
Changes to enabled on-line compiler
Embed:
(wiki syntax)
Show/hide line numbers
aws_iot_jobs_json.h
Go to the documentation of this file.
00001 /* 00002 * Copyright 2015-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"). 00005 * You may not use this file except in compliance with the License. 00006 * A copy of the License is located at 00007 * 00008 * http://aws.amazon.com/apache2.0 00009 * 00010 * or in the "license" file accompanying this file. This file is distributed 00011 * on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either 00012 * express or implied. See the License for the specific language governing 00013 * permissions and limitations under the License. 00014 */ 00015 00016 /** 00017 * @file aws_iot_jobs_json.h 00018 * @brief Functions for mapping between json and the AWS Iot Job data structures. 00019 */ 00020 00021 #ifdef DISABLE_IOT_JOBS 00022 #error "Jobs API is disabled" 00023 #endif 00024 00025 #ifndef AWS_IOT_JOBS_JSON_H_ 00026 #define AWS_IOT_JOBS_JSON_H_ 00027 00028 #include <stdbool.h> 00029 #include "jsmn.h" 00030 #include "aws_iot_jobs_types.h" 00031 00032 #ifdef __cplusplus 00033 extern "C" { 00034 #endif 00035 00036 /** 00037 * Serialize a job execution update request into a json string. 00038 * 00039 * \param requestBuffer buffer to contain the serialized request. If null 00040 * this function will return the size of the buffer required 00041 * \param bufferSize the size of the buffer. If this is smaller than the required 00042 * length the string will be truncated to fit. 00043 * \request the request to serialize. 00044 * \return The size of the json string to store the serialized request or -1 00045 * if the request is invalid. Note that the return value should be checked against 00046 * the size of the buffer and if its larger handle the fact that the string has 00047 * been truncated. 00048 */ 00049 int aws_iot_jobs_json_serialize_update_job_execution_request( 00050 char *requestBuffer, size_t bufferSize, 00051 const AwsIotJobExecutionUpdateRequest *request); 00052 00053 /** 00054 * Serialize a job API request that contains only a client token. 00055 * 00056 * \param requestBuffer buffer to contain the serialized request. If null 00057 * this function will return the size of the buffer required 00058 * \param bufferSize the size of the buffer. If this is smaller than the required 00059 * length the string will be truncated to fit. 00060 * \param clientToken the client token to use for the request. 00061 * \return The size of the json string to store the serialized request or -1 00062 * if the request is invalid. Note that the return value should be checked against 00063 * the size of the buffer and if its larger handle the fact that the string has 00064 * been truncated. 00065 */ 00066 int aws_iot_jobs_json_serialize_client_token_only_request( 00067 char *requestBuffer, size_t bufferSize, 00068 const char *clientToken); 00069 00070 /** 00071 * Serialize describe job execution request into json string. 00072 * 00073 * \param requestBuffer buffer to contain the serialized request. If null 00074 * this function will return the size of the buffer required 00075 * \param bufferSize the size of the buffer. If this is smaller than the required 00076 * length the string will be truncated to fit. 00077 * \param request the request to serialize. 00078 * \return The size of the json string to store the serialized request or -1 00079 * if the request is invalid. Note that the return value should be checked against 00080 * the size of the buffer and if its larger handle the fact that the string has 00081 * been truncated. 00082 */ 00083 int aws_iot_jobs_json_serialize_describe_job_execution_request( 00084 char *requestBuffer, size_t bufferSize, 00085 const AwsIotDescribeJobExecutionRequest *request); 00086 00087 /** 00088 * Serialize start next job execution request into json string. 00089 * 00090 * \param requestBuffer buffer to contain the serialized request. If null 00091 * this function will return the size of the buffer required 00092 * \param bufferSize the size of the buffer. If this is smaller than the required 00093 * length the string will be truncated to fit. 00094 * \param request the start-next request to serialize. 00095 * \return The size of the json string to store the serialized request or -1 00096 * if the request is invalid. Note that the return value should be checked against 00097 * the size of the buffer and if its larger handle the fact that the string has 00098 * been truncated. 00099 */ 00100 int aws_iot_jobs_json_serialize_start_next_job_execution_request( 00101 char *requestBuffer, size_t bufferSize, 00102 const AwsIotStartNextPendingJobExecutionRequest *request); 00103 00104 #ifdef __cplusplus 00105 } 00106 #endif 00107 00108 #endif /* AWS_IOT_JOBS_JSON_H_ */
Generated on Tue Jul 12 2022 19:02:38 by
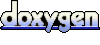