
Changes to enabled on-line compiler
Embed:
(wiki syntax)
Show/hide line numbers
subscribe_publish_cpp_sample.cpp
Go to the documentation of this file.
00001 /** 00002 * @file subscribe_publish_cpp_sample.cpp 00003 * @brief simple MQTT publish and subscribe on the same topic in C++ 00004 * 00005 * This example takes the parameters from the aws_iot_config.h file and establishes a connection to the AWS IoT MQTT Platform. 00006 * It subscribes and publishes to the same topic - "sdkTest/sub" 00007 * 00008 * If all the certs are correct, you should see the messages received by the application in a loop. 00009 * 00010 * The application takes in the certificate path, host name , port and the number of times the publish should happen. 00011 * 00012 */ 00013 #include "mbed.h" 00014 00015 #include <stdio.h> 00016 #include <stdlib.h> 00017 #include <ctype.h> 00018 #include <limits.h> 00019 #include <string.h> 00020 00021 #include "aws_iot_config.h" 00022 #include "aws_iot_log.h" 00023 #include "aws_iot_version.h" 00024 #include "aws_iot_mqtt_client_interface.h" 00025 00026 #include "easy-connect.h" 00027 00028 /** 00029 * @brief Default cert location 00030 */ 00031 char cPayload[100]; 00032 00033 Thread aws_subscribe_publish(osPriorityNormal, 8*1024, NULL); 00034 void aws_subscribe_publish_task(void); 00035 00036 /** 00037 * @brief This parameter will avoid infinite loop of publish and exit the program after certain number of publishes 00038 */ 00039 uint32_t publishCount = 0; 00040 00041 void iot_subscribe_callback_handler(AWS_IoT_Client *pClient, char *topicName, uint16_t topicNameLen, IoT_Publish_Message_Params *params, void *pData) 00042 { 00043 IOT_UNUSED(pData); 00044 IOT_UNUSED(pClient); 00045 IOT_INFO("Subscribe callback"); 00046 IOT_INFO("%.*s\t%.*s", topicNameLen, topicName, (int) params->payloadLen, (char *) params->payload); 00047 } 00048 00049 void disconnectCallbackHandler(AWS_IoT_Client *pClient, void *data) 00050 { 00051 IOT_WARN("MQTT Disconnect"); 00052 IoT_Error_t rc = FAILURE; 00053 00054 if(NULL == pClient) 00055 return; 00056 00057 IOT_UNUSED(data); 00058 00059 if(aws_iot_is_autoreconnect_enabled(pClient)) { 00060 IOT_INFO("Auto Reconnect is enabled, Reconnecting attempt will start now"); 00061 } 00062 else{ 00063 IOT_WARN("Auto Reconnect not enabled. Starting manual reconnect..."); 00064 rc = aws_iot_mqtt_attempt_reconnect(pClient); 00065 if(NETWORK_RECONNECTED == rc) { 00066 IOT_WARN("Manual Reconnect Successful"); 00067 } 00068 else{ 00069 IOT_WARN("Manual Reconnect Failed - %d", rc); 00070 } 00071 } 00072 } 00073 00074 int main() 00075 { 00076 printf("AWS %s Example.\n",__FILE__); 00077 IOT_INFO("\nAWS IoT SDK Version %d.%d.%d-%s\n", VERSION_MAJOR, VERSION_MINOR, VERSION_PATCH, VERSION_TAG); 00078 00079 aws_subscribe_publish.start(aws_subscribe_publish_task); 00080 aws_subscribe_publish.join(); 00081 printf(" - - - - - - - ALL DONE - - - - - - - \n"); 00082 } 00083 00084 void aws_subscribe_publish_task() 00085 { 00086 bool infinitePublishFlag = true; 00087 IoT_Error_t rc = FAILURE; 00088 00089 00090 int32_t i = 0; 00091 00092 AWS_IoT_Client client; 00093 IoT_Client_Init_Params mqttInitParams = iotClientInitParamsDefault; 00094 IoT_Client_Connect_Params connectParams = iotClientConnectParamsDefault; 00095 00096 IoT_Publish_Message_Params paramsQOS0; 00097 IoT_Publish_Message_Params paramsQOS1; 00098 00099 memset(cPayload, 0x00, sizeof(cPayload)); 00100 00101 mqttInitParams.enableAutoReconnect = false; // We enable this later