
Changes to enabled on-line compiler
Embed:
(wiki syntax)
Show/hide line numbers
aws_iot_shadow.c
Go to the documentation of this file.
00001 /* 00002 * Copyright 2010-2015 Amazon.com, Inc. or its affiliates. All Rights Reserved. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"). 00005 * You may not use this file except in compliance with the License. 00006 * A copy of the License is located at 00007 * 00008 * http://aws.amazon.com/apache2.0 00009 * 00010 * or in the "license" file accompanying this file. This file is distributed 00011 * on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either 00012 * express or implied. See the License for the specific language governing 00013 * permissions and limitations under the License. 00014 */ 00015 00016 /** 00017 * @file aws_iot_shadow.c 00018 * @brief Shadow client API definitions 00019 */ 00020 00021 #ifdef __cplusplus 00022 extern "C" { 00023 #endif 00024 00025 #include <string.h> 00026 #include "aws_iot_mqtt_client_interface.h" 00027 #include "aws_iot_shadow_interface.h" 00028 #include "aws_iot_error.h" 00029 #include "aws_iot_log.h" 00030 #include "aws_iot_shadow_actions.h" 00031 #include "aws_iot_shadow_json.h" 00032 #include "aws_iot_shadow_key.h" 00033 #include "aws_iot_shadow_records.h" 00034 00035 const ShadowInitParameters_t ShadowInitParametersDefault = {(char *) AWS_IOT_MQTT_HOST, AWS_IOT_MQTT_PORT, NULL, NULL, 00036 NULL, false, NULL}; 00037 00038 const ShadowConnectParameters_t ShadowConnectParametersDefault = {(char *) AWS_IOT_MY_THING_NAME, 00039 (char *) AWS_IOT_MQTT_CLIENT_ID, 0, NULL}; 00040 00041 static char deleteAcceptedTopic[MAX_SHADOW_TOPIC_LENGTH_BYTES]; 00042 00043 void aws_iot_shadow_reset_last_received_version(void) { 00044 shadowJsonVersionNum = 0; 00045 } 00046 00047 uint32_t aws_iot_shadow_get_last_received_version(void) { 00048 return shadowJsonVersionNum; 00049 } 00050 00051 void aws_iot_shadow_enable_discard_old_delta_msgs(void) { 00052 shadowDiscardOldDeltaFlag = true; 00053 } 00054 00055 void aws_iot_shadow_disable_discard_old_delta_msgs(void) { 00056 shadowDiscardOldDeltaFlag = false; 00057 } 00058 00059 IoT_Error_t aws_iot_shadow_free(AWS_IoT_Client *pClient) 00060 { 00061 IoT_Error_t rc; 00062 00063 if (NULL == pClient) { 00064 FUNC_EXIT_RC(NULL_VALUE_ERROR); 00065 } 00066 00067 rc = aws_iot_mqtt_free(pClient); 00068 00069 FUNC_EXIT_RC(rc); 00070 } 00071 00072 IoT_Error_t aws_iot_shadow_init(AWS_IoT_Client *pClient, ShadowInitParameters_t *pParams) { 00073 IoT_Client_Init_Params mqttInitParams = IoT_Client_Init_Params_initializer; 00074 IoT_Error_t rc; 00075 00076 FUNC_ENTRY; 00077 00078 if(NULL == pClient || NULL == pParams) { 00079 FUNC_EXIT_RC(NULL_VALUE_ERROR); 00080 } 00081 00082 mqttInitParams.enableAutoReconnect = pParams->enableAutoReconnect; 00083 mqttInitParams.pHostURL = pParams->pHost; 00084 mqttInitParams.port = pParams->port; 00085 mqttInitParams.pRootCALocation = pParams->pRootCA; 00086 mqttInitParams.pDeviceCertLocation = pParams->pClientCRT; 00087 mqttInitParams.pDevicePrivateKeyLocation = pParams->pClientKey; 00088 mqttInitParams.mqttPacketTimeout_ms = 5000; 00089 mqttInitParams.mqttCommandTimeout_ms = 20000; 00090 mqttInitParams.tlsHandshakeTimeout_ms = 5000; 00091 mqttInitParams.isSSLHostnameVerify = true; 00092 mqttInitParams.disconnectHandler = pParams->disconnectHandler; 00093 00094 rc = aws_iot_mqtt_init(pClient, &mqttInitParams); 00095 if(AWS_SUCCESS != rc) { 00096 FUNC_EXIT_RC(rc); 00097 } 00098 00099 resetClientTokenSequenceNum(); 00100 aws_iot_shadow_reset_last_received_version(); 00101 initDeltaTokens(); 00102 00103 FUNC_EXIT_RC(AWS_SUCCESS); 00104 } 00105 00106 IoT_Error_t aws_iot_shadow_connect(AWS_IoT_Client *pClient, ShadowConnectParameters_t *pParams) { 00107 IoT_Error_t rc = AWS_SUCCESS; 00108 uint16_t deleteAcceptedTopicLen; 00109 IoT_Client_Connect_Params ConnectParams = iotClientConnectParamsDefault; 00110 00111 FUNC_ENTRY; 00112 00113 if(NULL == pClient || NULL == pParams || NULL == pParams->pMqttClientId) { 00114 FUNC_EXIT_RC(NULL_VALUE_ERROR); 00115 } 00116 00117 snprintf(myThingName, MAX_SIZE_OF_THING_NAME, "%s", pParams->pMyThingName); 00118 snprintf(mqttClientID, MAX_SIZE_OF_UNIQUE_CLIENT_ID_BYTES, "%s", pParams->pMqttClientId); 00119 00120 ConnectParams.keepAliveIntervalInSec = 600; // NOTE: Temporary fix 00121 ConnectParams.MQTTVersion = MQTT_3_1_1; 00122 ConnectParams.isCleanSession = true; 00123 ConnectParams.isWillMsgPresent = false; 00124 ConnectParams.pClientID = pParams->pMqttClientId; 00125 ConnectParams.clientIDLen = pParams->mqttClientIdLen; 00126 ConnectParams.pPassword = NULL; 00127 ConnectParams.pUsername = NULL; 00128 00129 rc = aws_iot_mqtt_connect(pClient, &ConnectParams); 00130 00131 if(AWS_SUCCESS != rc) { 00132 FUNC_EXIT_RC(rc); 00133 } 00134 00135 initializeRecords(pClient); 00136 00137 if(NULL != pParams->deleteActionHandler) { 00138 snprintf(deleteAcceptedTopic, MAX_SHADOW_TOPIC_LENGTH_BYTES, 00139 "$aws/things/%s/shadow/delete/accepted", myThingName); 00140 deleteAcceptedTopicLen = (uint16_t) strlen(deleteAcceptedTopic); 00141 rc = aws_iot_mqtt_subscribe(pClient, deleteAcceptedTopic, deleteAcceptedTopicLen, QOS1, 00142 pParams->deleteActionHandler, (void *) myThingName); 00143 } 00144 00145 FUNC_EXIT_RC(rc); 00146 } 00147 00148 IoT_Error_t aws_iot_shadow_register_delta(AWS_IoT_Client *pMqttClient, jsonStruct_t *pStruct) { 00149 if(NULL == pMqttClient || NULL == pStruct) { 00150 return NULL_VALUE_ERROR; 00151 } 00152 00153 if(!aws_iot_mqtt_is_client_connected(pMqttClient)) { 00154 return MQTT_CONNECTION_ERROR; 00155 } 00156 00157 return registerJsonTokenOnDelta(pStruct); 00158 } 00159 00160 IoT_Error_t aws_iot_shadow_yield(AWS_IoT_Client *pClient, uint32_t timeout) { 00161 if(NULL == pClient) { 00162 return NULL_VALUE_ERROR; 00163 } 00164 00165 HandleExpiredResponseCallbacks(); 00166 return aws_iot_mqtt_yield(pClient, timeout); 00167 } 00168 00169 IoT_Error_t