
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
pal_fileSystem.h
00001 /******************************************************************************* 00002 * Copyright 2016, 2017 ARM Ltd. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 *******************************************************************************/ 00016 #ifndef PAL_FILE_SYSTEM_H 00017 #define PAL_FILE_SYSTEM_H 00018 00019 #ifndef _PAL_H 00020 #error "Please do not include this file directly, use pal.h instead" 00021 #endif 00022 00023 /*! \file pal_fileSystem.h 00024 * \brief PAL pal_fileSystem. 00025 * This file contains the file system APIs. This is part of the PAL service API. 00026 * It provides APIs to create/remove directories and open/read/write to files. 00027 */ 00028 00029 /*! \mainpage 00030 * 00031 *\section file_sec File System 00032 * 00033 *\subsection rev_hist Revision History 00034 * 19-Jan-2017 Created and First Draft\n 00035 * 25-Jan-2017 Updated Design according to DR meeting \n 00036 * 02-Jan-2017 Minor implementation Changes \n 00037 * 00038 * 00039 * 00040 * \subsection int_sec Introduction 00041 * This file gives the user an abstraction layer for POSIX like file systems. 00042 * 00043 * 00044 * \subsection req_sec Requirements 00045 * The requirements for PAL Version 1.2 are to support the following POSIX like APIs: 00046 * 00047 * 00048 * 00049 *\b Folder \b Operations \n 00050 * -# mkdir <a href="linkURL"> http://man7.org/linux/man-pages/man2/mkdir.2.html</a> \n 00051 * -# rmdir() <a href="linkURL"> http://man7.org/linux/man-pages/man2/rmdir.2.html</a> \n 00052 * 00053 * 00054 *\b File \b Operations \n 00055 * -# fopen() <a href="linkURL"> http://man7.org/linux/man-pages/man3/fopen.3.html</a> \n 00056 * -# fclose() <a href="linkURL"> http://man7.org/linux/man-pages/man3/fclose.3.html</a> \n 00057 * -# fread() <a href="linkURL"> http://man7.org/linux/man-pages/man3/fwrite.3.html</a> \n 00058 * -# fwrite() <a href="linkURL"> http://man7.org/linux/man-pages/man3/fwrite.3.html</a> \n 00059 * -# fseek() <a href="linkURL"> http://man7.org/linux/man-pages/man3/fseek.3.html</a> \n 00060 * -# ftell() <a href="linkURL"> http://man7.org/linux/man-pages/man3/fseek.3.html</a> \n 00061 * -# unlink() <a href="linkURL"> http://man7.org/linux/man-pages/man2/unlink.2.html</a> \n 00062 * 00063 * 00064 *\b Special \b Operations\n 00065 00066 * -# rmfiles() Delete folder content (files only) (flat deletion). 00067 * -# cpfiles() Copy all files in folder to a different folder (flat copy). 00068 * 00069 * 00070 * \subsection Prerequisites 00071 * User need to set up the file system on your project and mount the proper drive if needed. \n 00072 * 00073 * 00074 * \subsection Limitations 00075 * -# File size: Up to 2 GiB.\n 00076 * -# Filename length: PAL_MAX_FILE_NAME_SIZE.\n 00077 * -# Legal characters for object name: (file/directory name) are, (0-9), (a-z), (A - Z) (_ . # ). \n 00078 * -# System is case-insensitive. \n 00079 * -# The root folder can manage a maximum of 512 entries 00080 * -# Max path length is 66 Characters. 00081 * -# Folder shall be separated with "/" 00082 * -# All folder Paths shall end with "/" 00083 00084 * 00085 * 00086 * \subsection References 00087 * PAL_FileSystemSpecification.doc 00088 */ 00089 00090 /*! @defgroup PAL_GROUP_FS 00091 * 00092 * 00093 */ 00094 /** 00095 @defgroup PAL_DEFINES PAL Services Defined Symbols & Macros 00096 @ingroup PAL_GROUP_FS 00097 */ 00098 00099 /** 00100 @defgroup PAL_ENUM PAL Services Enumerated Data Types 00101 @ingroup PAL_GROUP_FS 00102 */ 00103 00104 /** 00105 @defgroup PAL_PUBLIC_FUNCTION PAL Services Public Functions 00106 @ingroup PAL_GROUP_FS 00107 */ 00108 00109 /** 00110 @addtogroup PAL_DEFINES 00111 @{*/ 00112 00113 00114 #define PAL_MAX_FILE_NAME_SIZE 8 //!