
bug fix
FtcdCommBase Class Reference
FtcdCommBase implements the logic of processing incoming requests from the remote Factory Tool Demo. More...
#include <ftcd_comm_base.h>
Inherited by FtcdCommSerial, and FtcdCommSocket.
Public Member Functions | |
virtual | ~FtcdCommBase ()=0 |
Not certain that we need to do anything here, but just in case we need to do some clean-up at some point. | |
virtual bool | init (void) |
Initializes Network interface and opens socket Prints IP address. | |
virtual void | finish (void) |
Closes the opened socket. | |
virtual ftcd_comm_status_e | wait_for_message (uint8_t **message_out, uint32_t *message_size_out) |
Wait and read complete message from the communication line. | |
ftcd_comm_status_e | send_response (const uint8_t *response_message, uint32_t response_message_size) |
Writes a response message to the communication line. | |
ftcd_comm_status_e | send_response (const uint8_t *response_message, uint32_t response_message_size, ftcd_comm_status_e status_code) |
Writes a response message with status to the communication line. | |
virtual bool | send (const uint8_t *response_message, uint32_t response_message_size)=0 |
Writes an allocated response message to the communication line medium. | |
virtual ftcd_comm_status_e | is_token_detected (void)=0 |
Detects the message token from the communication line medium. | |
virtual uint32_t | read_message_size (void)=0 |
Reads the message size in bytes from the communication line medium. | |
virtual bool | read_message (uint8_t *message_out, size_t message_size)=0 |
Reads the message size in bytes from the communication line medium. | |
virtual bool | read_message_signature (uint8_t *sig, size_t sig_size)=0 |
Reads the message size in bytes from the communication line medium. |
Detailed Description
FtcdCommBase implements the logic of processing incoming requests from the remote Factory Tool Demo.
Definition at line 70 of file ftcd_comm_base.h.
Constructor & Destructor Documentation
~FtcdCommBase | ( | ) | [pure virtual] |
Not certain that we need to do anything here, but just in case we need to do some clean-up at some point.
Definition at line 44 of file ftcd_comm_base.cpp.
Member Function Documentation
void finish | ( | void | ) | [virtual] |
Closes the opened socket.
Reimplemented in FtcdCommSocket.
Definition at line 57 of file ftcd_comm_base.cpp.
bool init | ( | void | ) | [virtual] |
Initializes Network interface and opens socket Prints IP address.
Reimplemented in FtcdCommSocket.
Definition at line 52 of file ftcd_comm_base.cpp.
virtual ftcd_comm_status_e is_token_detected | ( | void | ) | [pure virtual] |
Detects the message token from the communication line medium.
- Returns:
- zero, if token detected and different value otherwise
Implemented in FtcdCommSocket.
virtual bool read_message | ( | uint8_t * | message_out, |
size_t | message_size | ||
) | [pure virtual] |
Reads the message size in bytes from the communication line medium.
This is the amount of bytes needed to allocate for the upcoming message bytes.
- Parameters:
-
message_out The buffer to read into and return to the caller. message_size The message size in bytes.
- Returns:
- true upon success, false otherwise
Implemented in FtcdCommSocket.
virtual bool read_message_signature | ( | uint8_t * | sig, |
size_t | sig_size | ||
) | [pure virtual] |
Reads the message size in bytes from the communication line medium.
This is the amount of bytes needed to allocate for the upcoming message bytes.
- Parameters:
-
sig The buffer to read into and return to the caller. sig_size The sig buffer size in bytes.
- Returns:
- The message size in bytes in case of success, zero bytes otherwise.
Implemented in FtcdCommSocket.
virtual uint32_t read_message_size | ( | void | ) | [pure virtual] |
Reads the message size in bytes from the communication line medium.
This is the amount of bytes needed to allocate for the upcoming message bytes.
- Returns:
- The message size in bytes in case of success, zero bytes otherwise.
Implemented in FtcdCommSocket.
virtual bool send | ( | const uint8_t * | response_message, |
uint32_t | response_message_size | ||
) | [pure virtual] |
Writes an allocated response message to the communication line medium.
- Parameters:
-
response_message The message to send through the communication line medium response_message_size The message size in bytes
- Returns:
- true upon success, false otherwise
Implemented in FtcdCommSocket.
ftcd_comm_status_e send_response | ( | const uint8_t * | response_message, |
uint32_t | response_message_size, | ||
ftcd_comm_status_e | status_code | ||
) |
Writes a response message with status to the communication line.
The method build response message with status, header and signature (if requested) and writes it to the line
- Parameters:
-
response_message The message to send through the communication line medium response_message_size The message size in bytes
- Returns:
- FTCD_COMM_STATUS_SUCCESS on success, otherwise appropriate error from ftcd_comm_status_e
Definition at line 157 of file ftcd_comm_base.cpp.
ftcd_comm_status_e send_response | ( | const uint8_t * | response_message, |
uint32_t | response_message_size | ||
) |
Writes a response message to the communication line.
The method build response message with header and signature (if requested) and writes it to the line
- Parameters:
-
response_message The message to send through the communication line medium response_message_size The message size in bytes
- Returns:
- FTCD_COMM_STATUS_SUCCESS on success, otherwise appropriate error from ftcd_comm_status_e
Definition at line 152 of file ftcd_comm_base.cpp.
ftcd_comm_status_e wait_for_message | ( | uint8_t ** | message_out, |
uint32_t * | message_size_out | ||
) | [virtual] |
Wait and read complete message from the communication line.
The method waits in blocking mode for new message, allocate and read the message, and sets message_out and message_size_out
- Parameters:
-
message_out The message allocated and read from the communication line message_size_out The message size in bytes
- Returns:
- FTCD_COMM_STATUS_SUCCESS on success, otherwise appropriate error from ftcd_comm_status_e
Reimplemented in FtcdCommSocket.
Definition at line 61 of file ftcd_comm_base.cpp.
Generated on Tue Jul 12 2022 21:05:00 by
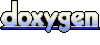