aws_iot_shadow_disconnect(AWS_IoT_Client *pClient) { 00170 return aws_iot_mqtt_disconnect(pClient); 00171 } 00172 00173 IoT_Error_t aws_iot_shadow_update(AWS_IoT_Client *pClient, const char *pThingName, char *pJsonString, 00174 fpActionCallback_t callback, void *pContextData, uint8_t timeout_seconds, 00175 bool isPersistentSubscribe) { 00176 IoT_Error_t rc; 00177 00178 if(NULL == pClient) { 00179 FUNC_EXIT_RC(NULL_VALUE_ERROR); 00180 } 00181 00182 if(!aws_iot_mqtt_is_client_connected(pClient)) { 00183 FUNC_EXIT_RC(MQTT_CONNECTION_ERROR); 00184 } 00185 00186 rc = aws_iot_shadow_internal_action(pThingName, SHADOW_UPDATE, pJsonString, strlen(pJsonString), callback, pContextData, 00187 timeout_seconds, isPersistentSubscribe); 00188 00189 FUNC_EXIT_RC(rc); 00190 } 00191 00192 IoT_Error_t aws_iot_shadow_delete(AWS_IoT_Client *pClient, const char *pThingName, fpActionCallback_t callback, 00193 void *pContextData, uint8_t timeout_seconds, bool isPersistentSubscribe) { 00194 char deleteRequestJsonBuf[MAX_SIZE_CLIENT_TOKEN_CLIENT_SEQUENCE]; 00195 IoT_Error_t rc; 00196 00197 FUNC_ENTRY; 00198 00199 if(NULL == pClient) { 00200 FUNC_EXIT_RC(NULL_VALUE_ERROR); 00201 } 00202 00203 if(!aws_iot_mqtt_is_client_connected(pClient)) { 00204 FUNC_EXIT_RC(MQTT_CONNECTION_ERROR); 00205 } 00206 00207 rc = aws_iot_shadow_internal_delete_request_json(deleteRequestJsonBuf, MAX_SIZE_CLIENT_TOKEN_CLIENT_SEQUENCE ); 00208 if ( AWS_SUCCESS != rc ) { 00209 FUNC_EXIT_RC( rc ); 00210 } 00211 00212 rc = aws_iot_shadow_internal_action(pThingName, SHADOW_DELETE, deleteRequestJsonBuf, MAX_SIZE_CLIENT_TOKEN_CLIENT_SEQUENCE, callback, pContextData, 00213 timeout_seconds, isPersistentSubscribe); 00214 00215 FUNC_EXIT_RC(rc); 00216 } 00217 00218 IoT_Error_t aws_iot_shadow_get(AWS_IoT_Client *pClient, const char *pThingName, fpActionCallback_t callback, 00219 void *pContextData, uint8_t timeout_seconds, bool isPersistentSubscribe) { 00220 char getRequestJsonBuf[MAX_SIZE_CLIENT_TOKEN_CLIENT_SEQUENCE]; 00221 IoT_Error_t rc; 00222 00223 FUNC_ENTRY; 00224 00225 if(NULL == pClient) { 00226 FUNC_EXIT_RC(NULL_VALUE_ERROR); 00227 } 00228 00229 if(!aws_iot_mqtt_is_client_connected(pClient)) { 00230 FUNC_EXIT_RC(MQTT_CONNECTION_ERROR); 00231 } 00232 00233 rc = aws_iot_shadow_internal_get_request_json(getRequestJsonBuf, MAX_SIZE_CLIENT_TOKEN_CLIENT_SEQUENCE ); 00234 if (AWS_SUCCESS != rc) { 00235 FUNC_EXIT_RC(rc); 00236 } 00237 00238 rc = aws_iot_shadow_internal_action(pThingName, SHADOW_GET, getRequestJsonBuf, MAX_SIZE_CLIENT_TOKEN_CLIENT_SEQUENCE, callback, pContextData, 00239 timeout_seconds, isPersistentSubscribe); 00240 FUNC_EXIT_RC(rc); 00241 } 00242 00243 IoT_Error_t aws_iot_shadow_set_autoreconnect_status(AWS_IoT_Client *pClient, bool newStatus) { 00244 return aws_iot_mqtt_autoreconnect_set_status(pClient, newStatus); 00245 } 00246 00247 #ifdef __cplusplus 00248 } 00249 #endif 00250
Generated on Tue Jul 12 2022 19:02:38 by
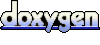