< Max length for file name received by user. 00115 #define PAL_MAX_FILE_NAME_SUFFIX 3 //!< Max length for file name suffix. 00116 #define PAL_MAX_FOLDER_DEPTH_CHAR 66 //!< Max folder length in chars. 00117 #define PAL_MAX_FILE_AND_FOLDER_LENGTH (PAL_MAX_FILE_NAME_SIZE + PAL_MAX_FILE_NAME_SUFFIX + PAL_MAX_FOLDER_DEPTH_CHAR + 1) //plus 1 is for "." 00118 #define PAL_MAX_FULL_FILE_NAME (PAL_MAX_FILE_NAME_SUFFIX + PAL_MAX_FOLDER_DEPTH_CHAR + 1) //plus 1 is for ".") 00119 00120 typedef uintptr_t palFileDescriptor_t; //!< Pointer to a generic File Descriptor object 00121 00122 /** 00123 @} */ 00124 /** 00125 @addtogroup PAL_ENUM 00126 @{*/ 00127 00128 /** \brief Enum for `fseek()` relative options. */ 00129 typedef enum { 00130 PAL_FS_OFFSET_KEEP_FIRST = 0, 00131 PAL_FS_OFFSET_SEEKSET, //!< Relative to the start of the file. 00132 PAL_FS_OFFSET_SEEKCUR, //!< The current position indicator. 00133 PAL_FS_OFFSET_SEEKEND, //!< End-of-file. 00134 PAL_FS_OFFSET_KEEP_LAST, 00135 00136 } pal_fsOffset_t; 00137 00138 /** \brief Enum for fopen() permission options*/ 00139 typedef enum { 00140 PAL_FS_FLAG_KEEP_FIRST = 0, 00141 PAL_FS_FLAG_READONLY, //!< Open file for reading. The stream is positioned at the beginning of the file (file must exist), same as "r".\n 00142 PAL_FS_FLAG_READWRITE, //!< Open for reading and writing. The stream is positioned at the beginning of the file (file must exist), same as "r+ ".\n 00143 PAL_FS_FLAG_READWRITEEXCLUSIVE, //!< Open for reading and writing exclusively. If the file already exists, `fopen()` fails. The stream is positioned at the beginning of the file. same as "w+x"\n 00144 PAL_FS_FLAG_READWRITETRUNC, //!< Open for reading and writing exclusively. If the file already exists, truncate file. The stream is positioned at the beginning of the file. same as "w+"\n 00145 PAL_FS_FLAG_KEEP_LAST, 00146 } pal_fsFileMode_t; 00147 /** 00148 @} */ 00149 00150 00151 /** \brief Enum for partition access. */ 00152 typedef enum { 00153 PAL_FS_PARTITION_PRIMARY = 0, //!< Primary partition.\n 00154 PAL_FS_PARTITION_SECONDARY, //!< Secondary partition.\n 00155 PAL_FS_PARTITION_LAST //!< Must be last value.\n 00156 } pal_fsStorageID_t; 00157 00158 00159 /** 00160 @addtogroup PAL_PUBLIC_FUNCTION 00161 @{*/ 00162 00163 /*! \brief This function attempts to create a directory named \c pathName. 00164 * 00165 00166 * @param[in] *pathName A pointer to the null-terminated string that specifies the directory name to create. 00167 00168 * 00169 * \return PAL_SUCCESS upon successful operation.\n 00170 * PAL_FILE_SYSTEM_ERROR - see error code description \c palError_t. 00171 * 00172 * \note To remove a directory, use \c PAL_ERR_FS_rmdir. 00173 * 00174 *\b Example 00175 \code{.cpp} 00176 palStatus_t ret; 00177 ret = PAL_ERR_FS_mkdir("Dir1"); 00178 if(!ret) 00179 { 00180 //Error 00181 } 00182 \endcode 00183 */ 00184 palStatus_t pal_fsMkDir(const char *pathName); 00185 00186 /*! \brief This function deletes a directory 00187 * 00188 00189 * @param[in] *pathName A pointer to the null-terminated string that specifies the directory name to be deleted. 00190 00191 * 00192 * \return PAL_SUCCESS upon successful operation.\n 00193 * PAL_FILE_SYSTEM_ERROR - see error code description \c palError_t. 00194 * 00195 00196 * \note The deleted directory \b must \b be \b empty and \b closed and the 00197 * folder path shall end with "/". 