circular_buffer_f Class Reference
Classes circular_buffer_f and circular_buffer_c. More...
#include <circular_buffer.h>
Public Member Functions | |
bool | readable () |
Returns true if stuff is in buffer to be read. | |
bool | writeable () |
Returns true if space exists in buffer to write more. | |
void | init (int size, float *buffstart, int flags) |
Use init to setup buffer size, buffer mem address, and OVERWRITE_ENABLE and SEEK_ENABLE flags If OVERWRITE_ENABLE is set, allows new data to overwrite old, unread data. | |
bool | seek (int distance_back) |
If SEEK_ENABLE flag set, sets read pointer to some distance_back in prep for multiple read using get_samps. | |
bool | write (float a) |
Write places one sample into buffer. | |
bool | read (float *rd) |
Read one sample out from buffer. | |
bool | get_samps (float *dest, int len) |
get_samps reads len samples from buffer into destination array |
Detailed Description
Classes circular_buffer_f and circular_buffer_c.
Example use:-
#include "mbed.h" #include "circular_buffer.h" Serial pc(USBTX, USBRX); // Comms to Tera Term on pc Serial gizmo(p28, p27); // Serial port for gizmo on pins 27, 28 circular_buffer_c chatter; // create instance of circular_buffer_c for chars void Rx_divert (void) { // Gets attached to Serial::RxIrq interrupt handler while (gizmo.readable()) // diverting rec'd chars into purpose provided, chatter.write(gizmo.getc()); // sufficiently sized, circular buffer } main () { char buff[BUF_SIZE]; // allocate memory for circular buffer_c char x; // Use init function to initialise (no constructor functions here) chatter.init(BUF_SIZE, buff, SEEK_ENABLE | OVERWRITE_ENABLE); gizmo.attach (Rx_divert, Serial::RxIrq); // gizmo rx now diverted to circ buff // serial_in buffer now ready to use while (1) { if (chatter.readable()) { chatter.read(&x); pc.putc(x); } } }
Definition at line 41 of file circular_buffer.h.
Member Function Documentation
bool get_samps | ( | float * | dest, |
int | len | ||
) |
get_samps reads len samples from buffer into destination array
Definition at line 47 of file circular_buffer.cpp.
void init | ( | int | size, |
float * | buffstart, | ||
int | flags | ||
) |
Use init to setup buffer size, buffer mem address, and OVERWRITE_ENABLE and SEEK_ENABLE flags If OVERWRITE_ENABLE is set, allows new data to overwrite old, unread data.
If OVERWRITE_ENABLE is clear, writes to a full buffer return false and new data is lost
Definition at line 14 of file circular_buffer.cpp.
bool read | ( | float * | rd ) |
Read one sample out from buffer.
Returns false if nothing to be read, true otherwise
Definition at line 24 of file circular_buffer.cpp.
bool readable | ( | ) |
Returns true if stuff is in buffer to be read.
Definition at line 52 of file circular_buffer.h.
bool seek | ( | int | distance_back ) |
If SEEK_ENABLE flag set, sets read pointer to some distance_back in prep for multiple read using get_samps.
Definition at line 6 of file circular_buffer.cpp.
bool write | ( | float | a ) |
Write places one sample into buffer.
Returns false if buffer already full, true otherwise
Definition at line 32 of file circular_buffer.cpp.
bool writeable | ( | ) |
Returns true if space exists in buffer to write more.
Definition at line 57 of file circular_buffer.h.
Generated on Mon Jul 18 2022 17:25:53 by
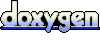