | |
class | I2CSlave |
An I2C Slave, used for communicating with an I2C Master device. More... | |
class | InterruptIn |
A digital interrupt input, used to call a function on a rising or falling edge. More... | |
class | InterruptManager |
Use this singleton if you need to chain interrupt handlers. More... | |
class | LowPowerTicker |
Low Power Ticker. More... | |
class | LowPowerTimeout |
Low Power Timout. More... | |
class | LowPowerTimer |
Low power timer. More... | |
class | PortIn |
A multiple pin digital input. More... | |
class | PortInOut |
A multiple pin digital in/out used to set/read multiple bi-directional pins. More... | |
class | PortOut |
A multiple pin digital out. More... | |
class | PwmOut |
A pulse-width modulation digital output. More... | |
class | RawSerial |
A serial port (UART) for communication with other serial devices This is a variation of the Serial class that doesn't use streams, thus making it safe to use in interrupt handlers with the RTOS. More... | |
class | Serial |
A serial port (UART) for communication with other serial devices. More... | |
class | SerialBase |
A base class for serial port implementations Can't be instantiated directly (use Serial or RawSerial) More... | |
class | SPI |
A SPI Master, used for communicating with SPI slave devices. More... | |
class | SPISlave |
A SPI slave, used for communicating with a SPI Master device. More... | |
class | Ticker |
A Ticker is used to call a function at a recurring interval. More... | |
class | Timeout |
A Timeout is used to call a function at a point in the future. More... | |
class | Timer |
A general purpose timer. More... | |
class | TimerEvent |
Base abstraction for timer interrupts. More... | |
class | Callback< R()> |
Callback class based on template specialization. More... | |
class | Callback< R(A0)> |
Callback class based on template specialization. More... | |
class | Callback< R(A0, A1)> |
Callback class based on template specialization. More... | |
class | Callback< R(A0, A1, A2)> |
Callback class based on template specialization. More... | |
class | Callback< R(A0, A1, A2, A3)> |
Callback class based on template specialization. More... | |
class | Callback< R(A0, A1, A2, A3, A4)> |
Callback class based on template specialization. More... | |
class | CircularBuffer |
Templated Circular buffer class. More... | |
class | DirHandle |
Represents a directory stream. More... | |
class | FileHandle |
Class FileHandle. More... | |
class | FileSystemHandle |
A filesystem-like object is one that can be used to open file-like objects though it by fopen("/name/filename", mode) More... | |
class | FileSystemLike |
A filesystem-like object is one that can be used to open file-like objects though it by fopen("/name/filename", mode) More... | |
class | LocalFileSystem |
A filesystem for accessing the local mbed Microcontroller USB disk drive. More... | |
class | NonCopyable |
Inheriting from this class autogeneration of copy construction and copy assignement operations. More... | |
class | Stream |
File stream. More... | |
struct | transaction_t |
Transaction structure. More... | |
class | Transaction |
Transaction class defines a transaction. More... | |
Typedefs | |
typedef Callback< void()> * | pFunctionPointer_t |
Group one or more functions in an instance of a CallChain, then call them in sequence using CallChain::call(). | |
Functions | |
template<typename R > | |
Callback< R()> | callback (R(*func)()=0) |
Create a callback class with type infered from the arguments. | |
template<typename R > | |
Callback< R()> | callback (const Callback< R()> &func) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R > | |
Callback< R()> | callback (U *obj, R(T::*method)()) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R > | |
Callback< R()> | callback (const U *obj, R(T::*method)() const) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R > | |
Callback< R()> | callback (volatile U *obj, R(T::*method)() volatile) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R > | |
Callback< R()> | callback (const volatile U *obj, R(T::*method)() const volatile) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R > | |
Callback< R()> | callback (R(*func)(T *), U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R > | |
Callback< R()> | callback (R(*func)(const T *), const U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R > | |
Callback< R()> | callback (R(*func)(volatile T *), volatile U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R > | |
