
慣性航法で用いられる座標変換をプログラムにしました。ECI座標の初期位置を設定した後、ECI,ECEF,NED,機体座標系の変換を行います。行列計算の方法や値の設定などは、ヘッダーファイル内の記述を見れば分かると思います。 また計算結果はTeratermで確認する事が出来ます。 (行列を見る場合はtoString関数、ベクトルを見る場合はtoString_V関数を使用します)
Vector.cpp
00001 #include "myConstants.h" 00002 #include "Vector.h" 00003 00004 00005 Vector::Vector(int dim) : dim(dim), components(0){ 00006 components = new float[dim]; 00007 if (!components) error("Memory Allocation Error"); 00008 for(int i=0; i<dim; i++) components[i] = 0.0f; 00009 } 00010 00011 00012 Vector::~Vector() { 00013 delete[] components; 00014 } 00015 00016 Vector::Vector(const Vector& v) : dim(v.dim), components(0) { 00017 components = new float[dim]; 00018 if (!components) error("Memory Allocation Error"); 00019 memcpy(components, v.GetpComponents(), sizeof(float)*dim); 00020 } 00021 00022 Vector& Vector::operator=(const Vector& v) { 00023 if (this == &v) return *this; 00024 dim = v.dim; 00025 delete[] components; 00026 components = new float[dim]; 00027 if (!components) error("Memory Allocation Error"); 00028 memcpy(components, v.GetpComponents(), sizeof(float)*dim); 00029 00030 return *this; 00031 } 00032 00033 Vector Vector::operator+() { 00034 return *this; 00035 } 00036 00037 Vector Vector::operator-() { 00038 Vector retVec(*this); 00039 retVec *= -1; 00040 return retVec; 00041 } 00042 00043 Vector& Vector::operator*=(float c) { 00044 for (int i = 0; i < dim; i++) { 00045 components[i] *= c; 00046 } 00047 00048 return *this; 00049 } 00050 00051 Vector& Vector::operator/=(float c) { 00052 if (fabs(c) < NEARLY_ZERO) error("Division by Zero"); 00053 for (int i = 0; i < dim; i++) { 00054 components[i] /= c; 00055 } 00056 00057 return *this; 00058 } 00059 00060 Vector& Vector::operator+=(const Vector& v) { 00061 if (dim != v.dim) error("failed to add: Irregular Dimention"); 00062 for (int i = 0; i < dim; i++) { 00063 components[i] += v.components[i]; 00064 } 00065 00066 this->CleanUp(); 00067 00068 return *this; 00069 } 00070 00071 Vector& Vector::operator-=(const Vector& v) { 00072 if (dim != v.dim) error("failed to subtract: Irregular Dimention"); 00073 for (int i = 0; i < dim; i++) { 00074 components[i] -= v.components[i]; 00075 } 00076 00077 this->CleanUp(); 00078 00079 return *this; 00080 } 00081 00082 void Vector::SetComp(int dimNo, float val) { 00083 if (dimNo > dim) error("Index Out of Bounds Error"); 00084 components[dimNo-1] = val; 00085 } 00086 00087 void Vector::SetComps(float* pComps) { 00088 memcpy(components, pComps, sizeof(float) * dim); 00089 } 00090 00091 float Vector::GetNorm() const { 00092 float norm = 0.0f; 00093 for (int i = 0; i < dim; i++) { 00094 norm += components[i] * components[i]; 00095 } 00096 return sqrt(norm); 00097 } 00098 00099 Vector Vector::Normalize() const { 00100 float norm = GetNorm(); 00101 Vector temp(*this); 00102 for (int i = 0; i < dim; i++) { 00103 temp.components[i] /= norm; 00104 } 00105 temp.CleanUp(); 00106 return temp; 00107 } 00108 00109 Vector Vector::GetParaCompTo(Vector v) { 00110 Vector norm_v = v.Normalize(); 00111 return (*this * norm_v) * norm_v; 00112 } 00113 00114 Vector Vector::GetPerpCompTo(Vector v) { 00115 return (*this - this->GetParaCompTo(v)); 00116 } 00117 00118 void Vector::CleanUp() { 00119 float maxComp = 0.0f; 00120 for (int i = 0; i < dim; i++) { 00121 if (fabs(components[i]) > maxComp) maxComp = fabs(components[i]); 00122 } 00123 if (maxComp > NEARLY_ZERO) { 00124 for (int i = 0; i < dim; i++) { 00125 if (fabs(components[i]) / maxComp < ZERO_TOLERANCE) components[i] = 0.0f; 00126 } 00127 } 00128 } 00129 00130 Vector operator+(const Vector& lhv, const Vector& rhv) { 00131 Vector retVec(lhv); 00132 retVec += rhv; 00133 return retVec; 00134 } 00135 00136 Vector operator-(const Vector& lhv, const Vector& rhv) { 00137 Vector retVec(lhv); 00138 retVec -= rhv; 00139 return retVec; 00140 } 00141 00142 Vector Cross(const Vector& lhv, const Vector& rhv) { 00143 if (lhv.GetDim() != 3) error("failed to cross: variable 'dim' must be 3"); 00144 if (lhv.GetDim() != rhv.GetDim()) error("failed to cross: Irregular Dimention"); 00145 00146 Vector retVec(lhv.GetDim()); 00147 00148 for (int i = 0; i < lhv.GetDim(); i++) { 00149 retVec.SetComp(i + 1, lhv.GetComp((i + 1) % 3 + 1) * rhv.GetComp((i + 2) % 3 + 1) 00150 - lhv.GetComp((i + 2) % 3 + 1) * rhv.GetComp((i + 1) % 3 + 1)); 00151 } 00152 00153 return retVec; 00154 } 00155 00156 Vector operator*(const float c, const Vector& rhv) { 00157 Vector retVec(rhv); 00158 retVec *= c; 00159 return retVec; 00160 } 00161 00162 Vector operator*(const Vector& lhv, const float c) { 00163 Vector retVec(lhv); 00164 retVec *= c; 00165 return retVec; 00166 } 00167 00168 float operator*(const Vector& lhv, const Vector& rhv) { 00169 if (lhv.GetDim() != rhv.GetDim()) error("Irregular Dimention"); 00170 float retVal = 0.0f; 00171 00172 for (int i = 1; i <= lhv.GetDim(); i++) { 00173 retVal += lhv.GetComp(i) * rhv.GetComp(i); 00174 } 00175 00176 return retVal; 00177 }
Generated on Wed Jul 20 2022 18:18:03 by
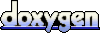