forked
Embed:
(wiki syntax)
Show/hide line numbers
CAN.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_CAN_H 00017 #define MBED_CAN_H 00018 00019 #include "platform/platform.h" 00020 00021 #if defined (DEVICE_CAN) || defined(DOXYGEN_ONLY) 00022 00023 #include "hal/can_api.h" 00024 #include "platform/Callback.h" 00025 #include "platform/PlatformMutex.h" 00026 #include "platform/NonCopyable.h" 00027 00028 namespace mbed { 00029 /** \addtogroup drivers */ 00030 00031 /** CANMessage class 00032 * 00033 * @note Synchronization level: Thread safe 00034 * @ingroup drivers 00035 */ 00036 class CANMessage : public CAN_Message { 00037 00038 public: 00039 /** Creates empty CAN message. 00040 */ 00041 CANMessage() : CAN_Message() { 00042 len = 8; 00043 type = CANData; 00044 format = CANStandard; 00045 id = 0; 00046 memset(data, 0, 8); 00047 } 00048 00049 /** Creates CAN message with specific content. 00050 * 00051 * @param _id Message ID 00052 * @param _data Mesaage Data 00053 * @param _len Message Data length 00054 * @param _type Type of Data: Use enum CANType for valid parameter values 00055 * @param _format Data Format: Use enum CANFormat for valid parameter values 00056 */ 00057 CANMessage(int _id, const char *_data, char _len = 8, CANType _type = CANData, CANFormat _format = CANStandard) { 00058 len = _len & 0xF; 00059 type = _type; 00060 format = _format; 00061 id = _id; 00062 memcpy(data, _data, _len); 00063 } 00064 00065 /** Creates CAN remote message. 00066 * 00067 * @param _id Message ID 00068 * @param _format Data Format: Use enum CANType for valid parameter values 00069 */ 00070 CANMessage(int _id, CANFormat _format = CANStandard) { 00071 len = 0; 00072 type = CANRemote; 00073 format = _format; 00074 id = _id; 00075 memset(data, 0, 8); 00076 } 00077 }; 00078 00079 /** A can bus client, used for communicating with can devices 00080 * @ingroup drivers 00081 */ 00082 class CAN : private NonCopyable<CAN> { 00083 00084 public: 00085 /** Creates an CAN interface connected to specific pins. 00086 * 00087 * @param rd read from transmitter 00088 * @param td transmit to transmitter 00089 * 00090 * Example: 00091 * @code 00092 * #include "mbed.h" 00093 * 00094 * Ticker ticker; 00095 * DigitalOut led1(LED1); 00096 * DigitalOut led2(LED2); 00097 * CAN can1(p9, p10); 00098 * CAN can2(p30, p29); 00099 * 00100 * char counter = 0; 00101 * 00102 * void send() { 00103 * if(can1.write(CANMessage(1337, &counter, 1))) { 00104 * printf("Message sent: %d\n", counter); 00105 * counter++; 00106 * } 00107 * led1 = !led1; 00108 * } 00109 * 00110 * int main() { 00111 * ticker.attach(&send, 1); 00112 * CANMessage msg; 00113 * while(1) { 00114 * if(can2.read(msg)) { 00115 * printf("Message received: %d\n\n", msg.data[0]); 00116 * led2 = !led2; 00117 * } 00118 * wait(0.2); 00119 * } 00120 * } 00121 * @endcode 00122 */ 00123 CAN(PinName rd, PinName td); 00124 00125 /** Initialize CAN interface and set the frequency 00126 * 00127 * @param rd the rd pin 00128 * @param td the td pin 00129 * @param hz the bus frequency in hertz 00130 */ 00131 CAN(PinName rd, PinName td, int hz); 00132 00133 virtual ~CAN(); 00134 00135 /** Set the frequency of the CAN interface 00136 * 00137 * @param hz The bus frequency in hertz 00138 * 00139 * @returns 00140 * 1 if successful, 00141 * 0 otherwise 00142 */ 00143 int frequency(int hz); 00144 00145 /** Write a CANMessage to the bus. 00146 * 00147 * @param msg The CANMessage to write. 00148 * 00149 * @returns 00150 * 0 if write failed, 00151 * 1 if write was successful 00152 */ 00153 int write(CANMessage msg); 00154 00155 /** Read a CANMessage from the bus. 00156 * 00157 * @param msg A CANMessage to read to. 00158 * @param handle message filter handle (0 for any message) 00159 * 00160 * @returns 00161 * 0 if no message arrived, 00162 * 1 if message arrived 00163 */ 00164 int read(CANMessage &msg, int handle = 0); 00165 00166 /** Reset CAN interface. 00167 * 00168 * To use after error overflow. 