forked
Embed:
(wiki syntax)
Show/hide line numbers
ATCmdParser.h
00001 /* Copyright (c) 2017 ARM Limited 00002 * 00003 * Licensed under the Apache License, Version 2.0 (the "License"); 00004 * you may not use this file except in compliance with the License. 00005 * You may obtain a copy of the License at 00006 * 00007 * http://www.apache.org/licenses/LICENSE-2.0 00008 * 00009 * Unless required by applicable law or agreed to in writing, software 00010 * distributed under the License is distributed on an "AS IS" BASIS, 00011 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00012 * See the License for the specific language governing permissions and 00013 * limitations under the License. 00014 * 00015 * @section DESCRIPTION 00016 * 00017 * Parser for the AT command syntax 00018 * 00019 */ 00020 #ifndef MBED_ATCMDPARSER_H 00021 #define MBED_ATCMDPARSER_H 00022 00023 #include "mbed.h" 00024 #include <cstdarg> 00025 #include "Callback.h" 00026 00027 /** 00028 * Parser class for parsing AT commands 00029 * 00030 * Here are some examples: 00031 * @code 00032 * UARTSerial serial = UARTSerial(D1, D0); 00033 * ATCmdParser at = ATCmdParser(&serial, "\r\n"); 00034 * int value; 00035 * char buffer[100]; 00036 * 00037 * at.send("AT") && at.recv("OK"); 00038 * at.send("AT+CWMODE=%d", 3) && at.recv("OK"); 00039 * at.send("AT+CWMODE?") && at.recv("+CWMODE:%d\r\nOK", &value); 00040 * at.recv("+IPD,%d:", &value); 00041 * at.read(buffer, value); 00042 * at.recv("OK"); 00043 * @endcode 00044 */ 00045 00046 namespace mbed { 00047 00048 class ATCmdParser : private NonCopyable<ATCmdParser> 00049 { 00050 private: 00051 // File handle 00052 // Not owned by ATCmdParser 00053 FileHandle *_fh; 00054 00055 int _buffer_size; 00056 char *_buffer; 00057 int _timeout; 00058 00059 // Parsing information 00060 const char *_output_delimiter; 00061 int _output_delim_size; 00062 char _in_prev; 00063 bool _dbg_on; 00064 bool _aborted; 00065 00066 struct oob { 00067 unsigned len; 00068 const char *prefix; 00069 mbed::Callback<void()> cb; 00070 oob *next; 00071 }; 00072 oob *_oobs; 00073 00074 public: 00075 00076 /** 00077 * Constructor 00078 * 00079 * @param fh A FileHandle to a digital interface to use for AT commands 00080 * @param output_delimiter end of command line termination 00081 * @param buffer_size size of internal buffer for transaction 00082 * @param timeout timeout of the connection 00083 * @param debug turns on/off debug output for AT commands 00084 */ 00085 ATCmdParser(FileHandle *fh, const char *output_delimiter = "\r", 00086 int buffer_size = 256, int timeout = 8000, bool debug = false) 00087 : _fh(fh), _buffer_size(buffer_size), _in_prev(0), _oobs(NULL) 00088 { 00089 _buffer = new char[buffer_size]; 00090 set_timeout(timeout); 00091 set_delimiter(output_delimiter); 00092 debug_on(debug); 00093 } 00094 00095 /** 00096 * Destructor 00097 */ 00098 ~ATCmdParser() 00099 { 00100 while (_oobs) { 00101 struct oob *oob = _oobs; 00102 _oobs = oob->next; 00103 delete oob; 00104 } 00105 delete[] _buffer; 00106 } 00107 00108 /** 00109 * Allows timeout to be changed between commands 00110 * 00111 * @param timeout timeout of the connection 00112 */ 00113 void set_timeout(int timeout) 00114 { 00115 _timeout = timeout; 00116 } 00117 00118 /** 00119 * For backwards compatibility. 00120 * 00121 * Please use set_timeout(int) API only from now on. 00122 * Allows timeout to be changed between commands 00123 * 00124 * @param timeout timeout of the connection 00125 */ 00126 MBED_DEPRECATED_SINCE("mbed-os-5.5.0", "Replaced with set_timeout for consistency") 00127 void setTimeout(int timeout) 00128 { 00129 set_timeout(timeout); 00130 } 00131 00132 /** 00133 * Sets string of characters to use as line delimiters 00134 * 00135 * @param output_delimiter string of characters to use as line delimiters 00136 */ 00137 void set_delimiter(const char *output_delimiter) 00138 { 00139 _output_delimiter = output_delimiter; 00140 _output_delim_size = strlen(output_delimiter); 00141 } 00142 00143 /** 00144 * For backwards compatibility. 