Driver to read and control a serial (i2c) temperature sensor, The Microchip MCP9808 is the digital sensor to control, can be read it, set its resolution, shutdown and also set alarms.
Dependents: Hotboards_temp_alarms Hotboards_temp_fahrenheit Hotboards_temp_reading_temperature LCD_Temperatura
Hotboards_temp.cpp
00001 /* 00002 Hotboards_temp.cpp - Driver to read and control a serial (i2c) temperature sensor, The Microchip 00003 MCP9808 is the digital sensor to control, can be read it, set its resolution, shutdown and also 00004 set alarms. 00005 Hotboards eeprom board (http://hotboards.org) 00006 Created by Diego Perez, March 19, 2016. 00007 Released into the public domain. 00008 */ 00009 #include "Hotboards_temp.h" 00010 00011 #define SENSOR_ADDR (uint8_t)0x3E 00012 #define REG_CONFIG (uint8_t)0x01 00013 #define REG_ALERT_UPPER (uint8_t)0x02 00014 #define REG_ALERT_LOWER (uint8_t)0x03 00015 #define REG_CRITICAL_TEMP (uint8_t)0x04 00016 #define REG_TEMPERATURE (uint8_t)0x05 00017 #define REG_MANU_ID (uint8_t)0x06 00018 #define REG_DEVICE_ID (uint8_t)0x07 00019 #define REG_RESOLUTION (uint8_t)0x08 00020 00021 Hotboards_temp::Hotboards_temp( I2C &i2c, uint8_t address, uint8_t resolution ) 00022 : _i2c(i2c) 00023 { 00024 _address = (address<<1)|0x30; 00025 _resolution = resolution; 00026 } 00027 00028 bool Hotboards_temp::init( void ) 00029 { 00030 bool flag = 0; // lets assume device is not here 00031 uint16_t val = readReg( REG_MANU_ID ); 00032 if( val == 0x0054 ) 00033 { 00034 // device is presence, default set resolution 00035 writeReg( REG_RESOLUTION, _resolution); 00036 // clear configuration register (alarms included) 00037 writeReg( REG_CONFIG, 0x00 ); 00038 flag = 1; 00039 } 00040 return flag; 00041 } 00042 00043 00044 float Hotboards_temp::read( void ) 00045 { 00046 uint16_t val; 00047 float temp; 00048 00049 // read the Ta register 00050 val = readReg( REG_TEMPERATURE ); 00051 00052 // small algorithm to calculate tmeperature in Celcius 00053 // borrowed from https://github.com/adafruit/Adafruit_MCP9808_Library/blob/master/Adafruit_MCP9808.cpp 00054 temp = val & 0x0FFF; 00055 00056 temp /= (float)16.0; 00057 //check if a negative temperature 00058 if( val & 0x1000 ) temp -= 256; 00059 00060 return temp; 00061 } 00062 00063 void Hotboards_temp::setAlarms( float lower, float upper ) 00064 { 00065 // set alarm values 00066 writeAlarm( REG_ALERT_UPPER, upper ); 00067 writeAlarm( REG_CRITICAL_TEMP, upper ); 00068 writeAlarm( REG_ALERT_LOWER, lower ); 00069 00070 uint16_t val = readReg( REG_CONFIG ); 00071 // set alarm only in comparator mode with LAERT pin set to LOW 00072 writeReg( REG_CONFIG, val | 0x0008 ); 00073 } 00074 00075 void Hotboards_temp::disableAlarms( void ) 00076 { 00077 uint16_t val = readReg( REG_CONFIG ); 00078 // just clear the Alert Output Control bit 00079 writeReg( REG_CONFIG, val ^ 0x0008 ); 00080 } 00081 00082 void Hotboards_temp::shutdown( bool state ) 00083 { 00084 uint16_t val = readReg( REG_CONFIG ); 00085 00086 if( state == HT_SENSOR_OFF ) 00087 {// shutdown, curretn under 1uA, and disable convertions 00088 writeReg( REG_CONFIG, val | 0x0100 ); 00089 } 00090 else 00091 {// power on 00092 writeReg( REG_CONFIG, val ^ 0x0100 ); 00093 } 00094 } 00095 00096 void Hotboards_temp::setResolution( uint8_t resolution ) 00097 { 00098 resolution &= 0x03; 00099 writeReg( REG_RESOLUTION, resolution << 8 ); 00100 } 00101 00102 float Hotboards_temp::CelsiusToFarenheit( float celsius ) 00103 { 00104 return celsius * (float)9.0 / (float)5.0 + 32; 00105 } 00106 00107 float Hotboards_temp::FarenheitToCelsius( float farenheit ) 00108 { 00109 return ( farenheit - 32 ) * (float)5.0 / (float)9.0; 00110 } 00111 00112 uint16_t Hotboards_temp::readReg( uint8_t reg ) 00113 { 00114 uint16_t val; 00115 char buffer[2]; 00116 00117 _address &= 0x3E; 00118 buffer[0] = reg; 00119 _i2c.write( _address, buffer, 1, true ); 00120 _address |= 0x01; 00121 _i2c.read( _address, buffer, 2, false ); 00122 00123 val = buffer[0] << 8; 00124 val |= buffer[1]; 00125 00126 return val; 00127 } 00128 00129 void Hotboards_temp::writeReg( uint8_t reg, uint16_t val ) 00130 { 00131 char buffer[3] = { reg, val >> 8, val & 0x00FF }; 00132 _address &= 0x3E; 00133 _i2c.write( _address, buffer, 3 ); 00134 } 00135 00136 void Hotboards_temp::writeAlarm( uint16_t reg, float temp ) 00137 { 00138 uint16_t val = 0x0000; 00139 // check if negative temp 00140 if( temp < 0 ) 00141 { 00142 temp += (float)256.0; 00143 // set sign bit 00144 val = 0x1000; 00145 } 00146 // convert to binary 00147 val |= (uint16_t)( temp *= (float)16.0 ); 00148 writeReg( reg, val ); 00149 }
Generated on Sun Jul 17 2022 03:25:46 by
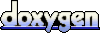