
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
m2mresourceinstance.cpp
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include <stdlib.h> 00017 #include "mbed-client/m2mresource.h" 00018 #include "mbed-client/m2mconstants.h" 00019 #include "mbed-client/m2mobservationhandler.h" 00020 #include "mbed-client/m2mobject.h" 00021 #include "mbed-client/m2mobjectinstance.h" 00022 #include "include/m2mcallbackstorage.h" 00023 #include "include/m2mreporthandler.h" 00024 #include "mbed-client/m2mblockmessage.h" 00025 #include "mbed-trace/mbed_trace.h" 00026 00027 #define TRACE_GROUP "mClt" 00028 00029 M2MResourceInstance::M2MResourceInstance(M2MResource &parent, 00030 const String &res_name, 00031 M2MBase::Mode resource_mode, 00032 const String &resource_type, 00033 M2MBase::DataType type, 00034 char* path, 00035 bool external_blockwise_store, 00036 bool multiple_instance) 00037 : M2MResourceBase(res_name, 00038 resource_mode, 00039 resource_type, 00040 type, 00041 path, 00042 external_blockwise_store, 00043 multiple_instance 00044 ), 00045 _parent_resource(parent) 00046 { 00047 set_base_type(M2MBase::ResourceInstance); 00048 } 00049 00050 M2MResourceInstance::M2MResourceInstance(M2MResource &parent, 00051 const String &res_name, 00052 M2MBase::Mode resource_mode, 00053 const String &resource_type, 00054 M2MBase::DataType type, 00055 const uint8_t *value, 00056 const uint8_t value_length, 00057 char* path, 00058 bool external_blockwise_store, 00059 bool multiple_instance) 00060 : M2MResourceBase(res_name, 00061 resource_mode, 00062 resource_type, 00063 type, 00064 value, 00065 value_length, 00066 path, 00067 external_blockwise_store, 00068 multiple_instance), 00069 _parent_resource(parent) 00070 { 00071 set_base_type(M2MBase::ResourceInstance); 00072 } 00073 00074 M2MResourceInstance::M2MResourceInstance(M2MResource &parent, 00075 const lwm2m_parameters_s* s, 00076 M2MBase::DataType type) 00077 : M2MResourceBase(s, type), 00078 _parent_resource(parent) 00079 { 00080 00081 assert(base_type() == M2MBase::ResourceInstance); 00082 } 00083 00084 M2MResourceInstance::~M2MResourceInstance() 00085 { 00086 free_resources(); 00087 } 00088 00089 M2MObservationHandler* M2MResourceInstance::observation_handler() const 00090 { 00091 const M2MResource& parent_resource = get_parent_resource(); 00092 00093 // XXX: need to check the flag too 00094 return parent_resource.observation_handler(); 00095 } 00096 00097 void M2MResourceInstance::set_observation_handler(M2MObservationHandler *handler) 00098 { 00099 M2MResource& parent_resource = get_parent_resource(); 00100 00101 // XXX: need to set the flag too 00102 parent_resource.set_observation_handler(handler); 00103 } 00104 00105 bool M2MResourceInstance::handle_observation_attribute(const char *query) 00106 { 00107 tr_debug("M2MResourceInstance::handle_observation_attribute - is_under_observation(%d)", is_under_observation()); 00108 bool success = false; 00109 00110 M2MReportHandler *handler = M2MBase::report_handler(); 00111 if (!handler) { 00112 handler = M2MBase::create_report_handler(); 00113 } 00114 00115 if (handler) { 00116 success = handler->parse_notification_attribute(query, 00117 M2MBase::base_type(), resource_instance_type()); 00118 if(success) { 00119 if (is_under_observation()) { 00120 handler->set_under_observation(true); 00121 } 00122 } else { 00123 handler->set_default_values(); 00124 } 00125 } 00126 return success; 00127 } 00128 00129 uint16_t M2MResourceInstance::object_instance_id() const 00130 { 00131 const M2MObjectInstance& parent_object_instance = get_parent_resource().get_parent_object_instance(); 00132 return parent_object_instance.instance_id(); 00133 } 00134 00135 M2MResource& M2MResourceInstance::get_parent_resource() const 00136 { 00137 return _parent_resource; 00138 } 00139 00140 M2MBase *M2MResourceInstance::get_parent() const 00141 { 00142 return (M2MBase *) &get_parent_resource(); 00143 } 00144 00145 const char* M2MResourceInstance::object_name() const 00146 { 00147 const M2MObjectInstance& parent_object_instance = _parent_resource.get_parent_object_instance(); 00148 const M2MObject& parent_object = parent_object_instance.get_parent_object(); 00149 00150 return parent_object.name(); 00151 } 00152
Generated on Tue Jul 12 2022 19:12:13 by
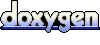