
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
m2mendpoint.cpp
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifdef MBED_CLOUD_CLIENT_EDGE_EXTENSION 00018 00019 #include "mbed-client/m2mendpoint.h" 00020 #include "mbed-client/m2mobject.h" 00021 #include "mbed-client/m2mconstants.h" 00022 #include "include/m2mtlvserializer.h" 00023 #include "include/m2mtlvdeserializer.h" 00024 #include "include/m2mreporthandler.h" 00025 #include "mbed-trace/mbed_trace.h" 00026 #include "mbed-client/m2mstringbuffer.h" 00027 #include "mbed-client/m2mstring.h" 00028 #include "nsdl-c/sn_nsdl_lib.h" 00029 00030 #include <stdlib.h> 00031 00032 #define BUFFER_SIZE 10 00033 #define TRACE_GROUP "mClt" 00034 00035 M2MEndpoint::M2MEndpoint(const String &object_name, char *path) 00036 : M2MBase(object_name, 00037 M2MBase::Dynamic, 00038 #ifndef DISABLE_RESOURCE_TYPE 00039 "", 00040 #endif 00041 path, 00042 false, 00043 false), 00044 _observation_handler(NULL), 00045 _ctx(NULL), 00046 _changed(true) 00047 { 00048 M2MBase::set_base_type(M2MBase::ObjectDirectory); 00049 get_nsdl_resource()->always_publish = false; 00050 #ifdef RESOURCE_ATTRIBUTES_LIST 00051 sn_nsdl_attribute_item_s item; 00052 item.attribute_name = ATTR_ENDPOINT_NAME; 00053 item.value = (char*)alloc_string_copy((uint8_t*) object_name.c_str(), object_name.length()); 00054 sn_nsdl_set_resource_attribute(get_nsdl_resource()->static_resource_parameters, &item); 00055 #endif 00056 } 00057 00058 00059 M2MEndpoint::~M2MEndpoint() 00060 { 00061 tr_debug("~M2MEndpoint %p", this); 00062 if(!_object_list.empty()) { 00063 00064 M2MObjectList::const_iterator it; 00065 it = _object_list.begin(); 00066 M2MObject* obj = NULL; 00067 uint16_t index = 0; 00068 for (; it!=_object_list.end(); it++, index++ ) { 00069 //Free allocated memory for object instances. 00070 obj = *it; 00071 tr_debug(" deleting object %p", obj); 00072 delete obj; 00073 } 00074 00075 _object_list.clear(); 00076 } 00077 00078 free_resources(); 00079 } 00080 00081 M2MObject* M2MEndpoint::create_object(const String &name) 00082 { 00083 M2MObject *obj = NULL; 00084 if (object(name) == NULL) { 00085 char *path = create_path(*this, name.c_str()); 00086 obj = new M2MObject(name, path, false); 00087 if (obj != NULL) { 00088 _object_list.push_back(obj); 00089 } 00090 } 00091 return obj; 00092 } 00093 00094 bool M2MEndpoint::remove_object(const String &name) 00095 { 00096 bool success = false; 00097 if (object_count() == 0) { 00098 return success; 00099 } 00100 M2MObjectList::const_iterator it; 00101 M2MObject *obj = NULL; 00102 int pos = 0; 00103 it = _object_list.begin(); 00104 for (; it != _object_list.end(); it++, pos++) { 00105 obj = *it; 00106 if (name == obj->name()) { 00107 delete obj; 00108 _object_list.erase(pos); 00109 success = true; 00110 break; 00111 } 00112 } 00113 return success; 00114 00115 } 00116 00117 M2MObject* M2MEndpoint::object(const String &name) const 00118 { 00119 M2MObject *obj = NULL; 00120 if (object_count() == 0) { 00121 return obj; 00122 } 00123 M2MObjectList::const_iterator it = _object_list.begin(); 00124 for (; it != _object_list.end(); it++) { 00125 if (name == (*it)->name()) { 00126 obj = *it; 00127 break; 00128 } 00129 } 00130 return obj; 00131 } 00132 00133 const M2MObjectList& M2MEndpoint::objects() const 00134 { 00135 return _object_list; 00136 } 00137 00138 uint16_t M2MEndpoint::object_count() const 00139 { 00140 return _object_list.size(); 00141 } 00142 00143 M2MObservationHandler* M2MEndpoint::observation_handler() const 00144 { 00145 return _observation_handler; 00146 } 00147 00148 void M2MEndpoint::set_observation_handler(M2MObservationHandler *handler) 00149 { 00150 _observation_handler = handler; 00151 } 00152 00153 void M2MEndpoint::add_observation_level(M2MBase::Observation observation_level) 00154 { 00155 (void)observation_level; 00156 } 00157 00158 void M2MEndpoint::remove_observation_level(M2MBase::Observation observation_level) 00159 { 00160 (void)observation_level; 00161 } 00162 00163 sn_coap_hdr_s* M2MEndpoint::handle_get_request(nsdl_s *nsdl, 00164 sn_coap_hdr_s *received_coap_header, 00165 M2MObservationHandler *observation_handler) 00166 { 00167 tr_debug("M2MEndpoint::handle_get_request()"); 00168 sn_coap_msg_code_e msg_code = COAP_MSG_CODE_RESPONSE_METHOD_NOT_ALLOWED; 00169 sn_coap_hdr_s * coap_response = sn_nsdl_build_response(nsdl, 00170 received_coap_header, msg_code); 00171 return coap_response; 00172 00173 } 00174 00175 sn_coap_hdr_s* M2MEndpoint::handle_put_request(nsdl_s *nsdl, 00176 sn_coap_hdr_s *received_coap_header, 00177 M2MObservationHandler */*observation_handler*/, 00178 bool &/*execute_value_updated*/) 00179 { 00180 tr_debug("M2MEndpoint::handle_put_request()"); 00181 sn_coap_msg_code_e msg_code = COAP_MSG_CODE_RESPONSE_METHOD_NOT_ALLOWED; 00182 sn_coap_hdr_s * coap_response = sn_nsdl_build_response(nsdl, 00183 received_coap_header, msg_code); 00184 return coap_response; 00185 } 00186 00187 00188 sn_coap_hdr_s* M2MEndpoint::handle_post_request(nsdl_s *nsdl, 00189 sn_coap_hdr_s *received_coap_header, 00190 M2MObservationHandler *observation_handler, 00191 bool &execute_value_updated, 00192 sn_nsdl_addr_s *) 00193 { 00194 tr_debug("M2MEndpoint::handle_post_request()"); 00195 sn_coap_msg_code_e msg_code = COAP_MSG_CODE_RESPONSE_METHOD_NOT_ALLOWED; 00196 sn_coap_hdr_s * coap_response = sn_nsdl_build_response(nsdl, 00197 received_coap_header, msg_code); 00198 return coap_response; 00199 } 00200 00201 void M2MEndpoint::set_context(void *ctx) 00202 { 00203 _ctx = ctx; 00204 } 00205 00206 void* M2MEndpoint::get_context() const 00207 { 00208 return _ctx; 00209 } 00210 00211 void M2MEndpoint::set_changed() 00212 { 00213 _changed = true; 00214 } 00215 00216 void M2MEndpoint::clear_changed() 00217 { 00218 _changed = false; 00219 } 00220 00221 bool M2MEndpoint::get_changed() const 00222 { 00223 return _changed; 00224 } 00225 00226 #endif // MBED_CLOUD_CLIENT_EDGE_EXTENSION
Generated on Tue Jul 12 2022 19:12:13 by
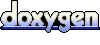