00198 00199 * 00200 *\b Example 00201 \code{.cpp} 00202 palStatus_t ret; 00203 ret = PAL_ERR_FS_mkdir("Dir1"); //Create folder name "Dir1" 00204 if(!ret) 00205 { 00206 //Error 00207 } 00208 ret = PAL_ERR_FS_rmdir("Dir1); //Remove directory from partition 00209 if(!ret) 00210 { 00211 //Error 00212 } 00213 \endcode 00214 */ 00215 palStatus_t pal_fsRmDir(const char *pathName); 00216 00217 00218 /*!\brief This function opens the file whose name is specified in the parameter `pathName` and associates it with a stream 00219 * that can be identified in future operations by the `fd` pointer returned. 00220 * 00221 * @param[out] fd The file descriptor to the file entered in the `pathName`. 00222 * @param[in] *pathName A pointer to the null-terminated string that specifies the file name to open or create. 00223 * @param[in] mode A mode flag that specifies the type of access and open method for the file. 00224 00225 * 00226 * 00227 * \return PAL_SUCCESS upon successful operation.\n 00228 * PAL_FILE_SYSTEM_ERROR - see error code description \c palError_t. 00229 * 00230 00231 * \note The folder path shall end with "/". 00232 00233 * 00234 *\b Example 00235 \code{.cpp} 00236 //Copy File from "File1" to "File2" 00237 palStatus_t ret; 00238 palFileDescriptor_t fd1 = NULL,fd2 = NULL ; // File Object 1 & 2 00239 uint8 buffer[1024]; 00240 size_t bytes_read = 0, Bytes_wrote = 0; 00241 00242 //Open first file with Read permission 00243 ret = PAL_ERR_FS_fopen(&fd1, "File1", PAL_ERR_FS_READWRITEEXCLUSIVE); 00244 if(ret) {//Error} 00245 00246 //Create second file with Read/Write permissions 00247 ret = PAL_ERR_FS_fopen(&fd2, "File2", PAL_ERR_FS_READWRITEEXCLUSIVE); 00248 if(ret) {//Error} 00249 00250 // Copy source to destination 00251 for (;;) 00252 { 00253 ret = PAL_ERR_FS_read(&fd1, buffer, sizeof(buffer), &bytes_read); // Read a chunk of source file 00254 if (ret || bytes_read == 0) break; // error or EOF 00255 ret = PAL_ERR_FS_write(&fd2, buffer, sizeof(buffer), &Bytes_wrote); // Write it to the destination file 00256 if (ret || Bytes_wrote < bytes_read) break; // error or disk full 00257 } 00258 00259 PAL_ERR_FS_close(&fd1); 00260 PAL_ERR_FS_close(&fd2); 00261 } 00262 \endcode 00263 */ 00264 palStatus_t pal_fsFopen(const char *pathName, pal_fsFileMode_t mode, 00265 palFileDescriptor_t *fd); 00266 00267 /*! \brief This function closes an open file object. 00268 * 00269 * @param[in] fd A pointer to the open file object structure to be closed. 00270 * 00271 * 00272 * \return PAL_SUCCESS upon successful operation. \n 00273 * PAL_FILE_SYSTEM_ERROR - see error code \c palError_t. 00274 * 00275 * \note When the function has completed successfully, the file object is no longer valid and it can be discarded. 00276 * 00277 */ 00278 palStatus_t pal_fsFclose(palFileDescriptor_t *fd); 00279 00280 00281 /*! \brief This function reads an array of bytes from the stream and stores it in the block of memory 00282 * specified by buffer. The position indicator of the stream is advanced by the total amount of bytes read. 00283 * 00284 * @param[in] fd A pointer to the open file object structure. 00285 * @param[in] buffer The buffer to store the read data. 00286 * @param[in] numOfBytes The number of bytes to read. 00287 * @param[out] numberOfBytesRead The number of bytes read. 00288 00289 * 00290 * \return PAL_SUCCESS upon successful operation.\n 00291 * PAL_FILE_SYSTEM_ERROR - see error code description \c palError_t. 00292 * 00293 * \note When the function has completed successfully, 00294 * `numberOfBytesRead` should be checked to detect end of the file. 00295 * If `numberOfBytesRead` is less than `numOfBytes`, 00296 * the read/write pointer has reached the end of the file during the read operation or there is an error. 