
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
m2mnotificationhandler.cpp
00001 /* 00002 * Copyright (c) 2018 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "m2mnotificationhandler.h" 00017 #include "eventOS_scheduler.h" 00018 #include "m2mnsdlinterface.h" 00019 #include "mbed-trace/mbed_trace.h" 00020 #include <assert.h> 00021 #include <inttypes.h> 00022 #include <stdlib.h> 00023 00024 #define MBED_CLIENT_NOTIFICATION_HANDLER_TASKLET_INIT_EVENT 0 // Tasklet init occurs always when generating a tasklet 00025 #define MBED_CLIENT_NOTIFICATION_HANDLER_EVENT 40 00026 #define TRACE_GROUP "mClt" 00027 00028 int8_t M2MNotificationHandler::_tasklet_id = -1; 00029 00030 extern "C" void notification_tasklet_func(arm_event_s *event) 00031 { 00032 M2MNsdlInterface *iface = (M2MNsdlInterface*)event->data_ptr; 00033 if (event->event_type == MBED_CLIENT_NOTIFICATION_HANDLER_EVENT) { 00034 iface->send_next_notification(false); 00035 event->event_data = 0; 00036 } 00037 } 00038 00039 M2MNotificationHandler::M2MNotificationHandler() 00040 { 00041 if (M2MNotificationHandler::_tasklet_id < 0) { 00042 M2MNotificationHandler::_tasklet_id = eventOS_event_handler_create(notification_tasklet_func, MBED_CLIENT_NOTIFICATION_HANDLER_TASKLET_INIT_EVENT); 00043 assert(M2MNotificationHandler::_tasklet_id >= 0); 00044 } 00045 00046 initialize_event(); 00047 } 00048 00049 M2MNotificationHandler::~M2MNotificationHandler() 00050 { 00051 } 00052 00053 void M2MNotificationHandler::send_notification(M2MNsdlInterface *interface) 00054 { 00055 tr_debug("M2MNotificationHandler::send_notification"); 00056 if (!_event.data.event_data) { 00057 _event.data.event_data = 1; 00058 _event.data.event_type = MBED_CLIENT_NOTIFICATION_HANDLER_EVENT; 00059 _event.data.data_ptr = interface; 00060 00061 eventOS_event_send_user_allocated(&_event); 00062 } else { 00063 tr_debug("M2MNotificationHandler::send_notification - event already in queue"); 00064 } 00065 } 00066 00067 void M2MNotificationHandler::initialize_event() 00068 { 00069 _event.data.data_ptr = NULL; 00070 _event.data.event_data = 0; 00071 _event.data.event_id = 0; 00072 _event.data.sender = 0; 00073 _event.data.event_type = 0; 00074 _event.data.priority = ARM_LIB_MED_PRIORITY_EVENT; 00075 _event.data.receiver = M2MNotificationHandler::_tasklet_id; 00076 }
Generated on Tue Jul 12 2022 19:12:13 by
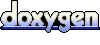