
Example program to test AES-GCM functionality. Used for a workshop
entropy.h File Reference
Entropy accumulator implementation. More...
Go to the source code of this file.
Data Structures | |
struct | source_state |
Entropy source state. More... | |
struct | entropy_context |
Entropy context structure. More... | |
Typedefs | |
typedef int(* | f_source_ptr )(void *data, unsigned char *output, size_t len, size_t *olen) |
Entropy poll callback pointer. | |
Functions | |
void | entropy_init (entropy_context *ctx) |
Initialize the context. | |
void | entropy_free (entropy_context *ctx) |
Free the data in the context. | |
int | entropy_add_source (entropy_context *ctx, f_source_ptr f_source, void *p_source, size_t threshold) |
Adds an entropy source to poll (Thread-safe if POLARSSL_THREADING_C is enabled) | |
int | entropy_gather (entropy_context *ctx) |
Trigger an extra gather poll for the accumulator (Thread-safe if POLARSSL_THREADING_C is enabled) | |
int | entropy_func (void *data, unsigned char *output, size_t len) |
Retrieve entropy from the accumulator (Maximum length: ENTROPY_BLOCK_SIZE) (Thread-safe if POLARSSL_THREADING_C is enabled) | |
int | entropy_update_manual (entropy_context *ctx, const unsigned char *data, size_t len) |
Add data to the accumulator manually (Thread-safe if POLARSSL_THREADING_C is enabled) | |
int | entropy_write_seed_file (entropy_context *ctx, const char *path) |
Write a seed file. | |
int | entropy_update_seed_file (entropy_context *ctx, const char *path) |
Read and update a seed file. |
Detailed Description
Entropy accumulator implementation.
Copyright (C) 2006-2014, Brainspark B.V.
This file is part of PolarSSL (http://www.polarssl.org) Lead Maintainer: Paul Bakker <polarssl_maintainer at polarssl.org>
All rights reserved.
This program is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation; either version 2 of the License, or (at your option) any later version.
This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details.
You should have received a copy of the GNU General Public License along with this program; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
Definition in file entropy.h.
Typedef Documentation
typedef int(* f_source_ptr)(void *data, unsigned char *output, size_t len, size_t *olen) |
Entropy poll callback pointer.
- Parameters:
-
data Callback-specific data pointer output Data to fill len Maximum size to provide olen The actual amount of bytes put into the buffer (Can be 0)
- Returns:
- 0 if no critical failures occurred, POLARSSL_ERR_ENTROPY_SOURCE_FAILED otherwise
Function Documentation
int entropy_add_source | ( | entropy_context * | ctx, |
f_source_ptr | f_source, | ||
void * | p_source, | ||
size_t | threshold | ||
) |
Adds an entropy source to poll (Thread-safe if POLARSSL_THREADING_C is enabled)
- Parameters:
-
ctx Entropy context f_source Entropy function p_source Function data threshold Minimum required from source before entropy is released ( with entropy_func() )
- Returns:
- 0 if successful or POLARSSL_ERR_ENTROPY_MAX_SOURCES
void entropy_free | ( | entropy_context * | ctx ) |
int entropy_func | ( | void * | data, |
unsigned char * | output, | ||
size_t | len | ||
) |
Retrieve entropy from the accumulator (Maximum length: ENTROPY_BLOCK_SIZE) (Thread-safe if POLARSSL_THREADING_C is enabled)
- Parameters:
-
data Entropy context output Buffer to fill len Length of buffer
- Returns:
- 0 if successful, or POLARSSL_ERR_ENTROPY_SOURCE_FAILED
int entropy_gather | ( | entropy_context * | ctx ) |
void entropy_init | ( | entropy_context * | ctx ) |
int entropy_update_manual | ( | entropy_context * | ctx, |
const unsigned char * | data, | ||
size_t | len | ||
) |
int entropy_update_seed_file | ( | entropy_context * | ctx, |
const char * | path | ||
) |
Read and update a seed file.
Seed is added to this instance. No more than ENTROPY_MAX_SEED_SIZE bytes are read from the seed file. The rest is ignored.
- Parameters:
-
ctx Entropy context path Name of the file
- Returns:
- 0 if successful, POLARSSL_ERR_ENTROPY_FILE_IO_ERROR on file error, POLARSSL_ERR_ENTROPY_SOURCE_FAILED
int entropy_write_seed_file | ( | entropy_context * | ctx, |
const char * | path | ||
) |
Generated on Tue Jul 12 2022 19:40:22 by
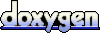