
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
mbed_trace.h File Reference
Trace interface for MbedOS applications. More...
Go to the source code of this file.
Functions | |
int | mbed_trace_init (void) |
Initialize trace functionality. | |
void | mbed_trace_free (void) |
Free trace memory. | |
void | mbed_trace_buffer_sizes (int lineLength, int tmpLength) |
Resize buffers (line / tmp ) sizes. | |
void | mbed_trace_config_set (uint8_t config) |
Set trace configurations Possible parameters: | |
uint8_t | mbed_trace_config_get (void) |
get trace configurations | |
void | mbed_trace_prefix_function_set (char *(*pref_f)(size_t)) |
Set trace prefix function pref_f -function return string with null terminated Can be used for e.g. | |
void | mbed_trace_suffix_function_set (char *(*suffix_f)(void)) |
Set trace suffix function suffix -function return string with null terminated Can be used for e.g. | |
void | mbed_trace_print_function_set (void(*print_f)(const char *)) |
Set trace print function By default, trace module print using printf() function, but with this you can write own print function, for e.g. | |
void | mbed_trace_cmdprint_function_set (void(*printf)(const char *)) |
Set trace print function for tr_cmdline() | |
void | mbed_trace_mutex_wait_function_set (void(*mutex_wait_f)(void)) |
Set trace mutex wait function By default, trace calls are not thread safe. | |
void | mbed_trace_mutex_release_function_set (void(*mutex_release_f)(void)) |
Set trace mutex release function By default, trace calls are not thread safe. | |
void | mbed_trace_exclude_filters_set (char *filters) |
When trace group contains text in filters, trace print will be ignored. | |
const char * | mbed_trace_exclude_filters_get (void) |
get trace exclude filters | |
void | mbed_trace_include_filters_set (char *filters) |
When trace group contains text in filter, trace will be printed. | |
const char * | mbed_trace_include_filters_get (void) |
get trace include filters | |
void | mbed_tracef (uint8_t dlevel, const char *grp, const char *fmt,...) __attribute__((__format__(__printf__ |
General trace function This should be used every time when user want to print out something important thing Usage e.g. | |
void | mbed_vtracef (uint8_t dlevel, const char *grp, const char *fmt, va_list ap) __attribute__((__format__(__printf__ |
General trace function This should be used every time when user want to print out something important thing and vprintf functionality is desired Usage e.g. | |
const char * | mbed_trace_last (void) |
Get last trace from buffer. | |
char * | mbed_trace_ipv6 (const void *addr_ptr) |
mbed_tracef helping function for convert ipv6 table to human readable string. | |
char * | mbed_trace_ipv6_prefix (const uint8_t *prefix, uint8_t prefix_len) |
mbed_tracef helping function for print ipv6 prefix usage e.g. | |
char * | mbed_trace_array (const uint8_t *buf, uint16_t len) |
mbed_tracef helping function for convert hex-array to string. |
Detailed Description
Trace interface for MbedOS applications.
This file provide simple but flexible way to handle software traces. Trace library are abstract layer, which use stdout (printf) by default, but outputs can be easily redirect to custom function, for example to store traces to memory or other interfaces.
usage example:
(main.c:) #include "mbed_trace.h" #define TRACE_GROUP "main" int main(void){ mbed_trace_init(); // initialize trace library tr_debug("this is debug msg"); //print debug message to stdout: "[DBG] tr_info("this is info msg"); tr_warn("this is warning msg"); tr_err("this is error msg"); return 0; }
Activate with compiler flag: YOTTA_CFG_MBED_TRACE Configure trace line buffer size with compiler flag: YOTTA_CFG_MBED_TRACE_LINE_LENGTH. Default length: 1024. Limit the size of flash by setting MBED_TRACE_MAX_LEVEL value. Default is TRACE_LEVEL_DEBUG (all included)
Definition in file mbed_trace.h.