below 00102 mqttInitParams.pHostURL = AWS_IOT_MQTT_HOST; 00103 mqttInitParams.port = AWS_IOT_MQTT_PORT; 00104 mqttInitParams.pRootCALocation = AWS_IOT_ROOT_CA_FILENAME; 00105 mqttInitParams.pDeviceCertLocation = AWS_IOT_CERTIFICATE_FILENAME; 00106 mqttInitParams.pDevicePrivateKeyLocation = AWS_IOT_PRIVATE_KEY_FILENAME; 00107 mqttInitParams.mqttCommandTimeout_ms = 20000; 00108 mqttInitParams.tlsHandshakeTimeout_ms = 5000; 00109 mqttInitParams.isSSLHostnameVerify = true; 00110 mqttInitParams.disconnectHandler = disconnectCallbackHandler; 00111 mqttInitParams.disconnectHandlerData = NULL; 00112 rc = aws_iot_mqtt_init(&client, &mqttInitParams); 00113 if(AWS_SUCCESS != rc) { 00114 IOT_ERROR("aws_iot_mqtt_init returned error : %d ", rc); 00115 return; 00116 } 00117 00118 connectParams.keepAliveIntervalInSec = 600; 00119 connectParams.isCleanSession = true; 00120 connectParams.MQTTVersion = MQTT_3_1_1; 00121 connectParams.pClientID = (char *)AWS_IOT_MQTT_CLIENT_ID; 00122 connectParams.clientIDLen = (uint16_t) strlen(AWS_IOT_MQTT_CLIENT_ID); 00123 connectParams.isWillMsgPresent = false; 00124 00125 IOT_INFO("Connecting..."); 00126 rc = aws_iot_mqtt_connect(&client, &connectParams); 00127 if(AWS_SUCCESS != rc) { 00128 IOT_ERROR("Error(%d) connecting to %s:%d", rc, mqttInitParams.pHostURL, mqttInitParams.port); 00129 return; 00130 } 00131 00132 /* 00133 * Enable Auto Reconnect functionality. Minimum and Maximum time of Exponential backoff are set in aws_iot_config.h 00134 * #AWS_IOT_MQTT_MIN_RECONNECT_WAIT_INTERVAL 00135 * #AWS_IOT_MQTT_MAX_RECONNECT_WAIT_INTERVAL 00136 */ 00137 rc = aws_iot_mqtt_autoreconnect_set_status(&client, true); 00138 if(AWS_SUCCESS != rc) { 00139 IOT_ERROR("Unable to set Auto Reconnect to true - %d", rc); 00140 return; 00141 } 00142 00143 IOT_INFO("Subscribing..."); 00144 rc = aws_iot_mqtt_subscribe(&client, "sdkTest/sub", 11, QOS0, iot_subscribe_callback_handler, NULL); 00145 if(AWS_SUCCESS != rc) { 00146 IOT_ERROR("Error subscribing : %d ", rc); 00147 return; 00148 } 00149 00150 sprintf(cPayload, "%s : %ld ", "hello from SDK", i); 00151 00152 paramsQOS0.qos = QOS0; 00153 paramsQOS0.payload = (void *) cPayload; 00154 paramsQOS0.isRetained = 0; 00155 00156 paramsQOS1.qos = QOS1; 00157 paramsQOS1.payload = (void *) cPayload; 00158 paramsQOS1.isRetained = 0; 00159 00160 if(publishCount != 0) { 00161 infinitePublishFlag = false; 00162 } 00163 00164 while( (NETWORK_ATTEMPTING_RECONNECT == rc || NETWORK_RECONNECTED == rc || AWS_SUCCESS == rc) && (publishCount > 0 || infinitePublishFlag)) { 00165 sprintf(cPayload, "%s : %ld ", "hello from SDK QOS0", i++); 00166 paramsQOS0.payloadLen = strlen(cPayload); 00167 rc = aws_iot_mqtt_publish(&client, "sdkTest/sub", 11, ¶msQOS0); 00168 if(publishCount > 0) 00169 publishCount--; 00170 00171 sprintf(cPayload, "%s : %ld ", "hello from SDK QOS1", i++); 00172 paramsQOS1.payloadLen = strlen(cPayload); 00173 rc = aws_iot_mqtt_publish(&client, "sdkTest/sub", 11, ¶msQOS1); 00174 if (rc == MQTT_REQUEST_TIMEOUT_ERROR) { 00175 IOT_WARN("QOS1 publish ack not received.\n"); 00176 rc = AWS_SUCCESS; 00177 } 00178 if(publishCount > 0) 00179 publishCount--; 00180 00181 IOT_INFO("-->sleep"); 00182 wait(5); 00183 } 00184 00185 if(AWS_SUCCESS != rc) { 00186 IOT_ERROR("An error occurred in the loop.\n"); 00187 } 00188 else{ 00189 IOT_INFO("Publish done\n"); 00190 } 00191 printf("... AWS_SUBSCRIBE_PUBLISH EXAMPLE DONE!\n"); 00192 }
Generated on Tue Jul 12 2022 19:02:38 by
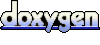