
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
mbed-trace-helper.c
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // Licensed under the Apache License, Version 2.0 (the "License"); 00005 // you may not use this file except in compliance with the License. 00006 // You may obtain a copy of the License at 00007 // 00008 // http://www.apache.org/licenses/LICENSE-2.0 00009 // 00010 // Unless required by applicable law or agreed to in writing, software 00011 // distributed under the License is distributed on an "AS IS" BASIS, 00012 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 // See the License for the specific language governing permissions and 00014 // limitations under the License. 00015 // ---------------------------------------------------------------------------- 00016 00017 #include "pv_log.h" 00018 #include <stdarg.h> 00019 #include <inttypes.h> 00020 #include <stdlib.h> 00021 #include "pal.h" 00022 #include "pv_error_handling.h" 00023 #include "mbed-trace/mbed_trace.h" 00024 /** 00025 * Mutex for printing logs in a thread safe manner. 00026 */ 00027 palMutexID_t g_pv_logger_mutex = NULLPTR; 00028 00029 void mbed_trace_helper_print(const char* format) 00030 { 00031 fprintf(stdout, "%s\n", format); 00032 } 00033 00034 void mbed_trace_helper_mutex_wait() 00035 { 00036 (void)pal_osMutexWait(g_pv_logger_mutex, PAL_RTOS_WAIT_FOREVER); 00037 } 00038 00039 void mbed_trace_helper_mutex_release() 00040 { 00041 (void)pal_osMutexRelease(g_pv_logger_mutex); 00042 } 00043 /** 00044 * Creates mutex 00045 */ 00046 bool mbed_trace_helper_create_mutex(void) 00047 { 00048 palStatus_t status; 00049 00050 // g_pv_logger_mutex already created - no need to recreate it. 00051 if (g_pv_logger_mutex) { 00052 goto exit; 00053 } 00054 00055 status = pal_osMutexCreate(&g_pv_logger_mutex); 00056 if (status != PAL_SUCCESS) { 00057 SA_PV_LOG_INFO("Error creating g_pv_logger_mutex (pal err = %d)", (int)status); 00058 return false; 00059 } 00060 00061 exit: 00062 return true; 00063 } 00064 00065 /** 00066 * Deletes mutex 00067 */ 00068 void mbed_trace_helper_delete_mutex(void) 00069 { 00070 // g_pv_logger_mutex already created - no need to recreate it. 00071 if (g_pv_logger_mutex == NULLPTR) { 00072 return; 00073 } 00074 00075 pal_osMutexDelete(&g_pv_logger_mutex); 00076 g_pv_logger_mutex = NULLPTR; 00077 } 00078 00079 uint8_t mbed_trace_helper_check_activated_trace_level() 00080 { 00081 uint8_t config_active_level = 0; 00082 uint8_t activated_level = 0; 00083 00084 SA_PV_LOG_INFO_FUNC_ENTER("MBED_TRACE_MAX_LEVEL = %d", MBED_TRACE_MAX_LEVEL); 00085 00086 config_active_level = mbed_trace_config_get() & TRACE_MASK_LEVEL; 00087 SA_PV_LOG_INFO("config_active_level is %d", config_active_level); 00088 00089 activated_level = config_active_level & MBED_TRACE_MAX_LEVEL; 00090 SA_PV_LOG_INFO("activated_level is %d", activated_level); 00091 00092 if (activated_level == 0) { 00093 SA_PV_LOG_CRITICAL("The compiled maximum trace level %d, is higher than activated trace level", MBED_TRACE_MAX_LEVEL); 00094 SA_PV_LOG_CRITICAL("If you want to use the requested log level, please change MBED_TRACE_MAX_LEVEL compilation flag and recompile the code"); 00095 } 00096 00097 SA_PV_LOG_INFO_FUNC_EXIT_NO_ARGS(); 00098 00099 return activated_level; 00100 } 00101 00102 bool mbed_trace_helper_init(uint8_t config, bool is_mutex_used) 00103 { 00104 bool success = true; 00105 int rc = 0; 00106 00107 rc = mbed_trace_init(); 00108 00109 if (rc != 0) { 00110 return false; 00111 } 00112 00113 if (is_mutex_used) { 00114 // Create mutex 00115 success = mbed_trace_helper_create_mutex(); 00116 if (success != true) { 00117 mbed_trace_free(); 00118 return false; 00119 } 00120 } 00121 // Set trace level, TRACE_MODE_PLAIN used to ignore mbed trace print pattern ([trace_level] [trace_group] format) 00122 mbed_trace_config_set(config); 00123 00124 // Set trace print function 00125 mbed_trace_print_function_set(mbed_trace_helper_print); 00126 00127 if (is_mutex_used) { 00128 // Set mutex wait function for mbed trace 00129 mbed_trace_mutex_wait_function_set(mbed_trace_helper_mutex_wait); 00130 // Set mutex release function for mbed trace 00131 mbed_trace_mutex_release_function_set(mbed_trace_helper_mutex_release); 00132 } 00133 return true; 00134 } 00135 00136 void mbed_trace_helper_finish() 00137 { 00138 mbed_trace_helper_delete_mutex(); 00139 mbed_trace_free(); 00140 } 00141 00142 00143
Generated on Tue Jul 12 2022 19:12:13 by
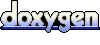