Callback< R()> | callback (R(*func)(const volatile T *), const volatile U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to callback(func, arg)") Callback< R()> callback(U *obj | |
Create a callback class with type infered from the arguments. | |
template<typename R , typename A0 > | |
Callback< R(A0)> | callback (R(*func)(A0)=0) |
Create a callback class with type infered from the arguments. | |
template<typename R , typename A0 > | |
Callback< R(A0)> | callback (const Callback< R(A0)> &func) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 > | |
Callback< R(A0)> | callback (U *obj, R(T::*method)(A0)) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 > | |
Callback< R(A0)> | callback (const U *obj, R(T::*method)(A0) const) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 > | |
Callback< R(A0)> | callback (volatile U *obj, R(T::*method)(A0) volatile) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 > | |
Callback< R(A0)> | callback (const volatile U *obj, R(T::*method)(A0) const volatile) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 > | |
Callback< R(A0)> | callback (R(*func)(T *, A0), U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 > | |
Callback< R(A0)> | callback (R(*func)(const T *, A0), const U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 > | |
Callback< R(A0)> | callback (R(*func)(volatile T *, A0), volatile U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 > | |
Callback< R(A0)> | callback (R(*func)(const volatile T *, A0), const volatile U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to callback(func, arg)") Callback< R(A0)> callback(U *obj | |
Create a callback class with type infered from the arguments. | |
template<typename R , typename A0 , typename A1 > | |
Callback< R(A0, A1)> | callback (R(*func)(A0, A1)=0) |
Create a callback class with type infered from the arguments. | |
template<typename R , typename A0 , typename A1 > | |
Callback< R(A0, A1)> | callback (const Callback< R(A0, A1)> &func) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 > | |
Callback< R(A0, A1)> | callback (U *obj, R(T::*method)(A0, A1)) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 > | |
Callback< R(A0, A1)> | callback (const U *obj, R(T::*method)(A0, A1) const) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 > | |
Callback< R(A0, A1)> | callback (volatile U *obj, R(T::*method)(A0, A1) volatile) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 > | |
Callback< R(A0, A1)> | callback (const volatile U *obj, R(T::*method)(A0, A1) const volatile) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 > | |
Callback< R(A0, A1)> | callback (R(*func)(T *, A0, A1), U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 > | |
Callback< R(A0, A1)> | callback (R(*func)(const T *, A0, A1), const U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 > | |
Callback< R(A0, A1)> | callback (R(*func)(volatile T *, A0, A1), volatile U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 > | |
Callback< R(A0, A1)> | callback (R(*func)(const volatile T *, A0, A1), const volatile U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to callback(func, arg)") Callback< R(A0 | |
Create a callback class with type infered from the arguments. | |
template<typename R , typename A0 , typename A1 , typename A2 > | |
Callback< R(A0, A1, A2)> | callback (R(*func)(A0, A1, A2)=0) |
Create a callback class with type infered from the arguments. | |
template<typename R , typename A0 , typename A1 , typename A2 > | |
Callback< R(A0, A1, A2)> | callback (const Callback< R(A0, A1, A2)> &func) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 > | |
Callback< R(A0, A1, A2)> | callback (U *obj, R(T::*method)(A0, A1, A2)) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 > | |
Callback< R(A0, A1, A2)> | callback (const U *obj, R(T::*method)(A0, A1, A2) const) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 > | |
Callback< R(A0, A1, A2)> | callback (volatile U *obj, R(T::*method)(A0, A1, A2) volatile) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 > | |
Callback< R(A0, A1, A2)> | callback (const volatile U *obj, R(T::*method)(A0, A1, A2) const volatile) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 > | |
Callback< R(A0, A1, A2)> | callback (R(*func)(T *, A0, A1, A2), U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 > | |
Callback< R(A0, A1, A2)> | callback (R(*func)(const T *, A0, A1, A2), const U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 > | |
