
wifi test
Dependencies: X_NUCLEO_IKS01A2 mbed-http
easy-connect.cpp
00001 /* 00002 * FILE: easy-connect.cpp 00003 * 00004 * Copyright (c) 2015 - 2017 ARM Limited. All rights reserved. 00005 * SPDX-License-Identifier: Apache-2.0 00006 * Licensed under the Apache License, Version 2.0 (the License); you may 00007 * not use this file except in compliance with the License. 00008 * You may obtain a copy of the License at 00009 * 00010 * http://www.apache.org/licenses/LICENSE-2.0 00011 * 00012 * Unless required by applicable law or agreed to in writing, software 00013 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00014 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 * See the License for the specific language governing permissions and 00016 * limitations under the License. 00017 */ 00018 00019 #include "mbed.h" 00020 #include "easy-connect.h" 00021 00022 /* 00023 * Instantiate the configured network interface 00024 */ 00025 #if MBED_CONF_APP_NETWORK_INTERFACE == WIFI_ESP8266 00026 #include "ESP8266Interface.h" 00027 #define EASY_CONNECT_WIFI_TYPE "ESP8266" 00028 00029 #ifdef MBED_CONF_APP_ESP8266_DEBUG 00030 ESP8266Interface wifi(MBED_CONF_EASY_CONNECT_WIFI_ESP8266_TX, MBED_CONF_EASY_CONNECT_WIFI_ESP8266_RX, MBED_CONF_EASY_CONNECT_WIFI_ESP8266_DEBUG); 00031 #else 00032 ESP8266Interface wifi(MBED_CONF_EASY_CONNECT_WIFI_ESP8266_TX, MBED_CONF_EASY_CONNECT_WIFI_ESP8266_RX); 00033 #endif 00034 00035 #elif MBED_CONF_APP_NETWORK_INTERFACE == WIFI_ODIN 00036 #define EASY_CONNECT_WIFI_TYPE "Odin" 00037 #include "OdinWiFiInterface.h" 00038 OdinWiFiInterface wifi; 00039 00040 #elif MBED_CONF_APP_NETWORK_INTERFACE == WIFI_RTW 00041 #define EASY_CONNECT_WIFI_TYPE "RTW" 00042 #include "RTWInterface.h" 00043 RTWInterface wifi; 00044 00045 #elif MBED_CONF_APP_NETWORK_INTERFACE == WIFI_IDW0XX1 00046 #include "SpwfSAInterface.h" 00047 00048 #if MBED_CONF_IDW0XX1_EXPANSION_BOARD == IDW01M1 00049 #define EASY_CONNECT_WIFI_TYPE "IDW01M1" 00050 SpwfSAInterface wifi(MBED_CONF_EASY_CONNECT_WIFI_IDW01M1_TX, MBED_CONF_EASY_CONNECT_WIFI_IDW01M1_RX); 00051 #endif // MBED_CONF_IDW0XX1_EXPANSION_BOARD == IDW01M1 00052 00053 #if MBED_CONF_IDW0XX1_EXPANSION_BOARD == IDW04A1 00054 #define EASY_CONNECT_WIFI_TYPE "IDW04A1" 00055 SpwfSAInterface wifi(MBED_CONF_EASY_CONNECT_WIFI_IDW04A1_TX, MBED_CONF_EASY_CONNECT_WIFI_IDW04A1_RX); 00056 #endif // MBED_CONF_IDW0XX1_EXPANSION_BOARD == IDW04A1 00057 00058 #elif MBED_CONF_APP_NETWORK_INTERFACE == WIFI_ISM43362 00059 #include "ISM43362Interface.h" 00060 #define EASY_CONNECT_WIFI_TYPE "ISM43362" 00061 00062 #ifdef MBED_CONF_APP_ISM43362_DEBUG 00063 ISM43362Interface wifi(true); 00064 #else 00065 ISM43362Interface wifi; 00066 #endif 00067 00068 #elif MBED_CONF_APP_NETWORK_INTERFACE == ETHERNET 