forked
Embed:
(wiki syntax)
Show/hide line numbers
Ticker.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_TICKER_H 00017 #define MBED_TICKER_H 00018 00019 #include "drivers/TimerEvent.h" 00020 #include "platform/Callback.h" 00021 #include "platform/mbed_toolchain.h" 00022 #include "platform/NonCopyable.h" 00023 00024 namespace mbed { 00025 /** \addtogroup drivers */ 00026 00027 /** A Ticker is used to call a function at a recurring interval 00028 * 00029 * You can use as many seperate Ticker objects as you require. 00030 * 00031 * @note Synchronization level: Interrupt safe 00032 * 00033 * Example: 00034 * @code 00035 * // Toggle the blinking led after 5 seconds 00036 * 00037 * #include "mbed.h" 00038 * 00039 * Ticker timer; 00040 * DigitalOut led1(LED1); 00041 * DigitalOut led2(LED2); 00042 * 00043 * int flip = 0; 00044 * 00045 * void attime() { 00046 * flip = !flip; 00047 * } 00048 * 00049 * int main() { 00050 * timer.attach(&attime, 5); 00051 * while(1) { 00052 * if(flip == 0) { 00053 * led1 = !led1; 00054 * } else { 00055 * led2 = !led2; 00056 * } 00057 * wait(0.2); 00058 * } 00059 * } 00060 * @endcode 00061 * @ingroup drivers 00062 */ 00063 class Ticker : public TimerEvent, private NonCopyable<Ticker> { 00064 00065 public: 00066 Ticker() : TimerEvent() { 00067 } 00068 00069 Ticker(const ticker_data_t *data) : TimerEvent(data) { 00070 data->interface->init(); 00071 } 00072 00073 /** Attach a function to be called by the Ticker, specifiying the interval in seconds 00074 * 00075 * @param func pointer to the function to be called 00076 * @param t the time between calls in seconds 00077 */ 00078 void attach(Callback<void()> func, float t) { 00079 attach_us(func, t * 1000000.0f); 00080 } 00081 00082 /** Attach a member function to be called by the Ticker, specifiying the interval in seconds 00083 * 00084 * @param obj pointer to the object to call the member function on 00085 * @param method pointer to the member function to be called 00086 * @param t the time between calls in seconds 00087 * @deprecated 00088 * The attach function does not support cv-qualifiers. Replaced by 00089 * attach(callback(obj, method), t). 00090 */ 00091 template<typename T, typename M> 00092 MBED_DEPRECATED_SINCE("mbed-os-5.1", 00093 "The attach function does not support cv-qualifiers. Replaced by " 00094 "attach(callback(obj, method), t).") 00095 void attach(T *obj, M method, float t) { 00096 attach(callback(obj, method), t); 00097 } 00098 00099 /** Attach a function to be called by the Ticker, specifiying the interval in micro-seconds 00100 * 00101 * @param func pointer to the function to be called 00102 * @param t the time between calls in micro-seconds 00103 */ 00104 void attach_us(Callback<void()> func, us_timestamp_t t) { 00105 _function = func; 00106 setup(t); 00107 } 00108 00109 /** Attach a member function to be called by the Ticker, specifiying the interval in micro-seconds 00110 * 00111 * @param obj pointer to the object to call the member function on 00112 * @param method pointer to the member function to be called 00113 * @param t the time between calls in micro-seconds 00114 * @deprecated 00115 * The attach_us function does not support cv-qualifiers. Replaced by 00116 * attach_us(callback(obj, method), t). 00117 */ 00118 template<typename T, typename M> 00119 MBED_DEPRECATED_SINCE("mbed-os-5.1", 00120 "The attach_us function does not support cv-qualifiers. Replaced by " 00121 "attach_us(callback(obj, method), t).") 00122 void attach_us(T *obj, M method, us_timestamp_t t) { 00123 attach_us(Callback<void()>(obj, method), t); 00124 } 00125 00126 virtual ~Ticker() { 00127 detach(); 00128 } 00129 00130 /** Detach the function 00131 */ 00132 void detach(); 00133 00134 protected: 00135 void setup(us_timestamp_t t); 00136 virtual void handler(); 00137 00138 protected: 00139 us_timestamp_t _delay; /**< Time delay (in microseconds) for re-setting the multi-shot callback. */ 00140 Callback<void()> _function; /**< Callback. */ 00141 }; 00142 00143 } // namespace mbed 00144 00145 #endif
Generated on Tue Jul 12 2022 16:02:33 by
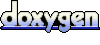