Embed:
(wiki syntax)
Show/hide line numbers
circular_buffer.h
00001 #ifndef MBED_CIRC_BUFFER_H 00002 #define MBED_CIRC_BUFFER_H 00003 00004 #include "mbed.h" 00005 00006 #define OVERWRITE_ENABLE 1 00007 #define SEEK_ENABLE 2 00008 00009 /** Classes circular_buffer_f and circular_buffer_c 00010 * 00011 * Example use:- 00012 * @code 00013 * #include "mbed.h" 00014 * #include "circular_buffer.h" 00015 * Serial pc(USBTX, USBRX); // Comms to Tera Term on pc 00016 * Serial gizmo(p28, p27); // Serial port for gizmo on pins 27, 28 00017 * circular_buffer_c chatter; // create instance of circular_buffer_c for chars 00018 * 00019 * void Rx_divert (void) { // Gets attached to Serial::RxIrq interrupt handler 00020 * while (gizmo.readable()) // diverting rec'd chars into purpose provided, 00021 * chatter.write(gizmo.getc()); // sufficiently sized, circular buffer 00022 * } 00023 * 00024 * main () { 00025 * char buff[BUF_SIZE]; // allocate memory for circular buffer_c 00026 * char x; 00027 * // Use init function to initialise (no constructor functions here) 00028 * chatter.init(BUF_SIZE, buff, SEEK_ENABLE | OVERWRITE_ENABLE); 00029 * 00030 * gizmo.attach (Rx_divert, Serial::RxIrq); // gizmo rx now diverted to circ buff 00031 * // serial_in buffer now ready to use 00032 * while (1) { 00033 * if (chatter.readable()) { 00034 * chatter.read(&x); 00035 * pc.putc(x); 00036 * } 00037 * } 00038 * } 00039 * @endcode 00040 */ 00041 class circular_buffer_f { // Circular buffer of floats 00042 float *buffbase, *Onbuff, *Offbuff, *buffend; 00043 int buffsize; 00044 bool emptyf, fullf, // Buffer full and empty flags 00045 overwrite_enable, // To allow new data to overwrite old, unread data 00046 seek_enable; // To allow read pointer repositioning 'n' behind current newest 00047 public: 00048 00049 /** Returns true if stuff is in buffer to be read 00050 * 00051 */ 00052 bool readable () {return !emptyf;} 00053 00054 /** Returns true if space exists in buffer to write more 00055 * 00056 */ 00057 bool writeable () {return !fullf;} 00058 00059 /** Use init to setup buffer size, buffer mem address, and OVERWRITE_ENABLE and SEEK_ENABLE flags 00060 * If OVERWRITE_ENABLE is set, allows new data to overwrite old, unread data. 00061 * If OVERWRITE_ENABLE is clear, writes to a full buffer return false and new data is lost 00062 */ 00063 void init (int size, float *buffstart, int flags); // Size and address of buffer to work with 00064 00065 /** If SEEK_ENABLE flag set, sets read pointer to some distance_back in prep for multiple read using get_samps 00066 * 00067 */ 00068 bool seek (int distance_back) ; // Assumes at least that much has been written to buff beforehand 00069 00070 /** Write places one sample into buffer. Returns false if buffer already full, true otherwise 00071 * 00072 */ 00073 bool write (float a) ; // Put value into circular buffer 00074 00075 /** Read one sample out from buffer. Returns false if nothing to be read, true otherwise 00076 * 00077 */ 00078 bool read (float *rd) ; 00079 00080 00081 /** get_samps reads len samples from buffer into destination array 00082 * 00083 */ 00084 bool get_samps (float *dest, int len) ; 00085 } ; // end of class circular_buffer_f 00086 00087 00088 00089 class circular_buffer_c { // Circular buffer of char 00090 char *buffbase, *Onbuff, *Offbuff, *buffend; 00091 int buffsize; 00092 bool emptyf, fullf, // Buffer full and empty flags 00093 overwrite_enable, // To allow new data to overwrite old, unread data 00094 seek_enable; // To allow read pointer repositioning 'n' behind current newest 00095 public: 00096 bool readable () {return !emptyf;} 00097 bool writeable () {return !fullf;} 00098 00099 void init (int size, char *buffstart, int flags); // Size and address of buffer to work with 00100 bool seek (int distance_back) ; // Assumes at least that much has been written to buff beforehand 00101 bool write (char a) ; // Put value into circular buffer 00102 bool read (char *rd) ; 00103 bool get_samps (char *dest, int len) ; 00104 } ; // end of class circular_buffer_c 00105 00106 #endif
Generated on Mon Jul 18 2022 17:25:53 by
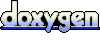