Callback< R(A0, A1, A2)> | callback (R(*func)(volatile T *, A0, A1, A2), volatile U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 > | |
Callback< R(A0, A1, A2)> | callback (R(*func)(const volatile T *, A0, A1, A2), const volatile U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to callback(func, arg)") Callback< R(A0 | |
Create a callback class with type infered from the arguments. | |
template<typename R , typename A0 , typename A1 , typename A2 , typename A3 > | |
Callback< R(A0, A1, A2, A3)> | callback (R(*func)(A0, A1, A2, A3)=0) |
Create a callback class with type infered from the arguments. | |
template<typename R , typename A0 , typename A1 , typename A2 , typename A3 > | |
Callback< R(A0, A1, A2, A3)> | callback (const Callback< R(A0, A1, A2, A3)> &func) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 > | |
Callback< R(A0, A1, A2, A3)> | callback (U *obj, R(T::*method)(A0, A1, A2, A3)) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 > | |
Callback< R(A0, A1, A2, A3)> | callback (const U *obj, R(T::*method)(A0, A1, A2, A3) const) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 > | |
Callback< R(A0, A1, A2, A3)> | callback (volatile U *obj, R(T::*method)(A0, A1, A2, A3) volatile) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 > | |
Callback< R(A0, A1, A2, A3)> | callback (const volatile U *obj, R(T::*method)(A0, A1, A2, A3) const volatile) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 > | |
Callback< R(A0, A1, A2, A3)> | callback (R(*func)(T *, A0, A1, A2, A3), U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 > | |
Callback< R(A0, A1, A2, A3)> | callback (R(*func)(const T *, A0, A1, A2, A3), const U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 > | |
Callback< R(A0, A1, A2, A3)> | callback (R(*func)(volatile T *, A0, A1, A2, A3), volatile U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 > | |
Callback< R(A0, A1, A2, A3)> | callback (R(*func)(const volatile T *, A0, A1, A2, A3), const volatile U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to callback(func, arg)") Callback< R(A0 | |
Create a callback class with type infered from the arguments. | |
template<typename R , typename A0 , typename A1 , typename A2 , typename A3 , typename A4 > | |
Callback< R(A0, A1, A2, A3, A4)> | callback (R(*func)(A0, A1, A2, A3, A4)=0) |
Create a callback class with type infered from the arguments. | |
template<typename R , typename A0 , typename A1 , typename A2 , typename A3 , typename A4 > | |
Callback< R(A0, A1, A2, A3, A4)> | callback (const Callback< R(A0, A1, A2, A3, A4)> &func) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 , typename A4 > | |
Callback< R(A0, A1, A2, A3, A4)> | callback (U *obj, R(T::*method)(A0, A1, A2, A3, A4)) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 , typename A4 > | |
Callback< R(A0, A1, A2, A3, A4)> | callback (const U *obj, R(T::*method)(A0, A1, A2, A3, A4) const) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 , typename A4 > | |
Callback< R(A0, A1, A2, A3, A4)> | callback (volatile U *obj, R(T::*method)(A0, A1, A2, A3, A4) volatile) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 , typename A4 > | |
Callback< R(A0, A1, A2, A3, A4)> | callback (const volatile U *obj, R(T::*method)(A0, A1, A2, A3, A4) const volatile) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 , typename A4 > | |
Callback< R(A0, A1, A2, A3, A4)> | callback (R(*func)(T *, A0, A1, A2, A3, A4), U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 , typename A4 > | |
Callback< R(A0, A1, A2, A3, A4)> | callback (R(*func)(const T *, A0, A1, A2, A3, A4), const U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 , typename A4 > | |
Callback< R(A0, A1, A2, A3, A4)> | callback (R(*func)(volatile T *, A0, A1, A2, A3, A4), volatile U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 , typename A4 > | |
Callback< R(A0, A1, A2, A3, A4)> | callback (R(*func)(const volatile T *, A0, A1, A2, A3, A4), const volatile U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 , typename A4 > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to callback(func, arg)") Callback< R(A0 | |
Create a callback class with type infered from the arguments. | |
std::FILE * | fdopen (FileHandle *fh, const char *mode) |
Not a member function This call is equivalent to posix fdopen(). | |
int | poll (pollfh fhs[], unsigned nfhs, int timeout) |
A mechanism to multiplex input/output over a set of file handles(file descriptors). |
Detailed Description
Parser class for parsing AT commands.