Function Documentation
char* mbed_trace_array | ( | const uint8_t * | buf, |
uint16_t | len | ||
) |
mbed_tracef helping function for convert hex-array to string.
usage e.g. char myarr[] = {0x10, 0x20}; mbed_tracef(TRACE_LEVEL_INFO, "mygr", "arr: %s", mbed_trace_array(myarr, 2));
- Parameters:
-
buf hex array pointer len buffer length
- Returns:
- temporary buffer where string copied if array as string not fit to temp buffer, this function write '*' as last character, which indicate that buffer is too small for array.
Acquire mutex. It is released before returning from mbed_vtracef.
Definition at line 551 of file mbed_trace.c.
void mbed_trace_buffer_sizes | ( | int | lineLength, |
int | tmpLength | ||
) |
Resize buffers (line / tmp ) sizes.
- Parameters:
-
lineLength new maximum length for trace line (0 = do no resize) tmpLength new maximum length for trace tmp buffer (used for trace_array, etc) (0 = do no resize)
Definition at line 218 of file mbed_trace.c.
void mbed_trace_cmdprint_function_set | ( | void(*)(const char *) | printf ) |
Set trace print function for tr_cmdline()
Definition at line 248 of file mbed_trace.c.
uint8_t mbed_trace_config_get | ( | void | ) |
get trace configurations
- Returns:
- trace configuration byte
Definition at line 232 of file mbed_trace.c.
void mbed_trace_config_set | ( | uint8_t | config ) |
Set trace configurations Possible parameters:
TRACE_MODE_COLOR TRACE_MODE_PLAIN (this exclude color mode) TRACE_CARRIAGE_RETURN (print CR before trace line)
TRACE_ACTIVE_LEVEL_ALL - to activate all trace levels or TRACE_ACTIVE_LEVEL_DEBUG (alternative) TRACE_ACTIVE_LEVEL_INFO TRACE_ACTIVE_LEVEL_WARN TRACE_ACTIVE_LEVEL_ERROR TRACE_ACTIVE_LEVEL_CMD TRACE_LEVEL_NONE - to deactivate all traces
- Parameters:
-
config Byte size Bit-mask. Bits are descripted above. usage e.g. mbed_trace_config_set( TRACE_ACTIVE_LEVEL_ALL|TRACE_MODE_COLOR );
Definition at line 228 of file mbed_trace.c.
const char* mbed_trace_exclude_filters_get | ( | void | ) |
get trace exclude filters
Definition at line 268 of file mbed_trace.c.
void mbed_trace_exclude_filters_set | ( | char * | filters ) |
When trace group contains text in filters, trace print will be ignored.
e.g.: mbed_trace_exclude_filters_set("mygr"); mbed_tracef(TRACE_ACTIVE_LEVEL_DEBUG, "ougr", "This is not printed");
Definition at line 260 of file mbed_trace.c.
void mbed_trace_free | ( | void | ) |
Free trace memory.
Definition at line 187 of file mbed_trace.c.
const char* mbed_trace_include_filters_get | ( | void | ) |
get trace include filters
Definition at line 272 of file mbed_trace.c.
void mbed_trace_include_filters_set | ( | char * | filters ) |
When trace group contains text in filter, trace will be printed.
e.g.: set_trace_include_filters("mygr"); mbed_tracef(TRACE_ACTIVE_LEVEL_DEBUG, "mygr", "Hi There"); mbed_tracef(TRACE_ACTIVE_LEVEL_DEBUG, "grp2", "This is not printed");
Definition at line 276 of file mbed_trace.c.
int mbed_trace_init | ( | void | ) |
Initialize trace functionality.
- Returns:
- 0 when all success, otherwise non zero
Definition at line 154 of file mbed_trace.c.
char* mbed_trace_ipv6 | ( | const void * | addr_ptr ) |
mbed_tracef helping function for convert ipv6 table to human readable string.
usage e.g. char ipv6[16] = {...}; // ! array length is 16 bytes ! mbed_tracef(TRACE_LEVEL_INFO, "mygr", "ipv6 addr: %s", mbed_trace_ipv6(ipv6));
- Parameters:
-
add_ptr IPv6 Address pointer
- Returns:
- temporary buffer where ipv6 is in string format
Acquire mutex. It is released before returning from mbed_vtracef.
