
Example program to test AES-GCM functionality. Used for a workshop
Embed:
(wiki syntax)
Show/hide line numbers
compat-1.2.h
Go to the documentation of this file.
00001 /** 00002 * \file compat-1.2.h 00003 * 00004 * \brief Backwards compatibility header for PolarSSL-1.2 from PolarSSL-1.3 00005 * 00006 * Copyright (C) 2006-2013, Brainspark B.V. 00007 * 00008 * This file is part of PolarSSL (http://www.polarssl.org) 00009 * Lead Maintainer: Paul Bakker <polarssl_maintainer at polarssl.org> 00010 * 00011 * All rights reserved. 00012 * 00013 * This program is free software; you can redistribute it and/or modify 00014 * it under the terms of the GNU General Public License as published by 00015 * the Free Software Foundation; either version 2 of the License, or 00016 * (at your option) any later version. 00017 * 00018 * This program is distributed in the hope that it will be useful, 00019 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00020 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00021 * GNU General Public License for more details. 00022 * 00023 * You should have received a copy of the GNU General Public License along 00024 * with this program; if not, write to the Free Software Foundation, Inc., 00025 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00026 */ 00027 #ifndef POLARSSL_COMPAT_1_2_H 00028 #define POLARSSL_COMPAT_1_2_H 00029 00030 #if !defined(POLARSSL_CONFIG_FILE) 00031 #include "config.h" 00032 #else 00033 #include POLARSSL_CONFIG_FILE 00034 #endif 00035 00036 // Comment out to disable prototype change warnings 00037 #define SHOW_PROTOTYPE_CHANGE_WARNINGS 00038 00039 #if defined(_MSC_VER) && !defined(inline) 00040 #define inline _inline 00041 #else 00042 #if defined(__ARMCC_VERSION) && !defined(inline) 00043 #define inline __inline 00044 #endif /* __ARMCC_VERSION */ 00045 #endif /* _MSC_VER */ 00046 00047 #if defined(_MSC_VER) 00048 // MSVC does not support #warning 00049 #undef SHOW_PROTOTYPE_CHANGE_WARNINGS 00050 #endif 00051 00052 #if defined(SHOW_PROTOTYPE_CHANGE_WARNINGS) 00053 #warning "You can disable these warnings by commenting SHOW_PROTOTYPE_CHANGE_WARNINGS in compat-1.2.h" 00054 #endif 00055 00056 #if defined(POLARSSL_SHA256_C) 00057 #define POLARSSL_SHA2_C 00058 #include "sha256.h" 00059 00060 /* 00061 * SHA-2 -> SHA-256 00062 */ 00063 typedef sha256_context sha2_context; 00064 00065 static inline void sha2_starts( sha256_context *ctx, int is224 ) { 00066 sha256_starts( ctx, is224 ); 00067 } 00068 static inline void sha2_update( sha256_context *ctx, const unsigned char *input, 00069 size_t ilen ) { 00070 sha256_update( ctx, input, ilen ); 00071 } 00072 static inline void sha2_finish( sha256_context *ctx, unsigned char output[32] ) { 00073 sha256_finish( ctx, output ); 00074 } 00075 static inline int sha2_file( const char *path, unsigned char output[32], int is224 ) { 00076 return sha256_file( path, output, is224 ); 00077 } 00078 static inline void sha2( const unsigned char *input, size_t ilen, 00079 unsigned char output[32], int is224 ) { 00080 sha256( input, ilen, output, is224 ); 00081 } 00082 static inline void