Here are some examples:
UARTSerial serial = UARTSerial(D1, D0); ATCmdParser at = ATCmdParser(&serial, "\r\n"); int value; char buffer[100]; at.send("AT") && at.recv("OK"); at.send("AT+CWMODE=%d", 3) && at.recv("OK"); at.send("AT+CWMODE?") && at.recv("+CWMODE:%d\r\nOK", &value); at.recv("+IPD,%d:", &value); at.read(buffer, value); at.recv("OK");
Function Documentation
Callback<R()> mbed::callback | ( | R(*)() | func = 0 ) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach
- Returns:
- Callback with infered type
Definition at line 3536 of file Callback.h.
Callback<R()> mbed::callback | ( | const Callback< R()> & | func ) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach
- Returns:
- Callback with infered type
Definition at line 3546 of file Callback.h.
Callback<R()> mbed::callback | ( | const volatile U * | obj, |
R(T::*)() const volatile | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 3590 of file Callback.h.
Callback<R(A0)> mbed::callback | ( | U * | obj, |
R(T::*)(A0) | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 3726 of file Callback.h.
Callback<R(A0, A1)> mbed::callback | ( | R(*)(const T *, A0, A1) | func, |
const U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 3950 of file Callback.h.
Callback<R(A0, A1, A2, A3)> mbed::callback | ( | R(*)(const volatile T *, A0, A1, A2, A3) | func, |
const volatile U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4310 of file Callback.h.
Callback<R(A0, A1)> mbed::callback | ( | R(*)(volatile T *, A0, A1) | func, |
volatile U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 3961 of file Callback.h.
Callback<R(A0)> mbed::callback | ( | const U * | obj, |
R(T::*)(A0) const | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 3737 of file Callback.h.
Callback<R(A0, A1)> mbed::callback | ( | R(*)(const volatile T *, A0, A1) | func, |
const volatile U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 3972 of file Callback.h.
Callback<R(A0, A1, A2, A3, A4)> mbed::callback | ( | R(*)(const volatile T *, A0, A1, A2, A3, A4) | func, |
const volatile U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4479 of file Callback.h.
Callback<R(A0, A1, A2, A3, A4)> mbed::callback | ( | R(*)(volatile T *, A0, A1, A2, A3, A4) | func, |
volatile U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4468 of file Callback.h.
Callback<R(A0, A1, A2, A3, A4)> mbed::callback | ( | R(*)(const T *, A0, A1, A2, A3, A4) | func, |
const U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4457 of file Callback.h.
Callback<R(A0, A1, A2, A3, A4)> mbed::callback | ( | R(*)(T *, A0, A1, A2, A3, A4) | func, |
U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4446 of file Callback.h.
Callback<R(A0, A1, A2, A3, A4)> mbed::callback | ( | const volatile U * | obj, |
R(T::*)(A0, A1, A2, A3, A4) const volatile | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4435 of file Callback.h.
Callback<R(A0, A1, A2, A3, A4)> mbed::callback | ( | volatile U * | obj, |
R(T::*)(A0, A1, A2, A3, A4) volatile | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4424 of file Callback.h.
Callback<R(A0, A1, A2, A3, A4)> mbed::callback | ( | const U * | obj, |
R(T::*)(A0, A1, A2, A3, A4) const | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4413 of file Callback.h.
Callback<R(A0, A1, A2, A3, A4)> mbed::callback | ( | U * | obj, |
R(T::*)(A0, A1, A2, A3, A4) | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4402 of file Callback.h.
Callback<R(A0, A1, A2, A3, A4)> mbed::callback | ( | R(*)(A0, A1, A2, A3, A4) | func = 0 ) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach
- Returns:
- Callback with infered type
Definition at line 4381 of file Callback.h.
Callback<R(A0, A1, A2, A3, A4)> mbed::callback | ( | const Callback< R(A0, A1, A2, A3, A4)> & | func ) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach
- Returns:
- Callback with infered type
Definition at line 4391 of file Callback.h.
Callback<R()> mbed::callback | ( | R(*)(T *) | func, |
U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 3601 of file Callback.h.
Callback<R(A0)> mbed::callback | ( | volatile U * | obj, |
R(T::*)(A0) volatile | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 3748 of file Callback.h.