Definition at line 507 of file mbed_trace.c.
char* mbed_trace_ipv6_prefix | ( | const uint8_t * | prefix, |
uint8_t | prefix_len | ||
) |
mbed_tracef helping function for print ipv6 prefix usage e.g.
char ipv6[16] = {...}; // ! array length is 16 bytes ! mbed_tracef(TRACE_LEVEL_INFO, "mygr", "ipv6 addr: %s", mbed_trace_ipv6_prefix(ipv6, 4));
- Parameters:
-
prefix IPv6 Address pointer prefix_len prefix length
- Returns:
- temporary buffer where ipv6 is in string format
Acquire mutex. It is released before returning from mbed_vtracef.
Definition at line 528 of file mbed_trace.c.
const char* mbed_trace_last | ( | void | ) |
Get last trace from buffer.
Definition at line 500 of file mbed_trace.c.
void mbed_trace_mutex_release_function_set | ( | void(*)(void) | mutex_release_f ) |
Set trace mutex release function By default, trace calls are not thread safe.
If thread safety is required this can be used to set a callback function that will be called before returning from each trace call. The specific implementation is up to the application developer, but the mutex must count so it can be acquired from a single thread repeatedly.
Definition at line 256 of file mbed_trace.c.
void mbed_trace_mutex_wait_function_set | ( | void(*)(void) | mutex_wait_f ) |
Set trace mutex wait function By default, trace calls are not thread safe.
If thread safety is required this can be used to set a callback function that will be called before each trace call. The specific implementation is up to the application developer, but the mutex must count so it can be acquired from a single thread repeatedly.
Definition at line 252 of file mbed_trace.c.
void mbed_trace_prefix_function_set | ( | char *(*)(size_t) | pref_f ) |
Set trace prefix function pref_f -function return string with null terminated Can be used for e.g.
time string e.g. char* trace_time(){ return "rtc-time-in-string"; } mbed_trace_prefix_function_set( &trace_time );
Definition at line 236 of file mbed_trace.c.
void mbed_trace_print_function_set | ( | void(*)(const char *) | print_f ) |
Set trace print function By default, trace module print using printf() function, but with this you can write own print function, for e.g.
to other IO device.
Definition at line 244 of file mbed_trace.c.
void mbed_trace_suffix_function_set | ( | char *(*)(void) | suffix_f ) |
Set trace suffix function suffix -function return string with null terminated Can be used for e.g.
time string e.g. char* trace_suffix(){ return " END"; } mbed_trace_suffix_function_set( &trace_suffix );
Definition at line 240 of file mbed_trace.c.
void void mbed_tracef | ( | uint8_t | dlevel, |
const char * | grp, | ||
const char * | fmt, | ||
... | |||
) |
General trace function This should be used every time when user want to print out something important thing Usage e.g.
mbed_tracef( TRACE_LEVEL_INFO, "mygr", "Hello world!");
- Parameters:
-
dlevel debug level grp trace group fmt trace format (like printf) ... variable arguments related to fmt
void void mbed_vtracef | ( | uint8_t | dlevel, |
const char * | grp, | ||
const char * | fmt, | ||
va_list | ap | ||
) |
General trace function This should be used every time when user want to print out something important thing and vprintf functionality is desired Usage e.g.
va_list ap; va_start (ap, fmt); mbed_vtracef( TRACE_LEVEL_INFO, "mygr", fmt, ap ); va_end (ap);
- Parameters:
-
dlevel debug level grp trace group fmt trace format (like vprintf) ap variable arguments list (like vprintf)
Generated on Tue Jul 12 2022 19:12:17 by
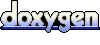