sha2_hmac_starts( sha256_context *ctx, const unsigned char *key, 00083 size_t keylen, int is224 ) { 00084 sha256_hmac_starts( ctx, key, keylen, is224 ); 00085 } 00086 static inline void sha2_hmac_update( sha256_context *ctx, const unsigned char *input, size_t ilen ) { 00087 sha256_hmac_update( ctx, input, ilen ); 00088 } 00089 static inline void sha2_hmac_finish( sha256_context *ctx, unsigned char output[32] ) { 00090 sha256_hmac_finish( ctx, output ); 00091 } 00092 static inline void sha2_hmac_reset( sha256_context *ctx ) { 00093 sha256_hmac_reset( ctx ); 00094 } 00095 static inline void sha2_hmac( const unsigned char *key, size_t keylen, 00096 const unsigned char *input, size_t ilen, 00097 unsigned char output[32], int is224 ) { 00098 sha256_hmac( key, keylen, input, ilen, output, is224 ); 00099 } 00100 static inline int sha2_self_test( int verbose ) { 00101 return sha256_self_test( verbose ); 00102 } 00103 #endif /* POLARSSL_SHA256_C */ 00104 00105 #if defined(POLARSSL_SHA512_C) 00106 #define POLARSSL_SHA4_C 00107 #include "sha512.h" 00108 00109 /* 00110 * SHA-4 -> SHA-512 00111 */ 00112 typedef sha512_context sha4_context; 00113 00114 static inline void sha4_starts( sha512_context *ctx, int is384 ) { 00115 sha512_starts( ctx, is384 ); 00116 } 00117 static inline void sha4_update( sha512_context *ctx, const unsigned char *input, 00118 size_t ilen ) { 00119 sha512_update( ctx, input, ilen ); 00120 } 00121 static inline void sha4_finish( sha512_context *ctx, unsigned char output[64] ) { 00122 sha512_finish( ctx, output ); 00123 } 00124 static inline int sha4_file( const char *path, unsigned char output[64], int is384 ) { 00125 return sha512_file( path, output, is384 ); 00126 } 00127 static inline void sha4( const unsigned char *input, size_t ilen, 00128 unsigned char output[32], int is384 ) { 00129 sha512( input, ilen, output, is384 ); 00130 } 00131 static inline void sha4_hmac_starts( sha512_context *ctx, const unsigned char *key, 00132 size_t keylen, int is384 ) { 00133 sha512_hmac_starts( ctx, key, keylen, is384 ); 00134 } 00135 static inline void sha4_hmac_update( sha512_context *ctx, const unsigned char *input, size_t ilen ) { 00136 sha512_hmac_update( ctx, input, ilen ); 00137 } 00138 static inline void sha4_hmac_finish( sha512_context *ctx, unsigned char output[64] ) { 00139 sha512_hmac_finish( ctx, output ); 00140 } 00141 static inline void sha4_hmac_reset( sha512_context *ctx ) { 00142 sha512_hmac_reset( ctx ); 00143 } 00144 static inline void sha4_hmac( const unsigned char *key, size_t keylen, 00145 const unsigned char *input, size_t ilen, 00146 unsigned char output[64], int is384 ) { 00147 sha512_hmac( key, keylen, input, ilen, output, is384 ); 00148 } 00149 static inline int sha4_self_test( int verbose ) { 00150 return sha512_self_test( verbose ); 00151 } 00152 #endif /* POLARSSL_SHA512_C */ 00153 00154 #if defined(POLARSSL_CIPHER_C) 00155 #if defined(SHOW_PROTOTYPE_CHANGE_WARNINGS) 00156 #warning "cipher_reset() prototype changed. Manual change required if used" 00157 #endif 00158 #endif 00159 00160 #if defined(POLARSSL_RSA_C) 00161 #define SIG_RSA_RAW