00297 * 00298 */ 00299 palStatus_t pal_fsFread(palFileDescriptor_t *fd, void * buffer, 00300 size_t numOfBytes, size_t *numberOfBytesRead); 00301 00302 /*! \brief This function starts to write data from \c buffer to the file at the position pointed by the read/write pointer. 00303 * 00304 00305 * @param[in] fd A pointer to the open file object structure. 00306 * @param[in] buffer A pointer to the data to be written. 00307 * @param[in] numOfBytes The number of bytes to write. 00308 * @param[out] numberOfBytesWritten The number of bytes written. 00309 * 00310 * \return PAL_SUCCESS upon successful operation. \n 00311 * PAL_FILE_SYSTEM_ERROR - see error code \c palError_t. 00312 * 00313 * \note The read/write pointer advances as number of bytes written. When the function has completed successfully, 00314 * \note `numberOfBytesWritten` should be checked to detect the whether the disk is full. 00315 * If `numberOfBytesWritten` is less than `numOfBytes`, the volume got full during the write operation. 00316 00317 * 00318 */ 00319 palStatus_t pal_fsFwrite(palFileDescriptor_t *fd, const void * buffer, 00320 size_t numOfBytes, size_t *numberOfBytesWritten); 00321 00322 00323 /*! \brief This function moves the file read/write pointer without any read/write operation to the file. 00324 * 00325 * @param[in] fd A pointer to the open file object structure. 00326 * @param[in] offset The byte offset from the top of the file to set the read/write pointer. 00327 * @param[out] whence Where the offset is relative to. 00328 * 00329 * \return PAL_SUCCESS upon successful operation.\n 00330 * PAL_FILE_SYSTEM_ERROR - see error code description \c palError_t. 00331 * 00332 * \note The `whence` options are: \n 00333 * -# PAL_ERR_FS_SEEKSET - Relative to the start of the file. 00334 * -# PAL_ERR_FS_SEEKCUR - The current position indicator. 00335 * -# PAL_ERR_FS_SEEKEND - End-of-file. 00336 * 00337 *\b Example 00338 \code{.cpp} 00339 palStatus_t ret; 00340 palFileDescriptor_t fd1 = NULL; // File Object 1 00341 uint8 buffer[1024]; 00342 size_t bytes_read = 0, Bytes_wrote = 0; 00343 00344 //Open file with Read permission 00345 ret = PAL_ERR_FS_fopen(&fd1, "File1", PAL_ERR_FS_READ); 00346 if(ret) {//Error} 00347 00348 ret = PAL_ERR_FS_fseek(&fd1, 500, PAL_ERR_FS_SEEKSET) 00349 00350 \endcode 00351 */ 00352 palStatus_t pal_fsFseek(palFileDescriptor_t *fd, int32_t offset, 00353 pal_fsOffset_t whence); 00354 00355 /*! \brief This function gets the current read/write pointer of a file. 00356 * 00357 * @param[in] fd A pointer to the open file object structure. 00358 * 00359 * \return PAL_SUCCESS upon successful operation.\n 00360 * PAL_FILE_SYSTEM_ERROR - see error code description \c palError_t. 00361 * 00362 */ 00363 palStatus_t pal_fsFtell(palFileDescriptor_t *fd, int32_t *pos); 00364 00365 /*! \brief This function deletes a \b single file from the file system. 00366 * 00367 * @param[in] pathName A pointer to a null-terminated string that specifies the \b file to be removed. 00368 * 00369 * \return PAL_SUCCESS upon successful operation.\n 00370 * PAL_FILE_SYSTEM_ERROR - see error code description \c palError_t. 00371 * 00372 * \note The file \b must \b not \b be \b open. 00373 * 00374 */ 00375 palStatus_t pal_fsUnlink(const char *pathName); 00376 00377 /*! \brief This function deletes \b all files and folders in a folder from the file system (FLAT remove only). 00378 * 00379 * @param[in] pathName A pointer to a null-terminated string that specifies the folder. 