
wifi test
Dependencies: X_NUCLEO_IKS01A2 mbed-http
WNCDebug.h
00001 /** 00002 * copyright (c) 2017-2018, James Flynn 00003 * SPDX-License-Identifier: Apache-2.0 00004 */ 00005 00006 /* 00007 * Licensed under the Apache License, Version 2.0 (the "License"); 00008 * you may not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, 00015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * 00017 * See the License for the specific language governing permissions and 00018 * limitations under the License. 00019 */ 00020 00021 /** 00022 * @file WNCDebug.h 00023 * @brief A debug class that coordinates the output of debug messages to 00024 * either stdio or a serial port based on instantiation. 00025 * 00026 * @author James Flynn 00027 * 00028 * @date 1-Feb-2018 00029 * 00030 */ 00031 00032 #ifndef __WNCDEBUG__ 00033 #define __WNCDEBUG__ 00034 #include <stdio.h> 00035 #include "WNCIO.h" 00036 00037 /** WNCDebug class 00038 * Used to write debug data to the user designated IO. Currently 00039 * The class expects either a stdio element (defaults to stderr) or 00040 * a pointer to a WncIO object. 00041 */ 00042 00043 class WNCDebug 00044 { 00045 public: 00046 //! Create class with either stdio or a pointer to a uart 00047 WNCDebug( FILE * fd = stderr ): m_puart(NULL) {m_stdiofp=fd;}; 00048 WNCDebug( WncIO * uart): m_stdiofp(NULL) {m_puart=uart;}; 00049 ~WNCDebug() {}; 00050 00051 //! standard printf() functionallity 00052 int printf( char * fmt, ...) { 00053 char buffer[256]; 00054 int ret=0; 00055 va_list args; 00056 va_start (args, fmt); 00057 vsnprintf(buffer, sizeof(buffer), fmt, args); 00058 prt.lock(); 00059 if( m_stdiofp ) 00060 ret=fputs(buffer,m_stdiofp); 00061 else 00062 ret=m_puart->puts(buffer); 00063 prt.unlock(); 00064 va_end (args); 00065 return ret; 00066 } 00067 00068 //! standard putc() functionallity 00069 int putc( int c ) { 00070 int ret=0; 00071 prt.lock(); 00072 if( m_stdiofp ) 00073 ret=fputc(c, m_stdiofp); 00074 else 00075 ret=m_puart->putc(c); 00076 prt.unlock(); 00077 return ret; 00078 } 00079 00080 //! standard puts() functionallity 00081 int puts( const char * str ) { 00082 int ret=0; 00083 prt.lock(); 00084 if( m_stdiofp ) 00085 ret=fputs(str,m_stdiofp); 00086 else 00087 ret=m_puart->puts(str); 00088 prt.unlock(); 00089 return ret; 00090 } 00091 00092 private: 00093 std::FILE *m_stdiofp; 00094 WncIO *m_puart; 00095 Mutex prt; 00096 }; 00097 00098 #endif 00099
Generated on Tue Jul 12 2022 17:09:10 by
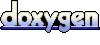