Callback<R(A0)> mbed::callback | ( | const volatile U * | obj, |
R(T::*)(A0) const volatile | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 3759 of file Callback.h.
Callback<R(A0, A1, A2, A3)> mbed::callback | ( | R(*)(volatile T *, A0, A1, A2, A3) | func, |
volatile U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4299 of file Callback.h.
Callback<R(A0, A1, A2, A3)> mbed::callback | ( | R(*)(const T *, A0, A1, A2, A3) | func, |
const U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4288 of file Callback.h.
Callback<R(A0, A1, A2, A3)> mbed::callback | ( | R(*)(T *, A0, A1, A2, A3) | func, |
U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4277 of file Callback.h.
Callback<R(A0, A1, A2, A3)> mbed::callback | ( | const volatile U * | obj, |
R(T::*)(A0, A1, A2, A3) const volatile | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4266 of file Callback.h.
Callback<R(A0, A1, A2, A3)> mbed::callback | ( | volatile U * | obj, |
R(T::*)(A0, A1, A2, A3) volatile | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4255 of file Callback.h.
Callback<R(A0, A1, A2, A3)> mbed::callback | ( | const U * | obj, |
R(T::*)(A0, A1, A2, A3) const | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4244 of file Callback.h.
Callback<R(A0, A1, A2, A3)> mbed::callback | ( | U * | obj, |
R(T::*)(A0, A1, A2, A3) | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4233 of file Callback.h.
Callback<R(A0, A1, A2, A3)> mbed::callback | ( | const Callback< R(A0, A1, A2, A3)> & | func ) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach
- Returns:
- Callback with infered type
Definition at line 4222 of file Callback.h.
Callback<R(A0, A1, A2, A3)> mbed::callback | ( | R(*)(A0, A1, A2, A3) | func = 0 ) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach
- Returns:
- Callback with infered type
Definition at line 4212 of file Callback.h.
Callback<R()> mbed::callback | ( | U * | obj, |
R(T::*)() | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 3557 of file Callback.h.
Callback<R(A0, A1, A2)> mbed::callback | ( | R(*)(const volatile T *, A0, A1, A2) | func, |
const volatile U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4141 of file Callback.h.
Callback<R()> mbed::callback | ( | R(*)(const T *) | func, |
const U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 3612 of file Callback.h.
Callback<R(A0, A1, A2)> mbed::callback | ( | R(*)(const T *, A0, A1, A2) | func, |
const U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4119 of file Callback.h.
Callback<R(A0, A1, A2)> mbed::callback | ( | R(*)(T *, A0, A1, A2) | func, |
U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4108 of file Callback.h.
Callback<R(A0, A1, A2)> mbed::callback | ( | const volatile U * | obj, |
R(T::*)(A0, A1, A2) const volatile | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4097 of file Callback.h.
Callback<R(A0, A1, A2)> mbed::callback | ( | volatile U * | obj, |
R(T::*)(A0, A1, A2) volatile | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4086 of file Callback.h.
Callback<R(A0, A1, A2)> mbed::callback | ( | const U * | obj, |
R(T::*)(A0, A1, A2) const | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4075 of file Callback.h.
Callback<R(A0, A1, A2)> mbed::callback | ( | U * | obj, |
R(T::*)(A0, A1, A2) | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4064 of file Callback.h.
Callback<R(A0)> mbed::callback | ( | R(*)(T *, A0) | func, |
U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 3770 of file Callback.h.
Callback<R(A0, A1, A2)> mbed::callback | ( | R(*)(A0, A1, A2) | func = 0 ) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach
- Returns:
- Callback with infered type
Definition at line 4043 of file Callback.h.
Callback<R(A0, A1, A2)> mbed::callback | ( | const Callback< R(A0, A1, A2)> & | func ) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach
- Returns:
- Callback with infered type
Definition at line 4053 of file Callback.h.
Callback<R(A0)> mbed::callback | ( | R(*)(const T *, A0) | func, |
const U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 3781 of file Callback.h.
Callback<R()> mbed::callback | ( | R(*)(volatile T *) | func, |
volatile U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 3623 of file Callback.h.