00069 #include "EthernetInterface.h" 00070 EthernetInterface eth; 00071 00072 #elif MBED_CONF_APP_NETWORK_INTERFACE == MESH_LOWPAN_ND 00073 #define EASY_CONNECT_MESH 00074 #include "NanostackInterface.h" 00075 LoWPANNDInterface mesh; 00076 00077 #elif MBED_CONF_APP_NETWORK_INTERFACE == MESH_THREAD 00078 #define EASY_CONNECT_MESH 00079 #include "NanostackInterface.h" 00080 ThreadInterface mesh; 00081 00082 #elif MBED_CONF_APP_NETWORK_INTERFACE == CELLULAR_ONBOARD 00083 #include "OnboardCellularInterface.h" 00084 OnboardCellularInterface cellular; 00085 00086 #elif MBED_CONF_APP_NETWORK_INTERFACE == CELLULAR 00087 #include "EasyCellularConnection.h" 00088 EasyCellularConnection cellular; 00089 00090 #elif MBED_CONF_APP_NETWORK_INTERFACE == WIFI_WIZFI310 00091 #include "WizFi310Interface.h" 00092 #define EASY_CONNECT_WIFI_TYPE "WizFi310" 00093 00094 #ifdef MBED_CONF_APP_WIZFI310_DEBUG 00095 WizFi310Interface wifi(MBED_CONF_EASY_CONNECT_WIFI_WIZFI310_TX, MBED_CONF_EASY_CONNECT_WIFI_WIZFI310_RX, MBED_CONF_EASY_CONNECT_WIFI_WIZFI310_DEBUG); 00096 #else 00097 WizFi310Interface wifi(MBED_CONF_EASY_CONNECT_WIFI_WIZFI310_TX, MBED_CONF_EASY_CONNECT_WIFI_WIZFI310_RX); 00098 #endif 00099 00100 #elif MBED_CONF_APP_NETWORK_INTERFACE == CELLULAR_WNC14A2A 00101 #include "WNC14A2AInterface.h" 00102 00103 #if MBED_CONF_APP_WNC_DEBUG == true 00104 #include "WNCDebug.h" 00105 WNCDebug dbgout(stderr); 00106 WNC14A2AInterface wnc(&dbgout); 00107 #else 00108 WNC14A2AInterface wnc; 00109 #endif 00110 00111 00112 #else 00113 #error "No connectivity method chosen. Please add 'config.network-interfaces.value' to your mbed_app.json (see README.md for more information)." 00114 #endif // MBED_CONF_APP_NETWORK_INTERFACE 00115 00116 /* 00117 * In case of Mesh, instantiate the configured RF PHY. 00118 */ 00119 #if defined (EASY_CONNECT_MESH) 00120 #if MBED_CONF_APP_MESH_RADIO_TYPE == ATMEL 00121 #include "NanostackRfPhyAtmel.h" 00122 #define EASY_CONNECT_MESH_TYPE "Atmel" 00123 NanostackRfPhyAtmel rf_phy(ATMEL_SPI_MOSI, ATMEL_SPI_MISO, ATMEL_SPI_SCLK, ATMEL_SPI_CS, 00124 ATMEL_SPI_RST, ATMEL_SPI_SLP, ATMEL_SPI_IRQ, ATMEL_I2C_SDA, ATMEL_I2C_SCL); 00125 00126 #elif MBED_CONF_APP_MESH_RADIO_TYPE == MCR20 00127 #include "NanostackRfPhyMcr20a.h" 00128 #define EASY_CONNECT_MESH_TYPE "Mcr20A" 00129 NanostackRfPhyMcr20a rf_phy(MCR20A_SPI_MOSI, MCR20A_SPI_MISO, MCR20A_SPI_SCLK, MCR20A_SPI_CS, MCR20A_SPI_RST, MCR20A_SPI_IRQ); 00130 00131 #elif MBED_CONF_APP_MESH_RADIO_TYPE == SPIRIT1 00132 #include "NanostackRfPhySpirit1.h" 00133 #define EASY_CONNECT_MESH_TYPE "Spirit1" 00134 NanostackRfPhySpirit1 rf_phy(SPIRIT1_SPI_MOSI, SPIRIT1_SPI_MISO, SPIRIT1_SPI_SCLK, 00135 SPIRIT1_DEV_IRQ, SPIRIT1_DEV_CS, SPIRIT1_DEV_SDN, SPIRIT1_BRD_LED); 00136 00137 #elif