POLARSSL_MD_NONE 00162 #define SIG_RSA_MD2 POLARSSL_MD_MD2 00163 #define SIG_RSA_MD4 POLARSSL_MD_MD4 00164 #define SIG_RSA_MD5 POLARSSL_MD_MD5 00165 #define SIG_RSA_SHA1 POLARSSL_MD_SHA1 00166 #define SIG_RSA_SHA224 POLARSSL_MD_SHA224 00167 #define SIG_RSA_SHA256 POLARSSL_MD_SHA256 00168 #define SIG_RSA_SHA384 POLARSSL_MD_SHA384 00169 #define SIG_RSA_SHA512 POLARSSL_MD_SHA512 00170 #if defined(SHOW_PROTOTYPE_CHANGE_WARNINGS) 00171 #warning "rsa_pkcs1_verify() prototype changed. Manual change required if used" 00172 #warning "rsa_pkcs1_decrypt() prototype changed. Manual change required if used" 00173 #endif 00174 #endif /* POLARSSL_RSA_C */ 00175 00176 #if defined(POLARSSL_DHM_C) 00177 #if defined(SHOW_PROTOTYPE_CHANGE_WARNINGS) 00178 #warning "dhm_calc_secret() prototype changed. Manual change required if used" 00179 #endif 00180 #endif 00181 00182 #if defined(POLARSSL_GCM_C) 00183 #if defined(SHOW_PROTOTYPE_CHANGE_WARNINGS) 00184 #warning "gcm_init() prototype changed. Manual change required if used" 00185 #endif 00186 #endif 00187 00188 #if defined(POLARSSL_SSL_CLI_C) 00189 #if defined(SHOW_PROTOTYPE_CHANGE_WARNINGS) 00190 #warning "ssl_set_own_cert() prototype changed. Change to ssl_set_own_cert_rsa(). Manual change required if used" 00191 #endif 00192 #endif 00193 00194 #if defined(POLARSSL_X509_USE_C) || defined(POLARSSL_X509_CREATE_C) 00195 #include "x509.h" 00196 00197 #define POLARSSL_ERR_X509_CERT_INVALID_FORMAT POLARSSL_ERR_X509_INVALID_FORMAT 00198 #define POLARSSL_ERR_X509_CERT_INVALID_VERSION POLARSSL_ERR_X509_INVALID_VERSION 00199 #define POLARSSL_ERR_X509_CERT_INVALID_ALG POLARSSL_ERR_X509_INVALID_ALG 00200 #define POLARSSL_ERR_X509_CERT_UNKNOWN_SIG_ALG POLARSSL_ERR_X509_UNKNOWN_SIG_ALG 00201 #define POLARSSL_ERR_X509_CERT_INVALID_NAME POLARSSL_ERR_X509_INVALID_NAME 00202 #define POLARSSL_ERR_X509_CERT_INVALID_DATE POLARSSL_ERR_X509_INVALID_DATE 00203 #define POLARSSL_ERR_X509_CERT_INVALID_EXTENSIONS POLARSSL_ERR_X509_INVALID_EXTENSIONS 00204 #define POLARSSL_ERR_X509_CERT_SIG_MISMATCH POLARSSL_ERR_X509_SIG_MISMATCH 00205 #define POLARSSL_ERR_X509_CERT_INVALID_SIGNATURE POLARSSL_ERR_X509_INVALID_SIGNATURE 00206 #define POLARSSL_ERR_X509_CERT_INVALID_SERIAL POLARSSL_ERR_X509_INVALID_SERIAL 00207 #define POLARSSL_ERR_X509_CERT_UNKNOWN_VERSION POLARSSL_ERR_X509_UNKNOWN_VERSION 00208 00209 static inline int x509parse_serial_gets( char *buf, size_t size, const x509_buf *serial ) { 00210 return x509_serial_gets( buf, size, serial ); 00211 } 00212 static inline int x509parse_dn_gets( char *buf, size_t size, const x509_name *dn ) { 00213 return x509_dn_gets( buf, size, dn ); 00214 } 00215 static inline int x509parse_time_expired( const x509_time *time ) { 00216 return x509_time_expired( time ); 00217 } 00218 #endif /* POLARSSL_X509_USE_C || POLARSSL_X509_CREATE_C */ 00219 00220 #if defined(POLARSSL_X509_CRT_PARSE_C) 00221 #define POLARSSL_X509_PARSE_C 00222 #include "x509_crt.h" 00223 typedef x509_crt x509_cert; 00224 00225 static inline int x509parse_crt_der( x509_cert *chain, const unsigned char *buf, 00226 size_t buflen ) { 00227 return x509_crt_parse_der( chain, buf, buflen ); 00228 } 00229 static inline int x509parse_crt( x509_cert *chain, const unsigned char *buf, size_t buflen ) { 00230 return x509_crt_parse( chain, buf, buflen ); 00231 } 00232 static inline int x509parse_crtfile( x509_cert *chain, const char *path ) { 00233 return x509_crt_parse_file( chain, path ); 00234 } 00235 static inline int x509parse_crtpath( x509_cert *chain, const char *path ) { 00236 return x509_crt_parse_path( chain, path ); 00237 } 00238 static inline int x509parse_cert_info( char *buf, size_t size, const char *prefix, 00239 const x509_cert *crt ) { 00240 return x509_crt_info( buf, size, prefix, crt ); 00241 } 00242 static inline int x509parse_verify( x509_cert *crt, x509_cert *trust_ca, 00243 x509_crl *ca_crl, const char *cn, int *flags, 00244 int (*f_vrfy)(void *, x509_cert *, int, int *), 00245 void *p_vrfy ) { 00246 return x509_crt_verify( crt, trust_ca, ca_crl, cn, flags, f_vrfy, p_vrfy ); 00247 } 00248 static inline int x509parse_revoked( const x509_cert *crt, const x509_crl *crl ) { 00249 return x509_crt_revoked( crt, crl ); 00250 } 00251 static inline void x509_free( x509_cert *crt ) { 00252 x509_crt_free( crt ); 00253 } 00254 #endif /* POLARSSL_X509_CRT_PARSE_C */ 00255 00256 #if defined(POLARSSL_X509_CRL_PARSE_C) 00257 #define POLARSSL_X509_PARSE_C 00258 #include "x509_crl.h" 00259 static inline int x509parse_crl( x509_crl *chain, const unsigned char *buf, size_t buflen ) { 00260 return x509_crl_parse( chain, buf, buflen ); 00261 } 00262 static inline int x509parse_crlfile( x509_crl *chain, const char *path ) { 00263 return x509_crl_parse_file( chain, path ); 00264 } 00265 static inline int x509parse_crl_info( char *buf, size_t size, const char *prefix, 00266 const x509_crl *crl ) { 00267 return x509_crl_info( buf, size, prefix, crl ); 00268 } 00269 #endif /* POLARSSL_X509_CRL_PARSE_C */ 00270 00271 #if defined(POLARSSL_X509_CSR_PARSE_C) 00272 #define POLARSSL_X509_PARSE_C 00273 #include "x509_csr.h" 00274 static inline int x509parse_csr( x509_csr *csr, const unsigned char *buf, size_t buflen ) { 00275 return x509_csr_parse( csr, buf, buflen ); 00276 } 00277 static inline int x509parse_csrfile( x509_csr *csr, const char *path ) { 00278 return x509_csr_parse_file( csr, path ); 00279 } 00280 static inline int x509parse_csr_info( char *buf, size_t size, const char *prefix, 00281 const x509_csr *csr ) { 00282 return x509_csr_info( buf, size, prefix, csr ); 00283 } 00284 #endif /* POLARSSL_X509_CSR_PARSE_C */ 00285 00286 #if defined(POLARSSL_SSL_TLS_C) 00287 #include "ssl_ciphersuites.h" 00288 00289 #define ssl_default_ciphersuites ssl_list_ciphersuites() 00290 #endif 00291 00292 #if defined(POLARSSL_PK_PARSE_C) && defined(POLARSSL_RSA_C) 00293 #include "rsa.h" 00294 #include "pk.h" 00295 00296 #define POLARSSL_ERR_X509_PASSWORD_MISMATCH POLARSSL_ERR_PK_PASSWORD_MISMATCH 00297 #define POLARSSL_ERR_X509_KEY_INVALID_FORMAT POLARSSL_ERR_PK_KEY_INVALID_FORMAT 00298 #define POLARSSL_ERR_X509_UNKNOWN_PK_ALG POLARSSL_ERR_PK_UNKNOWN_PK_ALG 00299 #define POLARSSL_ERR_X509_CERT_INVALID_PUBKEY POLARSSL_ERR_PK_INVALID_PUBKEY 00300 00301 #if defined(POLARSSL_FS_IO) 00302 static inline int x509parse_keyfile( rsa_context *rsa, const char *path, 00303 const char *pwd ) { 00304 int ret; 00305 pk_context pk; 00306 pk_init( &pk ); 00307 ret = pk_parse_keyfile( &pk, path, pwd ); 00308 if( ret == 0 && ! pk_can_do( &pk, POLARSSL_PK_RSA ) ) 00309 ret = POLARSSL_ERR_PK_TYPE_MISMATCH; 00310 if( ret == 0 ) 00311 rsa_copy( rsa, pk_rsa( pk ) ); 00312 else 00313 rsa_free( rsa ); 00314 pk_free( &pk ); 00315 return( ret ); 00316 } 00317 static inline int x509parse_public_keyfile( rsa_context *rsa, const char *path ) { 00318 int ret; 00319 pk_context pk; 00320 pk_init( &pk ); 00321 ret = pk_parse_public_keyfile( &pk, path ); 00322 if( ret == 0 && ! pk_can_do( &pk, POLARSSL_PK_RSA ) ) 00323 ret = POLARSSL_ERR_PK_TYPE_MISMATCH; 00324 if( ret == 0 ) 00325 rsa_copy( rsa, pk_rsa( pk ) ); 00326 else 00327 rsa_free( rsa ); 00328 pk_free( &pk ); 00329 return( ret ); 00330 } 00331 #endif /* POLARSSL_FS_IO */ 00332 00333 static inline int x509parse_key( rsa_context *rsa, const unsigned char *key, 00334 size_t keylen, 00335 const unsigned char *pwd, size_t pwdlen ) { 00336 int ret; 00337 pk_context pk; 00338 pk_init( &pk ); 00339 ret = pk_parse_key( &pk, key, keylen, pwd, pwdlen ); 00340 if( ret == 0 && ! pk_can_do( &pk, POLARSSL_PK_RSA ) ) 00341 ret = POLARSSL_ERR_PK_TYPE_MISMATCH; 00342 if( ret == 0 ) 00343 rsa_copy( rsa, pk_rsa( pk ) ); 00344 else 00345 rsa_free( rsa ); 00346 pk_free( &pk ); 00347 return( ret ); 00348 } 00349 00350 static inline int x509parse_public_key( rsa_context *rsa, 00351 const unsigned char *key, size_t keylen ) 00352 { 00353 int ret; 00354 pk_context pk; 00355 pk_init( &pk ); 00356 ret = pk_parse_public_key( &pk, key, keylen ); 00357 if( ret == 0 && ! pk_can_do( &pk, POLARSSL_PK_RSA ) ) 00358 ret = POLARSSL_ERR_PK_TYPE_MISMATCH; 00359 if( ret == 0 ) 00360 rsa_copy( rsa, pk_rsa( pk ) ); 00361 else 00362 rsa_free( rsa ); 00363 pk_free( &pk ); 00364 return( ret ); 00365 } 00366 #endif /* POLARSSL_PK_PARSE_C && POLARSSL_RSA_C */ 00367 00368 #if defined(POLARSSL_PK_WRITE_C) && defined(POLARSSL_RSA_C) 00369 #include "pk.h" 00370 static inline int x509_write_pubkey_der( unsigned char *buf, size_t len, rsa_context *rsa ) { 00371 int ret; 00372 pk_context ctx; 00373 if( ( ret = pk_init_ctx( &ctx, pk_info_from_type( POLARSSL_PK_RSA ) ) ) != 0 ) return( ret ); 00374 if( ( ret = rsa_copy( pk_rsa( ctx ), rsa ) ) != 0 ) return( ret ); 00375 ret = pk_write_pubkey_der( &ctx, buf, len ); 00376 pk_free( &ctx ); 00377 return( ret ); 00378 } 00379 static inline int x509_write_key_der( unsigned char *buf, size_t len, rsa_context *rsa ) { 00380 int ret; 00381 pk_context ctx; 00382 if( ( ret = pk_init_ctx( &ctx, pk_info_from_type( POLARSSL_PK_RSA ) ) ) != 0 ) return( ret ); 00383 if( ( ret = rsa_copy( pk_rsa( ctx ), rsa ) ) != 0 ) return( ret ); 00384 ret = pk_write_key_der( &ctx, buf, len ); 00385 pk_free( &ctx ); 00386 return( ret ); 00387 } 00388 #endif /* POLARSSL_PK_WRITE_C && POLARSSL_RSA_C */ 00389 #endif /* compat-1.2.h */ 00390 00391
Generated on Tue Jul 12 2022 19:40:15 by
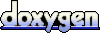