00145 * 00146 * Please use set_delimiter(const char *) API only from now on. 00147 * Sets string of characters to use as line delimiters 00148 * 00149 * @param output_delimiter string of characters to use as line delimiters 00150 */ 00151 MBED_DEPRECATED_SINCE("mbed-os-5.5.0", "Replaced with set_delimiter for consistency") 00152 void setDelimiter(const char *output_delimiter) 00153 { 00154 set_delimiter(output_delimiter); 00155 } 00156 00157 /** 00158 * Allows traces from modem to be turned on or off 00159 * 00160 * @param on set as 1 to turn on traces and vice versa. 00161 */ 00162 void debug_on(uint8_t on) 00163 { 00164 _dbg_on = (on) ? 1 : 0; 00165 } 00166 00167 /** 00168 * For backwards compatibility. 00169 * 00170 * Allows traces from modem to be turned on or off 00171 * 00172 * @param on set as 1 to turn on traces and vice versa. 00173 */ 00174 MBED_DEPRECATED_SINCE("mbed-os-5.5.0", "Replaced with debug_on for consistency") 00175 void debugOn(uint8_t on) 00176 { 00177 debug_on(on); 00178 } 00179 00180 /** 00181 * Sends an AT command 00182 * 00183 * Sends a formatted command using printf style formatting 00184 * @see printf 00185 * 00186 * @param command printf-like format string of command to send which 00187 * is appended with a newline 00188 * @param ... all printf-like arguments to insert into command 00189 * @return true only if command is successfully sent 00190 */ 00191 bool send(const char *command, ...) MBED_PRINTF_METHOD(1,2); 00192 00193 bool vsend(const char *command, va_list args); 00194 00195 /** 00196 * Receive an AT response 00197 * 00198 * Receives a formatted response using scanf style formatting 00199 * @see scanf 00200 * 00201 * Responses are parsed line at a time. 00202 * Any received data that does not match the response is ignored until 00203 * a timeout occurs. 00204 * 00205 * @param response scanf-like format string of response to expect 00206 * @param ... all scanf-like arguments to extract from response 00207 * @return true only if response is successfully matched 00208 */ 00209 bool recv(const char *response, ...) MBED_SCANF_METHOD(1,2); 00210 00211 bool vrecv(const char *response, va_list args); 00212 00213 /** 00214 * Write a single byte to the underlying stream 00215 * 00216 * @param c The byte to write 00217 * @return The byte that was written or -1 during a timeout 00218 */ 00219 int putc(char c); 00220 00221 /** 00222 * Get a single byte from the underlying stream 00223 * 00224 * @return The byte that was read or -1 during a timeout 00225 */ 00226 int getc(); 00227 00228 /** 00229 * Write an array of bytes to the underlying stream 00230 * 00231 * @param data the array of bytes to write 00232 * @param size number of bytes to write 00233 * @return number of bytes written or -1 on failure 00234 */ 00235 int write(const char *data, int size); 00236 00237 /** 00238 * Read an array of bytes from the underlying stream 00239 * 00240 * @param data the destination for the read bytes 00241 * @param size number of bytes to read 00242 * @return number of bytes read or -1 on failure 00243 */ 00244 int read(char *data, int size); 00245 00246 /** 00247 * Direct printf to underlying stream 00248 * @see printf 00249 * 00250 * @param format format string to pass to printf 00251 * @param ... arguments to printf 00252 * @return number of bytes written or -1 on failure 00253 */ 00254 int printf(const char *format, ...) MBED_PRINTF_METHOD(1,2); 00255 00256 int vprintf(const char *format, va_list args); 00257 00258 /** 00259 * Direct scanf on underlying stream 00260 * @see scanf 00261 * 00262 * @param format format string to pass to scanf 00263 * @param ... arguments to scanf 00264 * @return number of bytes read or -1 on failure 00265 */ 00266 int scanf(const char *format, ...) MBED_SCANF_METHOD(1,2); 00267 00268 int vscanf(const char *format, va_list args); 00269 00270 /** 00271 * Attach a callback for out-of-band data 00272 * 00273 * @param prefix string on when to initiate callback 00274 * @param func callback to call when string is read 00275 * @note out-of-band data is only processed during a scanf call 00276 */ 00277 void oob(const char *prefix, mbed::Callback<void()> func); 00278 00279 /** 00280 * Flushes the underlying stream 00281 */ 00282 void flush(); 00283 00284 /** 00285 * Abort current recv 00286 * 00287 * Can be called from oob handler to interrupt the current 00288 * recv operation. 00289 */ 00290 void abort(); 00291 }; 00292 } //namespace mbed 00293 00294 #endif //MBED_ATCMDPARSER_H
Generated on Tue Jul 12 2022 16:02:31 by
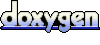