MBED_CONF_APP_MESH_RADIO_TYPE == EFR32 00138 #include "NanostackRfPhyEfr32.h" 00139 #define EASY_CONNECT_MESH_TYPE "EFR32" 00140 NanostackRfPhyEfr32 rf_phy; 00141 00142 #endif // MBED_CONF_APP_RADIO_TYPE 00143 #endif // EASY_CONNECT_MESH 00144 00145 #if defined (EASY_CONNECT_WIFI) 00146 #define WIFI_SSID_MAX_LEN 32 // As per IEEE 802.11 chapter 7.3.2.1 (SSID element) 00147 #define WIFI_PASSWORD_MAX_LEN 64 // 00148 00149 char* _ssid = NULL; 00150 char* _password = NULL; 00151 #endif // EASY_CONNECT_WIFI 00152 00153 /* \brief print_MAC - print_MAC - helper function to print out MAC address 00154 * in: network_interface - pointer to network i/f 00155 * bool log-messages print out logs or not 00156 * MAC address is printed, if it can be acquired & log_messages is true. 00157 * 00158 */ 00159 void print_MAC(NetworkInterface* network_interface, bool log_messages) { 00160 #if MBED_CONF_APP_NETWORK_INTERFACE != CELLULAR_ONBOARD && MBED_CONF_APP_NETWORK_INTERFACE != CELLULAR 00161 const char *mac_addr = network_interface->get_mac_address(); 00162 if (mac_addr == NULL) { 00163 if (log_messages) { 00164 printf("[EasyConnect] ERROR - No MAC address\n"); 00165 } 00166 return; 00167 } 00168 if (log_messages) { 00169 printf("[EasyConnect] MAC address %s\n", mac_addr); 00170 } 00171 #endif 00172 } 00173 00174 00175 00176 /* \brief easy_connect easy_connect() function to connect the pre-defined network bearer, 00177 * config done via mbed_app.json (see README.md for details). 00178 * 00179 * IN: bool log_messages print out diagnostics or not. 00180 */ 00181 NetworkInterface* easy_connect(bool log_messages) { 00182 NetworkInterface* network_interface = NULL; 00183 int connect_success = -1; 00184 00185 #if defined (EASY_CONNECT_WIFI) 00186 // We check if the _ssid and _password have already been set (via the easy_connect() 00187 // that takes thoses parameters or not. 00188 // If they have not been set, use the ones we can gain from mbed_app.json. 00189 if (_ssid == NULL) { 00190 if(strlen(MBED_CONF_APP_WIFI_SSID) > WIFI_SSID_MAX_LEN) { 00191 printf("ERROR - MBED_CONF_APP_WIFI_SSID is too long %d vs. %d\n", 00192 strlen(MBED_CONF_APP_WIFI_SSID), 00193 WIFI_SSID_MAX_LEN); 00194 return NULL; 00195 } 00196 } 00197 00198 if (_password == NULL) { 00199 if(strlen(MBED_CONF_APP_WIFI_PASSWORD) > WIFI_PASSWORD_MAX_LEN) { 00200 printf("ERROR - MBED_CONF_APP_WIFI_PASSWORD is too long %d vs. %d\n", 00201 strlen(MBED_CONF_APP_WIFI_PASSWORD), 00202 WIFI_PASSWORD_MAX_LEN); 00203 return NULL; 00204 } 00205 } 00206 #endif // EASY_CONNECT_WIFI 00207 00208 /// This should be removed once mbedOS supports proper dual-stack 00209 if (log_messages) { 00210 #if defined (EASY_CONNECT_MESH) || (MBED_CONF_LWIP_IPV6_ENABLED==true) 00211 printf("[EasyConnect] IPv6 