Callback<R(A0)> mbed::callback | ( | R(*)(volatile T *, A0) | func, |
volatile U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 3792 of file Callback.h.
Callback<R(A0)> mbed::callback | ( | R(*)(const volatile T *, A0) | func, |
const volatile U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 3803 of file Callback.h.
Callback<R()> mbed::callback | ( | const U * | obj, |
R(T::*)() const | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 3568 of file Callback.h.
Callback<R()> mbed::callback | ( | R(*)(const volatile T *) | func, |
const volatile U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 3634 of file Callback.h.
Callback<R(A0, A1)> mbed::callback | ( | R(*)(A0, A1) | func = 0 ) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach
- Returns:
- Callback with infered type
Definition at line 3874 of file Callback.h.
Callback<R(A0, A1)> mbed::callback | ( | R(*)(T *, A0, A1) | func, |
U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 3939 of file Callback.h.
Callback<R(A0, A1)> mbed::callback | ( | const volatile U * | obj, |
R(T::*)(A0, A1) const volatile | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 3928 of file Callback.h.
Callback<R(A0, A1)> mbed::callback | ( | volatile U * | obj, |
R(T::*)(A0, A1) volatile | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 3917 of file Callback.h.
Callback<R(A0, A1)> mbed::callback | ( | const U * | obj, |
R(T::*)(A0, A1) const | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 3906 of file Callback.h.
Callback<R(A0, A1)> mbed::callback | ( | U * | obj, |
R(T::*)(A0, A1) | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 3895 of file Callback.h.
Callback<R(A0, A1, A2)> mbed::callback | ( | R(*)(volatile T *, A0, A1, A2) | func, |
volatile U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4130 of file Callback.h.
Callback<R(A0, A1)> mbed::callback | ( | const Callback< R(A0, A1)> & | func ) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach
- Returns:
- Callback with infered type
Definition at line 3884 of file Callback.h.
Callback<R()> mbed::callback | ( | volatile U * | obj, |
R(T::*)() volatile | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 3579 of file Callback.h.
Callback<R(A0)> mbed::callback | ( | R(*)(A0) | func = 0 ) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach
- Returns:
- Callback with infered type
Definition at line 3705 of file Callback.h.
Callback<R(A0)> mbed::callback | ( | const Callback< R(A0)> & | func ) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach
- Returns:
- Callback with infered type
Definition at line 3715 of file Callback.h.
std::FILE * fdopen | ( | FileHandle * | fh, |
const char * | mode | ||
) |
Not a member function This call is equivalent to posix fdopen().
It associates a Stream to an already opened file descriptor (FileHandle)
- Parameters:
-
fh a pointer to an opened file descriptor mode operation upon the file descriptor, e.g., 'wb+'
- Returns:
- a pointer to std::FILE
Definition at line 36 of file FileHandle.cpp.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to callback(func, arg)" | |||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to callback(func, arg)" | |||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to callback(func, arg)" | |||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to callback(func, arg)" | |||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to callback(func, arg)" | |||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to callback(func, arg)" | |||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
int poll | ( | pollfh | fhs[], |
unsigned | nfhs, | ||
int | timeout | ||
) |
A mechanism to multiplex input/output over a set of file handles(file descriptors).
For every file handle provided, poll() examines it for any events registered for that particular file handle.
- Parameters:
-
fhs an array of PollFh struct carrying a FileHandle and bitmasks of events nfhs number of file handles timeout timer value to timeout or -1 for loop forever
- Returns:
- number of file handles selected (for which revents is non-zero). 0 if timed out with nothing selected. -1 for error.
TODO Proper wake-up mechanism. In order to correctly detect availability of read/write a FileHandle, we needed a select or poll mechanisms. We opted for poll as POSIX defines in http://pubs.opengroup.org/onlinepubs/009695399/functions/poll.html Currently, mbed::poll() just spins and scans filehandles looking for any events we are interested in. In future, his spinning behaviour will be replaced with condition variables.
Definition at line 26 of file mbed_poll.cpp.
Generated on Tue Jul 12 2022 16:02:35 by
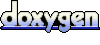