00169 */ 00170 void reset(); 00171 00172 /** Puts or removes the CAN interface into silent monitoring mode 00173 * 00174 * @param silent boolean indicating whether to go into silent mode or not 00175 */ 00176 void monitor(bool silent); 00177 00178 enum Mode { 00179 Reset = 0, 00180 Normal, 00181 Silent, 00182 LocalTest, 00183 GlobalTest, 00184 SilentTest 00185 }; 00186 00187 /** Change CAN operation to the specified mode 00188 * 00189 * @param mode The new operation mode (CAN::Normal, CAN::Silent, CAN::LocalTest, CAN::GlobalTest, CAN::SilentTest) 00190 * 00191 * @returns 00192 * 0 if mode change failed or unsupported, 00193 * 1 if mode change was successful 00194 */ 00195 int mode(Mode mode); 00196 00197 /** Filter out incomming messages 00198 * 00199 * @param id the id to filter on 00200 * @param mask the mask applied to the id 00201 * @param format format to filter on (Default CANAny) 00202 * @param handle message filter handle (Optional) 00203 * 00204 * @returns 00205 * 0 if filter change failed or unsupported, 00206 * new filter handle if successful 00207 */ 00208 int filter(unsigned int id, unsigned int mask, CANFormat format = CANAny, int handle = 0); 00209 00210 /** Detects read errors - Used to detect read overflow errors. 00211 * 00212 * @returns number of read errors 00213 */ 00214 unsigned char rderror(); 00215 00216 /** Detects write errors - Used to detect write overflow errors. 00217 * 00218 * @returns number of write errors 00219 */ 00220 unsigned char tderror(); 00221 00222 enum IrqType { 00223 RxIrq = 0, 00224 TxIrq, 00225 EwIrq, 00226 DoIrq, 00227 WuIrq, 00228 EpIrq, 00229 AlIrq, 00230 BeIrq, 00231 IdIrq, 00232 00233 IrqCnt 00234 }; 00235 00236 /** Attach a function to call whenever a CAN frame received interrupt is 00237 * generated. 00238 * 00239 * @param func A pointer to a void function, or 0 to set as none 00240 * @param type Which CAN interrupt to attach the member function to (CAN::RxIrq for message received, CAN::TxIrq for transmitted or aborted, CAN::EwIrq for error warning, CAN::DoIrq for data overrun, CAN::WuIrq for wake-up, CAN::EpIrq for error passive, CAN::AlIrq for arbitration lost, CAN::BeIrq for bus error) 00241 */ 00242 void attach(Callback<void()> func, IrqType type=RxIrq); 00243 00244 /** Attach a member function to call whenever a CAN frame received interrupt 00245 * is generated. 00246 * 00247 * @param obj pointer to the object to call the member function on 00248 * @param method pointer to the member function to be called 00249 * @param type Which CAN interrupt to attach the member function to (CAN::RxIrq for message received, TxIrq for transmitted or aborted, EwIrq for error warning, DoIrq for data overrun, WuIrq for wake-up, EpIrq for error passive, AlIrq for arbitration lost, BeIrq for bus error) 00250 * @deprecated 00251 * The attach function does not support cv-qualifiers. Replaced by 00252 * attach(callback(obj, method), type). 00253 */ 00254 template<typename T> 00255 MBED_DEPRECATED_SINCE("mbed-os-5.1", 00256 "The attach function does not support cv-qualifiers. Replaced by " 00257 "attach(callback(obj, method), type).") 00258 void attach(T* obj, void (T::*method)(), IrqType type=RxIrq) { 00259 // Underlying call thread safe 00260 attach(callback(obj, method), type); 00261 } 00262 00263 /** Attach a member function to call whenever a CAN frame received interrupt 00264 * is generated. 00265 * 00266 * @param obj pointer to the object to call the member function on 00267 * @param method pointer to the member function to be called 00268 * @param type Which CAN interrupt to attach the member function to (CAN::RxIrq for message received, TxIrq for transmitted or aborted, EwIrq for error warning, DoIrq for data overrun, WuIrq for wake-up, EpIrq for error passive, AlIrq for arbitration lost, BeIrq for bus error) 00269 * @deprecated 00270 * The attach function does not support cv-qualifiers. Replaced by 00271 * attach(callback(obj, method), type). 00272 */ 00273 template<typename T> 00274 MBED_DEPRECATED_SINCE("mbed-os-5.1", 00275 "The attach function does not support cv-qualifiers. Replaced by " 00276 "attach(callback(obj, method), type).") 00277 void attach(T* obj, void (*method)(T*), IrqType type=RxIrq) { 00278 // Underlying call thread safe 00279 attach(callback(obj, method), type); 00280 } 00281 00282 static void _irq_handler(uint32_t id, CanIrqType type); 00283 00284 protected: 00285 virtual void lock(); 00286 virtual void unlock(); 00287 can_t _can; 00288 Callback<void()> _irq[IrqCnt]; 00289 PlatformMutex _mutex; 00290 }; 00291 00292 } // namespace mbed 00293 00294 #endif 00295 00296 #endif // MBED_CAN_H 00297
Generated on Tue Jul 12 2022 16:02:31 by
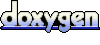