mode\n"); 00212 #else 00213 printf("[EasyConnect] IPv4 mode\n"); 00214 #endif 00215 } 00216 00217 #if defined (EASY_CONNECT_WIFI) 00218 if (log_messages) { 00219 printf("[EasyConnect] Using WiFi (%s) \n", EASY_CONNECT_WIFI_TYPE); 00220 printf("[EasyConnect] Connecting to WiFi %s\n", 00221 ((_ssid == NULL) ? MBED_CONF_APP_WIFI_SSID : _ssid) ); 00222 } 00223 network_interface = &wifi; 00224 if (_ssid == NULL) { 00225 connect_success = wifi.connect(MBED_CONF_APP_WIFI_SSID, MBED_CONF_APP_WIFI_PASSWORD, 00226 (strlen(MBED_CONF_APP_WIFI_PASSWORD) > 1) ? NSAPI_SECURITY_WPA_WPA2 : NSAPI_SECURITY_NONE); 00227 } 00228 else { 00229 connect_success = wifi.connect(_ssid, _password, (strlen(_password) > 1) ? NSAPI_SECURITY_WPA_WPA2 : NSAPI_SECURITY_NONE); 00230 } 00231 #elif MBED_CONF_APP_NETWORK_INTERFACE == CELLULAR_ONBOARD || MBED_CONF_APP_NETWORK_INTERFACE == CELLULAR 00232 # ifdef MBED_CONF_APP_CELLULAR_SIM_PIN 00233 cellular.set_sim_pin(MBED_CONF_APP_CELLULAR_SIM_PIN); 00234 # endif 00235 # ifdef MBED_CONF_APP_CELLULAR_APN 00236 # ifndef MBED_CONF_APP_CELLULAR_USERNAME 00237 # define MBED_CONF_APP_CELLULAR_USERNAME 0 00238 # endif 00239 # ifndef MBED_CONF_APP_CELLULAR_PASSWORD 00240 # define MBED_CONF_APP_CELLULAR_PASSWORD 0 00241 # endif 00242 cellular.set_credentials(MBED_CONF_APP_CELLULAR_APN, MBED_CONF_APP_CELLULAR_USERNAME, MBED_CONF_APP_CELLULAR_PASSWORD); 00243 if (log_messages) { 00244 printf("[EasyConnect] Connecting using Cellular interface and APN %s\n", MBED_CONF_APP_CELLULAR_APN); 00245 } 00246 # else 00247 if (log_messages) { 00248 printf("[EasyConnect] Connecting using Cellular interface and default APN\n"); 00249 } 00250 # endif 00251 connect_success = cellular.connect(); 00252 network_interface = &cellular; 00253 00254 #elif MBED_CONF_APP_NETWORK_INTERFACE == CELLULAR_WNC14A2A 00255 if (log_messages) { 00256 printf("[EasyConnect] Using WNC14A2A\n"); 00257 } 00258 # if MBED_CONF_APP_WNC_DEBUG == true 00259 printf("[EasyConnect] With WNC14A2A debug output set to 0x%02X\n",MBED_CONF_APP_WNC_DEBUG_SETTING); 00260 wnc.doDebug(MBED_CONF_APP_WNC_DEBUG_SETTING); 00261 # endif 00262 network_interface = &wnc; 00263 connect_success = wnc.connect(); 00264 00265 #elif MBED_CONF_APP_NETWORK_INTERFACE == ETHERNET 00266 if (log_messages) { 00267 printf("[EasyConnect] Using Ethernet\n"); 00268 } 00269 network_interface = ð 00270 #if MBED_CONF_EVENTS_SHARED_DISPATCH_FROM_APPLICATION 00271 eth.set_blocking(false); 00272 #endif 00273 connect_success = eth.connect(); 00274 #endif 00275 00276 #ifdef EASY_CONNECT_MESH 00277 if (log_messages) { 00278 printf("[EasyConnect] Using Mesh (%s)\n", EASY_CONNECT_MESH_TYPE); 00279 printf("[EasyConnect] Connecting to Mesh...