
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
unity_test_summary.py
00001 #! python3 00002 # ========================================== 00003 # Unity Project - A Test Framework for C 00004 # Copyright (c) 2015 Alexander Mueller / XelaRellum@web.de 00005 # [Released under MIT License. Please refer to license.txt for details] 00006 # Based on the ruby script by Mike Karlesky, Mark VanderVoord, Greg Williams 00007 # ========================================== 00008 import sys 00009 import os 00010 import re 00011 from glob import glob 00012 00013 class UnityTestSummary: 00014 def __init__(self): 00015 self.report = '' 00016 self.total_tests = 0 00017 self.failures = 0 00018 self.ignored = 0 00019 00020 def run(self): 00021 # Clean up result file names 00022 results = [] 00023 for target in self.targets: 00024 results.append(target.replace('\\', '/')) 00025 00026 # Dig through each result file, looking for details on pass/fail: 00027 failure_output = [] 00028 ignore_output = [] 00029 00030 for result_file in results: 00031 lines = list(map(lambda line: line.rstrip(), open(result_file, "r").read().split('\n'))) 00032 if len(lines) == 0: 00033 raise Exception("Empty test result file: %s" % result_file) 00034 00035 details = self.get_details(result_file, lines) 00036 failures = details['failures'] 00037 ignores = details['ignores'] 00038 if len(failures) > 0: failure_output.append('\n'.join(failures)) 00039 if len(ignores) > 0: ignore_output.append('n'.join(ignores)) 00040 tests,failures,ignored = self.parse_test_summary('\n'.join(lines)) 00041 self.total_tests += tests 00042 self.failures += failures 00043 self.ignored += ignored 00044 00045 if self.ignored > 0: 00046 self.report += "\n" 00047 self.report += "--------------------------\n" 00048 self.report += "UNITY IGNORED TEST SUMMARY\n" 00049 self.report += "--------------------------\n" 00050 self.report += "\n".join(ignore_output) 00051 00052 if self.failures > 0: 00053 self.report += "\n" 00054 self.report += "--------------------------\n" 00055 self.report += "UNITY FAILED TEST SUMMARY\n" 00056 self.report += "--------------------------\n" 00057 self.report += '\n'.join(failure_output) 00058 00059 self.report += "\n" 00060 self.report += "--------------------------\n" 00061 self.report += "OVERALL UNITY TEST SUMMARY\n" 00062 self.report += "--------------------------\n" 00063 self.report += "{total_tests} TOTAL TESTS {failures} TOTAL FAILURES {ignored} IGNORED\n".format(total_tests = self.total_tests, failures=self.failures, ignored=self.ignored) 00064 self.report += "\n" 00065 00066 return self.report 00067 00068 def set_targets(self, target_array): 00069 self.targets = target_array 00070 00071 def set_root_path(self, path): 00072 self.root = path 00073 00074 def usage(self, err_msg=None): 00075 print("\nERROR: ") 00076 if err_msg: 00077 print(err_msg) 00078 print("\nUsage: unity_test_summary.py result_file_directory/ root_path/") 00079 print(" result_file_directory - The location of your results files.") 00080 print(" Defaults to current directory if not specified.") 00081 print(" Should end in / if specified.") 00082 print(" root_path - Helpful for producing more verbose output if using relative paths.") 00083 sys.exit(1) 00084 00085 def get_details(self, result_file, lines): 00086 results = { 'failures': [], 'ignores': [], 'successes': [] } 00087 for line in lines: 00088 parts = line.split(':') 00089 if len(parts) != 5: 00090 continue 00091 src_file,src_line,test_name,status,msg = parts 00092 if len(self.root) > 0: 00093 line_out = "%s%s" % (self.root, line) 00094 else: 00095 line_out = line 00096 if status == 'IGNORE': 00097 results['ignores'].append(line_out) 00098 elif status == 'FAIL': 00099 results['failures'].append(line_out) 00100 elif status == 'PASS': 00101 results['successes'].append(line_out) 00102 return results 00103 00104 def parse_test_summary(self, summary): 00105 m = re.search(r"([0-9]+) Tests ([0-9]+) Failures ([0-9]+) Ignored", summary) 00106 if not m: 00107 raise Exception("Couldn't parse test results: %s" % summary) 00108 00109 return int(m.group(1)), int(m.group(2)), int(m.group(3)) 00110 00111 00112 if __name__ == '__main__': 00113 uts = UnityTestSummary() 00114 try: 00115 #look in the specified or current directory for result files 00116 if len(sys.argv) > 1: 00117 targets_dir = sys.argv[1] 00118 else: 00119 targets_dir = './' 00120 targets = list(map(lambda x: x.replace('\\', '/'), glob(targets_dir + '*.test*'))) 00121 if len(targets) == 0: 00122 raise Exception("No *.testpass or *.testfail files found in '%s'" % targets_dir) 00123 uts.set_targets(targets) 00124 00125 #set the root path 00126 if len(sys.argv) > 2: 00127 root_path = sys.argv[2] 00128 else: 00129 root_path = os.path.split(__file__)[0] 00130 uts.set_root_path(root_path) 00131 00132 #run the summarizer 00133 print(uts.run()) 00134 except Exception as e: 00135 uts.usage(e)
Generated on Tue Jul 12 2022 19:12:17 by
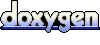