00380 * 00381 * \return PAL_SUCCESS upon successful operation.\n 00382 * PAL_FILE_SYSTEM_ERROR - see error code description \c palError_t. 00383 * 00384 * \note The folder \b must \b not \b be \b open and the folder path must end with "/". 00385 */ 00386 palStatus_t pal_fsRmFiles(const char *pathName); 00387 00388 /*! \brief This function copies \b all files from the source folder to the destination folder (FLAT copy only). 00389 * 00390 * @param[in] pathNameSrc A pointer to a null-terminated string that specifies the source folder. 00391 * @param[in] pathNameDest A pointer to a null-terminated string that specifies the destination folder (MUST exist). 00392 * 00393 * \return PAL_SUCCESS upon successful operation.\n 00394 * PAL_FILE_SYSTEM_ERROR - see error code description \c palError_t. 00395 * 00396 * \note Both folders \b must \b not \b be \b open. If the folders do not exist, the function fails. 00397 * 00398 * 00399 */ 00400 palStatus_t pal_fsCpFolder(const char *pathNameSrc, char *pathNameDest); 00401 00402 /*! \brief This function sets the mount directory for the given storage ID (primary or secondary), 00403 * 00404 * @param[in] Path A pointer to a null-terminated string that specifies the root folder. 00405 * 00406 * \return PAL_SUCCESS upon successful operation.\n 00407 * PAL_FILE_SYSTEM_ERROR - see error code description \c palError_t. 00408 * 00409 *\note If called with NULL, the ESFS root folder is set to default PAL_SOURCE_FOLDER. 00410 *\note The folder path must end with "/". 00411 */ 00412 palStatus_t pal_fsSetMountPoint(pal_fsStorageID_t dataID, const char *Path); 00413 00414 /*! \brief This function gets the mount directory for the given storage ID (primary or secondary), The function copies the path to the user pre allocated buffer. 00415 * 00416 * @param[in] length The length of the buffer. 00417 * @param[out] Path A pointer to \b pre-allocated \b buffer with \b size \c PAL_MAX_FOLDER_DEPTH_CHAR + 1 chars. 00418 * 00419 * \return PAL_SUCCESS upon successful operation.\n 00420 * PAL_FILE_SYSTEM_ERROR - see error code description \c palError_t. 00421 * 00422 * \note The plus 1 is for the '\0' terminator at the end of the buffer. 00423 */ 00424 palStatus_t pal_fsGetMountPoint(pal_fsStorageID_t dataID, size_t length, char *Path); 00425 00426 00427 /*! \brief This function formats the SD partition indentified by the `partitionID` parameter. 00428 * 00429 * @param[in] partitionID The ID of the partition to be formatted. (**Note:** The actual partition values mapped to the IDs is determined by the porting layer.) 00430 * 00431 * \return PAL_SUCCESS upon successful operation.\n 00432 * PAL_FILE_SYSTEM_ERROR - see error code description \c palError_t. \n 00433 * PAL_ERR_INVALID_ARGUMENT - an invalid `partitionID`. 00434 */ 00435 palStatus_t pal_fsFormat(pal_fsStorageID_t dataID); 00436 00437 00438 /*! \brief This function will return if the partition used by pal only or not 00439 * 00440 * @param[in] dataID - the ID of the data to be cleared (Note: the actual partition values mapped the IDs will be determined by the porting layer) 00441 * 00442 * \return true - if partition is used only by pal.\n 00443 * false - if partition is used by other component then pal.\n 00444 */ 00445 bool pal_fsIsPrivatePartition(pal_fsStorageID_t dataID); 00446 00447 00448 /*! \brief This function will clean all file system resources 00449 */ 00450 void pal_fsCleanup(void); 00451 00452 00453 /** 00454 @} */ 00455 00456 #endif//test
Generated on Tue Jul 12 2022 19:12:14 by
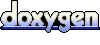