\n"); 00280 } 00281 network_interface = &mesh; 00282 #if MBED_CONF_EVENTS_SHARED_DISPATCH_FROM_APPLICATION 00283 mesh.set_blocking(false); 00284 #endif 00285 mesh.initialize(&rf_phy); 00286 connect_success = mesh.connect(); 00287 #endif 00288 if(connect_success == 0 00289 #if (MBED_CONF_EVENTS_SHARED_DISPATCH_FROM_APPLICATION && (MBED_CONF_APP_NETWORK_INTERFACE == ETHERNET || defined(EASY_CONNECT_MESH))) 00290 || connect_success == NSAPI_ERROR_IS_CONNECTED || connect_success == NSAPI_ERROR_ALREADY 00291 #endif 00292 ) { 00293 #if (MBED_CONF_EVENTS_SHARED_DISPATCH_FROM_APPLICATION && (MBED_CONF_APP_NETWORK_INTERFACE == ETHERNET || defined(EASY_CONNECT_MESH))) 00294 nsapi_connection_status_t connection_status; 00295 00296 for (;;) { 00297 00298 // Check current connection status. 00299 connection_status = network_interface->get_connection_status(); 00300 00301 if (connection_status == NSAPI_STATUS_GLOBAL_UP) { 00302 00303 // Connection ready. 00304 break; 00305 00306 } else if (connection_status == NSAPI_STATUS_ERROR_UNSUPPORTED) { 00307 00308 if (log_messages) { 00309 print_MAC(network_interface, log_messages); 00310 printf("[EasyConnect] Connection to Network Failed %d!\n", connection_status); 00311 } 00312 return NULL; 00313 00314 } 00315 00316 // Not ready yet, give some runtime to the network stack. 00317 mbed::mbed_event_queue()->dispatch(100); 00318 00319 } 00320 #endif 00321 00322 if (log_messages) { 00323 printf("[EasyConnect] Connected to Network successfully\n"); 00324 print_MAC(network_interface, log_messages); 00325 } 00326 } else { 00327 if (log_messages) { 00328 print_MAC(network_interface, log_messages); 00329 printf("[EasyConnect] Connection to Network Failed %d!\n", connect_success); 00330 } 00331 return NULL; 00332 } 00333 const char *ip_addr = network_interface->get_ip_address(); 00334 if (ip_addr == NULL) { 00335 if (log_messages) { 00336 printf("[EasyConnect] ERROR - No IP address\n"); 00337 } 00338 return NULL; 00339 } 00340 00341 if (log_messages) { 00342 printf("[EasyConnect] IP address %s\n", ip_addr); 00343 } 00344 return network_interface; 00345 } 00346 00347 /* \brief easy_connect - easy_connect function to connect the pre-defined network bearer, 00348 * config done via mbed_app.json (see README.md for details). 00349 * This version is just a helper version and uses the easy_connect() with 00350 * one parameters to do it's job. 00351 * IN: bool log_messages print out diagnostics or not. 00352 * char* WiFiSSID WiFi SSID - pointer to WiFi SSID, but if it is NULL 00353 * then MBED_CONF_APP_WIFI_SSID will be used 00354 * char* WiFiPassword WiFi Password - pointer to WiFI password, but if it's NULL 00355 * then MBED_CONF_APP_WIFI_PASSWORD will be used 00356 */ 00357 00358 NetworkInterface* easy_connect(bool log_messages, 00359 char* WiFiSSID, 00360 char* WiFiPassword ) { 00361 00362 // This functionality only makes sense when using WiFi 00363 #if defined (EASY_CONNECT_WIFI) 00364 // We essentially want to populate the _ssid and _password and then call easy_connect() again. 00365 if (WiFiSSID != NULL) { 00366 if(strlen(WiFiSSID) > WIFI_SSID_MAX_LEN) { 00367 printf("ERROR - WiFi SSID is too long - %d vs %d.\n", strlen(WiFiSSID), WIFI_SSID_MAX_LEN); 00368 return NULL; 00369 } 00370 _ssid = WiFiSSID; 00371 } 00372 00373 if (WiFiPassword != NULL) { 00374 if(strlen(WiFiPassword) > WIFI_PASSWORD_MAX_LEN) { 00375 printf("ERROR - WiFi Password is too long - %d vs %d\n", strlen(WiFiPassword), WIFI_PASSWORD_MAX_LEN); 00376 return NULL; 00377 } 00378 _password = WiFiPassword; 00379 } 00380 #endif // EASY_CONNECT_WIFI 00381 return easy_connect(log_messages); 00382 } 00383 00384 /* \brief easy_get_netif - easy_connect function to get pointer to network interface 00385 * without connecting to it. 00386 * 00387 * IN: bool log_messages print out diagnostics or not. 00388 */ 00389 NetworkInterface* easy_get_netif(bool log_messages) { 00390 #if defined (EASY_CONNECT_WIFI) 00391 if (log_messages) { 00392 printf("[EasyConnect] WiFi: %s\n", EASY_CONNECT_WIFI_TYPE); 00393 } 00394 return &wifi; 00395 00396 #elif MBED_CONF_APP_NETWORK_INTERFACE == ETHERNET 00397 if (log_messages) { 00398 printf("[EasyConnect] Ethernet\n"); 00399 } 00400 return ð 00401 00402 #elif defined (EASY_CONNECT_MESH) 00403 if (log_messages) { 00404 printf("[EasyConnect] Mesh : %s\n", EASY_CONNECT_MESH_TYPE); 00405 } 00406 return &mesh; 00407 00408 #elif MBED_CONF_APP_NETWORK_INTERFACE == CELLULAR_ONBOARD || MBED_CONF_APP_NETWORK_INTERFACE == CELLULAR 00409 if (log_messages) { 00410 printf("[EasyConnect] Cellular\n"); 00411 } 00412 return &cellular; 00413 00414 #elif MBED_CONF_APP_NETWORK_INTERFACE == CELLULAR_WNC14A2A 00415 if (log_messages) { 00416 printf("[EasyConnect] WNC14A2A\n"); 00417 } 00418 return &wnc; 00419 #endif 00420 } 00421 00422 /* \brief easy_get_wifi - easy_connect function to get pointer to Wifi interface 00423 * without connecting to it. You would want this 1st so that 00424 * you can scan the APNs, choose the right one and then connect. 00425 * 00426 * IN: bool log_messages print out diagnostics or not. 00427 */ 00428 WiFiInterface* easy_get_wifi(bool log_messages) { 00429 #if defined (EASY_CONNECT_WIFI) 00430 if (log_messages) { 00431 printf("[EasyConnect] WiFi: %s\n", EASY_CONNECT_WIFI_TYPE); 00432 } 00433 return &wifi; 00434 #else 00435 if (log_messages) { 00436 printf("[EasyConnect] ERROR - Wifi not in use, can not return WifiInterface.\n"); 00437 } 00438 return NULL; 00439 #endif 00440 }
Generated on Tue Jul 12 2022 17:09:09 by
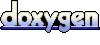